This example shows how to use the Array.FromContentStream function to parse a content stream and then use the Find method to search for certain types of color operators and replace them with other color operators. In this example this has the effect of converting some black parts of the PDF to red.
[C#]
using (Doc doc = new Doc()) {
doc.Read("spaceshuttle.pdf");
doc.RemapPages(new int[] { 1, 1 });
doc.PageNumber = 2;
Page page = doc.ObjectSoup[doc.Page] as Page;
StreamObject[] layers = page.GetLayers();
MemoryStream st = new MemoryStream();
foreach (StreamObject layer in layers) {
if (!layer.Decompress())
throw new Exception("Unable to decompress stream.");
byte[] data = layer.GetData();
st.Write(data, 0, data.Length);
layer.CompressFlate();
}
ArrayAtom array = ArrayAtom.FromContentStream(st.ToArray());
if (true) {
var items = OpAtom.Find(array, new string[] { "k" });
foreach (var pair in items) { // make red
Atom[] args = OpAtom.GetParameters(array, pair.Item2);
if (args != null) {
((NumAtom)args[0]).Real = 0;
((NumAtom)args[1]).Real = 1;
((NumAtom)args[2]).Real = 1;
((NumAtom)args[3]).Real = 0;
}
}
}
if (true) {
var items = OpAtom.Find(array, new string[] { "rg" });
foreach (var pair in items) { // make green
Atom[] args = OpAtom.GetParameters(array, pair.Item2);
if (args != null) {
((NumAtom)args[0]).Real = 0;
((NumAtom)args[1]).Real = 1;
((NumAtom)args[2]).Real = 0;
}
}
}
byte[] arrayData = array.GetData();
StreamObject so = new StreamObject(doc.ObjectSoup);
so.SetData(arrayData, 1, arrayData.Length - 2);
doc.SetInfo(page.ID, "/Contents:Del", "");
page.AddLayer(so);
doc.Save("ReplaceColors.pdf");
}
[Visual Basic]
Sub ...
Using doc As New Doc()
doc.RemapPages(New Integer[] { 1, 1 })
doc.PageNumber = 2
Dim page As Page = TryCast(doc.ObjectSoup(doc.Page), Page)
Dim layers As StreamObject() = page.GetLayers()
Dim st As New MemoryStream()
For Each layer As StreamObject In layers
If Not layer.Decompress() Then
Throw New Exception("Unable to decompress stream.")
End If
Dim data As Byte() = layer.GetData()
st.Write(data, 0, data.Length)
layer.CompressFlate()
Next
Dim array As ArrayAtom = ArrayAtom.FromContentStream(st.ToArray())
If True Then
Dim items = OpAtom.Find(array, New String() {"k"})
For Each pair As var In items
' make red
Dim args As Atom() = OpAtom.GetParameters(array, pair.Item2)
If args IsNot Nothing Then
DirectCast(args(0), NumAtom).Real = 0
DirectCast(args(1), NumAtom).Real = 1
DirectCast(args(2), NumAtom).Real = 1
DirectCast(args(3), NumAtom).Real = 0
End If
Next
End If
If True Then
Dim items = OpAtom.Find(array, New String() {"rg"})
For Each pair As var In items
' make green
Dim args As Atom() = OpAtom.GetParameters(array, pair.Item2)
If args IsNot Nothing Then
DirectCast(args(0), NumAtom).Real = 0
DirectCast(args(1), NumAtom).Real = 1
DirectCast(args(2), NumAtom).Real = 0
End If
Next
End If
Dim arrayData As Byte() = array.GetData()
Dim so As New StreamObject(doc.ObjectSoup)
so.SetData(arrayData, 1, arrayData.Length - 2)
doc.SetInfo(page.ID, "/Contents:Del", "")
page.AddLayer(so)
doc.Save("ReplaceColors.pdf")
End Using
End Sub
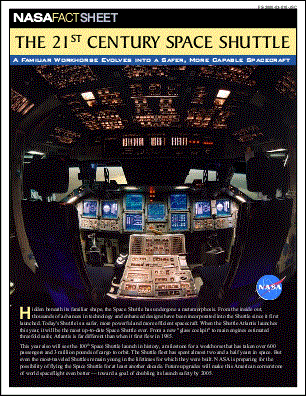
ReplaceColors.pdf [Page 1]
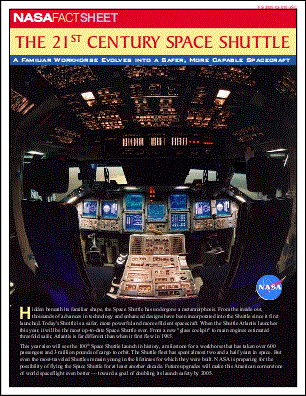
ReplaceColors.pdf [Page 2]
|
|
|