![]() |
OpenNI 1.5.4
|
XnContext.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XN_CONTEXT_H__ 00023 #define __XN_CONTEXT_H__ 00024 00078 //--------------------------------------------------------------------------- 00079 // Includes 00080 //--------------------------------------------------------------------------- 00081 #include <XnTypes.h> 00082 #include <XnQueries.h> 00083 #include <XnPrdNodeInfoList.h> 00084 00085 //--------------------------------------------------------------------------- 00086 // Functions 00087 //--------------------------------------------------------------------------- 00088 00101 XN_C_API XnStatus XN_C_DECL xnInit(XnContext** ppContext); 00102 00112 XN_C_API XnStatus XN_C_DECL xnContextRunXmlScriptFromFileEx(XnContext* pContext, const XnChar* strFileName, XnEnumerationErrors* pErrors, XnNodeHandle* phScriptNode); 00113 00125 XN_C_API XnStatus XN_API_DEPRECATED("Please use xnContextRunXmlScriptFromFileEx() instead") xnContextRunXmlScriptFromFile(XnContext* pContext, const XnChar* strFileName, XnEnumerationErrors* pErrors); 00126 00136 XN_C_API XnStatus XN_C_DECL xnContextRunXmlScriptEx(XnContext* pContext, const XnChar* xmlScript, XnEnumerationErrors* pErrors, XnNodeHandle* phScriptNode); 00137 00149 XN_C_API XnStatus XN_API_DEPRECATED("Please use xnContextRunXmlScriptEx() instead") XN_C_DECL xnContextRunXmlScript(XnContext* pContext, const XnChar* xmlScript, XnEnumerationErrors* pErrors); 00150 00160 XN_C_API XnStatus XN_C_DECL xnInitFromXmlFileEx(const XnChar* strFileName, XnContext** ppContext, XnEnumerationErrors* pErrors, XnNodeHandle* phScriptNode); 00161 00173 XN_C_API XnStatus XN_API_DEPRECATED("Please use xnInitFromXmlFileEx() instead") XN_C_DECL xnInitFromXmlFile(const XnChar* strFileName, XnContext** ppContext, XnEnumerationErrors* pErrors); 00174 00182 XN_C_API XnStatus XN_C_DECL xnContextOpenFileRecordingEx(XnContext* pContext, const XnChar* strFileName, XnNodeHandle* phPlayerNode); 00183 00194 XN_C_API XnStatus XN_API_DEPRECATED("Please use xnContextOpenFileRecordingEx() instead") XN_C_DECL xnContextOpenFileRecording(XnContext* pContext, const XnChar* strFileName); 00195 00201 XN_C_API XnStatus XN_C_DECL xnContextAddRef(XnContext* pContext); 00202 00209 XN_C_API void XN_C_DECL xnContextRelease(XnContext* pContext); 00210 00219 XN_C_API void XN_API_DEPRECATED("Use xnContextRelease() instead") XN_C_DECL xnShutdown(XnContext* pContext); 00220 00227 XN_C_API void XN_C_DECL xnForceShutdown(XnContext* pContext); 00228 00233 XN_C_API XnStatus XN_C_DECL xnContextRegisterForShutdown(XnContext* pContext, XnContextShuttingDownHandler pHandler, void* pCookie, XnCallbackHandle* phCallback); 00234 00239 XN_C_API void XN_C_DECL xnContextUnregisterFromShutdown(XnContext* pContext, XnCallbackHandle hCallback); 00240 00241 // @} 00242 00258 XN_C_API XnStatus XN_C_DECL xnEnumerateProductionTrees( 00259 XnContext* pContext, 00260 XnProductionNodeType Type, 00261 const XnNodeQuery* pQuery, 00262 XnNodeInfoList** ppTreesList, 00263 XnEnumerationErrors* pErrors 00264 ); 00265 00274 XN_C_API XnStatus XN_C_DECL xnCreateProductionTree( 00275 XnContext* pContext, 00276 XnNodeInfo* pTree, 00277 XnNodeHandle* phNode 00278 ); 00279 00291 XN_C_API XnStatus XN_C_DECL xnCreateAnyProductionTree( 00292 XnContext* pContext, 00293 XnProductionNodeType type, 00294 XnNodeQuery* pQuery, 00295 XnNodeHandle* phNode, 00296 XnEnumerationErrors* pErrors 00297 ); 00298 00310 XN_C_API XnStatus XN_C_DECL xnCreateMockNode( 00311 XnContext* pContext, 00312 XnProductionNodeType type, 00313 const XnChar* strName, 00314 