C:/nxpdrv/LPC1700CMSIS/Drivers/source/lpc17xx_emac.c
Go to the documentation of this file.00001 00020 /* Peripheral group ----------------------------------------------------------- */ 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_emac.h" 00027 #include "lpc17xx_clkpwr.h" 00028 00029 /* If this source file built with example, the LPC17xx FW library configuration 00030 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00031 * otherwise the default FW library configuration file must be included instead 00032 */ 00033 #ifdef __BUILD_WITH_EXAMPLE__ 00034 #include "lpc17xx_libcfg.h" 00035 #else 00036 #include "lpc17xx_libcfg_default.h" 00037 #endif /* __BUILD_WITH_EXAMPLE__ */ 00038 00039 00040 #ifdef _EMAC 00041 00042 /* Private Variables ---------------------------------------------------------- */ 00047 /* MII Mgmt Configuration register - Clock divider setting */ 00048 const uint8_t EMAC_clkdiv[] = { 4, 6, 8, 10, 14, 20, 28 }; 00049 00050 /* EMAC local DMA Descriptors */ 00051 00053 static RX_Desc Rx_Desc[EMAC_NUM_RX_FRAG]; 00054 00056 #if defined ( __CC_ARM ) 00057 static __align(8) RX_Stat Rx_Stat[EMAC_NUM_RX_FRAG]; 00058 #elif defined ( __ICCARM__ ) 00059 #pragma data_alignment=8 00060 static RX_Stat Rx_Stat[EMAC_NUM_RX_FRAG]; 00061 #elif defined ( __GNUC__ ) 00062 static __attribute__ ((aligned (8))) RX_Stat Rx_Stat[EMAC_NUM_RX_FRAG]; 00063 #endif 00064 00066 static TX_Desc Tx_Desc[EMAC_NUM_TX_FRAG]; 00068 static TX_Stat Tx_Stat[EMAC_NUM_TX_FRAG]; 00069 00070 /* EMAC local DMA buffers */ 00072 static uint32_t rx_buf[EMAC_NUM_RX_FRAG][EMAC_ETH_MAX_FLEN>>2]; 00074 static uint32_t tx_buf[EMAC_NUM_TX_FRAG][EMAC_ETH_MAX_FLEN>>2]; 00075 00076 /* EMAC call-back function pointer data */ 00077 static EMAC_IntCBSType *_pfnIntCbDat[10]; 00078 00084 /* Private Functions ---------------------------------------------------------- */ 00089 static void rx_descr_init (void); 00090 static void tx_descr_init (void); 00091 static int32_t write_PHY (uint32_t PhyReg, uint16_t Value); 00092 static int32_t read_PHY (uint32_t PhyReg); 00093 00094 00095 /*--------------------------- rx_descr_init ---------------------------------*/ 00096 00102 static void rx_descr_init (void) 00103 { 00104 /* Initialize Receive Descriptor and Status array. */ 00105 uint32_t i; 00106 00107 for (i = 0; i < EMAC_NUM_RX_FRAG; i++) { 00108 Rx_Desc[i].Packet = (uint32_t)&rx_buf[i]; 00109 Rx_Desc[i].Ctrl = EMAC_RCTRL_INT | (EMAC_ETH_MAX_FLEN - 1); 00110 Rx_Stat[i].Info = 0; 00111 Rx_Stat[i].HashCRC = 0; 00112 } 00113 00114 /* Set EMAC Receive Descriptor Registers. */ 00115 LPC_EMAC->RxDescriptor = (uint32_t)&Rx_Desc[0]; 00116 LPC_EMAC->RxStatus = (uint32_t)&Rx_Stat[0]; 00117 LPC_EMAC->RxDescriptorNumber = EMAC_NUM_RX_FRAG - 1; 00118 00119 /* Rx Descriptors Point to 0 */ 00120 