C:/nxpdrv/LPC1700CMSIS/Drivers/source/lpc17xx_gpio.c
Go to the documentation of this file.00001 00020 /* Peripheral group ----------------------------------------------------------- */ 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_gpio.h" 00027 00028 /* If this source file built with example, the LPC17xx FW library configuration 00029 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00030 * otherwise the default FW library configuration file must be included instead 00031 */ 00032 #ifdef __BUILD_WITH_EXAMPLE__ 00033 #include "lpc17xx_libcfg.h" 00034 #else 00035 #include "lpc17xx_libcfg_default.h" 00036 #endif /* __BUILD_WITH_EXAMPLE__ */ 00037 00038 00039 #ifdef _GPIO 00040 00041 /* Private Functions ---------------------------------------------------------- */ 00045 /*********************************************************************/ 00050 static LPC_GPIO_TypeDef *GPIO_GetPointer(uint8_t portNum) 00051 { 00052 LPC_GPIO_TypeDef *pGPIO = NULL; 00053 00054 switch (portNum) { 00055 case 0: 00056 pGPIO = LPC_GPIO0; 00057 break; 00058 case 1: 00059 pGPIO = LPC_GPIO1; 00060 break; 00061 case 2: 00062 pGPIO = LPC_GPIO2; 00063 break; 00064 case 3: 00065 pGPIO = LPC_GPIO3; 00066 break; 00067 case 4: 00068 pGPIO = LPC_GPIO4; 00069 break; 00070 default: 00071 break; 00072 } 00073 00074 return pGPIO; 00075 } 00076 00077 /*********************************************************************/ 00083 static GPIO_HalfWord_TypeDef *FIO_HalfWordGetPointer(uint8_t portNum) 00084 { 00085 GPIO_HalfWord_TypeDef *pFIO = NULL; 00086 00087 switch (portNum) { 00088 case 0: 00089 pFIO = GPIO0_HalfWord; 00090 break; 00091 case 1: 00092 pFIO = GPIO1_HalfWord; 00093 break; 00094 case 2: 00095 pFIO = GPIO2_HalfWord; 00096 break; 00097 case 3: 00098 pFIO = GPIO3_HalfWord; 00099 break; 00100 case 4: 00101 pFIO = GPIO4_HalfWord; 00102 break; 00103 default: 00104 break; 00105 } 00106 00107 return pFIO; 00108 } 00109 00110 /*********************************************************************/ 00116 static GPIO_Byte_TypeDef *FIO_ByteGetPointer(uint8_t portNum) 00117 { 00118 GPIO_Byte_TypeDef *pFIO = NULL; 00119 00120 switch (portNum) { 00121 case 0: 00122 pFIO = GPIO0_Byte; 00123 break; 00124 case 1: 00125 pFIO = GPIO1_Byte; 00126 break; 00127 case 2: 00128 pFIO = GPIO2_Byte; 00129 break; 00130 case 3: 00131 pFIO = GPIO3_Byte; 00132 break; 00133 case 4: 00134 pFIO = GPIO4_Byte; 00135 break; 00136 default: 00137 break; 00138 } 00139 00140 return pFIO; 00141 } 00142 00148 /* Public Functions ----------------------------------------------------------- */ 00154 /* GPIO ------------------------------------------------------------------------------ */ 00155 00156 /*********************************************************************/ 00170 void GPIO_SetDir(uint8_t portNum, uint32_t bitValue, uint8_t dir) 00171 { 00172 LPC_GPIO_TypeDef *pGPIO = GPIO_GetPointer(portNum); 00173 00174 if (pGPIO != NULL) { 00175 // Enable Output 00176 if (dir) { 00177 pGPIO->FIODIR |= bitValue; 00178 } 00179 // Enable Input 00180 else { 00181 pGPIO->FIODIR &= ~bitValue; 00182 } 00183 } 00184 } 00185 00186 00187 /*********************************************************************/ 00201 void GPIO_SetValue(uint8_t portNum, uint32_t bitValue) 00202 { 00203 LPC_GPIO_TypeDef *pGPIO = GPIO_GetPointer(portNum); 00204 00205 if (pGPIO != NULL) { 00206 pGPIO->FIOSET = bitValue; 00207 } 00208 } 00209 00210 /*********************************************************************/ 00224 void GPIO_ClearValue(uint8_t