C:/nxpdrv/LPC1700CMSIS/Drivers/include/lpc17xx_spi.h File Reference
: Contains all macro definitions and function prototypes support for SPI firmware library on LPC17xx More...
#include "LPC17xx.h"
#include "lpc_types.h"
Go to the source code of this file.
Data Structures | |
struct | SPI_CFG_Type |
SPI configuration structure. More... | |
struct | SPI_DATA_SETUP_Type |
SPI Data configuration structure definitions. More... | |
Defines | |
#define | SPI_SPCR_BIT_EN ((uint32_t)(1<<2)) |
#define | SPI_SPCR_CPHA_SECOND ((uint32_t)(1<<3)) |
#define | SPI_SPCR_CPOL_LOW ((uint32_t)(1<<4)) |
#define | SPI_SPCR_MSTR ((uint32_t)(1<<5)) |
#define | SPI_SPCR_LSBF ((uint32_t)(1<<6)) |
#define | SPI_SPCR_SPIE ((uint32_t)(1<<7)) |
#define | SPI_SPCR_BITS(n) ((n==0) ? ((uint32_t)0) : ((uint32_t)((n&0x0F)<<8))) |
#define | SPI_SPCR_BITMASK ((uint32_t)(0xFFC)) |
#define | SPI_SPSR_ABRT ((uint32_t)(1<<3)) |
#define | SPI_SPSR_MODF ((uint32_t)(1<<4)) |
#define | SPI_SPSR_ROVR ((uint32_t)(1<<5)) |
#define | SPI_SPSR_WCOL ((uint32_t)(1<<6)) |
#define | SPI_SPSR_SPIF ((uint32_t)(1<<7)) |
#define | SPI_SPSR_BITMASK ((uint32_t)(0xF8)) |
#define | SPI_SPDR_LO_MASK ((uint32_t)(0xFF)) |
#define | SPI_SPDR_HI_MASK ((uint32_t)(0xFF00)) |
#define | SPI_SPDR_BITMASK ((uint32_t)(0xFFFF)) |
#define | SPI_SPCCR_COUNTER(n) ((uint32_t)(n&0xFF)) |
#define | SPI_SPCCR_BITMASK ((uint32_t)(0xFF)) |
#define | SPI_SPTCR_TEST_MASK ((uint32_t)(0xFE)) |
#define | SPI_SPTCR_BITMASK ((uint32_t)(0xFE)) |
#define | SPI_SPTSR_ABRT ((uint32_t)(1<<3)) |
#define | SPI_SPTSR_MODF ((uint32_t)(1<<4)) |
#define | SPI_SPTSR_ROVR ((uint32_t)(1<<5)) |
#define | SPI_SPTSR_WCOL ((uint32_t)(1<<6)) |
#define | SPI_SPTSR_SPIF ((uint32_t)(1<<7)) |
#define | SPI_SPTSR_MASKBIT ((uint32_t)(0xF8)) |
#define | SPI_SPINT_INTFLAG ((uint32_t)(1<<0)) |
#define | SPI_SPINT_BITMASK ((uint32_t)(0x01)) |
#define | PARAM_SPIx(n) (((uint32_t *)n)==((uint32_t *)LPC_SPI)) |
#define | SPI_CPHA_FIRST ((uint32_t)(0)) |
#define | SPI_CPHA_SECOND SPI_SPCR_CPHA_SECOND |
#define | PARAM_SPI_CPHA(n) ((n==SPI_CPHA_FIRST) || (n==SPI_CPHA_SECOND)) |
#define | SPI_CPOL_HI ((uint32_t)(0)) |
#define | SPI_CPOL_LO SPI_SPCR_CPOL_LOW |
#define | PARAM_SPI_CPOL(n) ((n==SPI_CPOL_HI) || (n==SPI_CPOL_LO)) |
#define | SPI_SLAVE_MODE ((uint32_t)(0)) |
#define | SPI_MASTER_MODE SPI_SPCR_MSTR |
#define | PARAM_SPI_MODE(n) ((n==SPI_SLAVE_MODE) || (n==SPI_MASTER_MODE)) |
#define | SPI_DATA_MSB_FIRST ((uint32_t)(0)) |
#define | SPI_DATA_LSB_FIRST SPI_SPCR_LSBF |
#define | PARAM_SPI_DATA_ORDER(n) ((n==SPI_DATA_MSB_FIRST) || (n==SPI_DATA_LSB_FIRST)) |
#define | SPI_DATABIT_16 SPI_SPCR_BITS(0) |
#define | SPI_DATABIT_8 SPI_SPCR_BITS(0x08) |
