Here we import 24 frames from a Flash movie alternately into two
PDF documents. The frames are 0.2 seconds apart. Doc.Rect is
set so that the outputs are 6-up and preserve the aspect ratio. For
the fifth to the eighth imported frames in each document, the
background is suppressed and a transparent oval in the same color is
drawn instead.
[C#]
Doc doc1 = new
Doc(); Doc doc2 = new Doc(); using(SwfImportOperation
operation = new SwfImportOperation()) { const int
fontSize = 20; int k = 0; bool failed
= false; operation.ProcessingObject +=
delegate(object sender, ProcessingObjectEventArgs e)
{ switch(e.Info.SourceType)
{ case
ProcessingSourceType.MultiFrameImage: if(failed
||
k>=24) e.Info.FrameNumber
=
null; else e.Info.FrameNumber
=
1+Convert.ToInt64(0.2*k*e.Info.FrameRate.Value); e.Tag
=
ProcessingSourceType.MultiFrameImage; break; case
ProcessingSourceType.ImageFrame:
{ SwfImportOperation op =
(SwfImportOperation)sender; op.Doc =
op.Doc==doc1? doc2: doc1; const int
distance = 20; const int margin =
30; double width =
op.Doc.MediaBox.Width-2*margin; double
height =
op.Doc.MediaBox.Height-2*margin; double
scale =
Math.Min((width-distance)/(2*e.Info.Width.Value), (height-2*distance-3*fontSize)/(3*e.Info.Height.Value));
int
p = k/2; double rectWidth =
scale*e.Info.Width.Value; double
rectHeight =
scale*e.Info.Height.Value; op.Doc.Rect.SetRect(margin+(width+distance)*(p%2)/2, margin+height-rectHeight-(height+2*distance)*(p/2%3)/3, rectWidth,
rectHeight); if(p%6==0) op.Doc.Page
= op.Doc.AddPage(); if(p>=4 &&
p<8 && e.Info.BackgroundColor!=null)
{ op.BackgroundRegion =
null; op.Doc.Color.String =
e.Info.BackgroundColor.String; op.Doc.Color.Alpha
=
127; op.Doc.AddOval(true); } }
break; } }; operation.ProcessedObject +=
delegate(object sender, ProcessedObjectEventArgs e)
{ if(!e.Successful)
{ failed =
true; return; } if(e.Tag
is ProcessingSourceType &&
(ProcessingSourceType)e.Tag==ProcessingSourceType.MultiFrameImage) { SwfImportOperation
op =
(SwfImportOperation)sender; op.Doc.Color.Gray
= 0; op.Doc.Color.Alpha =
255; op.Doc.FontSize =
fontSize; op.Doc.TextStyle.HPos =
0.5; op.Doc.Rect.Top =
op.Doc.Rect.Bottom; op.Doc.Rect.Bottom =
op.Doc.MediaBox.Bottom; op.Doc.AddText(string.Format("{0}
secs",
0.2*k)); ++k; } PixMap
pixmap = e.Object as
PixMap; if(pixmap!=null) pixmap.Compress(); }; operation.Import(Server.MapPath("ABCpdf.swf")); } doc1.Save(Server.MapPath("swf1.pdf")); doc1.Clear(); doc2.Save(Server.MapPath("swf2.pdf")); doc2.Clear(); [Visual Basic]
Dim theMyOp As
MyOp Using theOperation As
New SwfImportOperation theMyOp = New
MyOp(theOperation) theOperation.Import(Server.MapPath("ABCpdf.swf")) End Using theMyOp.Save(Server.MapPath("swf1.pdf"),
Server.MapPath("swf2.pdf")) theMyOp.Clear()
Private
NotInheritable Class MyOp Private Const theFontSize
