The following examples show the effect of the default kernel - a Sobel Edge Detector.
[C#]
void function() {
using (Doc doc = new Doc()) {
AddImagePage(doc, img3); // original image
doc.Rendering.Save("EffectConvolution.jpg");
using (ImageLayer layer = AddImagePage(doc, img3)) {
using (EffectOperation effect = new EffectOperation("Convolution")) {
// the default is a Sobel Edge Detector
effect.Apply(layer.PixMap);
}
}
doc.Rendering.Save("EffectConvolutionDefault.jpg");
using (ImageLayer layer = AddImagePage(doc, img3)) {
using (EffectOperation effect = new EffectOperation("Convolution")) {
// the following is a high intensity sharpen filter
double[] k = new double[] { 0, -1, 0, -1, 5, -1, 0, -1, 0 };
effect.Parameters["Number"].Value = 1;
effect.Parameters["Values"].Values = k;
effect.Apply(layer.PixMap);
}
}
doc.Rendering.Save("EffectConvolutionSharpen.jpg");
}
}
[Visual Basic]
Sub ...
Using doc As New Doc()
AddImagePage(doc, img3)
' original image
doc.Rendering.Save("EffectConvolution.jpg")
Using layer As ImageLayer = AddImagePage(doc, img3)
Using effect As New EffectOperation("Convolution")
' the default is a Sobel Edge Detector
effect.Apply(layer.PixMap)
End Using
End Using
doc.Rendering.Save("EffectConvolutionDefault.jpg")
Using layer As ImageLayer = AddImagePage(doc, img3)
Using effect As New EffectOperation("Convolution")
' the following is a high intensity sharpen filter
Dim k As Double() = New Double() {0, -1, 0, -1, 5, -1, _
0, -1, 0}
effect.Parameters("Number").Value = 1
effect.Parameters("Values").Values = k
effect.Apply(layer.PixMap)
End Using
End Using
doc.Rendering.Save("EffectConvolutionSharpen.jpg")
End Using
End Sub
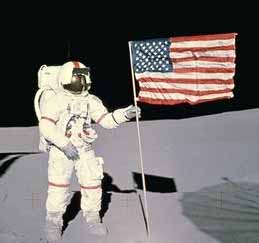
Original Image
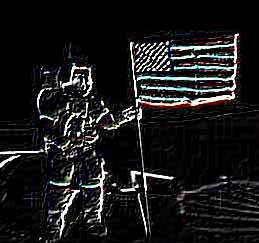
After Convolution Applied
|
|
|