The following example shows the effect that this parameter has
on PDF rendering.
[C#]
Doc theDoc = new Doc();
theDoc.Rect.Inset(50, 50);
// Add some text
theDoc.FontSize = 48;
theDoc.Pos.String = "50 690";
int id = theDoc.AddText("Abc");
// Render images
theDoc.Rect.String = theDoc.GetInfo(id, "rect");
theDoc.Rendering.AntiAliasText = true;
Bitmap antialiasedBitmap = theDoc.Rendering.GetBitmap();
theDoc.Rendering.AntiAliasText = false;
Bitmap aliasedBitmap = theDoc.Rendering.GetBitmap();
// Add enlarged images to the pdf file
theDoc.Rect.Magnify(5, 5);
theDoc.Rect.Move(0, -300);
theDoc.AddImageBitmap(aliasedBitmap, false);
theDoc.Rect.Move(0, -300);
theDoc.AddImageBitmap(antialiasedBitmap, false);
// Annotate
theDoc.Rect.String = theDoc.MediaBox.String;
theDoc.Color.String = "255 0 0";
theDoc.FontSize = 36;
theDoc.Pos.String = "50 740";
theDoc.AddText("Original text:");
theDoc.Pos.String = "50 620";
theDoc.AddText("Magnified aliased image:");
theDoc.Pos.String = "50 320";
theDoc.AddText("Magnified antialiased image:");
// Save render of pdf files
theDoc.Rendering.AntiAliasText = true;
theDoc.Rendering.DotsPerInch = 36;
theDoc.Rendering.Save(Server.MapPath("RenderingAntiAliasText.png"));
theDoc.Clear();
[Visual Basic]
Dim theDoc As Doc = New Doc()
theDoc.Rect.Inset(50, 50)
' Add some text
theDoc.FontSize = 48
theDoc.Pos.String = "50 690"
Dim id As Integer = theDoc.AddText("Abc")
' Render images
theDoc.Rect.String = theDoc.GetInfo(id, "rect")
theDoc.Rendering.AntiAliasText = True
Dim antialiasedBitmap As Bitmap = theDoc.Rendering.GetBitmap()
theDoc.Rendering.AntiAliasText = False
Dim aliasedBitmap As Bitmap = theDoc.Rendering.GetBitmap()
' Add enlarged images to the pdf file
theDoc.Rect.Magnify(5, 5)
theDoc.Rect.Move(0, -300)
theDoc.AddImageBitmap(aliasedBitmap, False)
theDoc.Rect.Move(0, -300)
theDoc.AddImageBitmap(antialiasedBitmap, False)
' Annotate
theDoc.Rect.String = theDoc.MediaBox.String
theDoc.Color.String = "255 0 0"
theDoc.FontSize = 36
theDoc.Pos.String = "50 740"
theDoc.AddText("Original text:")
theDoc.Pos.String = "50 620"
theDoc.AddText("Magnified aliased image:")
theDoc.Pos.String = "50 320"
theDoc.AddText("Magnified antialiased image:")
' Save render of pdf files
theDoc.Rendering.AntiAliasText = True
theDoc.Rendering.DotsPerInch = 36
theDoc.Rendering.Save(Server.MapPath("RenderingAntiAliasText.png"))
theDoc.Clear()
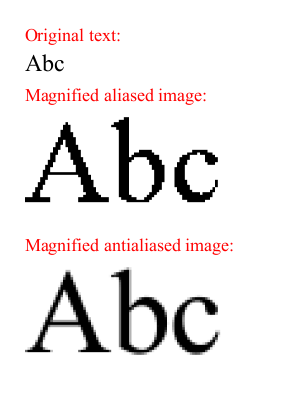
RenderingAntiAliasText.png
|
|
|