SSP_Public_Functions
[SSP]
Functions | |
void | SSP_SetClock (LPC_SSP_TypeDef *SSPx, uint32_t target_clock) |
Setup clock rate for SSP device. | |
void | SSP_DeInit (LPC_SSP_TypeDef *SSPx) |
De-initializes the SSPx peripheral registers to their default reset values. | |
void | SSP_Init (LPC_SSP_TypeDef *SSPx, SSP_CFG_Type *SSP_ConfigStruct) |
Initializes the SSPx peripheral according to the specified parameters in the SSP_ConfigStruct. | |
void | SSP_ConfigStructInit (SSP_CFG_Type *SSP_InitStruct) |
Fills each SSP_InitStruct member with its default value:
| |
void | SSP_Cmd (LPC_SSP_TypeDef *SSPx, FunctionalState NewState) |
Enable or disable SSP peripheral's operation. | |
void | SSP_LoopBackCmd (LPC_SSP_TypeDef *SSPx, FunctionalState NewState) |
Enable or disable Loop Back mode function in SSP peripheral. | |
void | SSP_SlaveOutputCmd (LPC_SSP_TypeDef *SSPx, FunctionalState NewState) |
Enable or disable Slave Output function in SSP peripheral. | |
void | SSP_SendData (LPC_SSP_TypeDef *SSPx, uint16_t Data) |
Transmit a single data through SSPx peripheral. | |
uint16_t | SSP_ReceiveData (LPC_SSP_TypeDef *SSPx) |
Receive a single data from SSPx peripheral. | |
int32_t | SSP_ReadWrite (LPC_SSP_TypeDef *SSPx, SSP_DATA_SETUP_Type *dataCfg, SSP_TRANSFER_Type xfType) |
SSP Read write data function. | |
FlagStatus | SSP_GetStatus (LPC_SSP_TypeDef *SSPx, uint32_t FlagType) |
Checks whether the specified SSP status flag is set or not. | |
void | SSP_IntConfig (LPC_SSP_TypeDef *SSPx, uint32_t IntType, FunctionalState NewState) |
Enable or disable specified interrupt type in SSP peripheral. | |
IntStatus | SSP_GetRawIntStatus (LPC_SSP_TypeDef *SSPx, uint32_t RawIntType) |
Check whether the specified Raw interrupt status flag is set or not. | |
IntStatus | SSP_GetIntStatus (LPC_SSP_TypeDef *SSPx, uint32_t IntType) |
Check whether the specified interrupt status flag is set or not. | |
void | SSP_ClearIntPending (LPC_SSP_TypeDef *SSPx, uint32_t IntType) |
Clear specified interrupt pending in SSP peripheral. | |
void | SSP_DMACmd (LPC_SSP_TypeDef *SSPx, uint32_t DMAMode, FunctionalState NewState) |
Enable/Disable DMA function for SSP peripheral. | |
void | SSP0_StdIntHandler (void) |
Standard SSP0 Interrupt handler. | |
void | SSP1_StdIntHandler (void) |
Standard SSP1 Interrupt handler. |
Function Documentation
void SSP0_StdIntHandler | ( | void | ) |
Standard SSP0 Interrupt handler.
- Parameters:
-
[in] None
- Returns:
- None
Definition at line 803 of file lpc17xx_ssp.c.
void SSP1_StdIntHandler | ( | void | ) |
Standard SSP1 Interrupt handler.
- Parameters:
-
[in] None
- Returns:
- None
Definition at line 814 of file lpc17xx_ssp.c.
void SSP_ClearIntPending | ( | LPC_SSP_TypeDef * | SSPx, | |
uint32_t | IntType | |||
) |
Clear specified interrupt pending in SSP peripheral.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1 [in] IntType Interrupt pending to clear, should be: - SSP_INTCLR_ROR: clears the "frame was received when RxFIFO was full" interrupt.
- SSP_INTCLR_RT: clears the "Rx FIFO was not empty and has not been read for a timeout period" interrupt.
- Returns:
- None
Definition at line 762 of file lpc17xx_ssp.c.
void SSP_Cmd | ( | LPC_SSP_TypeDef * | SSPx, | |
FunctionalState | NewState | |||
) |
Enable or disable SSP peripheral's operation.
