The following example images show the effect of Gaussian Blur.
[C#]
void function() {
using (Doc doc = new Doc()) {
AddImagePage(doc, img3); // original image
doc.Rendering.Save("EffectGaussianBlur.jpg");
using (ImageLayer layer = AddImagePage(doc, img3)) {
using (EffectOperation effect = new EffectOperation("Gaussian Blur")) {
effect.Parameters["Radius"].Value = 1.2;
effect.Apply(layer.PixMap);
}
}
doc.Rendering.Save("EffectGaussianBlur12.jpg");
using (ImageLayer layer = AddImagePage(doc, img3)) {
using (EffectOperation effect = new EffectOperation("Gaussian Blur")) {
effect.Parameters["Radius"].Value = 2.5;
effect.Apply(layer.PixMap);
}
}
doc.Rendering.Save("EffectGaussianBlur25.jpg");
using (ImageLayer layer = AddImagePage(doc, img3)) {
using (EffectOperation effect = new EffectOperation("Gaussian Blur")) {
effect.Parameters["Radius"].Value = 5.0;
effect.Apply(layer.PixMap);
}
}
doc.Rendering.Save("EffectGaussianBlur50.jpg");
}
}
[Visual Basic]
Sub ...
Using doc As New Doc()
AddImagePage(doc, img3)
' original image
doc.Rendering.Save("EffectGaussianBlur.jpg")
Using layer As ImageLayer = AddImagePage(doc, img3)
Using effect As New EffectOperation("Gaussian Blur")
effect.Parameters("Radius").Value = 1.2
effect.Apply(layer.PixMap)
End Using
End Using
doc.Rendering.Save("EffectGaussianBlur12.jpg")
Using layer As ImageLayer = AddImagePage(doc, img3)
Using effect As New EffectOperation("Gaussian Blur")
effect.Parameters("Radius").Value = 2.5
effect.Apply(layer.PixMap)
End Using
End Using
doc.Rendering.Save("EffectGaussianBlur25.jpg")
Using layer As ImageLayer = AddImagePage(doc, img3)
Using effect As New EffectOperation("Gaussian Blur")
effect.Parameters("Radius").Value = 5.0
effect.Apply(layer.PixMap)
End Using
End Using
doc.Rendering.Save("EffectGaussianBlur50.jpg")
End Using
End Sub
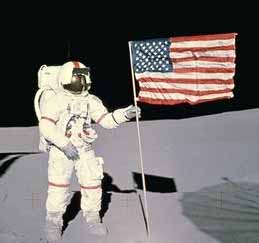
Original Image before Gaussian Blur
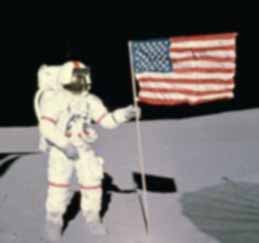
After Gaussian Blur Radius 1.2 pixels
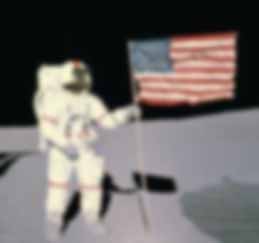
After Gaussian Blur Radius 2.5 pixels
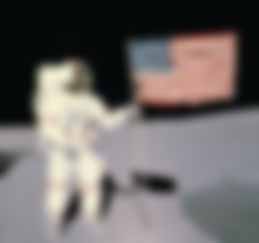
After Gaussian Blur Radius 5.0 pixels
|