This example shows how to draw one PDF into another. It takes a
PDF document and creates a 'four-up' summary document by drawing
four pages on each page of the new document.
First we create an ABCpdf Doc object and read in our source
document.
[C#]
Doc theSrc = new
Doc(); theSrc.Read(Server.MapPath("../Rez/spaceshuttle.pdf")); int
theCount = theSrc.PageCount;
[Visual Basic]
Dim theSrc As Doc =
New Doc()
theSrc.Read(Server.MapPath("../Rez/spaceshuttle.pdf")) Dim
theCount As Integer = theSrc.PageCount
Next we create a destination Doc object and set the MediaBox so that the page
size will match that of the source document. We change the rect so
that it occupies a quarter of the page with room to accomodate a
small margin.
[C#]
Doc theDst = new
Doc(); theDst.MediaBox.String =
theSrc.MediaBox.String; theDst.Rect.String =
theDst.MediaBox.String; theDst.Rect.Magnify(0.5,
0.5); theDst.Rect.Inset(10, 10); double theX, theY; theX =
theDst.MediaBox.Width / 2; theY = theDst.MediaBox.Height /
2;
[Visual
Basic]
Dim theDst As Doc = New Doc()
theDst.MediaBox.String =
theSrc.MediaBox.String theDst.Rect.String =
theDst.MediaBox.String theDst.Rect.Magnify(0.5,
0.5) theDst.Rect.Inset(10, 10) Dim theX As Double,theY As
Double theX = theDst.MediaBox.Width / 2 theY =
theDst.MediaBox.Height / 2
We go through every page in the source document drawing a framed
copy of each page at a different position on our four-up document.
Every fourth page we add a new page into our destination
document.
[C#]
for (int i = 1; i <=
theCount; i++) { switch (i % 4)
{ case
1: theDst.Page =
theDst.AddPage(); theDst.Rect.Position(10,
theY +
10); break; case
2: theDst.Rect.Position(theX
+ 10, theY + 10); break;
case
3: theDst.Rect.Position(10,
10); break;
case
0: theDst.Rect.Position(theX
+ 10, 10); break;
} theDst.AddImageDoc(theSrc, i,
null); theDst.FrameRect(); }
[Visual Basic]
For i As Integer = 1
To theCount Select Case i Mod
4 Case
1 theDst.Page =
theDst.AddPage() theDst.Rect.Position(10,
theY + 10) Case
2 theDst.Rect.Position(theX +
10, theY + 10) Case
3 theDst.Rect.Position(10,
10) Case
0 theDst.Rect.Position(theX +
10, 10) End
Select theDst.AddImageDoc(theSrc, i,
Nothing) theDst.FrameRect() Next
Finally we save.
[C#]
theDst.Save(Server.MapPath("fourup.pdf")); //
finished
[Visual
Basic]
theDst.Save(Server.MapPath("fourup.pdf")) '
finished
We get the following output.
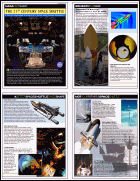 fourup.pdf - [Page 1] |
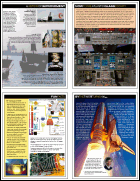 fourup.pdf - [Page 2] |
|