- Parameters:
-
[in] SSPx SSP peripheral, should be SSP0 or SSP1 [in] NewState New State of SSPx peripheral's operation
- Returns:
- none
Definition at line 370 of file lpc17xx_ssp.c.
void SSP_ConfigStructInit | ( | SSP_CFG_Type * | SSP_InitStruct | ) |
Fills each SSP_InitStruct member with its default value:
- CPHA = SSP_CPHA_FIRST
- CPOL = SSP_CPOL_HI
- ClockRate = 1000000
- Databit = SSP_DATABIT_8
- Mode = SSP_MASTER_MODE
- FrameFormat = SSP_FRAME_SSP.
- Parameters:
-
[in] SSP_InitStruct Pointer to a SSP_CFG_Type structure which will be initialized.
- Returns:
- None
Definition at line 353 of file lpc17xx_ssp.c.
void SSP_DeInit | ( | LPC_SSP_TypeDef * | SSPx | ) |
De-initializes the SSPx peripheral registers to their default reset values.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1
- Returns:
- None
Definition at line 276 of file lpc17xx_ssp.c.
void SSP_DMACmd | ( | LPC_SSP_TypeDef * | SSPx, | |
uint32_t | DMAMode, | |||
FunctionalState | NewState | |||
) |
Enable/Disable DMA function for SSP peripheral.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1 [in] DMAMode Type of DMA, should be: - SSP_DMA_TX: DMA for the transmit FIFO
- SSP_DMA_RX: DMA for the Receive FIFO
[in] NewState New State of DMA function on SSP peripheral, should be: - ENALBE: Enable this function
- DISABLE: Disable this function
- Returns:
- None
Definition at line 782 of file lpc17xx_ssp.c.
IntStatus SSP_GetIntStatus | ( | LPC_SSP_TypeDef * | SSPx, | |
uint32_t | IntType | |||
) |
Check whether the specified interrupt status flag is set or not.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1 [in] IntType Raw Interrupt Type, should be: - SSP_INTSTAT_ROR: Receive Overrun interrupt
- SSP_INTSTAT_RT: Receive Time out interrupt
- SSP_INTSTAT_RX: RX FIFO is at least half full interrupt
- SSP_INTSTAT_TX: TX FIFO is at least half empty interrupt
- Returns:
- New State of specified interrupt status flag in SSP peripheral Note: Enabling/Disabling specified interrupt in SSP peripheral effects to Interrupt Status flag.
Definition at line 742 of file lpc17xx_ssp.c.
IntStatus SSP_GetRawIntStatus | ( | LPC_SSP_TypeDef * | SSPx, | |
uint32_t | RawIntType | |||
) |
Check whether the specified Raw interrupt status flag is set or not.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1 [in] RawIntType Raw Interrupt Type, should be: - SSP_INTSTAT_RAW_ROR: Receive Overrun interrupt
- SSP_INTSTAT_RAW_RT: Receive Time out interrupt
- SSP_INTSTAT_RAW_RX: RX FIFO is at least half full interrupt
- SSP_INTSTAT_RAW_TX: TX FIFO is at least half empty interrupt
- Returns:
- New State of specified Raw interrupt status flag in SSP peripheral Note: Enabling/Disabling specified interrupt in SSP peripheral does not effect to Raw Interrupt Status flag.
Definition at line 720 of file lpc17xx_ssp.c.
FlagStatus SSP_GetStatus | ( | LPC_SSP_TypeDef * | SSPx, | |
uint32_t | FlagType | |||
) |
Checks whether the specified SSP status flag is set or not.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1 [in] FlagType Type of flag to check status, should be one of following: - SSP_STAT_TXFIFO_EMPTY: TX FIFO is empty
- SSP_STAT_TXFIFO_NOTFULL: TX FIFO is not full
- SSP_STAT_RXFIFO_NOTEMPTY: RX FIFO is not empty
- SSP_STAT_RXFIFO_FULL: RX FIFO is full
- SSP_STAT_BUSY: SSP peripheral is busy
- Returns:
- New State of specified SSP status flag
Definition at line 668 of file lpc17xx_ssp.c.
void SSP_Init | ( | LPC_SSP_TypeDef * | SSPx, | |
SSP_CFG_Type * | SSP_ConfigStruct | |||
) |
Initializes the SSPx peripheral according to the specified parameters in the SSP_ConfigStruct.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1 [in] SSP_ConfigStruct Pointer to a SSP_CFG_Type structure that contains the configuration information for the specified SSP peripheral.