XnNodeHandle* phNode 00315 ); 00316 00328 XN_C_API XnStatus XN_C_DECL xnCreateMockNodeBasedOn( 00329 XnContext* pContext, 00330 XnNodeHandle hOriginalNode, 00331 const XnChar* strName, 00332 XnNodeHandle* phMockNode 00333 ); 00334 00340 XN_C_API XnStatus XN_C_DECL xnProductionNodeAddRef(XnNodeHandle hNode); 00341 00348 XN_C_API XnStatus XN_API_DEPRECATED("Please use xnProductionNodeAddRef() instead.") XN_C_DECL xnRefProductionNode(XnNodeHandle hNode); 00349 00356 XN_C_API void XN_C_DECL xnProductionNodeRelease(XnNodeHandle hNode); 00357 00365 XN_C_API void XN_API_DEPRECATED("Please use xnProductionNodeRelease() instead.") XN_C_DECL xnUnrefProductionNode(XnNodeHandle hNode); 00366 00374 XN_C_API XnStatus XN_C_DECL xnEnumerateExistingNodes(XnContext* pContext, XnNodeInfoList** ppList); 00375 00384 XN_C_API XnStatus XN_C_DECL xnEnumerateExistingNodesByType(XnContext* pContext, XnProductionNodeType type, XnNodeInfoList** ppList); 00385 00393 XN_C_API XnStatus XN_C_DECL xnFindExistingRefNodeByType( 00394 XnContext* pContext, 00395 XnProductionNodeType type, 00396 XnNodeHandle* phNode 00397 ); 00398 00399 XN_C_API XnStatus XN_API_DEPRECATED("Please use xnFindExistingRefNodeByType() instead") XN_C_DECL xnFindExistingNodeByType( 00400 XnContext* pContext, 00401 XnProductionNodeType type, 00402 XnNodeHandle* phNode 00403 ); 00404 00414 XN_C_API XnStatus XN_C_DECL xnGetRefNodeHandleByName( 00415 XnContext* pContext, 00416 const XnChar* strInstanceName, 00417 XnNodeHandle* phNode 00418 ); 00419 00420 XN_C_API XnStatus XN_API_DEPRECATED("Please use xnGetRefNodeHandleByName() instead") XN_C_DECL xnGetNodeHandleByName( 00421 XnContext* pContext, 00422 const XnChar* strInstanceName, 00423 XnNodeHandle* phNode 00424 ); 00425 00426 // @} 00427 00438 XN_C_API XnStatus XN_C_DECL xnWaitAndUpdateAll(XnContext* pContext); 00439 00446 XN_C_API XnStatus XN_C_DECL xnWaitOneUpdateAll(XnContext* pContext, XnNodeHandle hNode); 00447 00453 XN_C_API XnStatus XN_C_DECL xnWaitAnyUpdateAll(XnContext* pContext); 00454 00461 XN_C_API XnStatus XN_C_DECL xnWaitNoneUpdateAll(XnContext* pContext); 00462 00468 XN_C_API XnStatus XN_C_DECL xnStartGeneratingAll(XnContext* pContext); 00469 00475 XN_C_API XnStatus XN_C_DECL xnStopGeneratingAll(XnContext* pContext); 00476 00484 XN_C_API XnStatus XN_C_DECL xnSetGlobalMirror(XnContext* pContext, XnBool bMirror); 00485 00491 XN_C_API XnBool XN_C_DECL xnGetGlobalMirror(XnContext* pContext); 00492 00500 XN_C_API XnStatus XN_C_DECL xnGetGlobalErrorState(XnContext* pContext); 00501 00510 XN_C_API XnStatus XN_C_DECL xnRegisterToGlobalErrorStateChange 00511 (XnContext* pContext, XnErrorStateChangedHandler handler, 00512 void* pCookie, XnCallbackHandle* phCallback); 00513 00520 XN_C_API void XN_C_DECL xnUnregisterFromGlobalErrorStateChange 00521 (XnContext* pContext, XnCallbackHandle hCallback); 00522 00531 XN_C_API XnStatus XN_C_DECL xnRegisterToNodeCreation 00532 (XnContext* pContext, XnNodeCreationHandler handler, 00533 void* pCookie, XnCallbackHandle* phCallback); 00534 00541 XN_C_API void XN_C_DECL xnUnregisterFromNodeCreation 00542 (XnContext* pContext, XnCallbackHandle hCallback); 00543 00552 XN_C_API XnStatus XN_C_DECL xnRegisterToNodeDestruction 00553 (XnContext* pContext, XnNodeDestructionHandler handler, 00554 void* pCookie, XnCallbackHandle* phCallback); 00555 00562 XN_C_API void XN_C_DECL xnUnregisterFromNodeDestruction 00563 (XnContext* pContext, XnCallbackHandle hCallback); 00564 00566 00569 #endif // __XN_CONTEXT_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
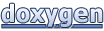