Abstract base class for streamed audio sources. More...
#include <SoundStream.hpp>
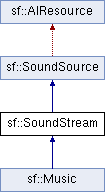
Classes | |
struct | Chunk |
Structure defining a chunk of audio data to stream. More... | |
Public Types | |
enum | Status { Stopped, Paused, Playing } |
Enumeration of the sound source states. More... | |
Public Member Functions | |
virtual | ~SoundStream () |
Destructor. More... | |
void | play () |
Start or resume playing the audio stream. More... | |
void | pause () |
Pause the audio stream. More... | |
void | stop () |
Stop playing the audio stream. More... | |
unsigned int | getChannelCount () const |
Return the number of channels of the stream. More... | |
unsigned int | getSampleRate () const |
Get the stream sample rate of the stream. More... | |
Status | getStatus () const |
Get the current status of the stream (stopped, paused, playing) More... | |
void | setPlayingOffset (Time timeOffset) |
Change the current playing position of the stream. More... | |
Time | getPlayingOffset () const |
Get the current playing position of the stream. More... | |
void | setLoop (bool loop) |
Set whether or not the stream should loop after reaching the end. More... | |
bool | getLoop () const |
Tell whether or not the stream is in loop mode. More... | |
void | setPitch (float pitch) |
Set the pitch of the sound. More... | |
void | setVolume (float volume) |
Set the volume of the sound. More... | |
void | setPosition (float x, float y, float z) |
Set the 3D position of the sound in the audio scene. More... | |
void | setPosition (const Vector3f &position) |
Set the 3D position of the sound in the audio scene. More... | |
void | setRelativeToListener (bool relative) |
Make the sound's position relative to the listener or absolute. More... | |
void | setMinDistance (float distance) |
Set the minimum distance of the sound. More... | |
void | setAttenuation (float attenuation) |
Set the attenuation factor of the sound. More... | |
float | getPitch () const |
Get the pitch of the sound. More... | |
float | getVolume () const |
Get the volume of the sound. More... | |
Vector3f | getPosition () const |
Get the 3D position of the sound in the audio scene. More... | |
bool | isRelativeToListener () const |
Tell whether the sound's position is relative to the listener or is absolute. More... | |
float | getMinDistance () const |
Get the minimum distance of the sound. More... | |
float | getAttenuation () const |
Get the attenuation factor of the sound. More... | |
Protected Member Functions | |
SoundStream () | |
Default constructor. More... | |
void | initialize (unsigned int channelCount, unsigned int sampleRate) |
Define the audio stream parameters. More... | |
virtual bool | onGetData (Chunk &data)=0 |
Request a new chunk of audio samples from the stream source. More... | |
virtual void | onSeek (Time timeOffset)=0 |
Change the current playing position in the stream source. More... | |
Protected Attributes | |
unsigned int | m_source |
OpenAL source identifier. More... | |
Detailed Description
Abstract base class for streamed audio sources.
Unlike audio buffers (see sf::SoundBuffer), audio streams are never completely loaded in memory.
Instead, the audio data is acquired continuously while the stream is playing. This behavior allows to play a sound with no loading delay, and keeps the memory consumption very low.
Sound sources that need to be streamed are usually big files (compressed audio musics that would eat hundreds of MB in memory) or files that would take a lot of time to be received (sounds played over the network).
sf::SoundStream is a base class that doesn't care about the stream source, which is left to the derived class. SFML provides a built-in specialization for big files (see sf::Music). No network stream source is provided, but you can write your own by combining this class with the network module.
A derived class has to override two virtual functions:
- onGetData fills a new chunk of audio data to be played
- onSeek changes the current playing position in the source
It is important to note that each SoundStream is played in its own separate thread, so that the streaming loop doesn't block the rest of the program. In particular, the OnGetData and OnSeek virtual functions may sometimes be called from this separate thread. It is important to keep this in mind, because you may have to take care of synchronization issues if you share data between threads.
Usage example:
- See also
- sf::Music
Definition at line 45 of file SoundStream.hpp.
Member Enumeration Documentation
|
inherited |
Enumeration of the sound source states.
Enumerator | |
---|---|
Stopped |
Sound is not playing. |
Paused |
Sound is paused. |
Playing |
Sound is playing. |
Definition at line 50 of file SoundSource.hpp.
Constructor & Destructor Documentation
|
virtual |
Destructor.
|
protected |
Default constructor.
This constructor is only meant to be called by derived classes.
Member Function Documentation
|
inherited |
Get the attenuation factor of the sound.
- Returns
- Attenuation factor of the sound
- See also
- setAttenuation, getMinDistance
unsigned int sf::SoundStream::getChannelCount | ( | ) | const |
Return the number of channels of the stream.
1 channel means a mono sound, 2 means stereo, etc.
- Returns
- Number of channels
bool sf::SoundStream::getLoop | ( | ) | const |
Tell whether or not the stream is in loop mode.
- Returns
- True if the stream is looping, false otherwise
- See also
- setLoop
|
inherited |
Get the minimum distance of the sound.
- Returns
- Minimum distance of the sound
- See also
- setMinDistance, getAttenuation
|
inherited |
Time sf::SoundStream::getPlayingOffset | ( | ) | const |
Get the current playing position of the stream.
- Returns
- Current playing position, from the beginning of the stream
- See also
- setPlayingOffset
|
inherited |
Get the 3D position of the sound in the audio scene.
