C:/nxpdrv/LPC1700CMSIS/Drivers/include/lpc17xx_gpio.h
Go to the documentation of this file.00001 /***********************************************************************/ 00021 /* Peripheral group ----------------------------------------------------------- */ 00027 #ifndef LPC17XX_GPIO_H_ 00028 #define LPC17XX_GPIO_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "LPC17xx.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 00041 /* Public Types --------------------------------------------------------------- */ 00049 typedef struct { 00050 __IO uint8_t FIODIR[4]; 00051 uint32_t RESERVED0[3]; 00052 __IO uint8_t FIOMASK[4]; 00053 __IO uint8_t FIOPIN[4]; 00054 __IO uint8_t FIOSET[4]; 00055 __O uint8_t FIOCLR[4]; 00056 } GPIO_Byte_TypeDef; 00057 00058 00062 typedef struct { 00063 __IO uint16_t FIODIRL; 00064 __IO uint16_t FIODIRU; 00065 uint32_t RESERVED0[3]; 00066 __IO uint16_t FIOMASKL; 00067 __IO uint16_t FIOMASKU; 00068 __IO uint16_t FIOPINL; 00069 __IO uint16_t FIOPINU; 00070 __IO uint16_t FIOSETL; 00071 __IO uint16_t FIOSETU; 00072 __O uint16_t FIOCLRL; 00073 __O uint16_t FIOCLRU; 00074 } GPIO_HalfWord_TypeDef; 00075 00076 00082 /* Public Macros -------------------------------------------------------------- */ 00088 #define GPIO0_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO0_BASE)) 00089 00090 #define GPIO1_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO1_BASE)) 00091 00092 #define GPIO2_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO2_BASE)) 00093 00094 #define GPIO3_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO3_BASE)) 00095 00096 #define GPIO4_Byte ((GPIO_Byte_TypeDef *)(LPC_GPIO4_BASE)) 00097 00098 00099 00101 #define GPIO0_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO0_BASE)) 00102 00103 #define GPIO1_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO1_BASE)) 00104 00105 #define GPIO2_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO2_BASE)) 00106 00107 #define GPIO3_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO3_BASE)) 00108 00109 #define GPIO4_HalfWord ((GPIO_HalfWord_TypeDef *)(LPC_GPIO4_BASE)) 00110 00111 00117 /* Public Functions ----------------------------------------------------------- */ 00122 /* GPIO style ------------------------------- */ 00123 void GPIO_SetDir(uint8_t portNum, uint32_t bitValue, uint8_t dir); 00124 void GPIO_SetValue(uint8_t portNum, uint32_t bitValue); 00125 void GPIO_ClearValue(uint8_t portNum, uint32_t bitValue); 00126 uint32_t GPIO_ReadValue(uint8_t portNum); 00127 00128 /* FIO (word-accessible) style ------------------------------- */ 00129 void FIO_SetDir(uint8_t portNum, uint32_t bitValue, uint8_t dir); 00130 void FIO_SetValue(uint8_t portNum, uint32_t bitValue); 00131 void FIO_ClearValue(uint8_t portNum, uint32_t bitValue); 00132 uint32_t FIO_ReadValue(uint8_t portNum); 00133 void FIO_SetMask(uint8_t portNum, uint32_t bitValue, uint8_t maskValue); 00134 00135 /* FIO (halfword-accessible) style ------------------------------- */ 00136 void FIO_HalfWordSetDir(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue, uint8_t dir); 00137 void FIO_HalfWordSetMask(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue, uint8_t maskValue); 00138 void FIO_HalfWordSetValue(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue); 00139 void FIO_HalfWordClearValue(uint8_t portNum, uint8_t halfwordNum, uint16_t bitValue); 00140 uint16_t FIO_HalfWordReadValue(uint8_t portNum, uint8_t halfwordNum); 00141 00142 /* FIO (byte-accessible) style ------------------------------- */ 00143 void FIO_ByteSetDir(uint8_t portNum, uint8_t byteNum, uint8_t bitValue, uint8_t dir); 00144 void FIO_ByteSetMask(uint8_t portNum, uint8_t byteNum, uint8_t bitValue, uint8_t maskValue); 00145 void FIO_ByteSetValue(uint8_t portNum, uint8_t byteNum, uint8_t bitValue); 00146 void FIO_ByteClearValue(uint8_t portNum, uint8_t byteNum, uint8_t bitValue); 00147 uint8_t FIO_ByteReadValue(uint8_t portNum, uint8_t byteNum); 00148 00149 00150 00156 #ifdef __cplusplus 00157 } 00158 #endif 00159 00160 #endif /* LPC17XX_GPIO_H_ */ 00161 00166 /* --------------------------------- End Of File ------------------------------ */
Generated on Mon Feb 8 10:01:36 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
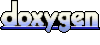