The following example shows the effect that this parameter has
on PDF rendering.
[C#]
Doc theDoc = new Doc();
// Add a polygon
theDoc.Color.String = "255 0 0";
theDoc.AddPoly("32 650 50 704 68 650 22 683 79 683 32 650",
true);
theDoc.Rect.String = "20 650 80 704";
// Render the drawn area with AntiAliasPolygons (default)
Bitmap antiAliasedBitmap = theDoc.Rendering.GetBitmap();
// Render the drawn area without AntiAliasPolygons
theDoc.Rendering.AntiAliasPolygons = false;
Bitmap aliasedBitmap = theDoc.Rendering.GetBitmap();
// Add magnified aliased image
theDoc.Rect.String = "5 20 605 560";
theDoc.AddImageBitmap(aliasedBitmap, false);
// Anotate
theDoc.Color.String = "black";
theDoc.FontSize = 30;
theDoc.Pos.String = "20 750";
theDoc.AddText("Original path:");
theDoc.Pos.String = "20 620";
theDoc.AddText("Magnified rendered image:");
// Render the document with aliased image
theDoc.Rendering.DotsPerInch = 36;
theDoc.Rect.String = theDoc.MediaBox.String;
theDoc.Rendering.Save(Server.MapPath("RenderingAntiAliasPolygonsFalse.png"));
// Add magnified antialiased image
theDoc.Rect.String = "5 20 605 560";
theDoc.AddImageBitmap(antiAliasedBitmap, false);
// Render the document with antialiased image
theDoc.Rendering.DotsPerInch = 36;
theDoc.Rect.String = theDoc.MediaBox.String;
theDoc.Rendering.Save(Server.MapPath("RenderingAntiAliasPolygonsTrue.png"));
theDoc.Clear();
[Visual Basic]
Dim theDoc As Doc = New Doc()
' Add a polygon
theDoc.Color.String = "255 0 0"
theDoc.AddPoly("32 650 50 704 68 650 22 683 79 683 32 650",
True)
theDoc.Rect.String = "20 650 80 704"
' Render the drawn area with AntiAliasPolygons (default)
Dim antiAliasedBitmap As Bitmap = theDoc.Rendering.GetBitmap()
' Render the drawn area without AntiAliasPolygons
theDoc.Rendering.AntiAliasPolygons = False
Dim aliasedBitmap As Bitmap = theDoc.Rendering.GetBitmap()
' Add magnified aliased image
theDoc.Rect.String = "5 20 605 560"
theDoc.AddImageBitmap(aliasedBitmap, False)
' Anotate
theDoc.Color.String = "black"
theDoc.FontSize = 30
theDoc.Pos.String = "20 750"
theDoc.AddText("Original path:")
theDoc.Pos.String = "20 620"
theDoc.AddText("Magnified rendered image:")
' Render the document with aliased image
theDoc.Rendering.DotsPerInch = 36
theDoc.Rect.String = theDoc.MediaBox.String
theDoc.Rendering.Save(Server.MapPath("RenderingAntiAliasPolygonsFalse.png"))
' Add magnified antialiased image
theDoc.Rect.String = "5 20 605 560"
theDoc.AddImageBitmap(antiAliasedBitmap, False)
' Render the document with antialiased image
theDoc.Rendering.DotsPerInch = 36
theDoc.Rect.String = theDoc.MediaBox.String
theDoc.Rendering.Save(Server.MapPath("RenderingAntiAliasPolygonsTrue.png"))
theDoc.Clear()
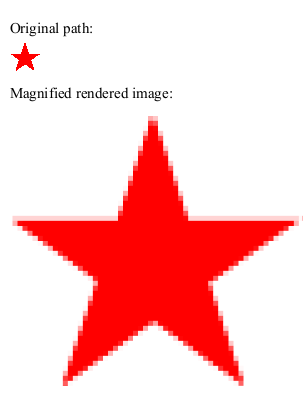
RenderingAntiAliasPolygonsTrue.png
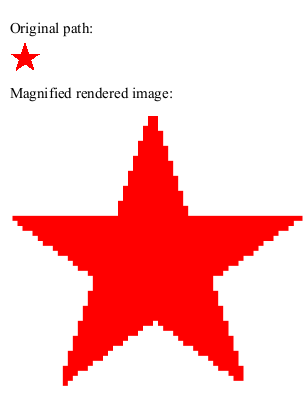
RenderingAntiAliasPolygonsFalse.png
|