- Returns
- Position of the sound
- See also
- setPosition
unsigned int sf::SoundStream::getSampleRate | ( | ) | const |
Get the stream sample rate of the stream.
The sample rate is the number of audio samples played per second. The higher, the better the quality.
- Returns
- Sample rate, in number of samples per second
Status sf::SoundStream::getStatus | ( | ) | const |
Get the current status of the stream (stopped, paused, playing)
- Returns
- Current status
|
inherited |
|
protected |
Define the audio stream parameters.
This function must be called by derived classes as soon as they know the audio settings of the stream to play. Any attempt to manipulate the stream (play(), ...) before calling this function will fail. It can be called multiple times if the settings of the audio stream change, but only when the stream is stopped.
- Parameters
-
channelCount Number of channels of the stream sampleRate Sample rate, in samples per second
|
inherited |
Tell whether the sound's position is relative to the listener or is absolute.
- Returns
- True if the position is relative, false if it's absolute
- See also
- setRelativeToListener
|
protectedpure virtual |
Request a new chunk of audio samples from the stream source.
This function must be overridden by derived classes to provide the audio samples to play. It is called continuously by the streaming loop, in a separate thread. The source can choose to stop the streaming loop at any time, by returning false to the caller. If you return true (i.e. continue streaming) it is important that the returned array of samples is not empty; this would stop the stream due to an internal limitation.
- Parameters
-
data Chunk of data to fill
- Returns
- True to continue playback, false to stop
Implemented in sf::Music.
|
protectedpure virtual |
Change the current playing position in the stream source.
This function must be overridden by derived classes to allow random seeking into the stream source.
- Parameters
-
timeOffset New playing position, relative to the beginning of the stream
Implemented in sf::Music.
void sf::SoundStream::pause | ( | ) |
void sf::SoundStream::play | ( | ) |
Start or resume playing the audio stream.
This function starts the stream if it was stopped, resumes it if it was paused, and restarts it from the beginning if it was already playing. This function uses its own thread so that it doesn't block the rest of the program while the stream is played.
|
inherited |
Set the attenuation factor of the sound.
The attenuation is a multiplicative factor which makes the sound more or less loud according to its distance from the listener. An attenuation of 0 will produce a non-attenuated sound, i.e. its volume will always be the same whether it is heard from near or from far. On the other hand, an attenuation value such as 100 will make the sound fade out very quickly as it gets further from the listener. The default value of the attenuation is 1.
- Parameters
-
attenuation New attenuation factor of the sound
- See also
- getAttenuation, setMinDistance
void sf::SoundStream::setLoop | ( | bool | loop | ) |
Set whether or not the stream should loop after reaching the end.
If set, the stream will restart from beginning after reaching the end and so on, until it is stopped or setLoop(false) is called. The default looping state for streams is false.
- Parameters
-
loop True to play in loop, false to play once
- See also
- getLoop
|
inherited |
Set the minimum distance of the sound.
The "minimum distance" of a sound is the maximum distance at which it is heard at its maximum volume. Further than the minimum distance, it will start to fade out according to its attenuation factor. A value of 0 ("inside the head of the listener") is an invalid value and is forbidden. The default value of the minimum distance is 1.
- Parameters
-
distance New minimum distance of the sound
- See also
- getMinDistance, setAttenuation
|
inherited |
Set the pitch of the sound.
The pitch represents the perceived fundamental frequency of a sound; thus you can make a sound more acute or grave by changing its pitch. A side effect of changing the pitch is to modify the playing speed of the sound as well. The default value for the pitch is 1.
- Parameters
-
pitch New pitch to apply to the sound
- See also
- getPitch
void sf::SoundStream::setPlayingOffset | ( | Time | timeOffset | ) |
Change the current playing position of the stream.
The playing position can be changed when the stream is either paused or playing. Changing the playing position when the stream is stopped has no effect, since playing the stream would reset its position.
- Parameters
-
timeOffset New playing position, from the beginning of the stream
- See also
- getPlayingOffset
|
inherited |
Set the 3D position of the sound in the audio scene.
Only sounds with one channel (mono sounds) can be spatialized. The default position of a sound is (0, 0, 0).
- Parameters
-
x X coordinate of the position of the sound in the scene y Y coordinate of the position of the sound in the scene z Z coordinate of the position of the sound in the scene
- See also
- getPosition
|
inherited |
Set the 3D position of the sound in the audio scene.
Only sounds with one channel (mono sounds) can be spatialized. The default position of a sound is (0, 0, 0).
- Parameters
-
position Position of the sound in the scene
- See also
- getPosition
|
inherited |
Make the sound's position relative to the listener or absolute.
Making a sound relative to the listener will ensure that it will always be played the same way regardless of the position of the listener. This can be useful for non-spatialized sounds, sounds that are produced by the listener, or sounds attached to it. The default value is false (position is absolute).
- Parameters
-
relative True to set the position relative, false to set it absolute
- See also
- isRelativeToListener
|
inherited |
Set the volume of the sound.
The volume is a value between 0 (mute) and 100 (full volume). The default value for the volume is 100.
- Parameters
-
volume Volume of the sound
- See also
- getVolume
void sf::SoundStream::stop | ( | ) |
Member Data Documentation
|
protectedinherited |
OpenAL source identifier.
Definition at line 274 of file SoundSource.hpp.
The documentation for this class was generated from the following file: