Specialized SoundRecorder which stores the captured audio data into a sound buffer. More...
#include <SoundBufferRecorder.hpp>
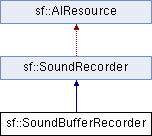
Public Member Functions | |
~SoundBufferRecorder () | |
destructor More... | |
const SoundBuffer & | getBuffer () const |
Get the sound buffer containing the captured audio data. More... | |
bool | start (unsigned int sampleRate=44100) |
Start the capture. More... | |
void | stop () |
Stop the capture. More... | |
unsigned int | getSampleRate () const |
Get the sample rate. More... | |
bool | setDevice (const std::string &name) |
Set the audio capture device. More... | |
const std::string & | getDevice () const |
Get the name of the current audio capture device. More... | |
void | setChannelCount (unsigned int channelCount) |
Set the channel count of the audio capture device. More... | |
unsigned int | getChannelCount () const |
Get the number of channels used by this recorder. More... | |
Static Public Member Functions | |
static std::vector< std::string > | getAvailableDevices () |
Get a list of the names of all available audio capture devices. More... | |
static std::string | getDefaultDevice () |
Get the name of the default audio capture device. More... | |
static bool | isAvailable () |
Check if the system supports audio capture. More... | |
Protected Member Functions | |
virtual bool | onStart () |
Start capturing audio data. More... | |
virtual bool | onProcessSamples (const Int16 *samples, std::size_t sampleCount) |
Process a new chunk of recorded samples. More... | |
virtual void | onStop () |
Stop capturing audio data. More... | |
void | setProcessingInterval (Time interval) |
Set the processing interval. More... | |
Detailed Description
Specialized SoundRecorder which stores the captured audio data into a sound buffer.
sf::SoundBufferRecorder allows to access a recorded sound through a sf::SoundBuffer, so that it can be played, saved to a file, etc.
It has the same simple interface as its base class (start(), stop()) and adds a function to retrieve the recorded sound buffer (getBuffer()).
As usual, don't forget to call the isAvailable() function before using this class (see sf::SoundRecorder for more details about this).
Usage example:
- See also
- sf::SoundRecorder
Definition at line 44 of file SoundBufferRecorder.hpp.
Constructor & Destructor Documentation
sf::SoundBufferRecorder::~SoundBufferRecorder | ( | ) |
destructor
Member Function Documentation
|
staticinherited |
Get a list of the names of all available audio capture devices.
This function returns a vector of strings, containing the names of all available audio capture devices.
- Returns
- A vector of strings containing the names
const SoundBuffer& sf::SoundBufferRecorder::getBuffer | ( | ) | const |
Get the sound buffer containing the captured audio data.
The sound buffer is valid only after the capture has ended. This function provides a read-only access to the internal sound buffer, but it can be copied if you need to make any modification to it.
- Returns
- Read-only access to the sound buffer
|
inherited |
Get the number of channels used by this recorder.
Currently only mono and stereo are supported, so the value is either 1 (for mono) or 2 (for stereo).
- Returns
- Number of channels
- See also
- setChannelCount
|
staticinherited |
Get the name of the default audio capture device.
This function returns the name of the default audio capture device. If none is available, an empty string is returned.
- Returns
- The name of the default audio capture device
|
inherited |
Get the name of the current audio capture device.
- Returns
- The name of the current audio capture device
|
inherited |
Get the sample rate.
The sample rate defines the number of audio samples captured per second. The higher, the better the quality (for example, 44100 samples/sec is CD quality).
- Returns
- Sample rate, in samples per second
|
staticinherited |
Check if the system supports audio capture.
This function should always be called before using the audio capture features. If it returns false, then any attempt to use sf::SoundRecorder or one of its derived classes will fail.
- Returns
- True if audio capture is supported, false otherwise
|
protectedvirtual |
Process a new chunk of recorded samples.
- Parameters
-
samples Pointer to the new chunk of recorded samples sampleCount Number of samples pointed by samples
- Returns
- True to continue the capture, or false to stop it
Implements sf::SoundRecorder.
|
protectedvirtual |
Start capturing audio data.
- Returns
- True to start the capture, or false to abort it
Reimplemented from sf::SoundRecorder.
|
protectedvirtual |
Stop capturing audio data.
Reimplemented from sf::SoundRecorder.
|
inherited |
Set the channel count of the audio capture device.
This method allows you to specify the number of channels used for recording. Currently only 16-bit mono and 16-bit stereo are supported.
- Parameters
-
channelCount Number of channels. Currently only mono (1) and stereo (2) are supported.
- See also
- getChannelCount
|
inherited |
Set the audio capture device.
This function sets the audio capture device to the device with the given name. It can be called on the fly (i.e: while recording). If you do so while recording and opening the device fails, it stops the recording.
- Parameters
-
name The name of the audio capture device
- Returns
- True, if it was able to set the requested device
- See also
- getAvailableDevices, getDefaultDevice
|
protectedinherited |
Set the processing interval.
The processing interval controls the period between calls to the onProcessSamples function. You may want to use a small interval if you want to process the recorded data in real time, for example.
Note: this is only a hint, the actual period may vary. So don't rely on this parameter to implement precise timing.
The default processing interval is 100 ms.
- Parameters
-
interval Processing interval
|
inherited |
Start the capture.
The sampleRate parameter defines the number of audio samples captured per second. The higher, the better the quality (for example, 44100 samples/sec is CD quality). This function uses its own thread so that it doesn't block the rest of the program while the capture runs. Please note that only one capture can happen at the same time. You can select which capture device will be used, by passing the name to the setDevice() method. If none was selected before, the default capture device will be used. You can get a list of the names of all available capture devices by calling getAvailableDevices().
- Parameters
-
sampleRate Desired capture rate, in number of samples per second
- Returns
- True, if start of capture was successful
- See also
- stop, getAvailableDevices
|
inherited |
Stop the capture.
- See also
- start
The documentation for this class was generated from the following file: