The following code adds a sequence of pages with a nested
sequence of bookmarks. The image shows the appearance of the
document outline. Note that none of the subject pages are visible
because the chapter pages were added in a collapsed state.
[C#]
Doc theDoc = new
Doc(); theDoc.FontSize = 64; string theSection, theChapter,
theSubject; for (int i = 1; i < 3; i++)
{ theDoc.Page =
theDoc.AddPage(); theSection = i.ToString() + "
Section"; theDoc.AddText(theSection); theDoc.AddBookmark(theSection,
true); for (int j = 1; j < 5; j++)
{ theDoc.Page =
theDoc.AddPage(); theChapter = theSection
+ "\\" + j.ToString() + "
Chapter"; theDoc.AddText(theChapter); theDoc.AddBookmark(theChapter,
false); for (int k = 1; k < 6; k++)
{ theDoc.Page =
theDoc.AddPage(); theSubject
= theChapter + "\\" + k.ToString() + "
Subject"; theDoc.AddText(theSubject); theDoc.AddBookmark(theSubject,
true); } } } theDoc.Save(Server.MapPath("docaddbookmark.pdf")); theDoc.Clear();
[Visual Basic]
Dim theDoc As Doc =
New Doc() theDoc.FontSize = 64 Dim theSection, theChapter,
theSubject As String For
i As Integer = 1 To 2 theDoc.Page =
theDoc.AddPage() theSection = i.ToString() + "
Section" theDoc.AddText(theSection) theDoc.AddBookmark(theSection,
True) For j As Integer = 1 To
4 theDoc.Page =
theDoc.AddPage() theChapter = theSection
+ "\" + j.ToString() + "
Chapter" theDoc.AddText(theChapter) theDoc.AddBookmark(theChapter,
False) For k As Integer = 1 To
5 theDoc.Page =
theDoc.AddPage() theSubject =
theChapter + "\" + k.ToString() + "
Subject" theDoc.AddText(theSubject) theDoc.AddBookmark(theSubject,
True) Next Next Next theDoc.Save(Server.MapPath("docaddbookmark.pdf")) theDoc.Clear()
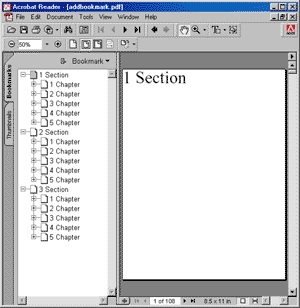 docaddbookmark.pdf
|
|
|