UART_Public_Functions
[UART]
Functions | |
void | UART_DeInit (LPC_UART_TypeDef *UARTx) |
De-initializes the UARTx peripheral registers to their default reset values. | |
void | UART_Init (LPC_UART_TypeDef *UARTx, UART_CFG_Type *UART_ConfigStruct) |
Initializes the UARTx peripheral according to the specified parameters in the UART_ConfigStruct. | |
void | UART_ConfigStructInit (UART_CFG_Type *UART_InitStruct) |
Fills each UART_InitStruct member with its default value: 9600 bps 8-bit data 1 Stopbit None Parity. | |
void | UART_SendData (LPC_UART_TypeDef *UARTx, uint8_t Data) |
Transmit a single data through UART peripheral. | |
uint8_t | UART_ReceiveData (LPC_UART_TypeDef *UARTx) |
Receive a single data from UART peripheral. | |
void | UART_ForceBreak (LPC_UART_TypeDef *UARTx) |
Force BREAK character on UART line, output pin UARTx TXD is forced to logic 0. | |
void | UART_IrDAInvtInputCmd (LPC_UART_TypeDef *UARTx, FunctionalState NewState) |
Enable or disable inverting serial input function of IrDA on UART peripheral. | |
void | UART_IrDACmd (LPC_UART_TypeDef *UARTx, FunctionalState NewState) |
Enable or disable IrDA function on UART peripheral. | |
void | UART_IrDAPulseDivConfig (LPC_UART_TypeDef *UARTx, UART_IrDA_PULSE_Type PulseDiv) |
Configure Pulse divider for IrDA function on UART peripheral. | |
void | UART_IntConfig (LPC_UART_TypeDef *UARTx, UART_INT_Type UARTIntCfg, FunctionalState NewState) |
Enable or disable specified UART interrupt. | |
uint8_t | UART_GetLineStatus (LPC_UART_TypeDef *UARTx) |
Get current value of Line Status register in UART peripheral. | |
FlagStatus | UART_CheckBusy (LPC_UART_TypeDef *UARTx) |
Check whether if UART is busy or not. | |
void | UART_FIFOConfig (LPC_UART_TypeDef *UARTx, UART_FIFO_CFG_Type *FIFOCfg) |
Configure FIFO function on selected UART peripheral. | |
void | UART_FIFOConfigStructInit (UART_FIFO_CFG_Type *UART_FIFOInitStruct) |
Fills each UART_FIFOInitStruct member with its default value:
| |
void | UART_ABCmd (LPC_UART_TypeDef *UARTx, UART_AB_CFG_Type *ABConfigStruct, FunctionalState NewState) |
Start/Stop Auto Baudrate activity. | |
void | UART_TxCmd (LPC_UART_TypeDef *UARTx, FunctionalState NewState) |
Enable/Disable transmission on UART TxD pin. | |
void | UART_FullModemForcePinState (LPC_UART1_TypeDef *UARTx, UART_MODEM_PIN_Type Pin, UART1_SignalState NewState) |
Force pin DTR/RTS corresponding to given state (Full modem mode). | |
void | UART_FullModemConfigMode (LPC_UART1_TypeDef *UARTx, UART_MODEM_MODE_Type Mode, FunctionalState NewState) |
Configure Full Modem mode for UART peripheral. | |
uint8_t | UART_FullModemGetStatus (LPC_UART1_TypeDef *UARTx) |
Get current status of modem status register. | |
void | UART_RS485Config (LPC_UART1_TypeDef *UARTx, UART1_RS485_CTRLCFG_Type *RS485ConfigStruct) |
Configure UART peripheral in RS485 mode according to the specified parameters in the RS485ConfigStruct. | |
void | UART_RS485ReceiverCmd (LPC_UART1_TypeDef *UARTx, FunctionalState NewState) |
Enable/Disable receiver in RS485 module in UART1. | |
void | UART_RS485SendSlvAddr (LPC_UART1_TypeDef *UARTx, uint8_t SlvAddr) |
Send Slave address frames on RS485 bus. | |
uint32_t | UART_RS485SendData (LPC_UART1_TypeDef *UARTx, uint8_t *pData, uint32_t size) |
Send Data frames on RS485 bus. | |
uint32_t | UART_Send (LPC_UART_TypeDef *UARTx, uint8_t *txbuf, uint32_t buflen, TRANSFER_BLOCK_Type flag) |
Send a block of data via UART peripheral. | |
uint32_t | UART_Receive (LPC_UART_TypeDef *UARTx, uint8_t *rxbuf, uint32_t buflen, TRANSFER_BLOCK_Type flag) |
