Here we import the pages with odd page numbers of an XPS document
up to page 8, 2 pages per sheet in landscape. The aspect ratio is
preserved and a border is also drawn.
[C#]
Doc doc = new
Doc(); MyImportOperation importOp = new
MyImportOperation(doc); importOp.Import(Server.MapPath("AdvancedGraphicsExamples.xps")); doc.Save(Server.MapPath("xps.pdf")); doc.Clear();
public
class MyImportOperation { private Doc _doc =
null; private double _margin =
10; private int _pagesAdded =
0;
public MyImportOperation(Doc doc)
{ _doc =
doc;
_doc.Transform.Rotate(90,
_doc.MediaBox.Left,
_doc.MediaBox.Bottom); _doc.Transform.Translate(_doc.MediaBox.Width,
0);
int theID =
_doc.GetInfoInt(_doc.Root,
"Pages"); _doc.SetInfo(theID, "/Rotate",
"90"); }
public void Import(string
inPath) { using(XpsImportOperation op
= new XpsImportOperation())
{ op.ProcessingObject +=
Processing; op.ProcessedObject
+= Processed; op.Import(_doc,
inPath); } }
public void
Processing(object sender, ProcessingObjectEventArgs e)
{ if (e.Info.SourceType ==
ProcessingSourceType.Page && e.Info.PageNumber != null)
{ if ((e.Info.PageNumber % 2)
==
0) e.Info.PageNumber++;
if
(e.Info.PageNumber >=
8) e.Info.PageNumber
= null;
e.Tag =
e.Info.PageNumber; } else if
(e.Info.SourceType == ProcessingSourceType.PageContent)
{ if ((_pagesAdded % 2) ==
0) _doc.Page =
_doc.AddPage();
double
width =
_doc.MediaBox.Height; double
height =
_doc.MediaBox.Width; double
scale = Math.Min((width - 4 * _margin) / (2 *
e.Info.Width.Value), (height
- 2 * _margin) /
e.Info.Height.Value);
double
rectWidth = scale *
e.Info.Width.Value; double
rectHeight = scale *
e.Info.Height.Value;
double
distanceX = (width - 2 * rectWidth) /
3; double distanceY = (height
- rectHeight) /
2;
_doc.Rect.SetRect(distanceX
+ (_pagesAdded % 2) * (distanceX +
rectWidth), distanceY,
rectWidth,
rectHeight);
e.Info.Handled
=
true; _pagesAdded++; } }
public
void Processed(object sender, ProcessedObjectEventArgs e)
{ if (e.Successful)
{ PixMap pixmap = e.Object as
PixMap; if (pixmap !=
null) pixmap.Compress();
GraphicLayer
graphic = e.Object as
GraphicLayer; if (graphic !=
null)
{ _doc.FrameRect();
int
pageNumber =
(int)e.Tag; _doc.FontSize
= 16; _doc.TextStyle.HPos =
0.5; _doc.Rect.Top
= _doc.Rect.Bottom -
_margin; _doc.Rect.Bottom
=
_doc.MediaBox.Bottom; _doc.AddText(string.Format("Page
{0}",
pageNumber)); } } } }
[Visual Basic]Dim doc As New
Doc() Dim importOp As MyImportOperation = New
MyImportOperation(doc) importOp.Import(Server.MapPath("AdvancedGraphicsExamples.xps")) doc.Save(Server.MapPath("xps.pdf")) doc.Clear();
Public
Class MyImportOperation Private _doc As Doc =
Nothing Private _margin As Double =
10 Private _pagesAdded As Integer =
0
Public Sub New(doc As
Doc) _doc =
doc
_doc.Transform.Rotate(90,
_doc.MediaBox.Left,
_doc.MediaBox.Bottom) _doc.Transform.Translate(_doc.MediaBox.Width,
0)
Dim theID As Integer =
_doc.GetInfoInt(_doc.Root,
"Pages") _doc.SetInfo(theID, "/Rotate",
"90") End Sub
Public Sub
Import(inPath As String) Using op As New
XpsImportOperation() AddHandler
op.ProcessingObject, AddressOf
Processing AddHandler
op.ProcessedObject, AddressOf
Processed op.Import(_doc,
inPath) End Using End
Sub
Public Sub Processing(sender As Object, e As
ProcessingObjectEventArgs) If
e.Info.SourceType = ProcessingSourceType.Page And e.Info.PageNumber
IsNot Nothing Then If
(e.Info.PageNumber Mod 2) = 0
Then e.Info.PageNumber
+= 1 End
If
If e.Info.PageNumber
>= 8
Then e.Info.PageNumber
= Nothing End
If
e.Tag =
e.Info.PageNumber ElseIf
e.Info.SourceType = ProcessingSourceType.PageContent
Then If (_pagesAdded Mod 2) =
0 Then _doc.Page
= _doc.AddPage() End
If
Dim width As Double =
_doc.MediaBox.Height Dim
height As Double =
_doc.MediaBox.Width Dim scale
As Double = Math.Min((width - 4 * _margin) / (2 *
e.Info.Width.Value),
_ (height - 2 *
_margin) /
e.Info.Height.Value)
Dim
rectWidth As Double = scale *
e.Info.Width.Value Dim
rectHeight As Double = scale *
e.Info.Height.Value
Dim
distanceX As Double = (width - 2 * rectWidth) /
3 Dim distanceY As Double =
(height - rectHeight) /
2
_doc.Rect.SetRect(distanceX
+ (_pagesAdded Mod 2) * (distanceX + rectWidth),
_ distanceY,
rectWidth,
rectHeight)
e.Info.Handled
= True _pagesAdded +=
1 End If End
Sub
Public Sub Processed(sender As Object, e As
ProcessedObjectEventArgs) If e.Successful
Then Dim pixmap As PixMap =
TryCast(e.Object, PixMap) If
pixmap IsNot Nothing
Then pixmap.Compress() End
If
Dim graphic As
GraphicLayer = TryCast(e.Object,
GraphicLayer) If graphic
IsNot Nothing
Then _doc.FrameRect()
Dim
pageNumber As Integer =
CInt(e.Tag) _doc.FontSize
= 16 _doc.TextStyle.HPos =
0.5 _doc.Rect.Top
= _doc.Rect.Bottom -
_margin _doc.Rect.Bottom
=
_doc.MediaBox.Bottom _doc.AddText(String.Format("Page
{0}", pageNumber)) End
If End If End Sub End
Class
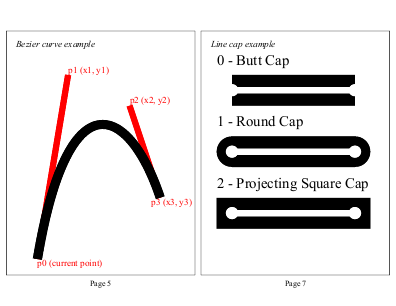
|
|
|