C:/nxpdrv/LPC1700CMSIS/Drivers/include/lpc17xx_rtc.h File Reference
: Contains all macro definitions and function prototypes support for RTC firmware library on LPC17xx More...
#include "LPC17xx.h"
#include "lpc_types.h"
Go to the source code of this file.
Data Structures | |
struct | RTC_TIME_Type |
Time structure definitions for easy manipulate the data. More... | |
Defines | |
#define | RTC_ILR_BITMASK ((0x00000003)) |
#define | RTC_IRL_RTCCIF ((1<<0)) |
#define | RTC_IRL_RTCALF ((1<<1)) |
#define | RTC_CCR_BITMASK ((0x00000013)) |
#define | RTC_CCR_CLKEN ((1<<0)) |
#define | RTC_CCR_CTCRST ((1<<1)) |
#define | RTC_CCR_CCALEN ((1<<4)) |
#define | RTC_CIIR_IMSEC ((1<<0)) |
#define | RTC_CIIR_IMMIN ((1<<1)) |
#define | RTC_CIIR_IMHOUR ((1<<2)) |
#define | RTC_CIIR_IMDOM ((1<<3)) |
#define | RTC_CIIR_IMDOW ((1<<4)) |
#define | RTC_CIIR_IMDOY ((1<<5)) |
#define | RTC_CIIR_IMMON ((1<<6)) |
#define | RTC_CIIR_IMYEAR ((1<<7)) |
#define | RTC_CIIR_BITMASK ((0xFF)) |
#define | RTC_AMR_AMRSEC ((1<<0)) |
#define | RTC_AMR_AMRMIN ((1<<1)) |
#define | RTC_AMR_AMRHOUR ((1<<2)) |
#define | RTC_AMR_AMRDOM ((1<<3)) |
#define | RTC_AMR_AMRDOW ((1<<4)) |
#define | RTC_AMR_AMRDOY ((1<<5)) |
#define | RTC_AMR_AMRMON ((1<<6)) |
#define | RTC_AMR_AMRYEAR ((1<<7)) |
#define | RTC_AMR_BITMASK ((0xFF)) |
#define | RTC_AUX_RTC_OSCF ((1<<4)) |
#define | RTC_AUXEN_RTC_OSCFEN ((1<<4)) |
#define | RTC_CTIME0_SECONDS_MASK ((0x3F)) |
#define | RTC_CTIME0_MINUTES_MASK ((0x3F00)) |
#define | RTC_CTIME0_HOURS_MASK ((0x1F0000)) |
#define | RTC_CTIME0_DOW_MASK ((0x7000000)) |
#define | RTC_CTIME1_DOM_MASK ((0x1F)) |
#define | RTC_CTIME1_MONTH_MASK ((0xF00)) |
#define | RTC_CTIME1_YEAR_MASK ((0xFFF0000)) |
#define | RTC_CTIME2_DOY_MASK ((0xFFF)) |
#define | RTC_SEC_MASK (0x0000003F) |
#define | RTC_MIN_MASK (0x0000003F) |
#define | RTC_HOUR_MASK (0x0000001F) |
#define | RTC_DOM_MASK (0x0000001F) |
#define | RTC_DOW_MASK (0x00000007) |
#define | RTC_DOY_MASK (0x000001FF) |
#define | RTC_MONTH_MASK (0x0000000F) |
#define | RTC_YEAR_MASK (0x00000FFF) |
#define | RTC_SECOND_MAX 59 |
#define | RTC_MINUTE_MAX 59 |
#define | RTC_HOUR_MAX 23 |
#define | RTC_MONTH_MIN 1 |
#define | RTC_MONTH_MAX 12 |
#define | RTC_DAYOFMONTH_MIN 1 |
#define | RTC_DAYOFMONTH_MAX 31 |
#define | RTC_DAYOFWEEK_MAX 6 |
#define | RTC_DAYOFYEAR_MIN 1 |
#define | RTC_DAYOFYEAR_MAX 366 |
#define | RTC_YEAR_MAX 4095 |
#define | RTC_CALIBRATION_CALVAL_MASK ((0x1FFFF)) |
#define | RTC_CALIBRATION_LIBDIR ((1<<17)) |
#define | RTC_CALIBRATION_MAX ((0x20000)) |
#define | PARAM_RTC_INT(n) ((n==RTC_INT_COUNTER_INCREASE) || (n==RTC_INT_ALARM)) |
#define | PARAM_RTC_TIMETYPE(n) |
#define | PARAM_RTCx(x) (((uint32_t *)x)==((uint32_t *)LPC_RTC)) |
#define | RTC_CALIB_DIR_FORWARD ((uint8_t)(0)) |
#define | RTC_CALIB_DIR_BACKWARD ((uint8_t)(1)) |
#define | PARAM_RTC_CALIB_DIR(n) ((n==RTC_CALIB_DIR_FORWARD) || (n==RTC_CALIB_DIR_BACKWARD)) |
#define | PARAM_RTC_GPREG_CH(n) ((n>=0) && (n<=4)) |
#define | PARAM_RTC_CALIBRATION_DIR(n) |
Enumerations | |
enum | RTC_INT_OPT { RTC_INT_COUNTER_INCREASE = RTC_IRL_RTCCIF, RTC_INT_ALARM = RTC_IRL_RTCALF } |
RTC interrupt source. More... | |