LPC_EMAC->RxConsumeIndex = 0; 00121 } 00122 00123 00124 /*--------------------------- tx_descr_init ---- ----------------------------*/ 00130 static void tx_descr_init (void) { 00131 /* Initialize Transmit Descriptor and Status array. */ 00132 uint32_t i; 00133 00134 for (i = 0; i < EMAC_NUM_TX_FRAG; i++) { 00135 Tx_Desc[i].Packet = (uint32_t)&tx_buf[i]; 00136 Tx_Desc[i].Ctrl = 0; 00137 Tx_Stat[i].Info = 0; 00138 } 00139 00140 /* Set EMAC Transmit Descriptor Registers. */ 00141 LPC_EMAC->TxDescriptor = (uint32_t)&Tx_Desc[0]; 00142 LPC_EMAC->TxStatus = (uint32_t)&Tx_Stat[0]; 00143 LPC_EMAC->TxDescriptorNumber = EMAC_NUM_TX_FRAG - 1; 00144 00145 /* Tx Descriptors Point to 0 */ 00146 LPC_EMAC->TxProduceIndex = 0; 00147 } 00148 00149 00150 /*--------------------------- write_PHY -------------------------------------*/ 00157 static int32_t write_PHY (uint32_t PhyReg, uint16_t Value) 00158 { 00159 /* Write a data 'Value' to PHY register 'PhyReg'. */ 00160 uint32_t tout; 00161 00162 LPC_EMAC->MADR = EMAC_DP83848C_DEF_ADR | PhyReg; 00163 LPC_EMAC->MWTD = Value; 00164 00165 /* Wait until operation completed */ 00166 tout = 0; 00167 for (tout = 0; tout < EMAC_MII_WR_TOUT; tout++) { 00168 if ((LPC_EMAC->MIND & EMAC_MIND_BUSY) == 0) { 00169 return (0); 00170 } 00171 } 00172 // Time out! 00173 return (-1); 00174 } 00175 00176 00177 /*--------------------------- read_PHY --------------------------------------*/ 00183 static int32_t read_PHY (uint32_t PhyReg) 00184 { 00185 /* Read a PHY register 'PhyReg'. */ 00186 uint32_t tout; 00187 00188 LPC_EMAC->MADR = EMAC_DP83848C_DEF_ADR | PhyReg; 00189 LPC_EMAC->MCMD = EMAC_MCMD_READ; 00190 00191 /* Wait until operation completed */ 00192 tout = 0; 00193 for (tout = 0; tout < EMAC_MII_RD_TOUT; tout++) { 00194 if ((LPC_EMAC->MIND & EMAC_MIND_BUSY) == 0) { 00195 LPC_EMAC->MCMD = 0; 00196 return (LPC_EMAC->MRDD); 00197 } 00198 } 00199 // Time out! 00200 return (-1); 00201 } 00202 00203 /*********************************************************************/ 00209 void setEmacAddr(uint8_t abStationAddr[]) 00210 { 00211 /* Set the Ethernet MAC Address registers */ 00212 LPC_EMAC->SA0 = ((uint32_t)abStationAddr[5] << 8) | (uint32_t)abStationAddr[4]; 00213 LPC_EMAC->SA1 = ((uint32_t)abStationAddr[3] << 8) | (uint32_t)abStationAddr[2]; 00214 LPC_EMAC->SA2 = ((uint32_t)abStationAddr[1] << 8) | (uint32_t)abStationAddr[0]; 00215 } 00216 00222 /* Public Functions ----------------------------------------------------------- */ 00228 /*********************************************************************/ 00247 Status EMAC_Init(EMAC_CFG_Type *EMAC_ConfigStruct) 00248 { 00249 /* Initialize the EMAC Ethernet controller. */ 00250 int32_t regv,tout, tmp; 00251 00252 /* Set up clock and power for Ethernet module */ 00253 