C:/nxpdrv/LPC1700CMSIS/Drivers/include/lpc17xx_timer.h File Reference
: Contains all functions support for Timer firmware library on LPC17xx More...
#include "LPC17xx.h"
#include "lpc_types.h"
Go to the source code of this file.
Data Structures | |
struct | TIM_TIMERCFG_Type |
Configuration structure in TIMER mode. More... | |
struct | TIM_COUNTERCFG_Type |
Configuration structure in COUNTER mode. More... | |
struct | TIM_MATCHCFG_Type |
Match channel configuration structure. More... | |
struct | TIM_CAPTURECFG_Type |
Capture Input configuration structure. More... | |
Defines | |
#define | TIM_IR_CLR(n) _BIT(n) |
#define | TIM_MATCH_INT(n) (_BIT(n & 0x0F)) |
#define | TIM_CAP_INT(n) (_BIT(((n & 0x0F) + 4))) |
#define | TIM_ENABLE ((uint32_t)(1<<0)) |
#define | TIM_RESET ((uint32_t)(1<<1)) |
#define | TIM_TCR_MASKBIT ((uint32_t)(3)) |
#define | TIM_INT_ON_MATCH(n) (_BIT((n * 3))) |
#define | TIM_RESET_ON_MATCH(n) (_BIT(((n * 3) + 1))) |
#define | TIM_STOP_ON_MATCH(n) (_BIT(((n * 3) + 2))) |
#define | TIM_MCR_MASKBIT ((uint32_t)(0x0FFF)) |
#define | TIM_MCR_CHANNEL_MASKBIT(n) ((uint32_t)(7<<n)) |
#define | TIM_CAP_RISING(n) (_BIT((n * 3))) |
#define | TIM_CAP_FALLING(n) (_BIT(((n * 3) + 1))) |
#define | TIM_INT_ON_CAP(n) (_BIT(((n * 3) + 2))) |
#define | TIM_EDGE_MASK(n) (_SBF((n * 3), 0x03)) |
#define | TIM_CCR_MASKBIT ((uint32_t)(0x3F)) |
#define | TIM_CCR_CHANNEL_MASKBIT(n) ((uint32_t)(7<<n)) |
#define | TIM_EM(n) _BIT(n) |
#define | TIM_EM_NOTHING ((uint8_t)(0x0)) |
#define | TIM_EM_LOW ((uint8_t)(0x1)) |
#define | TIM_EM_HIGH ((uint8_t)(0x2)) |
#define | TIM_EM_TOGGLE ((uint8_t)(0x3)) |
#define | TIM_EM_SET(n, s) (_SBF(((n << 1) + 4), (s & 0x03))) |
#define | TIM_EM_MASK(n) (_SBF(((n << 1) + 4), 0x03)) |
#define | TIM_EMR_MASKBIT 0x0FFF |
#define | TIM_CTCR_MODE_MASK 0x3 |
#define | TIM_CTCR_INPUT_MASK 0xC |
#define | TIM_CTCR_MASKBIT 0xF |
#define | TIM_COUNTER_MODE ((uint8_t)(1)) |
#define | PARAM_TIM_INT_TYPE(TYPE) |
#define | PARAM_TIM_MODE_OPT(MODE) |
#define | PARAM_TIM_PRESCALE_OPT(OPT) ((OPT == TIM_PRESCALE_TICKVAL)||(OPT == TIM_PRESCALE_USVAL)) |
#define | PARAM_TIM_COUNTER_INPUT_OPT(OPT) ((OPT == TIM_COUNTER_INCAP0)||(OPT == TIM_COUNTER_INCAP1)) |
#define | PARAM_TIM_EXTMATCH_OPT(OPT) |
#define | PARAM_TIM_CAP_MODE_OPT(OPT) |
#define | PARAM_TIMx(n) |
Enumerations | |
enum | TIM_INT_TYPE { TIM_MR0_INT = 0, TIM_MR1_INT = 1, TIM_MR2_INT = 2, TIM_MR3_INT = 3, TIM_CR0_INT = 4, TIM_CR1_INT = 5 } |
interrupt type More... | |
enum | TIM_MODE_OPT { TIM_TIMER_MODE = 0, TIM_COUNTER_RISING_MODE, TIM_COUNTER_FALLING_MODE, TIM_COUNTER_ANY_MODE } |
