RTC_Public_Functions
[RTC]
Functions | |
void | RTC_Init (LPC_RTC_TypeDef *RTCx) |
Initializes the RTC peripheral. | |
void | RTC_DeInit (LPC_RTC_TypeDef *RTCx) |
De-initializes the RTC peripheral registers to their default reset values. | |
void | RTC_ResetClockTickCounter (LPC_RTC_TypeDef *RTCx) |
Reset clock tick counter in RTC peripheral. | |
void | RTC_Cmd (LPC_RTC_TypeDef *RTCx, FunctionalState NewState) |
Start/Stop RTC peripheral. | |
void | RTC_CntIncrIntConfig (LPC_RTC_TypeDef *RTCx, uint32_t CntIncrIntType, FunctionalState NewState) |
Enable/Disable Counter increment interrupt for each time type in RTC peripheral. | |
void | RTC_AlarmIntConfig (LPC_RTC_TypeDef *RTCx, uint32_t AlarmTimeType, FunctionalState NewState) |
Enable/Disable Alarm interrupt for each time type in RTC peripheral. | |
void | RTC_SetTime (LPC_RTC_TypeDef *RTCx, uint32_t Timetype, uint32_t TimeValue) |
Set current time value for each time type in RTC peripheral. | |
uint32_t | RTC_GetTime (LPC_RTC_TypeDef *RTCx, uint32_t Timetype) |
Get current time value for each type time type. | |
void | RTC_SetFullTime (LPC_RTC_TypeDef *RTCx, RTC_TIME_Type *pFullTime) |
Set full of time in RTC peripheral. | |
void | RTC_GetFullTime (LPC_RTC_TypeDef *RTCx, RTC_TIME_Type *pFullTime) |
Get full of time in RTC peripheral. | |
void | RTC_SetAlarmTime (LPC_RTC_TypeDef *RTCx, uint32_t Timetype, uint32_t ALValue) |
Set alarm time value for each time type. | |
uint32_t | RTC_GetAlarmTime (LPC_RTC_TypeDef *RTCx, uint32_t Timetype) |
Get alarm time value for each time type. | |
void | RTC_SetFullAlarmTime (LPC_RTC_TypeDef *RTCx, RTC_TIME_Type *pFullTime) |
Set full of alarm time in RTC peripheral. | |
void | RTC_GetFullAlarmTime (LPC_RTC_TypeDef *RTCx, RTC_TIME_Type *pFullTime) |
Get full of alarm time in RTC peripheral. | |
IntStatus | RTC_GetIntPending (LPC_RTC_TypeDef *RTCx, uint32_t IntType) |
Check whether if specified Location interrupt in RTC peripheral is set or not. | |
void | RTC_ClearIntPending (LPC_RTC_TypeDef *RTCx, uint32_t IntType) |
Clear specified Location interrupt pending in RTC peripheral. | |
void | RTC_CalibCounterCmd (LPC_RTC_TypeDef *RTCx, FunctionalState NewState) |
Enable/Disable calibration counter in RTC peripheral. | |
void | RTC_CalibConfig (LPC_RTC_TypeDef *RTCx, uint32_t CalibValue, uint8_t CalibDir) |
Configures Calibration in RTC peripheral. | |
void | RTC_WriteGPREG (LPC_RTC_TypeDef *RTCx, uint8_t Channel, uint32_t Value) |
Write value to General purpose registers. | |
uint32_t | RTC_ReadGPREG (LPC_RTC_TypeDef *RTCx, uint8_t Channel) |
Read value from General purpose registers. |
Function Documentation
void RTC_AlarmIntConfig | ( | LPC_RTC_TypeDef * | RTCx, | |
uint32_t | AlarmTimeType, | |||
FunctionalState | NewState | |||
) |
Enable/Disable Alarm interrupt for each time type in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] AlarmTimeType,: Alarm Time Interrupt type, an matching of this type value below with current time in RTC will generates an interrupt, should be: - RTC_TIMETYPE_SECOND
- RTC_TIMETYPE_MINUTE
- RTC_TIMETYPE_HOUR
- RTC_TIMETYPE_DAYOFWEEK
- RTC_TIMETYPE_DAYOFMONTH
- RTC_TIMETYPE_DAYOFYEAR
- RTC_TIMETYPE_MONTH
- RTC_TIMETYPE_YEAR
[in] NewState New State of this function, should be: - ENABLE: Alarm interrupt for this time type are enabled
- DISABLE: Alarm interrupt for this time type are disabled
- Returns:
- None
Definition at line 236 of file lpc17xx_rtc.c.