portNum, uint32_t bitValue) 00225 { 00226 LPC_GPIO_TypeDef *pGPIO = GPIO_GetPointer(portNum); 00227 00228 if (pGPIO != NULL) { 00229 pGPIO->FIOCLR = bitValue; 00230 } 00231 } 00232 00233 /*********************************************************************/ 00241 uint32_t GPIO_ReadValue(uint8_t portNum) 00242 { 00243 LPC_GPIO_TypeDef *pGPIO = GPIO_GetPointer(portNum); 00244 00245 if (pGPIO != NULL) { 00246 return pGPIO->FIOPIN; 00247 } 00248 00249 return (0); 00250 } 00251 00252 /* FIO word accessible ----------------------------------------------------------------- */ 00253 /* Stub function for FIO (word-accessible) style */ 00254 00258 void FIO_SetDir(uint8_t portNum, uint32_t bitValue, uint8_t dir) 00259 { 00260 GPIO_SetDir(portNum, bitValue, dir); 00261 } 00262 00266 void FIO_SetValue(uint8_t portNum, uint32_t bitValue) 00267 { 00268 GPIO_SetValue(portNum, bitValue); 00269 } 00270 00274 void FIO_ClearValue(uint8_t portNum, uint32_t bitValue) 00275 { 00276 GPIO_ClearValue(portNum, bitValue); 00277 } 00278 00282 uint32_t FIO_ReadValue(uint8_t portNum) 00283 { 00284 return (GPIO_ReadValue(portNum)); 00285 } 00286 00287 00288 /*********************************************************************/ 00306 void FIO_SetMask(uint8_t portNum, uint32_t bitValue, uint8_t maskValue) 00307 { 00308 LPC_GPIO_TypeDef *pFIO = GPIO_GetPointer(portNum); 00309 if(pFIO != NULL) { 00310 // Mask 00311 if (maskValue){ 00312 pFIO->FIOMASK |= bitValue; 00313 } 00314 // Un-mask 00315 else { 00316 pFIO->FIOMASK &= ~bitValue; 00317 } 00318 } 00319 } 00320 00321 00322 /* FIO halfword accessible ------------------------------------------------------------- */ 00323 00324 /*********************************************************************/ 00338 void FIO_HalfWordSetDir(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue, uint8_t dir) 00339 { 00340 GPIO_HalfWord_TypeDef *pFIO = FIO_HalfWordGetPointer(portNum); 00341 if(pFIO != NULL) { 00342 // Output direction 00343 if (dir) { 00344 // Upper 00345 if(halfwordNum) { 00346 pFIO->FIODIRU |= bitValue; 00347 } 00348 // lower 00349 else { 00350 pFIO->FIODIRL |= bitValue; 00351 } 00352 } 00353 // Input direction 00354 else { 00355 // Upper 00356 if(halfwordNum) { 00357 pFIO->FIODIRU &= ~bitValue; 00358 } 00359 // lower 00360 else { 00361 pFIO->FIODIRL &= ~bitValue; 00362 } 00363 } 00364 } 00365 } 00366 00367 00368 /*********************************************************************/ 00387 void FIO_HalfWordSetMask(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue, uint8_t maskValue) 00388 { 00389 GPIO_HalfWord_TypeDef *pFIO = FIO_HalfWordGetPointer(portNum); 00390 if(pFIO != NULL) { 00391 // Mask 00392 if (maskValue){ 00393 // Upper 00394 if(halfwordNum) { 00395 pFIO->FIOMASKU |= bitValue; 00396 } 00397 // lower 00398 else { 00399 pFIO->FIOMASKL |= bitValue; 00400 } 00401 } 00402 // Un-mask 00403 else { 00404 // Upper 00405 if(halfwordNum) { 00406 pFIO->FIOMASKU &= ~bitValue; 00407 } 00408 // lower 00409 else { 00410 pFIO->FIOMASKL &= ~bitValue; 00411 } 00412 } 00413 } 00414 } 00415 00416 00417 /*********************************************************************/ 00431 void FIO_HalfWordSetValue(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue) 00432 { 00433 GPIO_HalfWord_TypeDef *pFIO = FIO_HalfWordGetPointer(portNum); 00434 if(pFIO != NULL) { 00435 // Upper 00436 if(halfwordNum) { 00437 pFIO->FIOSETU = bitValue; 00438 } 00439 // lower 00440 else { 00441 pFIO->FIOSETL = bitValue; 00442 } 00443 } 00444 } 