QEI_Public_Functions
[QEI]
Functions | |
void | QEI_Reset (LPC_QEI_TypeDef *QEIx, uint32_t ulResetType) |
Resets value for each type of QEI value, such as velocity, counter, position, etc.. | |
void | QEI_Init (LPC_QEI_TypeDef *QEIx, QEI_CFG_Type *QEI_ConfigStruct) |
Initializes the QEI peripheral according to the specified parameters in the QEI_ConfigStruct. | |
void | QEI_ConfigStructInit (QEI_CFG_Type *QIE_InitStruct) |
Fills each QIE_InitStruct member with its default value:
| |
void | QEI_DeInit (LPC_QEI_TypeDef *QEIx) |
De-initializes the QEI peripheral registers to their default reset values. | |
FlagStatus | QEI_GetStatus (LPC_QEI_TypeDef *QEIx, uint32_t ulFlagType) |
Check whether if specified flag status is set or not. | |
uint32_t | QEI_GetPosition (LPC_QEI_TypeDef *QEIx) |
Get current position value in QEI peripheral. | |
void | QEI_SetMaxPosition (LPC_QEI_TypeDef *QEIx, uint32_t ulMaxPos) |
Set max position value for QEI peripheral. | |
void | QEI_SetPositionComp (LPC_QEI_TypeDef *QEIx, uint8_t bPosCompCh, uint32_t ulPosComp) |
Set position compare value for QEI peripheral. | |
uint32_t | QEI_GetIndex (LPC_QEI_TypeDef *QEIx) |
Get current index counter of QEI peripheral. | |
void | QEI_SetIndexComp (LPC_QEI_TypeDef *QEIx, uint32_t ulIndexComp) |
Set value for index compare in QEI peripheral. | |
void | QEI_SetTimerReload (LPC_QEI_TypeDef *QEIx, QEI_RELOADCFG_Type *QEIReloadStruct) |
Set timer reload value for QEI peripheral. When the velocity timer is over-flow, the value that set for Timer Reload register will be loaded into the velocity timer for next period. The calculated velocity in RPM therefore will be affect by this value. | |
uint32_t | QEI_GetTimer (LPC_QEI_TypeDef *QEIx) |
Get current timer counter in QEI peripheral. | |
uint32_t | QEI_GetVelocity (LPC_QEI_TypeDef *QEIx) |
Get current velocity pulse counter in current time period. | |
uint32_t | QEI_GetVelocityCap (LPC_QEI_TypeDef *QEIx) |
Get the most recently measured velocity of the QEI. When the Velocity timer in QEI is over-flow, the current velocity value will be loaded into Velocity Capture register. | |
void | QEI_SetVelocityComp (LPC_QEI_TypeDef *QEIx, uint32_t ulVelComp) |
Set Velocity Compare value for QEI peripheral. | |
void | QEI_SetDigiFilter (LPC_QEI_TypeDef *QEIx, uint32_t ulSamplingPulse) |
Set value of sampling count for the digital filter in QEI peripheral. | |
FlagStatus | QEI_GetIntStatus (LPC_QEI_TypeDef *QEIx, uint32_t ulIntType) |
Check whether if specified interrupt flag status in QEI peripheral is set or not. | |
void | QEI_IntCmd (LPC_QEI_TypeDef *QEIx, uint32_t ulIntType, FunctionalState NewState) |
Enable/Disable specified interrupt in QEI peripheral. | |
void | QEI_IntSet (LPC_QEI_TypeDef *QEIx, uint32_t ulIntType) |
Sets (forces) specified interrupt in QEI peripheral. | |
void | QEI_IntClear (LPC_QEI_TypeDef *QEIx, uint32_t ulIntType) |
Clear (force) specified interrupt (pending) in QEI peripheral. | |
uint32_t | QEI_CalculateRPM (LPC_QEI_TypeDef *QEIx, uint32_t ulVelCapValue, uint32_t ulPPR) |
Calculates the actual velocity in RPM passed via velocity capture value and Pulse Per Round (of the encoder) value parameter input. |
Function Documentation
uint32_t QEI_CalculateRPM | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulVelCapValue, | |||
uint32_t | ulPPR | |||
) |
Calculates the actual velocity in RPM passed via velocity capture value and Pulse Per Round (of the encoder) value parameter input.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulVelCapValue Velocity capture input value that can be got from QEI_GetVelocityCap() function [in] ulPPR Pulse per round of encoder
- Returns:
- The actual value of velocity in RPM (Round per minute)
Definition at line 474 of file lpc17xx_qei.c.
void QEI_ConfigStructInit | ( | QEI_CFG_Type * | QIE_InitStruct | ) |
Fills each QIE_InitStruct member with its default value:
- DirectionInvert = QEI_DIRINV_NONE
- SignalMode = QEI_SIGNALMODE_QUAD
- CaptureMode = QEI_CAPMODE_4X
- InvertIndex = QEI_INVINX_NONE.
- Parameters:
-
[in] QIE_InitStruct Pointer to a QEI_CFG_Type structure which will be initialized.
- Returns:
- None
Definition at line 154 of file lpc17xx_qei.c.
void QEI_DeInit | ( | LPC_QEI_TypeDef * | QEIx | ) |
De-initializes the QEI peripheral registers to their default reset values.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI
- Returns:
- None
Definition at line 135 of file lpc17xx_qei.c.
uint32_t QEI_GetIndex | ( | LPC_QEI_TypeDef * | QEIx | ) |
Get current index counter of QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI
- Returns:
- Current value of QEI index counter
Definition at line 226 of file lpc17xx_qei.c.