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCENET, ENABLE); 00254 00255 /* Reset all EMAC internal modules */ 00256 LPC_EMAC->MAC1 = EMAC_MAC1_RES_TX | EMAC_MAC1_RES_MCS_TX | EMAC_MAC1_RES_RX | 00257 EMAC_MAC1_RES_MCS_RX | EMAC_MAC1_SIM_RES | EMAC_MAC1_SOFT_RES; 00258 00259 LPC_EMAC->Command = EMAC_CR_REG_RES | EMAC_CR_TX_RES | EMAC_CR_RX_RES | EMAC_CR_PASS_RUNT_FRM; 00260 00261 /* A short delay after reset. */ 00262 for (tout = 100; tout; tout--); 00263 00264 /* Initialize MAC control registers. */ 00265 LPC_EMAC->MAC1 = EMAC_MAC1_PASS_ALL; 00266 LPC_EMAC->MAC2 = EMAC_MAC2_CRC_EN | EMAC_MAC2_PAD_EN; 00267 LPC_EMAC->MAXF = EMAC_ETH_MAX_FLEN; 00268 /* 00269 * Find the clock that close to desired target clock 00270 */ 00271 tmp = SystemCoreClock / EMAC_MCFG_MII_MAXCLK; 00272 for (tout = 0; tout < sizeof (EMAC_clkdiv); tout++){ 00273 if (EMAC_clkdiv[tout] >= tmp) break; 00274 } 00275 tout++; 00276 // Write to MAC configuration register and reset 00277 LPC_EMAC->MCFG = EMAC_MCFG_CLK_SEL(tout) | EMAC_MCFG_RES_MII; 00278 // release reset 00279 LPC_EMAC->MCFG &= ~(EMAC_MCFG_RES_MII); 00280 LPC_EMAC->CLRT = EMAC_CLRT_DEF; 00281 LPC_EMAC->IPGR = EMAC_IPGR_P2_DEF; 00282 00283 /* Enable Reduced MII interface. */ 00284 LPC_EMAC->Command = EMAC_CR_RMII | EMAC_CR_PASS_RUNT_FRM; 00285 00286 /* Reset Reduced MII Logic. */ 00287 LPC_EMAC->SUPP = EMAC_SUPP_RES_RMII; 00288 00289 for (tout = 100; tout; tout--); 00290 LPC_EMAC->SUPP = 0; 00291 00292 /* Put the DP83848C in reset mode */ 00293 write_PHY (EMAC_PHY_REG_BMCR, EMAC_PHY_BMCR_RESET); 00294 00295 /* Wait for hardware reset to end. */ 00296 for (tout = EMAC_PHY_RESP_TOUT; tout; tout--) { 00297 regv = read_PHY (EMAC_PHY_REG_BMCR); 00298 if (!(regv & (EMAC_PHY_BMCR_RESET | EMAC_PHY_BMCR_POWERDOWN))) { 00299 /* Reset complete, device not Power Down. */ 00300 break; 00301 } 00302 if (tout == 0){ 00303 // Time out, return ERROR 00304 return (ERROR); 00305 } 00306 } 00307 00308 // Set PHY mode 00309 if (EMAC_SetPHYMode(EMAC_ConfigStruct->Mode) < 0){ 00310 return (ERROR); 00311 } 00312 00313 // Set EMAC address 00314 setEmacAddr(EMAC_ConfigStruct->pbEMAC_Addr); 00315 00316 /* Initialize Tx and Rx DMA Descriptors */ 00317 rx_descr_init (); 00318 tx_descr_init (); 00319 00320 // Set Receive Filter register: enable broadcast and multicast 00321 LPC_EMAC->RxFilterCtrl = EMAC_RFC_MCAST_EN | EMAC_RFC_BCAST_EN | EMAC_RFC_PERFECT_EN; 00322 00323 /* Enable Rx Done and Tx Done interrupt for EMAC */ 00324 LPC_EMAC->IntEnable = EMAC_INT_RX_DONE | EMAC_INT_TX_DONE; 00325 00326 /* Reset all interrupts */ 00327 LPC_EMAC->IntClear = 0xFFFF; 00328 00329 /* Enable receive and transmit mode of MAC Ethernet core */ 00330 LPC_EMAC->Command |= (EMAC_CR_RX_EN | EMAC_CR_TX_EN); 00331 LPC_EMAC->MAC1 |= EMAC_MAC1_REC_EN; 00332 00333 return SUCCESS; 00334 } 