void RTC_CalibConfig | ( | LPC_RTC_TypeDef * | RTCx, | |
uint32_t | CalibValue, | |||
uint8_t | CalibDir | |||
) |
Configures Calibration in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] CalibValue Calibration value, should be in range from 0 to 131,072 [in] CalibDir Calibration Direction, should be: - RTC_CALIB_DIR_FORWARD: Forward calibration
- RTC_CALIB_DIR_BACKWARD: Backward calibration
- Returns:
- None
Definition at line 719 of file lpc17xx_rtc.c.
void RTC_CalibCounterCmd | ( | LPC_RTC_TypeDef * | RTCx, | |
FunctionalState | NewState | |||
) |
Enable/Disable calibration counter in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] NewState New State of this function, should be: - ENABLE: The calibration counter is enabled and counting
- DISABLE: The calibration counter is disabled and reset to zero
- Returns:
- None
Definition at line 693 of file lpc17xx_rtc.c.
void RTC_ClearIntPending | ( | LPC_RTC_TypeDef * | RTCx, | |
uint32_t | IntType | |||
) |
Clear specified Location interrupt pending in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] IntType Interrupt location type, should be: - RTC_INT_COUNTER_INCREASE: Clear Counter Increment Interrupt pending.
- RTC_INT_ALARM: Clear alarm interrupt pending
- Returns:
- None
Definition at line 677 of file lpc17xx_rtc.c.
void RTC_Cmd | ( | LPC_RTC_TypeDef * | RTCx, | |
FunctionalState | NewState | |||
) |
Start/Stop RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] NewState New State of this function, should be: - ENABLE: The time counters are enabled
- DISABLE: The time counters are disabled
- Returns:
- None
Definition at line 106 of file lpc17xx_rtc.c.
void RTC_CntIncrIntConfig | ( | LPC_RTC_TypeDef * | RTCx, | |
uint32_t | CntIncrIntType, | |||
FunctionalState | NewState | |||
) |
Enable/Disable Counter increment interrupt for each time type in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] CntIncrIntType,: Counter Increment Interrupt type, an increment of this type value below will generates an interrupt, should be: - RTC_TIMETYPE_SECOND
- RTC_TIMETYPE_MINUTE
- RTC_TIMETYPE_HOUR
- RTC_TIMETYPE_DAYOFWEEK
- RTC_TIMETYPE_DAYOFMONTH
- RTC_TIMETYPE_DAYOFYEAR
- RTC_TIMETYPE_MONTH
- RTC_TIMETYPE_YEAR
[in] NewState New State of this function, should be: - ENABLE: Counter Increment interrupt for this time type are enabled
- DISABLE: Counter Increment interrupt for this time type are disabled
- Returns:
- None
Definition at line 144 of file lpc17xx_rtc.c.
void RTC_DeInit | ( | LPC_RTC_TypeDef * | RTCx | ) |
De-initializes the RTC peripheral registers to their default reset values.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC
- Returns:
- None
Definition at line 76 of file lpc17xx_rtc.c.
uint32_t RTC_GetAlarmTime | ( | LPC_RTC_TypeDef * | RTCx, | |
uint32_t | Timetype | |||
) |
Get alarm time value for each time type.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] Timetype,: Time Type, should be: - RTC_TIMETYPE_SECOND
- RTC_TIMETYPE_MINUTE
- RTC_TIMETYPE_HOUR
- RTC_TIMETYPE_DAYOFWEEK
- RTC_TIMETYPE_DAYOFMONTH
- RTC_TIMETYPE_DAYOFYEAR
- RTC_TIMETYPE_MONTH
- RTC_TIMETYPE_YEAR
- Returns:
- Value of Alarm time according to specified time type
Definition at line 567 of file lpc17xx_rtc.c.
void RTC_GetFullAlarmTime | ( | LPC_RTC_TypeDef * | RTCx, | |
RTC_TIME_Type * | pFullTime | |||
) |
Get full of alarm time in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] pFullTime Pointer to a RTC_TIME_Type structure that will be stored alarm time in full.
- Returns:
- None
Definition at line 631 of file lpc17xx_rtc.c.
void RTC_GetFullTime | ( | LPC_RTC_TypeDef * | RTCx, | |
RTC_TIME_Type * | pFullTime | |||
) |
Get full of time in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] pFullTime Pointer to a RTC_TIME_Type structure that will be stored time in full.
- Returns:
- None
Definition at line 462 of file lpc17xx_rtc.c.
IntStatus RTC_GetIntPending | ( | LPC_RTC_TypeDef * | RTCx, | |
uint32_t | IntType | |||
) |
Check whether if specified Location interrupt in RTC peripheral is set or not.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] IntType Interrupt location type, should be: - RTC_INT_COUNTER_INCREASE: Counter Increment Interrupt block generated an interrupt.
- RTC_INT_ALARM: Alarm generated an interrupt.
- Returns:
- New state of specified Location interrupt in RTC peripheral (SET or RESET)
Definition at line 658 of file lpc17xx_rtc.c.
uint32_t RTC_GetTime | ( | LPC_RTC_TypeDef * | RTCx, | |
uint32_t | Timetype | |||
) |
Get current time value for each type time type.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] Timetype,: Time Type, should be: - RTC_TIMETYPE_SECOND
- RTC_TIMETYPE_MINUTE
- RTC_TIMETYPE_HOUR
- RTC_TIMETYPE_DAYOFWEEK
- RTC_TIMETYPE_DAYOFMONTH
- RTC_TIMETYPE_DAYOFYEAR
- RTC_TIMETYPE_MONTH
- RTC_TIMETYPE_YEAR
- Returns:
- Value of time according to specified time type
Definition at line 395 of file lpc17xx_rtc.c.
void RTC_Init | ( | LPC_RTC_TypeDef * | RTCx | ) |
Initializes the RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC
- Returns:
- None
Definition at line 54 of file lpc17xx_rtc.c.
uint32_t RTC_ReadGPREG | ( | LPC_RTC_TypeDef * | RTCx, | |
uint8_t | Channel | |||
) |
Read value from General purpose registers.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] Channel General purpose registers Channel number, should be in range from 0 to 4.
- Returns:
- Read Value Note: These General purpose registers can be used to store important information when the main power supply is off. The value in these registers is not affected by chip reset.
Definition at line 764 of file lpc17xx_rtc.c.
void RTC_ResetClockTickCounter | ( | LPC_RTC_TypeDef * | RTCx | ) |
Reset clock tick counter in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC
- Returns:
- None
Definition at line 90 of file lpc17xx_rtc.c.
void RTC_SetAlarmTime | ( | LPC_RTC_TypeDef * | RTCx, | |
uint32_t | Timetype, | |||
uint32_t | ALValue | |||
) |
Set alarm time value for each time type.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] Timetype,: Time Type, should be: - RTC_TIMETYPE_SECOND
- RTC_TIMETYPE_MINUTE
- RTC_TIMETYPE_HOUR
- RTC_TIMETYPE_DAYOFWEEK
- RTC_TIMETYPE_DAYOFMONTH
- RTC_TIMETYPE_DAYOFYEAR
- RTC_TIMETYPE_MONTH
- RTC_TIMETYPE_YEAR
[in] ALValue Alarm time value to set
- Returns:
- None
Definition at line 492 of file lpc17xx_rtc.c.
void RTC_SetFullAlarmTime | ( | LPC_RTC_TypeDef * | RTCx, | |
RTC_TIME_Type * | pFullTime | |||
) |
Set full of alarm time in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] pFullTime Pointer to a RTC_TIME_Type structure that contains alarm time value in full.
- Returns:
- None
Definition at line 609 of file lpc17xx_rtc.c.
void RTC_SetFullTime | ( | LPC_RTC_TypeDef * | RTCx, | |
RTC_TIME_Type * | pFullTime | |||
) |
Set full of time in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] pFullTime Pointer to a RTC_TIME_Type structure that contains time value in full.
- Returns:
- None
Definition at line 440 of file lpc17xx_rtc.c.
void RTC_SetTime | ( | LPC_RTC_TypeDef * | RTCx, | |
uint32_t | Timetype, | |||
uint32_t | TimeValue | |||
) |
Set current time value for each time type in RTC peripheral.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] Timetype,: Time Type, should be: - RTC_TIMETYPE_SECOND
- RTC_TIMETYPE_MINUTE
- RTC_TIMETYPE_HOUR
- RTC_TIMETYPE_DAYOFWEEK
- RTC_TIMETYPE_DAYOFMONTH
- RTC_TIMETYPE_DAYOFYEAR
- RTC_TIMETYPE_MONTH
- RTC_TIMETYPE_YEAR
[in] TimeValue Time value to set
- Returns:
- None
Definition at line 321 of file lpc17xx_rtc.c.
void RTC_WriteGPREG | ( | LPC_RTC_TypeDef * | RTCx, | |
uint8_t | Channel, | |||
uint32_t | Value | |||
) |
Write value to General purpose registers.
- Parameters:
-
[in] RTCx RTC peripheral selected, should be RTC [in] Channel General purpose registers Channel number, should be in range from 0 to 4. [in] Value Value to write
- Returns:
- None Note: These General purpose registers can be used to store important information when the main power supply is off. The value in these registers is not affected by chip reset.
Definition at line 741 of file lpc17xx_rtc.c.
Generated on Mon Feb 8 10:01:47 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
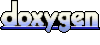