Receive a block of data via UART peripheral. | |
void | UART_SetupCbs (LPC_UART_TypeDef *UARTx, uint8_t CbType, void *pfnCbs) |
Setup call-back function for UART interrupt handler for each UART peripheral. | |
void | UART0_StdIntHandler (void) |
Standard UART0 interrupt handler. | |
void | UART1_StdIntHandler (void) |
Standard UART1 interrupt handler. | |
void | UART2_StdIntHandler (void) |
Standard UART2 interrupt handler. | |
void | UART3_StdIntHandler (void) |
Standard UART3 interrupt handler. | |
uint32_t | UART_RS485Send (LPC_UART1_TypeDef *UARTx, uint8_t *pDatFrm, uint32_t size, uint8_t ParityStick) |
Send data on RS485 bus with specified parity stick value (9-bit mode). |
Function Documentation
void UART0_StdIntHandler | ( | void | ) |
Standard UART0 interrupt handler.
- Parameters:
-
[in] None
- Returns:
- None
Definition at line 1491 of file lpc17xx_uart.c.
void UART1_StdIntHandler | ( | void | ) |
Standard UART1 interrupt handler.
- Parameters:
-
[in] None
- Returns:
- None
Definition at line 1501 of file lpc17xx_uart.c.
void UART2_StdIntHandler | ( | void | ) |
Standard UART2 interrupt handler.
- Parameters:
-
[in] None
- Returns:
- None
Definition at line 1511 of file lpc17xx_uart.c.
void UART3_StdIntHandler | ( | void | ) |
Standard UART3 interrupt handler.
- Parameters:
-
[in] None
- Returns:
Definition at line 1521 of file lpc17xx_uart.c.
void UART_ABCmd | ( | LPC_UART_TypeDef * | UARTx, | |
UART_AB_CFG_Type * | ABConfigStruct, | |||
FunctionalState | NewState | |||
) |
Start/Stop Auto Baudrate activity.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3. [in] ABConfigStruct A pointer to UART_AB_CFG_Type structure that contains specified information about UART auto baudrate configuration [in] NewState New State of Auto baudrate activity, should be: - ENABLE: Start this activity
- DISABLE: Stop this activity Note: Auto-baudrate mode enable bit will be cleared once this mode completed.
- Returns:
- none
Definition at line 980 of file lpc17xx_uart.c.
FlagStatus UART_CheckBusy | ( | LPC_UART_TypeDef * | UARTx | ) |
Check whether if UART is busy or not.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3.
- Returns:
- RESET if UART is not busy, otherwise return SET.
Definition at line 873 of file lpc17xx_uart.c.
void UART_ConfigStructInit | ( | UART_CFG_Type * | UART_InitStruct | ) |
Fills each UART_InitStruct member with its default value: 9600 bps 8-bit data 1 Stopbit None Parity.
- Parameters:
-
[in] UART_InitStruct Pointer to a UART_CFG_Type structure which will be initialized.
- Returns:
- None
Definition at line 599 of file lpc17xx_uart.c.
void UART_DeInit | ( | LPC_UART_TypeDef * | UARTx | ) |
De-initializes the UARTx peripheral registers to their default reset values.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3.
- Returns:
- None
Definition at line 342 of file lpc17xx_uart.c.
void UART_FIFOConfig | ( | LPC_UART_TypeDef * | UARTx, | |
UART_FIFO_CFG_Type * | FIFOCfg | |||
) |
Configure FIFO function on selected UART peripheral.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3. [in] FIFOCfg Pointer to a UART_FIFO_CFG_Type Structure that contains specified information about FIFO configuration
- Returns:
- none
Definition at line 891 of file lpc17xx_uart.c.
void UART_FIFOConfigStructInit | ( | UART_FIFO_CFG_Type * | UART_FIFOInitStruct | ) |
Fills each UART_FIFOInitStruct member with its default value:
- FIFO_DMAMode = DISABLE
- FIFO_Level = UART_FIFO_TRGLEV0
- FIFO_ResetRxBuf = ENABLE
- FIFO_ResetTxBuf = ENABLE
- FIFO_State = ENABLE.
- Parameters:
-
[in] UART_FIFOInitStruct Pointer to a UART_FIFO_CFG_Type structure which will be initialized.
- Returns:
- None
Definition at line 957 of file lpc17xx_uart.c.
void UART_ForceBreak | ( | LPC_UART_TypeDef * | UARTx | ) |
Force BREAK character on UART line, output pin UARTx TXD is forced to logic 0.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3.
- Returns:
- none
Definition at line 659 of file lpc17xx_uart.c.
void UART_FullModemConfigMode | ( | LPC_UART1_TypeDef * | UARTx, | |
UART_MODEM_MODE_Type | Mode, | |||
FunctionalState | NewState | |||
) |
Configure Full Modem mode for UART peripheral.
- Parameters:
-
[in] UARTx UART1 (only) [in] Mode Full Modem mode, should be: - UART1_MODEM_MODE_LOOPBACK: Loop back mode.
- UART1_MODEM_MODE_AUTO_RTS: Auto-RTS mode.
- UART1_MODEM_MODE_AUTO_CTS: Auto-CTS mode.
[in] NewState New State of this mode, should be: - ENABLE: Enable this mode.
- DISABLE: Disable this mode.
- Returns:
- none
Definition at line 1128 of file lpc17xx_uart.c.
void UART_FullModemForcePinState | ( | LPC_UART1_TypeDef * | UARTx, | |
UART_MODEM_PIN_Type | Pin, | |||
UART1_SignalState | NewState | |||
) |
Force pin DTR/RTS corresponding to given state (Full modem mode).
- Parameters:
-
[in] UARTx UART1 (only) [in] Pin Pin that NewState will be applied to, should be: - UART1_MODEM_PIN_DTR: DTR pin.
- UART1_MODEM_PIN_RTS: RTS pin.
[in] NewState New State of DTR/RTS pin, should be: - INACTIVE: Force the pin to inactive signal.
- ACTIVE: Force the pin to active signal.
- Returns:
- none
Definition at line 1088 of file lpc17xx_uart.c.
uint8_t UART_FullModemGetStatus | ( | LPC_UART1_TypeDef * | UARTx | ) |
Get current status of modem status register.
- Parameters:
-
[in] UARTx UART1 (only)
- Returns:
- Current value of modem status register Note: The return value of this function must be ANDed with each member UART_MODEM_STAT_type enumeration to determine current flag status corresponding to each modem flag status. Because some flags in modem status register will be cleared after reading, the next reading modem register could not be correct. So this function used to read modem status register in one time only, then the return value used to check all flags.
Definition at line 1174 of file lpc17xx_uart.c.
uint8_t UART_GetLineStatus | ( | LPC_UART_TypeDef * | UARTx | ) |
Get current value of Line Status register in UART peripheral.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3.
- Returns:
- Current value of Line Status register in UART peripheral. Note: The return value of this function must be ANDed with each member in UART_LS_Type enumeration to determine current flag status corresponding to each Line status type. Because some flags in Line Status register will be cleared after reading, the next reading Line Status register could not be correct. So this function used to read Line status register in one time only, then the return value used to check all flags.
Definition at line 853 of file lpc17xx_uart.c.
void UART_Init | ( | LPC_UART_TypeDef * | UARTx, | |
UART_CFG_Type * | UART_ConfigStruct | |||
) |
Initializes the UARTx peripheral according to the specified parameters in the UART_ConfigStruct.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3. [in] UART_ConfigStruct Pointer to a UART_CFG_Type structure that contains the configuration information for the specified UART peripheral.
