![]() |
X-CUBE-SPN11 for X-NUCLEO-IHM11M1
|
X-NUCLEO-IHM11M1.c
Go to the documentation of this file.
void STSPIN230_Current_Reference_Start()
Enable the Current Reference generation.
Definition: X-NUCLEO-IHM11M1.c:293
This file provides the set of functions to manage the X-Nucleo board.
void STSPIN230_HF_TIMx_SetDutyCycle_CH3(uint16_t CCR_value)
Set the Duty Cycle value for CH3.
Definition: X-NUCLEO-IHM11M1.c:274
void STSPIN230_EnableInput_CH1_E_CH2_D_CH3_E()
Enable Input channel CH1 and CH3 for STSPIN230.
Definition: X-NUCLEO-IHM11M1.c:104
void STSPIN230_HF_TIMx_SetDutyCycle_CH2(uint16_t CCR_value)
Set the Duty Cycle value for CH2.
Definition: X-NUCLEO-IHM11M1.c:250
void STSPIN230_DisableInput_CH1_D_CH2_D_CH3_D()
Enable Input channel CH2 and CH3 for STSPIN230.
Definition: X-NUCLEO-IHM11M1.c:154
This file provides the interface between the MC-lib and STM Nucleo.
void STSPIN230_EnableInput_CH1_D_CH2_E_CH3_E()
Enable Input channel CH2 and CH3 for STSPIN230.
Definition: X-NUCLEO-IHM11M1.c:129
void STSPIN230_HF_TIMx_SetDutyCycle_CH1(uint16_t CCR_value)
Set the Duty Cycle value for CH1.
Definition: X-NUCLEO-IHM11M1.c:230
This header file provides the set of functions for Motor Control library.
void STSPIN230_Current_Reference_Setvalue(uint16_t Iref)
Set the value for Current Reference.
Definition: X-NUCLEO-IHM11M1.c:334
void STSPIN230_Current_Reference_Stop()
Disable the Current Reference generation.
Definition: X-NUCLEO-IHM11M1.c:313
void STSPIN230_Start_PWM_driving()
Enable PWM channels for STSPIN230.
Definition: X-NUCLEO-IHM11M1.c:179
void STSPIN230_EnableInput_CH1_E_CH2_E_CH3_D()
Enable Input channel CH1 and CH2 for STSPIN230.
Definition: X-NUCLEO-IHM11M1.c:79
void STSPIN230_Stop_PWM_driving()
Disable PWM channels for STSPIN230.
Definition: X-NUCLEO-IHM11M1.c:204
Generated by
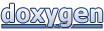