- Returns:
- None
Definition at line 300 of file lpc17xx_ssp.c.
void SSP_IntConfig | ( | LPC_SSP_TypeDef * | SSPx, | |
uint32_t | IntType, | |||
FunctionalState | NewState | |||
) |
Enable or disable specified interrupt type in SSP peripheral.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1 [in] IntType Interrupt type in SSP peripheral, should be: - SSP_INTCFG_ROR: Receive Overrun interrupt
- SSP_INTCFG_RT: Receive Time out interrupt
- SSP_INTCFG_RX: RX FIFO is at least half full interrupt
- SSP_INTCFG_TX: TX FIFO is at least half empty interrupt
[in] NewState New State of specified interrupt type, should be: - ENABLE: Enable this interrupt type
- DISABLE: Disable this interrupt type
- Returns:
- None
Definition at line 691 of file lpc17xx_ssp.c.
void SSP_LoopBackCmd | ( | LPC_SSP_TypeDef * | SSPx, | |
FunctionalState | NewState | |||
) |
Enable or disable Loop Back mode function in SSP peripheral.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1 [in] NewState New State of Loop Back mode, should be: - ENABLE: Enable this function
- DISABLE: Disable this function
- Returns:
- None
Definition at line 395 of file lpc17xx_ssp.c.
int32_t SSP_ReadWrite | ( | LPC_SSP_TypeDef * | SSPx, | |
SSP_DATA_SETUP_Type * | dataCfg, | |||
SSP_TRANSFER_Type | xfType | |||
) |
SSP Read write data function.
- Parameters:
-
[in] SSPx Pointer to SSP peripheral, should be SSP0 or SSP1 [in] dataCfg Pointer to a SSP_DATA_SETUP_Type structure that contains specified information about transmit data configuration. [in] xfType Transfer type, should be: - SSP_TRANSFER_POLLING: Polling mode
- SSP_TRANSFER_INTERRUPT: Interrupt mode
- Returns:
- Actual Data length has been transferred in polling mode. In interrupt mode, always return (0) Return (-1) if error. Note: This function can be used in both master and slave mode.
Definition at line 482 of file lpc17xx_ssp.c.
uint16_t SSP_ReceiveData | ( | LPC_SSP_TypeDef * | SSPx | ) |
Receive a single data from SSPx peripheral.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP
- Returns:
- Data received (16-bit long)
Definition at line 461 of file lpc17xx_ssp.c.
void SSP_SendData | ( | LPC_SSP_TypeDef * | SSPx, | |
uint16_t | Data | |||
) |
Transmit a single data through SSPx peripheral.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP [in] Data Data to transmit (must be 16 or 8-bit long, this depend on SSP data bit number configured)
- Returns:
- none
Definition at line 447 of file lpc17xx_ssp.c.
void SSP_SetClock | ( | LPC_SSP_TypeDef * | SSPx, | |
uint32_t | target_clock | |||
) |
Setup clock rate for SSP device.
- Parameters:
-
[in] SSPx SSP peripheral definition, should be SSP0 or SSP1. [in] target_clock : clock of SSP (Hz)
- Returns:
- None
Definition at line 227 of file lpc17xx_ssp.c.
void SSP_SlaveOutputCmd | ( | LPC_SSP_TypeDef * | SSPx, | |
FunctionalState | NewState | |||
) |
Enable or disable Slave Output function in SSP peripheral.
- Parameters:
-
[in] SSPx SSP peripheral selected, should be SSP0 or SSP1 [in] NewState New State of Slave Output function, should be: - ENABLE: Slave Output in normal operation
- DISABLE: Slave Output is disabled. This blocks SSP controller from driving the transmit data line (MISO) Note: This function is available when SSP peripheral in Slave mode
- Returns:
- None
Definition at line 423 of file lpc17xx_ssp.c.
Generated on Mon Feb 8 10:01:47 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
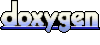