As Integer = 20 Private theDoc1 As
Doc Private theDoc2 As Doc Private k
As Integer Private theFailed As
Boolean
Public Sub New(theOperation As
SwfImportOperation) theDoc1 = New
Doc() theDoc2 = New
Doc() k =
0 theFailed =
False AddHandler
theOperation.ProcessingObject, AddressOf
Processing AddHandler
theOperation.ProcessedObject, AddressOf Processed End
Sub
Public Sub Save(path1 As String, path2 As
String) theDoc1.Save(Server.MapPath(path1)) theDoc2.Save(Server.MapPath(path2)) End
Sub
Public Sub
Clear() theDoc1.Clear() theDoc2.Clear() End
Sub
Private Sub Processing(sender As Object, e As
ProcessingObjectEventArgs) Select Case
e.Info.SourceType Case
ProcessingSourceType.MultiFrameImage If
theFailed Or k >= 24
Then e.Info.FrameNumber
=
Nothing Else e.Info.FrameNumber
= 1 + Convert.ToInt64(0.2 * k *
e.Info.FrameRate.Value) End
If e.Tag =
ProcessingSourceType.MultiFrameImage Case
ProcessingSourceType.ImageFrame Dim
op As SwfImportOperation = CType(sender,
SwfImportOperation) If op.Doc
Is theDoc1
Then op.Doc =
theDoc2 Else op.Doc
= theDoc1 End
If Const distance As Integer
= 20 Const margin As Integer
= 30 Dim width As Double =
op.Doc.MediaBox.Width - 2 *
margin Dim height As Double =
op.Doc.MediaBox.Height - 2 *
margin Dim scale As Double =
Math.Min((width - distance) / (2 * e.Info.Width.Value),
_ (height - 2 *
distance - 3 * theFontSize) / (3 *
e.Info.Height.Value))
Dim
p As Integer = k \ 2 Dim
rectWidth As Double = scale *
e.Info.Width.Value Dim
rectHeight As Double = scale *
e.Info.Height.Value op.Doc.Rect.SetRect(margin
+ (width + distance) * (p Mod 2) / 2,
_ margin + height
- rectHeight - (height + 2 * distance) * (p / 2 Mod 3) / 3,
_ rectWidth,
rectHeight) If p Mod 6 = 0
Then op.Doc.Page
= op.Doc.AddPage() End
If If p >= 4 And p < 8
And e.Info.BackgroundColor IsNot Nothing
Then op.BackgroundRegion
=
Nothing op.Doc.Color.String
=
e.Info.BackgroundColor.String op.Doc.Color.Alpha
=
127 op.Doc.AddOval(True) End
If End Select End
Sub
Private Sub Processed(sender As Object, e As
ProcessedObjectEventArgs) If Not
e.Successful Then theFailed =
True Return End
If If TypeOf e.Tag Is
ProcessingSourceType
Then If CType(e.Tag,
ProcessingSourceType) = ProcessingSourceType.MultiFrameImage
Then Dim op
As SwfImportOperation = CType(sender,
SwfImportOperation) op.Doc.Color.Gray
=
0 op.Doc.Color.Alpha
=
255 op.Doc.FontSize
=
theFontSize op.Doc.TextStyle.HPos
=
0.5 op.Doc.Rect.Top
=
op.Doc.Rect.Bottom op.Doc.Rect.Bottom
=
op.Doc.MediaBox.Bottom op.Doc.AddText(String.Format("{0}
secs", 0.2 *
k)) k +=
1 End
If End If Dim
thePixMap As PixMap = TryCast(e.Object,
PixMap) If thePixMap IsNot Nothing
Then
thePixMap.Compress() End
If End Sub End Class
swf1.pdf
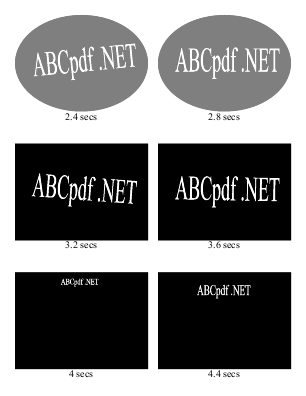
swf2.pdf
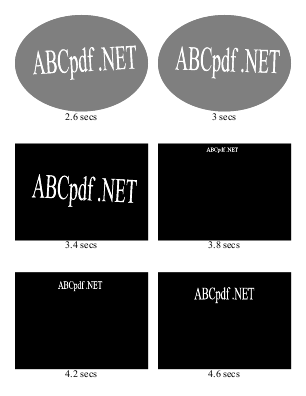 |
|
|