The following example shows how to use this method to generate various types of drop shadows.
[C#]
void function() {
using (Doc doc = new Doc()) {
// light blue background
doc.Color.SetCmyk(50, 0, 0, 0);
doc.FillRect();
doc.Color.SetRgb(0, 0, 0);
doc.Rect.Inset(20, 20);
doc.Rect.Pin = XRect.Corner.TopLeft;
doc.Rect.Height = doc.Rect.Height / 5;
// set up styles
doc.TextStyle.Size = 72;
double shift = doc.TextStyle.Size * 0.1;
XColor pink = new XColor();
pink.SetRgb(255, 128, 128);
XColor gray = new XColor();
gray.SetRgb(128, 128, 128);
XColor blue = new XColor();
blue.SetRgb(0, 0, 255);
// add text content
AddDropShadow(doc, doc.AddText("Sharp Shadow"), 0, shift, -shift, gray);
doc.Rect.Move(0, -doc.Rect.Height);
AddDropShadow(doc, doc.AddText("Blurred Shadow"), 1, shift, -shift, gray);
doc.Rect.Move(0, -doc.Rect.Height);
AddDropShadow(doc, doc.AddText("Pink Shadow"), 1, shift, -shift, pink);
doc.Rect.Move(0, -doc.Rect.Height);
// add drawn content
doc.Transform.Magnify(0.5, 0.5, 0, 0);
doc.Transform.Translate(50, 0);
string star = "124 158 300 700 476 158 15 493 585 493 124 158";
doc.Width = 20;
doc.Color.String = "255 0 0";
AddDropShadow(doc, doc.AddPoly(star, false), 3, shift, -shift, blue);
doc.Save("dropshadows.pdf");
}
}
void AddDropShadow(Doc doc, int id, double gaussianBlurRadius, double shadowHorizontalShift, double shadowVerticalShift, XColor shadowColor) {
string rect = doc.Rect.String;
string transform = doc.Transform.String;
string color = doc.Color.String;
double dpiX = doc.Rendering.DotsPerInchX;
double dpiY = doc.Rendering.DotsPerInchY;
bool saveAlpha = doc.Rendering.SaveAlpha;
int docLayer = doc.Layer;
try {
doc.Rendering.DotsPerInch = 72;
doc.Rendering.SaveAlpha = true;
Layer layer = doc.ObjectSoup[id] as Layer;
Page page = doc.ObjectSoup[doc.Page] as Page;
Bitmap bm = page.GetBitmap(new Layer[] { layer });
doc.Transform.Reset();
doc.Rect.String = layer.Rect.String;
doc.Rect.Move(shadowHorizontalShift, shadowVerticalShift);
doc.Layer = docLayer + 1;
int pid = doc.AddImageBitmap(bm, true);
// Here we set the base image to be one pixel of an appropriate color.
// This is what will determine the shadow color.
ImageLayer img = doc.ObjectSoup[pid] as ImageLayer;
PixMap pm = img.PixMap;
pm.ClearData(); // this will remove any compression settings
pm.SetData(new byte[] { (byte)shadowColor.Red, (byte)shadowColor.Green, (byte)shadowColor.Blue });
pm.Width = 1;
pm.Height = 1;
// The alpha channel is held as a separate soft mask and this is what will
// determine the shape of the shadow. If required we blur it to give it
// soft edges.
PixMap alpha = pm.SMask;
if (gaussianBlurRadius > 0) {
using (EffectOperation effect = new EffectOperation("Gaussian Blur")) {
effect.Parameters["Radius"].Value = gaussianBlurRadius;
effect.Apply(alpha);
}
}
}
finally {
doc.Rect.String = rect;
doc.Transform.String = transform;
doc.Color.String = color;
doc.Rendering.DotsPerInchX = dpiX;
doc.Rendering.DotsPerInchY = dpiY;
doc.Rendering.SaveAlpha = saveAlpha;
doc.Layer = docLayer;
}
}
[Visual Basic]
Sub ...