enum | RTC_TIMETYPE_Num { RTC_TIMETYPE_SECOND = 0, RTC_TIMETYPE_MINUTE = 1, RTC_TIMETYPE_HOUR = 2, RTC_TIMETYPE_DAYOFWEEK = 3, RTC_TIMETYPE_DAYOFMONTH = 4, RTC_TIMETYPE_DAYOFYEAR = 5, RTC_TIMETYPE_MONTH = 6, RTC_TIMETYPE_YEAR = 7 } |
RTC time type option. More... | |
Functions | |
void | RTC_Init (LPC_RTC_TypeDef *RTCx) |
Initializes the RTC peripheral. | |
void | RTC_DeInit (LPC_RTC_TypeDef *RTCx) |
De-initializes the RTC peripheral registers to their default reset values. | |
void | RTC_ResetClockTickCounter (LPC_RTC_TypeDef *RTCx) |
Reset clock tick counter in RTC peripheral. | |
void | RTC_Cmd (LPC_RTC_TypeDef *RTCx, FunctionalState NewState) |
Start/Stop RTC peripheral. | |
void | RTC_CntIncrIntConfig (LPC_RTC_TypeDef *RTCx, uint32_t CntIncrIntType, FunctionalState NewState) |
Enable/Disable Counter increment interrupt for each time type in RTC peripheral. | |
void | RTC_AlarmIntConfig (LPC_RTC_TypeDef *RTCx, uint32_t AlarmTimeType, FunctionalState NewState) |
Enable/Disable Alarm interrupt for each time type in RTC peripheral. | |
void | RTC_SetTime (LPC_RTC_TypeDef *RTCx, uint32_t Timetype, uint32_t TimeValue) |
Set current time value for each time type in RTC peripheral. | |
uint32_t | RTC_GetTime (LPC_RTC_TypeDef *RTCx, uint32_t Timetype) |
Get current time value for each type time type. | |
void | RTC_SetFullTime (LPC_RTC_TypeDef *RTCx, RTC_TIME_Type *pFullTime) |
Set full of time in RTC peripheral. | |
void | RTC_GetFullTime (LPC_RTC_TypeDef *RTCx, RTC_TIME_Type *pFullTime) |
Get full of time in RTC peripheral. | |
void | RTC_SetAlarmTime (LPC_RTC_TypeDef *RTCx, uint32_t Timetype, uint32_t ALValue) |
Set alarm time value for each time type. | |
uint32_t | RTC_GetAlarmTime (LPC_RTC_TypeDef *RTCx, uint32_t Timetype) |
Get alarm time value for each time type. | |
void | RTC_SetFullAlarmTime (LPC_RTC_TypeDef *RTCx, RTC_TIME_Type *pFullTime) |
Set full of alarm time in RTC peripheral. | |
void | RTC_GetFullAlarmTime (LPC_RTC_TypeDef *RTCx, RTC_TIME_Type *pFullTime) |
Get full of alarm time in RTC peripheral. | |
IntStatus | RTC_GetIntPending (LPC_RTC_TypeDef *RTCx, uint32_t IntType) |
Check whether if specified Location interrupt in RTC peripheral is set or not. | |
void | RTC_ClearIntPending (LPC_RTC_TypeDef *RTCx, uint32_t IntType) |
Clear specified Location interrupt pending in RTC peripheral. | |
void | RTC_CalibCounterCmd (LPC_RTC_TypeDef *RTCx, FunctionalState NewState) |
Enable/Disable calibration counter in RTC peripheral. | |
void | RTC_CalibConfig (LPC_RTC_TypeDef *RTCx, uint32_t CalibValue, uint8_t CalibDir) |
Configures Calibration in RTC peripheral. | |
void | RTC_WriteGPREG (LPC_RTC_TypeDef *RTCx, uint8_t Channel, uint32_t Value) |
Write value to General purpose registers. | |
uint32_t | RTC_ReadGPREG (LPC_RTC_TypeDef *RTCx, uint8_t Channel) |
Read value from General purpose registers. |
Detailed Description
: Contains all macro definitions and function prototypes support for RTC firmware library on LPC17xx
- Version:
- : 1.0
- Date:
- : 23. Apr. 2009
- Author:
- : HieuNguyen
Definition in file lpc17xx_rtc.h.
Generated on Mon Feb 8 10:01:40 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
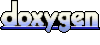