00445 00446 00447 /*********************************************************************/ 00461 void FIO_HalfWordClearValue(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue) 00462 { 00463 GPIO_HalfWord_TypeDef *pFIO = FIO_HalfWordGetPointer(portNum); 00464 if(pFIO != NULL) { 00465 // Upper 00466 if(halfwordNum) { 00467 pFIO->FIOCLRU = bitValue; 00468 } 00469 // lower 00470 else { 00471 pFIO->FIOCLRL = bitValue; 00472 } 00473 } 00474 } 00475 00476 00477 /*********************************************************************/ 00486 uint16_t FIO_HalfWordReadValue(uint8_t portNum, uint8_t halfwordNum) 00487 { 00488 GPIO_HalfWord_TypeDef *pFIO = FIO_HalfWordGetPointer(portNum); 00489 if(pFIO != NULL) { 00490 // Upper 00491 if(halfwordNum) { 00492 return (pFIO->FIOPINU); 00493 } 00494 // lower 00495 else { 00496 return (pFIO->FIOPINL); 00497 } 00498 } 00499 return (0); 00500 } 00501 00502 00503 /* FIO Byte accessible ------------------------------------------------------------ */ 00504 00505 /*********************************************************************/ 00519 void FIO_ByteSetDir(uint8_t portNum, uint8_t byteNum, uint8_t bitValue, uint8_t dir) 00520 { 00521 GPIO_Byte_TypeDef *pFIO = FIO_ByteGetPointer(portNum); 00522 if(pFIO != NULL) { 00523 // Output direction 00524 if (dir) { 00525 if ((byteNum >= 0) && (byteNum <= 3)) { 00526 pFIO->FIODIR[byteNum] |= bitValue; 00527 } 00528 } 00529 // Input direction 00530 else { 00531 if ((byteNum >= 0) && (byteNum <= 3)) { 00532 pFIO->FIODIR[byteNum] &= ~bitValue; 00533 } 00534 } 00535 } 00536 } 00537 00538 /*********************************************************************/ 00557 void FIO_ByteSetMask(uint8_t portNum, uint8_t byteNum, uint8_t bitValue, uint8_t maskValue) 00558 { 00559 GPIO_Byte_TypeDef *pFIO = FIO_ByteGetPointer(portNum); 00560 if(pFIO != NULL) { 00561 // Mask 00562 if (maskValue) { 00563 if ((byteNum >= 0) && (byteNum <= 3)) { 00564 pFIO->FIOMASK[byteNum] |= bitValue; 00565 } 00566 } 00567 // Un-mask 00568 else { 00569 if ((byteNum >= 0) && (byteNum <= 3)) { 00570 pFIO->FIOMASK[byteNum] &= ~bitValue; 00571 } 00572 } 00573 } 00574 } 00575 00576 00577 /*********************************************************************/ 00591 void FIO_ByteSetValue(uint8_t portNum, uint8_t byteNum, uint8_t bitValue) 00592 { 00593 GPIO_Byte_TypeDef *pFIO = FIO_ByteGetPointer(portNum); 00594 if (pFIO != NULL) { 00595 if ((byteNum >= 0) && (byteNum <= 3)){ 00596 pFIO->FIOSET[byteNum] = bitValue; 00597 } 00598 } 00599 } 00600 00601 00602 /*********************************************************************/ 00616 void FIO_ByteClearValue(uint8_t portNum, uint8_t byteNum, uint8_t bitValue) 00617 { 00618 GPIO_Byte_TypeDef *pFIO = FIO_ByteGetPointer(portNum); 00619 if (pFIO != NULL) { 00620 if ((byteNum >= 0) && (byteNum <= 3)){ 00621 pFIO->FIOCLR[byteNum] = bitValue; 00622 } 00623 } 00624 } 00625 00626 00627 /*********************************************************************/ 00636 uint8_t FIO_ByteReadValue(uint8_t portNum, uint8_t byteNum) 00637 { 00638 GPIO_Byte_TypeDef *pFIO = FIO_ByteGetPointer(portNum); 00639 if (pFIO != NULL) { 00640 if ((byteNum >= 0) && (byteNum <= 3)){ 00641 return (pFIO->FIOPIN[byteNum]); 00642 } 00643 } 00644 return (0); 00645 } 00646 00651 #endif /* _GPIO */ 00652 00657 /* --------------------------------- End Of File ------------------------------ */
Generated on Mon Feb 8 10:01:37 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
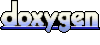