Provide read access to sound files. More...
#include <InputSoundFile.hpp>
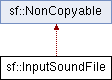
Public Member Functions | |
InputSoundFile () | |
Default constructor. More... | |
~InputSoundFile () | |
Destructor. More... | |
bool | openFromFile (const std::string &filename) |
Open a sound file from the disk for reading. More... | |
bool | openFromMemory (const void *data, std::size_t sizeInBytes) |
Open a sound file in memory for reading. More... | |
bool | openFromStream (InputStream &stream) |
Open a sound file from a custom stream for reading. More... | |
bool | openForWriting (const std::string &filename, unsigned int channelCount, unsigned int sampleRate) |
Open the sound file from the disk for writing. More... | |
Uint64 | getSampleCount () const |
Get the total number of audio samples in the file. More... | |
unsigned int | getChannelCount () const |
Get the number of channels used by the sound. More... | |
unsigned int | getSampleRate () const |
Get the sample rate of the sound. More... | |
Time | getDuration () const |
Get the total duration of the sound file. More... | |
void | seek (Uint64 sampleOffset) |
Change the current read position to the given sample offset. More... | |
void | seek (Time timeOffset) |
Change the current read position to the given time offset. More... | |
Uint64 | read (Int16 *samples, Uint64 maxCount) |
Read audio samples from the open file. More... | |
Detailed Description
Provide read access to sound files.
This class decodes audio samples from a sound file.
It is used internally by higher-level classes such as sf::SoundBuffer and sf::Music, but can also be useful if you want to process or analyze audio files without playing them, or if you want to implement your own version of sf::Music with more specific features.
Usage example:
- See also
- sf::SoundFileReader, sf::OutputSoundFile
Definition at line 46 of file InputSoundFile.hpp.
Constructor & Destructor Documentation
sf::InputSoundFile::InputSoundFile | ( | ) |
Default constructor.
sf::InputSoundFile::~InputSoundFile | ( | ) |
Destructor.
Member Function Documentation
unsigned int sf::InputSoundFile::getChannelCount | ( | ) | const |
Get the number of channels used by the sound.
- Returns
- Number of channels (1 = mono, 2 = stereo)
Time sf::InputSoundFile::getDuration | ( | ) | const |
Get the total duration of the sound file.
This function is provided for convenience, the duration is deduced from the other sound file attributes.
- Returns
- Duration of the sound file
Uint64 sf::InputSoundFile::getSampleCount | ( | ) | const |
Get the total number of audio samples in the file.
- Returns
- Number of samples
unsigned int sf::InputSoundFile::getSampleRate | ( | ) | const |
Get the sample rate of the sound.
- Returns
- Sample rate, in samples per second
bool sf::InputSoundFile::openForWriting | ( | const std::string & | filename, |
unsigned int | channelCount, | ||
unsigned int | sampleRate | ||
) |
Open the sound file from the disk for writing.
- Parameters
-
filename Path of the sound file to write channelCount Number of channels in the sound sampleRate Sample rate of the sound
- Returns
- True if the file was successfully opened
bool sf::InputSoundFile::openFromFile | ( | const std::string & | filename | ) |
Open a sound file from the disk for reading.
The supported audio formats are: WAV (PCM only), OGG/Vorbis, FLAC. The supported sample sizes for FLAC and WAV are 8, 16, 24 and 32 bit.
- Parameters
-
filename Path of the sound file to load
- Returns
- True if the file was successfully opened
bool sf::InputSoundFile::openFromMemory | ( | const void * | data, |
std::size_t | sizeInBytes | ||
) |
Open a sound file in memory for reading.
The supported audio formats are: WAV (PCM only), OGG/Vorbis, FLAC. The supported sample sizes for FLAC and WAV are 8, 16, 24 and 32 bit.
- Parameters
-
data Pointer to the file data in memory sizeInBytes Size of the data to load, in bytes
- Returns
- True if the file was successfully opened
bool sf::InputSoundFile::openFromStream | ( | InputStream & | stream | ) |
Open a sound file from a custom stream for reading.
The supported audio formats are: WAV (PCM only), OGG/Vorbis, FLAC. The supported sample sizes for FLAC and WAV are 8, 16, 24 and 32 bit.
- Parameters
-
stream Source stream to read from
- Returns
- True if the file was successfully opened
Uint64 sf::InputSoundFile::read | ( | Int16 * | samples, |
Uint64 | maxCount | ||
) |
Read audio samples from the open file.
- Parameters
-
samples Pointer to the sample array to fill maxCount Maximum number of samples to read
- Returns
- Number of samples actually read (may be less than maxCount)
void sf::InputSoundFile::seek | ( | Uint64 | sampleOffset | ) |
Change the current read position to the given sample offset.
This function takes a sample offset to provide maximum precision. If you need to jump to a given time, use the other overload.
The sample offset takes the channels into account. Offsets can be calculated like this: sampleNumber * sampleRate * channelCount
If the given offset exceeds to total number of samples, this function jumps to the end of the sound file.
- Parameters
-
sampleOffset Index of the sample to jump to, relative to the beginning
void sf::InputSoundFile::seek | ( | Time | timeOffset | ) |
Change the current read position to the given time offset.
Using a time offset is handy but imprecise. If you need an accurate result, consider using the overload which takes a sample offset.
If the given time exceeds to total duration, this function jumps to the end of the sound file.
- Parameters
-
timeOffset Time to jump to, relative to the beginning
The documentation for this class was generated from the following file: