动画(Animation)
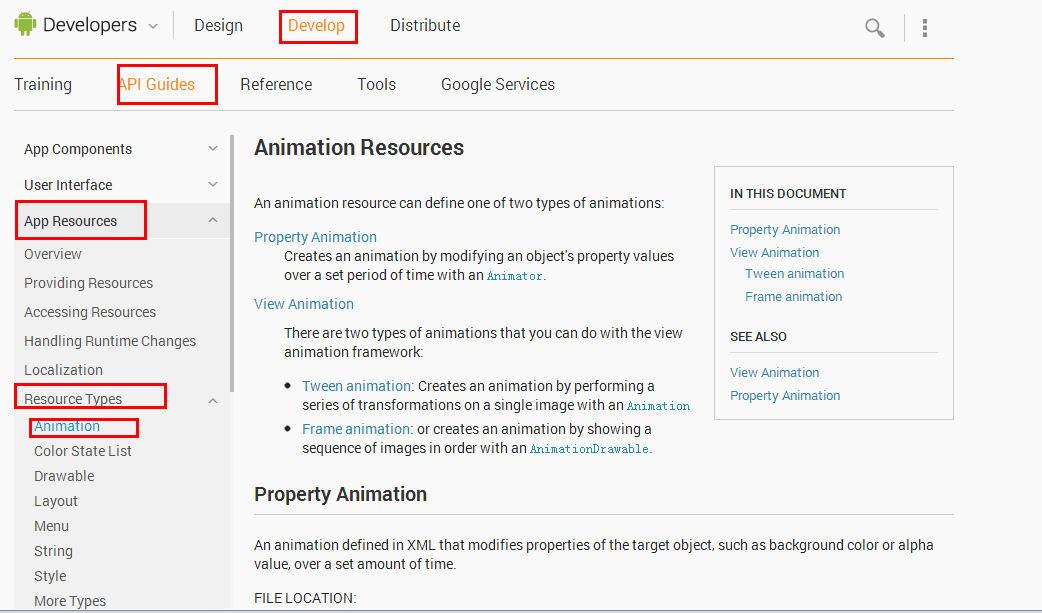
一、Tween animation
1、用代码写的
/**1、 播放透明度变化的动画
*/
public void alpha(View v){
AlphaAnimation aa = new AlphaAnimation(0.0f, 1.0f);
//设置播放的持续时间
aa.setDuration(5000);
//将此动画设置给ImageView
iv.startAnimation(aa);
}
/*
* 2、播放缩放变化的动画
*/
public void scale(View v){
ScaleAnimation sa = new ScaleAnimation(0.0f, 1.0f, 0.0f, 1.0f);
//设置播放的持续时间
sa.setDuration(5000);
//将此动画设置给ImageView
iv.startAnimation(sa);
}
/*
* 3、播放旋转变化的动画
*/
public void rotate(View v){
RotateAnimation ra = new RotateAnimation(0, 90);//从0度旋转到90度
//设置播放的持续时间
ra.setDuration(5000);
//将此动画设置给ImageView
iv.startAnimation(ra);
}
/*
* 4、播放平移变化的动画
*/
public void translate(View v){
TranslateAnimation ta = new TranslateAnimation(0, 100, 0, 100);//x从0到100,y从0到100
//设置播放的持续时间
ta.setDuration(5000);
//将此动画设置给ImageView
iv.startAnimation(ta);
}
3、用xml配置文件
在
配置:
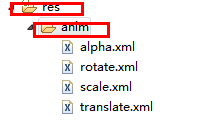
配置:
1、alpha.xml
<?xml version="1.0" encoding="utf-8"?><alpha xmlns:android="http://schemas.android.com/apk/res/android"android:fromAlpha="0.0"android:toAlpha="1.0"android:duration="2000"android:repeatCount="2"android:repeatMode="reverse"></alpha>
2、scale.xml
<?xml version="1.0" encoding="utf-8"?>
<scale xmlns:android="http://schemas.android.com/apk/res/android"
android:fromXScale="0.0"
android:toXScale="2.0"
android:fromYScale="0.0"
android:toYScale="2.0"
android:repeatCount="2"
android:repeatMode="restart"
android:duration="2000"
>
</scale>
3、rotate.xml
<?xml version="1.0" encoding="utf-8"?>
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:fromDegrees="0"
android:toDegrees="90"
android:pivotX="50%p" //按照父体来缩放
android:pivotY="50%p"
android:duration="3000"
></rotate>
4、translate.xml
<?xml version="1.0" encoding="utf-8"?>
<translate xmlns:android="http://schemas.android.com/apk/res/android"
android:fromXDelta="0"
android:toXDelta="100"
android:fromYDelta="0"
android:toYDelta="100"
android:duration="2000"
android:startOffset="1000"
>
</translate>
public void translate(View v){
TranslateAnimation ta = (TranslateAnimation) AnimationUtils.loadAnimation(this,R.anim.translate);//将此动画设置给ImageView
iv.startAnimation(ta);
}
3、组合动画
1)直接代码实现
AlphaAnimation aa = new AlphaAnimation(0.0f, 1.0f);
aa.setDuration(5000);RotateAnimation ra = new RotateAnimation(0, 90);//从0度旋转到90度
ra.setDuration(5000);
