Socket that listens to new TCP connections. More...
#include <TcpListener.hpp>
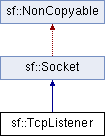
Public Types | |
enum | Status { Done, NotReady, Partial, Disconnected, Error } |
Status codes that may be returned by socket functions. More... | |
enum | { AnyPort = 0 } |
Some special values used by sockets. More... | |
Public Member Functions | |
TcpListener () | |
Default constructor. More... | |
unsigned short | getLocalPort () const |
Get the port to which the socket is bound locally. More... | |
Status | listen (unsigned short port, const IpAddress &address=IpAddress::Any) |
Start listening for connections. More... | |
void | close () |
Stop listening and close the socket. More... | |
Status | accept (TcpSocket &socket) |
Accept a new connection. More... | |
void | setBlocking (bool blocking) |
Set the blocking state of the socket. More... | |
bool | isBlocking () const |
Tell whether the socket is in blocking or non-blocking mode. More... | |
Protected Types | |
enum | Type { Tcp, Udp } |
Types of protocols that the socket can use. More... | |
Protected Member Functions | |
SocketHandle | getHandle () const |
Return the internal handle of the socket. More... | |
void | create () |
Create the internal representation of the socket. More... | |
void | create (SocketHandle handle) |
Create the internal representation of the socket from a socket handle. More... | |
Detailed Description
Socket that listens to new TCP connections.
A listener socket is a special type of socket that listens to a given port and waits for connections on that port.
This is all it can do.
When a new connection is received, you must call accept and the listener returns a new instance of sf::TcpSocket that is properly initialized and can be used to communicate with the new client.
Listener sockets are specific to the TCP protocol, UDP sockets are connectionless and can therefore communicate directly. As a consequence, a listener socket will always return the new connections as sf::TcpSocket instances.
A listener is automatically closed on destruction, like all other types of socket. However if you want to stop listening before the socket is destroyed, you can call its close() function.
Usage example:
- See also
- sf::TcpSocket, sf::Socket
Definition at line 44 of file TcpListener.hpp.
Member Enumeration Documentation
|
inherited |
Some special values used by sockets.
Enumerator | |
---|---|
AnyPort |
Special value that tells the system to pick any available port. |
Definition at line 66 of file Socket.hpp.
|
inherited |
Status codes that may be returned by socket functions.
Definition at line 53 of file Socket.hpp.
|
protectedinherited |
Types of protocols that the socket can use.
Enumerator | |
---|---|
Tcp |
TCP protocol. |
Udp |
UDP protocol. |
Definition at line 114 of file Socket.hpp.
Constructor & Destructor Documentation
sf::TcpListener::TcpListener | ( | ) |
Default constructor.
Member Function Documentation
void sf::TcpListener::close | ( | ) |
Stop listening and close the socket.
This function gracefully stops the listener. If the socket is not listening, this function has no effect.
- See also
- listen
|
protectedinherited |
Create the internal representation of the socket.
This function can only be accessed by derived classes.
|
protectedinherited |
Create the internal representation of the socket from a socket handle.
This function can only be accessed by derived classes.
- Parameters
-
handle OS-specific handle of the socket to wrap
|
protectedinherited |
Return the internal handle of the socket.
The returned handle may be invalid if the socket was not created yet (or already destroyed). This function can only be accessed by derived classes.
- Returns
- The internal (OS-specific) handle of the socket
unsigned short sf::TcpListener::getLocalPort | ( | ) | const |
Get the port to which the socket is bound locally.
If the socket is not listening to a port, this function returns 0.
- Returns
- Port to which the socket is bound
- See also
- listen
|
inherited |
Tell whether the socket is in blocking or non-blocking mode.
- Returns
- True if the socket is blocking, false otherwise
- See also
- setBlocking
Status sf::TcpListener::listen | ( | unsigned short | port, |
const IpAddress & | address = IpAddress::Any |
||
) |
Start listening for connections.
This functions makes the socket listen to the specified port, waiting for new connections. If the socket was previously listening to another port, it will be stopped first and bound to the new port.
- Parameters
-
port Port to listen for new connections address Address of the interface to listen on
- Returns
- Status code
|
inherited |
Set the blocking state of the socket.
In blocking mode, calls will not return until they have completed their task. For example, a call to Receive in blocking mode won't return until some data was actually received. In non-blocking mode, calls will always return immediately, using the return code to signal whether there was data available or not. By default, all sockets are blocking.
- Parameters
-
blocking True to set the socket as blocking, false for non-blocking
- See also
- isBlocking
The documentation for this class was generated from the following file: