MCPWM_Public_Functions
[MCPWM]
Functions | |
void | MCPWM_Init (LPC_MCPWM_TypeDef *MCPWMx) |
Initializes the MCPWM peripheral. | |
void | MCPWM_ConfigChannel (LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, MCPWM_CHANNEL_CFG_Type *channelSetup) |
Configures each channel in MCPWM peripheral according to the specified parameters in the MCPWM_CHANNEL_CFG_Type. | |
void | MCPWM_WriteToShadow (LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, MCPWM_CHANNEL_CFG_Type *channelSetup) |
Write to MCPWM shadow registers - Update the value for period and pulse width in MCPWM peripheral. | |
void | MCPWM_ConfigCapture (LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, MCPWM_CAPTURE_CFG_Type *captureConfig) |
Configures capture function in MCPWM peripheral. | |
void | MCPWM_ClearCapture (LPC_MCPWM_TypeDef *MCPWMx, uint32_t captureChannel) |
Clears current captured value in specified capture channel. | |
uint32_t | MCPWM_GetCapture (LPC_MCPWM_TypeDef *MCPWMx, uint32_t captureChannel) |
Get current captured value in specified capture channel. | |
void | MCPWM_CountConfig (LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, uint32_t countMode, MCPWM_COUNT_CFG_Type *countConfig) |
Configures Count control in MCPWM peripheral. | |
void | MCPWM_Start (LPC_MCPWM_TypeDef *MCPWMx, uint32_t channel0, uint32_t channel1, uint32_t channel2) |
Start MCPWM activity for each MCPWM channel. | |
void | MCPWM_Stop (LPC_MCPWM_TypeDef *MCPWMx, uint32_t channel0, uint32_t channel1, uint32_t channel2) |
Stop MCPWM activity for each MCPWM channel. | |
void | MCPWM_ACMode (LPC_MCPWM_TypeDef *MCPWMx, uint32_t acMode) |
Enables/Disables 3-phase AC motor mode on MCPWM peripheral. | |
void | MCPWM_DCMode (LPC_MCPWM_TypeDef *MCPWMx, uint32_t dcMode, uint32_t outputInvered, uint32_t outputPattern) |
Enables/Disables 3-phase DC motor mode on MCPWM peripheral. | |
void | MCPWM_IntConfig (LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType, FunctionalState NewState) |
Configures the specified interrupt in MCPWM peripheral. | |
void | MCPWM_IntSet (LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType) |
Sets/Forces the specified interrupt for MCPWM peripheral. | |
void | MCPWM_IntClear (LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType) |
Clear the specified interrupt pending for MCPWM peripheral. | |
FlagStatus | MCPWM_GetIntStatus (LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType) |
Check whether if the specified interrupt in MCPWM is set or not. |
Function Documentation
void MCPWM_ACMode | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | acMode | |||
) |
Enables/Disables 3-phase AC motor mode on MCPWM peripheral.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] acMode State of this command, should be: - ENABLE.
- DISABLE.
- Returns:
- None
Definition at line 320 of file lpc17xx_mcpwm.c.
void MCPWM_ClearCapture | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | captureChannel | |||
) |
Clears current captured value in specified capture channel.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] captureChannel Capture channel number, should be in range from 0 to 2
- Returns:
- None
Definition at line 205 of file lpc17xx_mcpwm.c.
void MCPWM_ConfigCapture | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | channelNum, | |||
MCPWM_CAPTURE_CFG_Type * | captureConfig | |||
) |
Configures capture function in MCPWM peripheral.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] channelNum MCI (Motor Control Input pin) number, should be in range from 0 to 2. [in] captureConfig Pointer to a MCPWM_CAPTURE_CFG_Type structure that contains the configuration information for the specified MCPWM capture.
- Returns:
Definition at line 167 of file lpc17xx_mcpwm.c.
void MCPWM_ConfigChannel | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | channelNum, | |||
MCPWM_CHANNEL_CFG_Type * | channelSetup | |||
) |
Configures each channel in MCPWM peripheral according to the specified parameters in the MCPWM_CHANNEL_CFG_Type.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] channelNum Channel number, should be in range from 0 to 2. [in] channelSetup Pointer to a MCPWM_CHANNEL_CFG_Type structure that contains the configuration information for the specified MCPWM channel.
- Returns:
- None
Definition at line 82 of file lpc17xx_mcpwm.c.
void MCPWM_CountConfig | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | channelNum, | |||
uint32_t | countMode, | |||
MCPWM_COUNT_CFG_Type * | countConfig | |||
) |
Configures Count control in MCPWM peripheral.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] channelNum Channel number, should be in range from 0 to 2 [in] countMode Count mode, should be: - ENABLE: Enables count mode.
- DISABLE: Disable count mode, the channel is in timer mode.
[in] countConfig Pointer to a MCPWM_COUNT_CFG_Type structure that contains the configuration information for the specified MCPWM count control.
- Returns:
- None
Definition at line 241 of file lpc17xx_mcpwm.c.
void MCPWM_DCMode | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | dcMode, | |||
uint32_t | outputInvered, | |||
uint32_t | outputPattern | |||
) |
Enables/Disables 3-phase DC motor mode on MCPWM peripheral.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] dcMode State of this command, should be: - ENABLE.
- DISABLE.
[in] outputInvered Polarity of the MCOB outputs for all 3 channels, should be: - ENABLE: The MCOB outputs have opposite polarity from the MCOA outputs.
- DISABLE: The MCOB outputs have the same basic polarity as the MCOA outputs.
[in] outputPattern A value contains bits that enables/disables the specified output pins route to the internal MCOA0 signal, should be: - MCPWM_PATENT_A0: MCOA0 tracks internal MCOA0
- MCPWM_PATENT_B0: MCOB0 tracks internal MCOA0
- MCPWM_PATENT_A1: MCOA1 tracks internal MCOA0
- MCPWM_PATENT_B1: MCOB1 tracks internal MCOA0
- MCPWM_PATENT_A2: MCOA2 tracks internal MCOA0
- MCPWM_PATENT_B2: MCOB2 tracks internal MCOA0
- Returns:
- None
Definition at line 354 of file lpc17xx_mcpwm.c.
uint32_t MCPWM_GetCapture | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | captureChannel | |||
) |
Get current captured value in specified capture channel.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] captureChannel Capture channel number, should be in range from 0 to 2
- Returns:
- None
Definition at line 216 of file lpc17xx_mcpwm.c.
FlagStatus MCPWM_GetIntStatus | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | ulIntType | |||
) |
Check whether if the specified interrupt in MCPWM is set or not.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] ulIntType Interrupt type, should be: - MCPWM_INTFLAG_LIM0: Limit interrupt for channel (0)
- MCPWM_INTFLAG_MAT0: Match interrupt for channel (0)
- MCPWM_INTFLAG_CAP0: Capture interrupt for channel (0)
- MCPWM_INTFLAG_LIM1: Limit interrupt for channel (1)
- MCPWM_INTFLAG_MAT1: Match interrupt for channel (1)
- MCPWM_INTFLAG_CAP1: Capture interrupt for channel (1)
- MCPWM_INTFLAG_LIM2: Limit interrupt for channel (2)
- MCPWM_INTFLAG_MAT2: Match interrupt for channel (2)
- MCPWM_INTFLAG_CAP2: Capture interrupt for channel (2)
- MCPWM_INTFLAG_ABORT: Fast abort interrupt
- Returns:
- None
Definition at line 466 of file lpc17xx_mcpwm.c.
void MCPWM_Init | ( | LPC_MCPWM_TypeDef * | MCPWMx | ) |
Initializes the MCPWM peripheral.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM
- Returns:
- None
Definition at line 53 of file lpc17xx_mcpwm.c.
void MCPWM_IntClear | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | ulIntType | |||
) |
Clear the specified interrupt pending for MCPWM peripheral.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] ulIntType Interrupt type, should be: - MCPWM_INTFLAG_LIM0: Limit interrupt for channel (0)
- MCPWM_INTFLAG_MAT0: Match interrupt for channel (0)
- MCPWM_INTFLAG_CAP0: Capture interrupt for channel (0)
- MCPWM_INTFLAG_LIM1: Limit interrupt for channel (1)
- MCPWM_INTFLAG_MAT1: Match interrupt for channel (1)
- MCPWM_INTFLAG_CAP1: Capture interrupt for channel (1)
- MCPWM_INTFLAG_LIM2: Limit interrupt for channel (2)
- MCPWM_INTFLAG_MAT2: Match interrupt for channel (2)
- MCPWM_INTFLAG_CAP2: Capture interrupt for channel (2)
- MCPWM_INTFLAG_ABORT: Fast abort interrupt
- Returns:
- None Note: all these ulIntType values above can be ORed together for using as input parameter.
Definition at line 444 of file lpc17xx_mcpwm.c.
void MCPWM_IntConfig | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | ulIntType, | |||
FunctionalState | NewState | |||
) |
Configures the specified interrupt in MCPWM peripheral.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] ulIntType Interrupt type, should be: - MCPWM_INTFLAG_LIM0: Limit interrupt for channel (0)
- MCPWM_INTFLAG_MAT0: Match interrupt for channel (0)
- MCPWM_INTFLAG_CAP0: Capture interrupt for channel (0)
- MCPWM_INTFLAG_LIM1: Limit interrupt for channel (1)
- MCPWM_INTFLAG_MAT1: Match interrupt for channel (1)
- MCPWM_INTFLAG_CAP1: Capture interrupt for channel (1)
- MCPWM_INTFLAG_LIM2: Limit interrupt for channel (2)
- MCPWM_INTFLAG_MAT2: Match interrupt for channel (2)
- MCPWM_INTFLAG_CAP2: Capture interrupt for channel (2)
- MCPWM_INTFLAG_ABORT: Fast abort interrupt
[in] NewState New State of this command, should be: - ENABLE.
- DISABLE.
- Returns:
- None
Definition at line 394 of file lpc17xx_mcpwm.c.
void MCPWM_IntSet | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | ulIntType | |||
) |
Sets/Forces the specified interrupt for MCPWM peripheral.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] ulIntType Interrupt type, should be: - MCPWM_INTFLAG_LIM0: Limit interrupt for channel (0)
- MCPWM_INTFLAG_MAT0: Match interrupt for channel (0)
- MCPWM_INTFLAG_CAP0: Capture interrupt for channel (0)
- MCPWM_INTFLAG_LIM1: Limit interrupt for channel (1)
- MCPWM_INTFLAG_MAT1: Match interrupt for channel (1)
- MCPWM_INTFLAG_CAP1: Capture interrupt for channel (1)
- MCPWM_INTFLAG_LIM2: Limit interrupt for channel (2)
- MCPWM_INTFLAG_MAT2: Match interrupt for channel (2)
- MCPWM_INTFLAG_CAP2: Capture interrupt for channel (2)
- MCPWM_INTFLAG_ABORT: Fast abort interrupt
- Returns:
- None Note: all these ulIntType values above can be ORed together for using as input parameter.
Definition at line 421 of file lpc17xx_mcpwm.c.
void MCPWM_Start | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | channel0, | |||
uint32_t | channel1, | |||
uint32_t | channel2 | |||
) |
Start MCPWM activity for each MCPWM channel.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] channel0 State of this command on channel 0: - ENABLE: 'Start' command will effect on channel 0
- DISABLE: 'Start' command will not effect on channel 0
[in] channel1 State of this command on channel 1: - ENABLE: 'Start' command will effect on channel 1
- DISABLE: 'Start' command will not effect on channel 1
[in] channel2 State of this command on channel 2: - ENABLE: 'Start' command will effect on channel 2
- DISABLE: 'Start' command will not effect on channel 2
- Returns:
- None
Definition at line 278 of file lpc17xx_mcpwm.c.
void MCPWM_Stop | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | channel0, | |||
uint32_t | channel1, | |||
uint32_t | channel2 | |||
) |
Stop MCPWM activity for each MCPWM channel.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] channel0 State of this command on channel 0: - ENABLE: 'Stop' command will effect on channel 0
- DISABLE: 'Stop' command will not effect on channel 0
[in] channel1 State of this command on channel 1: - ENABLE: 'Stop' command will effect on channel 1
- DISABLE: 'Stop' command will not effect on channel 1
[in] channel2 State of this command on channel 2: - ENABLE: 'Stop' command will effect on channel 2
- DISABLE: 'Stop' command will not effect on channel 2
- Returns:
- None
Definition at line 302 of file lpc17xx_mcpwm.c.
void MCPWM_WriteToShadow | ( | LPC_MCPWM_TypeDef * | MCPWMx, | |
uint32_t | channelNum, | |||
MCPWM_CHANNEL_CFG_Type * | channelSetup | |||
) |
Write to MCPWM shadow registers - Update the value for period and pulse width in MCPWM peripheral.
- Parameters:
-
[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM [in] channelNum Channel Number, should be in range from 0 to 2. [in] channelSetup Pointer to a MCPWM_CHANNEL_CFG_Type structure that contains the configuration information for the specified MCPWM channel.
- Returns:
- None
Definition at line 141 of file lpc17xx_mcpwm.c.
Generated on Mon Feb 8 10:01:45 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
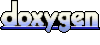