#define | SPI_DATABIT_9 SPI_SPCR_BITS(0x09) |
#define | SPI_DATABIT_10 SPI_SPCR_BITS(0x0A) |
#define | SPI_DATABIT_11 SPI_SPCR_BITS(0x0B) |
#define | SPI_DATABIT_12 SPI_SPCR_BITS(0x0C) |
#define | SPI_DATABIT_13 SPI_SPCR_BITS(0x0D) |
#define | SPI_DATABIT_14 SPI_SPCR_BITS(0x0E) |
#define | SPI_DATABIT_15 SPI_SPCR_BITS(0x0F) |
#define | PARAM_SPI_DATABIT(n) |
#define | SPI_STAT_ABRT SPI_SPSR_ABRT |
#define | SPI_STAT_MODF SPI_SPSR_MODF |
#define | SPI_STAT_ROVR SPI_SPSR_ROVR |
#define | SPI_STAT_WCOL SPI_SPSR_WCOL |
#define | SPI_STAT_SPIF SPI_SPSR_SPIF |
#define | PARAM_SPI_STAT(n) |
#define | SPI_STAT_DONE (1UL<<8) |
#define | SPI_STAT_ERROR (1UL<<9) |
Enumerations | |
enum | SPI_TRANSFER_Type { SPI_TRANSFER_POLLING = 0, SPI_TRANSFER_INTERRUPT } |
SPI Transfer Type definitions. More... | |
Functions | |
void | SPI_SetClock (LPC_SPI_TypeDef *SPIx, uint32_t target_clock) |
Setup clock rate for SPI device. | |
void | SPI_DeInit (LPC_SPI_TypeDef *SPIx) |
De-initializes the SPIx peripheral registers to their default reset values. | |
void | SPI_Init (LPC_SPI_TypeDef *SPIx, SPI_CFG_Type *SPI_ConfigStruct) |
Initializes the SPIx peripheral according to the specified parameters in the UART_ConfigStruct. | |
void | SPI_ConfigStructInit (SPI_CFG_Type *SPI_InitStruct) |
Fills each SPI_InitStruct member with its default value:
| |
void | SPI_SendData (LPC_SPI_TypeDef *SPIx, uint16_t Data) |
Transmit a single data through SPIx peripheral. | |
uint16_t | SPI_ReceiveData (LPC_SPI_TypeDef *SPIx) |
Receive a single data from SPIx peripheral. | |
int32_t | SPI_ReadWrite (LPC_SPI_TypeDef *SPIx, SPI_DATA_SETUP_Type *dataCfg, SPI_TRANSFER_Type xfType) |
SPI Read write data function. | |
void | SPI_IntCmd (LPC_SPI_TypeDef *SPIx, FunctionalState NewState) |
Enable or disable SPIx interrupt. | |
IntStatus | SPI_GetIntStatus (LPC_SPI_TypeDef *SPIx) |
Checks whether the SPI interrupt flag is set or not. | |
void | SPI_ClearIntPending (LPC_SPI_TypeDef *SPIx) |
Clear SPI interrupt flag. | |
uint32_t | SPI_GetStatus (LPC_SPI_TypeDef *SPIx) |
Get current value of SPI Status register in SPIx peripheral. | |
FlagStatus | SPI_CheckStatus (uint32_t inputSPIStatus, uint8_t SPIStatus) |
Checks whether the specified SPI Status flag is set or not via inputSPIStatus parameter. | |
void | SPI_StdIntHandler (void) |
Standard SPI Interrupt handler. |
Detailed Description
: Contains all macro definitions and function prototypes support for SPI firmware library on LPC17xx
- Version:
- : 1.0
- Date:
- : 3. April. 2009
- Author:
- : HieuNguyen
Definition in file lpc17xx_spi.h.
Generated on Mon Feb 8 10:01:40 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
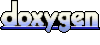