TranslateAnimation ta = new TranslateAnimation(0, 100, 0, 100);//x从0到100,y从0到100
ta.setDuration(5000);
AnimationSet set = new AnimationSet(false);//加速器,不要使用
set.addAnimation(aa);
set.addAnimation(ra);
set.addAnimation(ta);
iv.startAnimation(set);
2)xml配置实现
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<alpha
android:duration="2000"
android:fromAlpha="0.0"
android:repeatCount="2"
android:repeatMode="reverse"
android:toAlpha="1.0" >
</alpha>
<rotate
android:fromDegrees="0"
android:pivotX="50%p"
android:pivotY="50%p"
android:toDegrees="90" >
</rotate>
<translate
android:duration="2000"
android:fromXDelta="0"
android:fromYDelta="0"
android:startOffset="1000"
android:toXDelta="100"
android:toYDelta="100" >
</translate></set>
Animation a = AnimationUtils.loadAnimation(this, R.anim.set);
iv.startAnimation(a);
4、完整代码:
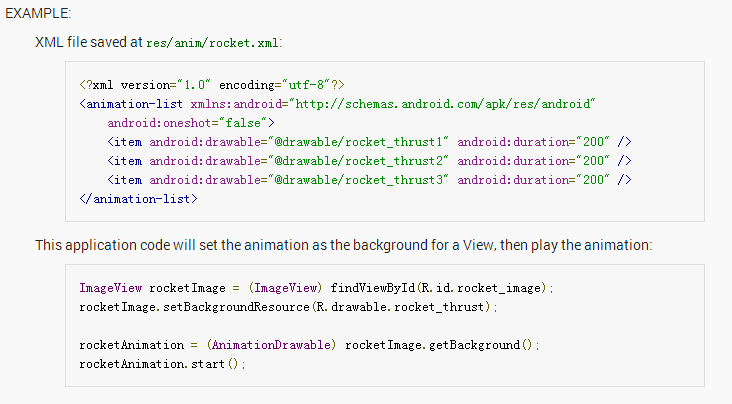
1、在
下放置图片及其下建立list.xml
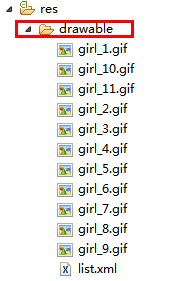
下放置图片及其下建立list.xml
<?xml version="1.0" encoding="utf-8"?>
<animation-list xmlns:android="http://schemas.android.com/apk/res/android"android:oneshot="false" ><itemandroid:drawable="@drawable/girl_1"android:duration="200"/><itemandroid:drawable="@drawable/girl_2"android:duration="200"/><itemandroid:drawable="@drawable/girl_3"android:duration="200"/><itemandroid:drawable="@drawable/girl_4"android:duration="200"/><itemandroid:drawable="@drawable/girl_5"android:duration="200"/><itemandroid:drawable="@drawable/girl_6"android:duration="200"/><itemandroid:drawable="@drawable/girl_7"android:duration="800"/><itemandroid:drawable="@drawable/girl_8"android:duration="200"/><itemandroid:drawable="@drawable/girl_9"android:duration="200"/><itemandroid:drawable="@drawable/girl_10"android:duration="200"/><itemandroid:drawable="@drawable/girl_11"android:duration="200"/></animation-list>
2、Activity代码:
public class FrameActivity extends Activity {private ImageView iv;private AnimationDrawable drawable;@Overridepublic void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(R.layout.main);iv = (ImageView) this.findViewById(R.id.iv);iv.setBackgroundResource(R.drawable.list);drawable = (AnimationDrawable) iv.getBackground();}@Override//触摸事件public boolean onTouchEvent(MotionEvent event) {//触摸下就开始播放帧动画if (event.getAction() == MotionEvent.ACTION_DOWN) {drawable.start();}return super.onTouchEvent(event);}}