FlagStatus QEI_GetIntStatus | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulIntType | |||
) |
Check whether if specified interrupt flag status in QEI peripheral is set or not.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulIntType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
- Returns:
- New State of specified interrupt flag status (SET or RESET)
Definition at line 353 of file lpc17xx_qei.c.
uint32_t QEI_GetPosition | ( | LPC_QEI_TypeDef * | QEIx | ) |
Get current position value in QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI
- Returns:
- Current position value of QEI peripheral
Definition at line 182 of file lpc17xx_qei.c.
FlagStatus QEI_GetStatus | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulFlagType | |||
) |
Check whether if specified flag status is set or not.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulFlagType Status Flag Type, should be one of the following: - QEI_STATUS_DIR: Direction Status
- Returns:
- New Status of this status flag (SET or RESET)
Definition at line 170 of file lpc17xx_qei.c.
uint32_t QEI_GetTimer | ( | LPC_QEI_TypeDef * | QEIx | ) |
Get current timer counter in QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI
- Returns:
- Current timer counter in QEI peripheral
Definition at line 274 of file lpc17xx_qei.c.
uint32_t QEI_GetVelocity | ( | LPC_QEI_TypeDef * | QEIx | ) |
Get current velocity pulse counter in current time period.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI
- Returns:
- Current velocity pulse counter value
Definition at line 285 of file lpc17xx_qei.c.
uint32_t QEI_GetVelocityCap | ( | LPC_QEI_TypeDef * | QEIx | ) |
Get the most recently measured velocity of the QEI. When the Velocity timer in QEI is over-flow, the current velocity value will be loaded into Velocity Capture register.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI
- Returns:
- The most recently measured velocity value
Definition at line 298 of file lpc17xx_qei.c.
void QEI_Init | ( | LPC_QEI_TypeDef * | QEIx, | |
QEI_CFG_Type * | QEI_ConfigStruct | |||
) |
Initializes the QEI peripheral according to the specified parameters in the QEI_ConfigStruct.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] QEI_ConfigStruct Pointer to a QEI_CFG_Type structure that contains the configuration information for the specified QEI peripheral
- Returns:
- None
Definition at line 94 of file lpc17xx_qei.c.
void QEI_IntClear | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulIntType | |||
) |
Clear (force) specified interrupt (pending) in QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulIntType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
- Returns:
- None
Definition at line 455 of file lpc17xx_qei.c.
void QEI_IntCmd | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulIntType, | |||
FunctionalState | NewState | |||
) |
Enable/Disable specified interrupt in QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulIntType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
- Parameters:
-
[in] NewState New function state, should be:
- DISABLE
- ENABLE
- Returns:
- None
Definition at line 387 of file lpc17xx_qei.c.
void QEI_IntSet | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulIntType | |||
) |
Sets (forces) specified interrupt in QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulIntType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
- Returns:
- None
Definition at line 424 of file lpc17xx_qei.c.
void QEI_Reset | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulResetType | |||
) |
Resets value for each type of QEI value, such as velocity, counter, position, etc..
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulResetType QEI Reset Type, should be one of the following: - QEI_RESET_POS: Reset Position Counter
- QEI_RESET_POSOnIDX: Reset Position Counter on Index signal
- QEI_RESET_VEL: Reset Velocity
- QEI_RESET_IDX: Reset Index Counter
- Returns:
- None
Definition at line 77 of file lpc17xx_qei.c.
void QEI_SetDigiFilter | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulSamplingPulse | |||
) |
Set value of sampling count for the digital filter in QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulSamplingPulse Value of sampling count to set
- Returns:
- None
Definition at line 323 of file lpc17xx_qei.c.
void QEI_SetIndexComp | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulIndexComp | |||
) |
Set value for index compare in QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulIndexComp Compare Index Value to set
- Returns:
- None
Definition at line 238 of file lpc17xx_qei.c.
void QEI_SetMaxPosition | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulMaxPos | |||
) |
Set max position value for QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulMaxPos Max position value to set
- Returns:
- None
Definition at line 194 of file lpc17xx_qei.c.
void QEI_SetPositionComp | ( | LPC_QEI_TypeDef * | QEIx, | |
uint8_t | bPosCompCh, | |||
uint32_t | ulPosComp | |||
) |
Set position compare value for QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] bPosCompCh Compare Position channel, should be: - QEI_COMPPOS_CH_0: QEI compare position channel 0
- QEI_COMPPOS_CH_1: QEI compare position channel 1
- QEI_COMPPOS_CH_2: QEI compare position channel 2
[in] ulPosComp Compare Position value to set
- Returns:
- None
Definition at line 210 of file lpc17xx_qei.c.
void QEI_SetTimerReload | ( | LPC_QEI_TypeDef * | QEIx, | |
QEI_RELOADCFG_Type * | QEIReloadStruct | |||
) |
Set timer reload value for QEI peripheral. When the velocity timer is over-flow, the value that set for Timer Reload register will be loaded into the velocity timer for next period. The calculated velocity in RPM therefore will be affect by this value.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] QEIReloadStruct QEI reload structure
- Returns:
- None
Definition at line 253 of file lpc17xx_qei.c.
void QEI_SetVelocityComp | ( | LPC_QEI_TypeDef * | QEIx, | |
uint32_t | ulVelComp | |||
) |
Set Velocity Compare value for QEI peripheral.
- Parameters:
-
[in] QEIx QEI peripheral, should be QEI [in] ulVelComp Compare Velocity value to set
- Returns:
- None
Definition at line 310 of file lpc17xx_qei.c.
Generated on Mon Feb 8 10:01:46 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
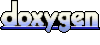