The following show the effect of increasing and decreasing contrast.
[C#]
void function() {
using (Doc doc = new Doc()) {
AddImagePage(doc, img2); // original image
doc.Rendering.Save("EffectContrast.jpg");
using (ImageLayer layer = AddImagePage(doc, img2)) {
using (EffectOperation effect = new EffectOperation("Contrast")) {
effect.Parameters["Amount"].Value = 25;
effect.Apply(layer.PixMap);
}
}
doc.Rendering.Save("EffectContrast25.jpg");
using (ImageLayer layer = AddImagePage(doc, img2)) {
using (EffectOperation effect = new EffectOperation("Contrast")) {
effect.Parameters["Amount"].Value = 50;
effect.Apply(layer.PixMap);
}
}
doc.Rendering.Save("EffectContrast50.jpg");
using (ImageLayer layer = AddImagePage(doc, img2)) {
using (EffectOperation effect = new EffectOperation("Contrast")) {
effect.Parameters["Amount"].Value = -50;
effect.Apply(layer.PixMap);
}
}
doc.Rendering.Save("EffectContrast-50.jpg");
}
}
[Visual Basic]
Sub ...
Using doc As New Doc()
AddImagePage(doc, img2)
' original image
doc.Rendering.Save("EffectContrast.jpg")
Using layer As ImageLayer = AddImagePage(doc, img2)
Using effect As New EffectOperation("Contrast")
effect.Parameters("Amount").Value = 25
effect.Apply(layer.PixMap)
End Using
End Using
doc.Rendering.Save("EffectContrast25.jpg")
Using layer As ImageLayer = AddImagePage(doc, img2)
Using effect As New EffectOperation("Contrast")
effect.Parameters("Amount").Value = 50
effect.Apply(layer.PixMap)
End Using
End Using
doc.Rendering.Save("EffectContrast50.jpg")
Using layer As ImageLayer = AddImagePage(doc, img2)
Using effect As New EffectOperation("Contrast")
effect.Parameters("Amount").Value = -50
effect.Apply(layer.PixMap)
End Using
End Using
doc.Rendering.Save("EffectContrast-50.jpg")
End Using
End Sub
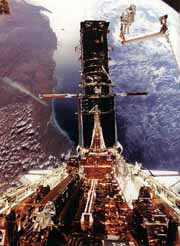
Original Image
|
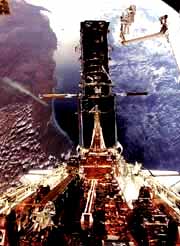
Contrast Amount 25
|
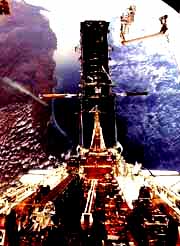
Contrast Amount 50
|
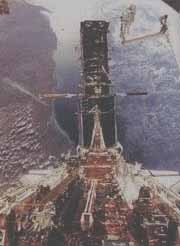
Contrast Amount -50
|
|
|
|