- Returns:
- None
Definition at line 392 of file lpc17xx_uart.c.
void UART_IntConfig | ( | LPC_UART_TypeDef * | UARTx, | |
UART_INT_Type | UARTIntCfg, | |||
FunctionalState | NewState | |||
) |
Enable or disable specified UART interrupt.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3. [in] UARTIntCfg Specifies the interrupt flag, should be one of the following: - UART_INTCFG_RBR : RBR Interrupt enable
- UART_INTCFG_THRE : THR Interrupt enable
- UART_INTCFG_RLS : RX line status interrupt enable
- UART1_INTCFG_MS : Modem status interrupt enable (UART1 only)
- UART1_INTCFG_CTS : CTS1 signal transition interrupt enable (UART1 only)
- UART_INTCFG_ABEO : Enables the end of auto-baud interrupt
- UART_INTCFG_ABTO : Enables the auto-baud time-out interrupt
[in] NewState New state of specified UART interrupt type, should be: - ENALBE: Enable this UART interrupt type.
- DISALBE: Disable this UART interrupt type.
- Returns:
- None
Definition at line 775 of file lpc17xx_uart.c.
void UART_IrDACmd | ( | LPC_UART_TypeDef * | UARTx, | |
FunctionalState | NewState | |||
) |
Enable or disable IrDA function on UART peripheral.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART3 (only) [in] NewState New state of IrDA function, should be: - ENABLE: Enable this function.
- DISABLE: Disable this function.
- Returns:
- none
Definition at line 709 of file lpc17xx_uart.c.
void UART_IrDAInvtInputCmd | ( | LPC_UART_TypeDef * | UARTx, | |
FunctionalState | NewState | |||
) |
Enable or disable inverting serial input function of IrDA on UART peripheral.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART3 (only) [in] NewState New state of inverting serial input, should be: - ENABLE: Enable this function.
- DISABLE: Disable this function.
- Returns:
- none
Definition at line 685 of file lpc17xx_uart.c.
void UART_IrDAPulseDivConfig | ( | LPC_UART_TypeDef * | UARTx, | |
UART_IrDA_PULSE_Type | PulseDiv | |||
) |
Configure Pulse divider for IrDA function on UART peripheral.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART3 (only) [in] PulseDiv Pulse Divider value from Peripheral clock, should be one of the following: - UART_IrDA_PULSEDIV2 : Pulse width = 2 * Tpclk
- UART_IrDA_PULSEDIV4 : Pulse width = 4 * Tpclk
- UART_IrDA_PULSEDIV8 : Pulse width = 8 * Tpclk
- UART_IrDA_PULSEDIV16 : Pulse width = 16 * Tpclk
- UART_IrDA_PULSEDIV32 : Pulse width = 32 * Tpclk
- UART_IrDA_PULSEDIV64 : Pulse width = 64 * Tpclk
- UART_IrDA_PULSEDIV128 : Pulse width = 128 * Tpclk
- UART_IrDA_PULSEDIV256 : Pulse width = 256 * Tpclk
- Returns:
- none
Definition at line 741 of file lpc17xx_uart.c.
uint32_t UART_Receive | ( | LPC_UART_TypeDef * | UARTx, | |
uint8_t * | rxbuf, | |||
uint32_t | buflen, | |||
TRANSFER_BLOCK_Type | flag | |||
) |
Receive a block of data via UART peripheral.
- Parameters:
-
[in] UARTx Selected UART peripheral used to send data, should be UART0, UART1, UART2 or UART3. [out] rxbuf Pointer to Received buffer [in] buflen Length of Received buffer [in] flag Flag mode, should be NONE_BLOCKING or BLOCKING
- Returns:
- Number of bytes received
Definition at line 1405 of file lpc17xx_uart.c.
uint8_t UART_ReceiveData | ( | LPC_UART_TypeDef * | UARTx | ) |
Receive a single data from UART peripheral.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3.
- Returns:
- Data received
Definition at line 637 of file lpc17xx_uart.c.
void UART_RS485Config | ( | LPC_UART1_TypeDef * | UARTx, | |
UART1_RS485_CTRLCFG_Type * | RS485ConfigStruct | |||
) |
Configure UART peripheral in RS485 mode according to the specified parameters in the RS485ConfigStruct.
- Parameters:
-
[in] UARTx UART1 (only) [in] RS485ConfigStruct Pointer to a UART1_RS485_CTRLCFG_Type structure that contains the configuration information for specified UART in RS485 mode.
