![]() |
OpenNI 1.5.4
|
XnBitSet.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XNBITSET_H__ 00023 #define __XNBITSET_H__ 00024 00025 #include <XnArray.h> 00026 00027 class XnBitSet 00028 { 00029 public: 00030 XnBitSet() : m_nSize(0) {} 00031 00034 XnStatus Reserve(XnUInt32 nBits) 00035 { 00036 return m_array.Reserve((nBits >> 5) + 1); 00037 } 00038 00040 XnStatus SetSize(XnUInt32 nBits) 00041 { 00042 return m_array.SetSize((nBits >> 5) + 1, 0); 00043 } 00044 00046 XnStatus Set(XnUInt32 nIndex, XnBool bValue) 00047 { 00048 XnUInt32 nArrayIndex = (nIndex >> 5); 00049 XnUInt32 nMask = (1 << ((~nIndex) & 0x1F)); 00050 XnUInt32 nOldVal = nArrayIndex < m_array.GetSize() ? m_array[nArrayIndex] : 0; 00051 XnUInt32 nNewVal = bValue ? (nOldVal | nMask) : (nOldVal & (~nMask)); 00052 XnStatus nRetVal = m_array.Set(nArrayIndex, nNewVal, 0); 00053 XN_IS_STATUS_OK(nRetVal); 00054 m_nSize = XN_MAX(m_nSize, nIndex + 1); 00055 return XN_STATUS_OK; 00056 } 00057 00059 XnBool IsSet(XnUInt32 nIndex) const 00060 { 00061 XnUInt32 nArrayIndex = (nIndex >> 5); 00062 if (nArrayIndex >= m_array.GetSize()) 00063 { 00064 return FALSE; 00065 } 00066 return (m_array[nArrayIndex] & (1 << ((~nIndex) & 0x1F))) ? TRUE : FALSE; 00067 } 00068 00070 XnStatus SetData(const XnUInt32* pData, XnUInt32 nSizeInDwords) 00071 { 00072 XnStatus nRetVal = m_array.SetData(pData, nSizeInDwords); 00073 XN_IS_STATUS_OK(nRetVal); 00074 m_nSize = (nSizeInDwords << 5); 00075 return XN_STATUS_OK; 00076 } 00077 00079 XnStatus SetDataBytes(const XnUInt8* pData, XnUInt32 nSizeInBytes) 00080 { 00081 //XnStatus nRetVal = m_array.SetData(reinterpret_cast<const XnUInt32*>(pData), XN_MAX(1, nSizeInBytes >> 2)); 00082 XnUInt32 nSizeInDwords = XN_MAX(1, nSizeInBytes >> 2); 00083 XnStatus nRetVal = m_array.SetSize(nSizeInDwords); 00084 XN_IS_STATUS_OK(nRetVal); 00085 for (XnUInt32 nDwordIdx = 0, nByteIdx = 0; nDwordIdx < nSizeInDwords; nDwordIdx++, nByteIdx += 4) 00086 { 00087 m_array[nDwordIdx] = ((pData[nByteIdx] << 24) | (pData[nByteIdx + 1] << 16) | (pData[nByteIdx + 2] << 8) | pData[nByteIdx + 3] ); 00088 } 00089 m_nSize = (nSizeInBytes << 3); 00090 return XN_STATUS_OK; 00091 } 00092 00094 const XnUInt32* GetData() const 00095 { 00096 return m_array.GetData(); 00097 } 00098 00100 XnUInt32* GetData() 00101 { 00102 return m_array.GetData(); 00103 } 00104 00106 XnUInt32 GetDataSize() const 00107 { 00108 return m_array.GetSize(); 00109 } 00110 00112 XnUInt32 GetSize() const 00113 { 00114 return m_nSize; 00115 } 00116 00118 void Clear() 00119 { 00120 m_array.Clear(); 00121 m_nSize = 0; 00122 } 00123 00125 XnBool IsEmpty() const 00126 { 00127 return m_array.IsEmpty(); 00128 } 00129 00130 private: 00131 XnArray<XnUInt32> m_array; 00132 XnUInt32 m_nSize; 00133 }; 00134 00135 #endif // __XNBITSET_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
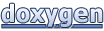