Timer/counter operating mode. More... | |
enum | TIM_PRESCALE_OPT { TIM_PRESCALE_TICKVAL = 0, TIM_PRESCALE_USVAL } |
Timer/Counter prescale option. More... | |
enum | TIM_COUNTER_INPUT_OPT { TIM_COUNTER_INCAP0 = 0, TIM_COUNTER_INCAP1 } |
Counter input option. More... | |
enum | TIM_EXTMATCH_OPT { TIM_EXTMATCH_NOTHING = 0, TIM_EXTMATCH_LOW, TIM_EXTMATCH_HIGH, TIM_EXTMATCH_TOGGLE } |
Timer/Counter external match option. More... | |
enum | TIM_CAP_MODE_OPT { TIM_CAPTURE_NONE = 0, TIM_CAPTURE_RISING, TIM_CAPTURE_FALLING, TIM_CAPTURE_ANY } |
Timer/counter capture mode options. More... | |
Functions | |
FlagStatus | TIM_GetIntStatus (LPC_TIM_TypeDef *TIMx, uint8_t IntFlag) |
Get Interrupt Status. | |
FlagStatus | TIM_GetIntCaptureStatus (LPC_TIM_TypeDef *TIMx, uint8_t IntFlag) |
Get Capture Interrupt Status. | |
void | TIM_ClearIntPending (LPC_TIM_TypeDef *TIMx, uint8_t IntFlag) |
Clear Interrupt pending. | |
void | TIM_ClearIntCapturePending (LPC_TIM_TypeDef *TIMx, uint8_t IntFlag) |
Clear Capture Interrupt pending. | |
void | TIM_Cmd (LPC_TIM_TypeDef *TIMx, FunctionalState NewState) |
Start/Stop Timer/Counter device. | |
void | TIM_ResetCounter (LPC_TIM_TypeDef *TIMx) |
Reset Timer/Counter device, Make TC and PC are synchronously reset on the next positive edge of PCLK. | |
void | TIM_Init (LPC_TIM_TypeDef *TIMx, uint8_t TimerCounterMode, void *TIM_ConfigStruct) |
Initial Timer/Counter device Set Clock frequency for Timer Set initial configuration for Timer. | |
void | TIM_DeInit (LPC_TIM_TypeDef *TIMx) |
Close Timer/Counter device. | |
void | TIM_ConfigStructInit (uint8_t TimerCounterMode, void *TIM_ConfigStruct) |
Configuration for Timer at initial time. | |
void | TIM_ConfigMatch (LPC_TIM_TypeDef *TIMx, TIM_MATCHCFG_Type *TIM_MatchConfigStruct) |
Configuration for Match register. | |
void | TIM_SetMatchExt (LPC_TIM_TypeDef *TIMx, TIM_EXTMATCH_OPT ext_match) |
void | TIM_ConfigCapture (LPC_TIM_TypeDef *TIMx, TIM_CAPTURECFG_Type *TIM_CaptureConfigStruct) |
Configuration for Capture register. | |
uint32_t | TIM_GetCaptureValue (LPC_TIM_TypeDef *TIMx, uint8_t CaptureChannel) |
Read value of capture register in timer/counter device. |
Detailed Description
: Contains all functions support for Timer firmware library on LPC17xx
- Version:
- : 1.0
- Date:
- : 14. April. 2009
- Author:
- : HieuNguyen
Definition in file lpc17xx_timer.h.
Generated on Mon Feb 8 10:01:41 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
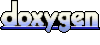