- Returns:
- None
Definition at line 1190 of file lpc17xx_uart.c.
void UART_RS485ReceiverCmd | ( | LPC_UART1_TypeDef * | UARTx, | |
FunctionalState | NewState | |||
) |
Enable/Disable receiver in RS485 module in UART1.
- Parameters:
-
[in] UARTx UART1 only. [in] NewState New State of command, should be: - ENABLE: Enable this function.
- DISABLE: Disable this function.
- Returns:
- None
Definition at line 1263 of file lpc17xx_uart.c.
uint32_t UART_RS485Send | ( | LPC_UART1_TypeDef * | UARTx, | |
uint8_t * | pDatFrm, | |||
uint32_t | size, | |||
uint8_t | ParityStick | |||
) |
Send data on RS485 bus with specified parity stick value (9-bit mode).
- Parameters:
-
[in] UARTx UART1 (only). [in] pDatFrm Pointer to data frame. [in] size Size of data. [in] ParityStick Parity Stick value, should be 0 or 1.
- Returns:
- None.
Definition at line 1281 of file lpc17xx_uart.c.
uint32_t UART_RS485SendData | ( | LPC_UART1_TypeDef * | UARTx, | |
uint8_t * | pData, | |||
uint32_t | size | |||
) |
Send Data frames on RS485 bus.
- Parameters:
-
[in] UARTx UART1 (only). [in] pData Pointer to data to be sent. [in] size Size of data frame to be sent.
- Returns:
- None.
Definition at line 1321 of file lpc17xx_uart.c.
void UART_RS485SendSlvAddr | ( | LPC_UART1_TypeDef * | UARTx, | |
uint8_t | SlvAddr | |||
) |
Send Slave address frames on RS485 bus.
- Parameters:
-
[in] UARTx UART1 (only). [in] SlvAddr Slave Address.
- Returns:
- None.
Definition at line 1308 of file lpc17xx_uart.c.
uint32_t UART_Send | ( | LPC_UART_TypeDef * | UARTx, | |
uint8_t * | txbuf, | |||
uint32_t | buflen, | |||
TRANSFER_BLOCK_Type | flag | |||
) |
Send a block of data via UART peripheral.
- Parameters:
-
[in] UARTx Selected UART peripheral used to send data, should be UART0, UART1, UART2 or UART3. [in] txbuf Pointer to Transmit buffer [in] buflen Length of Transmit buffer [in] flag Flag used in UART transfer, should be NONE_BLOCKING or BLOCKING
- Returns:
- Number of bytes sent.
Definition at line 1344 of file lpc17xx_uart.c.
void UART_SendData | ( | LPC_UART_TypeDef * | UARTx, | |
uint8_t | Data | |||
) |
Transmit a single data through UART peripheral.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3. [in] Data Data to transmit (must be 8-bit long)
- Returns:
- none
Definition at line 615 of file lpc17xx_uart.c.
void UART_SetupCbs | ( | LPC_UART_TypeDef * | UARTx, | |
uint8_t | CbType, | |||
void * | pfnCbs | |||
) |
Setup call-back function for UART interrupt handler for each UART peripheral.
- Parameters:
-
[in] UARTx Selected UART peripheral, should be UART0..3 [in] CbType Call-back type, should be: 0 - Receive Call-back 1 - Transmit Call-back 2 - Auto Baudrate Callback 3 - Error Call-back 4 - Modem Status Call-back (UART1 only) [in] pfnCbs Pointer to Call-back function
- Returns:
- None
Definition at line 1460 of file lpc17xx_uart.c.
void UART_TxCmd | ( | LPC_UART_TypeDef * | UARTx, | |
FunctionalState | NewState | |||
) |
Enable/Disable transmission on UART TxD pin.
- Parameters:
-
[in] UARTx UART peripheral selected, should be UART0, UART1, UART2 or UART3. [in] NewState New State of Tx transmission function, should be: - ENABLE: Enable this function
- DISABLE: Disable this function
- Returns:
- none
Definition at line 1046 of file lpc17xx_uart.c.
Generated on Mon Feb 8 10:01:49 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
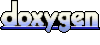