This example shows how to read an existing PDF document and
insert a background image into every page.
We start by reading our template PDF document and finding out
core information we will need to reference each page.
[C#]
Doc theDoc = new
Doc(); theDoc.Read(Server.MapPath("../mypics/sample.pdf")); int
theCount = theDoc.PageCount;
[Visual Basic]
Dim theDoc As Doc =
New Doc()
theDoc.Read(Server.MapPath("../mypics/sample.pdf")) Dim
theCount As Integer = theDoc.PageCount
We cycle through the pages inserting images as we go.
We set the layer property to ensure that the image gets added in
the background rather than on top of existing content.
The first time we add an image file. Subsequent times we
reference the image ID. This means that we embed only one copy of
the image data and simply reference that data from each page.
Finally we save the modified PDF.
[C#]
int theID = 0; for
(int i = 1; i <= theCount; i++)
{ theDoc.PageNumber = i; theDoc.Layer
= theDoc.LayerCount + 1; if (i == 1)
{ string thePath =
Server.MapPath("../mypics/light.jpg"); theID
= theDoc.AddImageFile(thePath,
1); } else theDoc.AddImageCopy(theID); } theDoc.Save(Server.MapPath("watermark.pdf")); theDoc.Clear();
[Visual Basic]
Dim theID As Integer =
0 For i As Integer = 1 To
theCount theDoc.PageNumber =
i theDoc.Layer = theDoc.LayerCount +
1 If i = 1 Then Dim
thePath As String = Server.MapPath("../mypics/light.jpg")
theID = theDoc.AddImageFile(thePath,
1) Else
theDoc.AddImageCopy(theID) End
If Next theDoc.Save(Server.MapPath("watermark.pdf")) theDoc.Clear()
Given the following document.
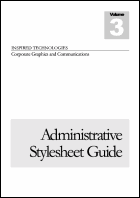 sample.pdf - [Page 1] |
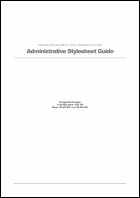 sample.pdf - [Page 2] |
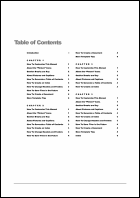 sample.pdf - [Page 3] |
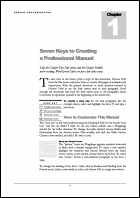 sample.pdf - [Page 4] |
And the following image.
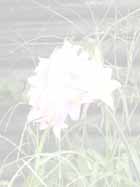
This is the kind of output you might expect.
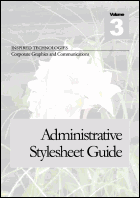 watermark.pdf - [Page 1] |
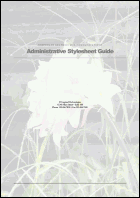 watermark.pdf - [Page 2] |
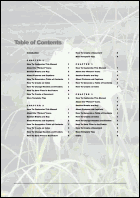 watermark.pdf - [Page 3] |
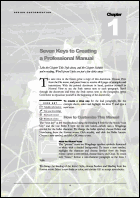 watermark.pdf - [Page 4] |
|