|
You may wish to generate a PDF document with markup created
dynamically at run-time.
The code below creates a set of markup Annotations including
squares, lines, text effects, circles and polygons.
[C#]
//Markup
annotations theDoc.Page = theDoc.AddPage(); theDoc.Pos.X =
40; theDoc.Pos.Y = theDoc.MediaBox.Top -
40; theDoc.AddText("Markup annotations");
SquareAnnotation
square = new SquareAnnotation(theDoc, "40 560 300 670", "255 0 0",
"0 0 255"); square.BorderWidth = 8;
LineAnnotation line =
new LineAnnotation(theDoc, "100 565 220 665", "255 0
0"); line.BorderWidth = 12; line.RichTextCaption = "<span
style= \"font-size:36pt;
color:#FF0000\">Line</span>";
theDoc.FontSize =
24; theDoc.Pos.String = "400 670"; int id =
theDoc.AddText("Underline"); TextMarkupAnnotation markup = new
TextMarkupAnnotation(theDoc, id, "Underline", "0 255
0"); theDoc.Pos.String = "400 640"; id =
theDoc.AddText("Highlight");
markup = new
TextMarkupAnnotation(theDoc, id, "Highlight", "255 255
0"); theDoc.Pos.String = "400 610"; id =
theDoc.AddText("StrikeOut");
markup = new
TextMarkupAnnotation(theDoc, id, "StrikeOut", "255 0
0"); theDoc.Pos.String = "400 580"; id =
theDoc.AddText("Squiggly");
markup = new
TextMarkupAnnotation(theDoc, id, "Squiggly", "0 0
255"); theDoc.FontSize = 36;
CircleAnnotation circle = new
CircleAnnotation(theDoc, "80 320 285 525", "255 255 0", "255 128
0"); circle.BorderWidth = 20; circle.BorderStyle =
"Dashed"; circle.BorderDash = "[3 2]";
LineAnnotation
arrowLine = new LineAnnotation(theDoc, "385 330 540 520", "255 0
0"); arrowLine.LineEndingsStyle = "ClosedArrow
ClosedArrow"; arrowLine.BorderWidth = 6; arrowLine.FillColor =
"255 0 0";
PolygonAnnotation polygon = new
PolygonAnnotation(theDoc, "100 70 50 120 50 220 100 270 200 270 250
220 250 120 200 70", "255 0 0", "0 255 0"); PolygonAnnotation
cloudyPolygon = new PolygonAnnotation(theDoc, "400 70 350 120 350
220 400 270 500 270 550 220 550 120 500 70", "255 0 0", "64 85
255"); cloudyPolygon.CloudyEffect = 1;
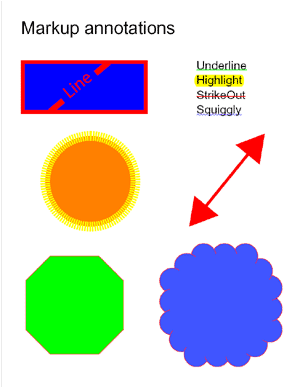
|
|
|