Using doc As New Doc()
' light blue background
doc.Color.SetCmyk(50, 0, 0, 0)
doc.FillRect()
doc.Color.SetRgb(0, 0, 0)
doc.Rect.Inset(20, 20)
doc.Rect.Pin = XRect.Corner.TopLeft
doc.Rect.Height = doc.Rect.Height / 5
' set up styles
doc.TextStyle.Size = 72
Dim shift As Double = doc.TextStyle.Size * 0.1
Dim pink As New XColor()
pink.SetRgb(255, 128, 128)
Dim gray As New XColor()
gray.SetRgb(128, 128, 128)
Dim blue As New XColor()
blue.SetRgb(0, 0, 255)
' add text content
AddDropShadow(doc, doc.AddText("Sharp Shadow"), 0, shift, -shift, gray)
doc.Rect.Move(0, -doc.Rect.Height)
AddDropShadow(doc, doc.AddText("Blurred Shadow"), 1, shift, -shift, gray)
doc.Rect.Move(0, -doc.Rect.Height)
AddDropShadow(doc, doc.AddText("Pink Shadow"), 1, shift, -shift, pink)
doc.Rect.Move(0, -doc.Rect.Height)
' add drawn content
doc.Transform.Magnify(0.5, 0.5, 0, 0)
doc.Transform.Translate(50, 0)
Dim star As String = "124 158 300 700 476 158 15 493 585 493 124 158"
doc.Width = 20
doc.Color.[String] = "255 0 0"
AddDropShadow(doc, doc.AddPoly(star, False), 3, shift, -shift, blue)
doc.Save("dropshadows.pdf")
End Using
End Sub
Private Sub AddDropShadow(doc As Doc, id As Integer, gaussianBlurRadius As Double, shadowHorizontalShift As Double, shadowVerticalShift As Double, shadowColor As XColor)
Dim rect As String = doc.Rect.[String]
Dim transform As String = doc.Transform.[String]
Dim color As String = doc.Color.[String]
Dim dpiX As Double = doc.Rendering.DotsPerInchX
Dim dpiY As Double = doc.Rendering.DotsPerInchY
Dim saveAlpha As Boolean = doc.Rendering.SaveAlpha
Dim docLayer As Integer = doc.Layer
Try
doc.Rendering.DotsPerInch = 72
doc.Rendering.SaveAlpha = True
Dim layer As Layer = TryCast(doc.ObjectSoup(id), Layer)
Dim page As Page = TryCast(doc.ObjectSoup(doc.Page), Page)
Dim bm As Bitmap = page.GetBitmap(New Layer() {layer})
doc.Transform.Reset()
doc.Rect.[String] = layer.Rect.[String]
doc.Rect.Move(shadowHorizontalShift, shadowVerticalShift)
doc.Layer = docLayer + 1
Dim pid As Integer = doc.AddImageBitmap(bm, True)
' Here we set the base image to be one pixel of an appropriate color.
' This is what will determine the shadow color.
Dim img As ImageLayer = TryCast(doc.ObjectSoup(pid), ImageLayer)
Dim pm As PixMap = img.PixMap
pm.ClearData()
' this will remove any compression settings
pm.SetData(New Byte() {CByte(shadowColor.Red), CByte(shadowColor.Green), CByte(shadowColor.Blue)})
pm.Width = 1
pm.Height = 1
' The alpha channel is held as a separate soft mask and this is what will
' determine the shape of the shadow. If required we blur it to give it
' soft edges.
Dim alpha As PixMap = pm.SMask
If gaussianBlurRadius > 0 Then
Using effect As New EffectOperation("Gaussian Blur")
effect.Parameters("Radius").Value = gaussianBlurRadius
effect.Apply(alpha)
End Using
End If
Finally
doc.Rect.[String] = rect
doc.Transform.[String] = transform
doc.Color.[String] = color
doc.Rendering.DotsPerInchX = dpiX
doc.Rendering.DotsPerInchY = dpiY
doc.Rendering.SaveAlpha = saveAlpha
doc.Layer = docLayer
End Try
End Sub
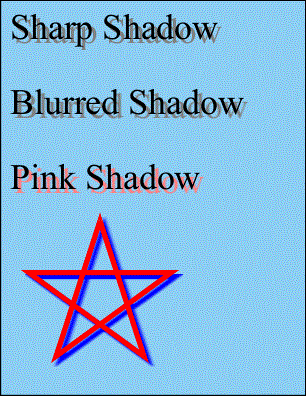
dropshadows.pdf
|
|
|