![]() |
X-CUBE-SPN11 for X-NUCLEO-IHM11M1
|
6Step_Lib.h
Go to the documentation of this file.
Definition: 6Step_Lib.h:72
Definition: 6Step_Lib.h:70
Definition: 6Step_Lib.h:71
Definition: 6Step_Lib.h:75
int16_t Upper_Limit_Output
Definition: 6Step_Lib.h:176
Definition: 6Step_Lib.h:76
Definition: 6Step_Lib.h:79
Definition: 6Step_Lib.h:77
Definition: 6Step_Lib.h:78
Definition: 6Step_Lib.h:73
uint16_t ADC_BEMF_threshold_UP
Definition: 6Step_Lib.h:113
uint16_t MediumFrequencyTask_flag
Definition: 6Step_Lib.h:142
uint32_t CurrentRegular_BEMF_ch
Definition: 6Step_Lib.h:107
Definition: 6Step_Lib.h:74
This file provides the interface between the MC-lib and STM Nucleo.
uint16_t ADC_BEMF_threshold_DOWN
Definition: 6Step_Lib.h:114
int16_t Lower_Limit_Output
Definition: 6Step_Lib.h:175
struct SIXSTEP_PI_PARAM_InitTypeDef_t * SIXSTEP_pi_PARAM_InitTypeDef_t
Definition: 6Step_Lib.h:80
Generated by
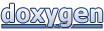