00335 00336 00337 /*********************************************************************/ 00343 void EMAC_DeInit(void) 00344 { 00345 // Disable all interrupt 00346 LPC_EMAC->IntEnable = 0x00; 00347 // Clear all pending interrupt 00348 LPC_EMAC->IntClear = (0xFF) | (EMAC_INT_SOFT_INT | EMAC_INT_WAKEUP); 00349 00350 /* TurnOff clock and power for Ethernet module */ 00351 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCENET, DISABLE); 00352 } 00353 00354 00355 /*********************************************************************/ 00375 int32_t EMAC_CheckPHYStatus(uint32_t ulPHYState) 00376 { 00377 int32_t regv, tmp; 00378 00379 regv = read_PHY (EMAC_PHY_REG_STS); 00380 switch(ulPHYState){ 00381 case EMAC_PHY_STAT_LINK: 00382 tmp = (regv & EMAC_PHY_SR_LINK) ? 1 : 0; 00383 break; 00384 case EMAC_PHY_STAT_SPEED: 00385 tmp = (regv & EMAC_PHY_SR_SPEED) ? 0 : 1; 00386 break; 00387 case EMAC_PHY_STAT_DUP: 00388 tmp = (regv & EMAC_PHY_SR_DUP) ? 1 : 0; 00389 break; 00390 default: 00391 tmp = -1; 00392 break; 00393 } 00394 return (tmp); 00395 } 00396 00397 00398 /*********************************************************************/ 00408 int32_t EMAC_SetPHYMode(uint32_t ulPHYMode) 00409 { 00410 int32_t id1, id2, tout, regv; 00411 00412 /* Check if this is a DP83848C PHY. */ 00413 id1 = read_PHY (EMAC_PHY_REG_IDR1); 00414 id2 = read_PHY (EMAC_PHY_REG_IDR2); 00415 00416 if (((id1 << 16) | (id2 & 0xFFF0)) == EMAC_DP83848C_ID) { 00417 /* Configure the PHY device */ 00418 switch(ulPHYMode){ 00419 case EMAC_MODE_AUTO: 00420 /* Use auto-negotiation about the link speed. */ 00421 write_PHY (EMAC_PHY_REG_BMCR, EMAC_PHY_AUTO_NEG); 00422 /* Wait to complete Auto_Negotiation */ 00423 for (tout = EMAC_PHY_RESP_TOUT; tout; tout--) { 00424 regv = read_PHY (EMAC_PHY_REG_BMSR); 00425 if (regv & EMAC_PHY_BMSR_AUTO_DONE) { 00426 /* Auto-negotiation Complete. */ 00427 break; 00428 } 00429 if (tout == 0){ 00430 // Time out, return error 00431 return (-1); 00432 } 00433 } 00434 break; 00435 case EMAC_MODE_10M_FULL: 00436 /* Connect at 10MBit full-duplex */ 00437 write_PHY (EMAC_PHY_REG_BMCR, EMAC_PHY_FULLD_10M); 00438 break; 00439 case EMAC_MODE_10M_HALF: 00440 /* Connect at 10MBit half-duplex */ 00441 write_PHY (EMAC_PHY_REG_BMCR, EMAC_PHY_HALFD_10M); 00442 break; 00443 case EMAC_MODE_100M_FULL: 00444 /* Connect at 100MBit full-duplex */ 00445 write_PHY (EMAC_PHY_REG_BMCR, EMAC_PHY_FULLD_100M); 00446 break; 00447 case EMAC_MODE_100M_HALF: 00448 /* Connect at 100MBit half-duplex */ 00449 write_PHY (EMAC_PHY_REG_BMCR, EMAC_PHY_HALFD_100M); 00450 break; 00451 default: 00452 // un-supported 00453 return (-1); 00454 } 00455 } 00456 // It's not correct module ID 00457 else { 00458 return (-1); 00459 } 00460 00461 // Update EMAC configuration with current PHY status 00462 if (EMAC_UpdatePHYStatus() < 0){ 00463 return (-1); 00464 } 00465 00466 // Complete 00467 return (0); 00468 } 00469 00470 00471 /*********************************************************************/ 00481 int32_t EMAC_UpdatePHYStatus(void) 00482 { 00483 int32_t regv, tout; 00484 00485 /* Check the link status. */ 00486 for (tout = EMAC_PHY_RESP_TOUT; tout; tout--) { 00487 regv = read_PHY (EMAC_PHY_REG_STS); 00488 if (regv & EMAC_PHY_SR_LINK) { 00489 /* Link is on. */ 00490 break; 00491 } 00492 if (tout == 0){ 00493 // time out 00494 return (-1); 00495 } 00496 } 00497 00498 /* Configure Full/Half Duplex mode. */ 00499 if (regv & EMAC_PHY_SR_DUP) { 00500 /* Full duplex is enabled. */ 00501 LPC_EMAC->MAC2 |= EMAC_MAC2_FULL_DUP; 00502 LPC_EMAC->Command |= EMAC_CR_FULL_DUP; 00503 LPC_EMAC->IPGT = EMAC_IPGT_FULL_DUP; 00504 } else { 00505 /* Half duplex mode. */ 00506 LPC_EMAC->IPGT = EMAC_IPGT_HALF_DUP; 00507 } 00508 00509 /* Configure 100MBit/10MBit mode. */ 00510 if (regv & EMAC_PHY_SR_SPEED) { 00511 /* 10MBit mode. */ 00512 LPC_EMAC->SUPP = 0; 00513 } else { 00514 /* 100MBit mode. */ 00515 LPC_EMAC->SUPP = EMAC_SUPP_SPEED; 00516 } 00517 00518 // Complete 00519 return (0); 00520 } 00521 00522 00523 /*********************************************************************/ 00542 void EMAC_SetHashFilter(uint8_t dstMAC_addr[], FunctionalState NewState) 00543 { 00544 uint32_t *pReg; 00545 uint32_t tmp; 00546 int32_t crc; 00547 00548 // Calculate the CRC from the destination MAC address 00549 crc = EMAC_CRCCalc(dstMAC_addr, 6); 00550 // Extract the value from CRC to get index value for hash filter table 00551 crc = (crc >> 23) & 0x3F; 00552 00553 pReg = (crc > 31) ? ((uint32_t *)&LPC_EMAC->HashFilterH) \ 00554 : ((uint32_t *)&LPC_EMAC->HashFilterL); 00555 tmp = (crc > 31) ? (crc - 32) : crc; 00556 if (NewState == ENABLE) { 00557 (*pReg) |= (1UL << tmp); 00558 } else { 00559 (*pReg) &= ~(1UL << tmp); 00560 } 00561 // Enable Rx Filter 00562 LPC_EMAC->Command &= ~EMAC_CR_PASS_RX_FILT; 00563 } 00564 00565 00566 /*********************************************************************/ 00572 int32_t EMAC_CRCCalc(uint8_t frame_no_fcs[], int32_t frame_len) 00573 { 00574 int i; // iterator 00575 int j; // another iterator 00576 char byte; // current byte 00577 int crc; // CRC result 00578 int q0, q1, q2, q3; // temporary variables 00579 crc = 0xFFFFFFFF; 00580 for (i = 0; i < frame_len; i++) { 00581 byte = *frame_no_fcs++; 00582 for (j = 0; j < 2; j++) { 00583 if (((crc >> 28) ^ (byte >> 3)) & 0x00000001) { 00584 q3 = 0x04C11DB7; 00585 } else { 00586 q3 = 0x00000000; 00587 } 00588 if (((crc >> 29) ^ (byte >> 2)) & 0x00000001) { 00589 q2 = 0x09823B6E; 00590 } else { 00591 q2 = 0x00000000; 00592 } 00593 if (((crc >> 30) ^ (byte >> 1)) & 0x00000001) { 00594 q1 = 0x130476DC; 00595 } else { 00596 q1 = 0x00000000; 00597 } 00598 if (((crc >> 31) ^ (byte >> 0)) & 0x00000001) { 00599 q0 = 0x2608EDB8; 00600 } else { 00601 q0 = 0x00000000; 00602 } 00603 crc = (crc << 4) ^ q3 ^ q2 ^ q1 ^ q0; 00604 byte >>= 4; 00605 } 00606 } 00607 return crc; 00608 } 00609 00610 /*********************************************************************/ 00637 void EMAC_SetFilterMode(uint32_t ulFilterMode, FunctionalState NewState) 00638 { 00639 if (NewState == ENABLE){ 00640 LPC_EMAC->RxFilterCtrl |= ulFilterMode; 00641 } else { 00642 LPC_EMAC->RxFilterCtrl &= ~ulFilterMode; 00643 } 00644 } 00645 00646 /*********************************************************************/ 00663 FlagStatus EMAC_GetWoLStatus(uint32_t ulWoLMode) 00664 { 00665 if (LPC_EMAC->RxFilterWoLStatus & ulWoLMode) { 00666 LPC_EMAC->RxFilterWoLClear = ulWoLMode; 00667 return SET; 00668 } else { 00669 return RESET; 00670 } 00671 } 00672 00673 00674 /*********************************************************************/ 00682 void EMAC_WritePacketBuffer(EMAC_PACKETBUF_Type *pDataStruct) 00683 { 00684 uint32_t idx,len; 00685 uint32_t *sp,*dp; 00686 00687 idx = LPC_EMAC->TxProduceIndex; 00688 sp = (uint32_t *)pDataStruct->pbDataBuf; 00689 dp = (uint32_t *)Tx_Desc[idx].Packet; 00690 /* Copy frame data to EMAC packet buffers. */ 00691 for (len = (pDataStruct->ulDataLen + 3) >> 2; len; len--) { 00692 *dp++ = *sp++; 00693 } 00694 Tx_Desc[idx].Ctrl = (pDataStruct->ulDataLen - 1) | (EMAC_TCTRL_INT | EMAC_TCTRL_LAST); 00695 } 00696 00697 /*********************************************************************/ 00705 void EMAC_ReadPacketBuffer(EMAC_PACKETBUF_Type *pDataStruct) 00706 { 00707 uint32_t idx, len; 00708 uint32_t *dp, *sp; 00709 00710 idx = LPC_EMAC->RxConsumeIndex; 00711 dp = (uint32_t *)pDataStruct->pbDataBuf; 00712 sp = (uint32_t *)Rx_Desc[idx].Packet; 00713 00714 if (pDataStruct->pbDataBuf != NULL) { 00715 for (len = (pDataStruct->ulDataLen + 3) >> 2; len; len--) { 00716 *dp++ = *sp++; 00717 } 00718 } 00719 } 00720 00721 /*********************************************************************/ 00740 void EMAC_StandardIRQHandler(void) 00741 { 00742 /* EMAC Ethernet Controller Interrupt function. */ 00743 uint32_t n, int_stat; 00744 00745 // Get EMAC interrupt status 00746 while ((int_stat = (LPC_EMAC->IntStatus & LPC_EMAC->IntEnable)) != 0) { 00747 // Clear interrupt status 00748 LPC_EMAC->IntClear = int_stat; 00749 // Execute call-back function 00750 for (n = 0; n <= 7; n++) { 00751 if ((int_stat & (1 << n)) && (_pfnIntCbDat[n] != NULL)) { 00752 _pfnIntCbDat[n](); 00753 } 00754 } 00755 // Soft interrupt 00756 if ((int_stat & EMAC_INT_SOFT_INT) && (_pfnIntCbDat[8] != NULL)) { 00757 _pfnIntCbDat[8](); 00758 } 00759 // WakeUp interrupt 00760 if ((int_stat & EMAC_INT_WAKEUP) && (_pfnIntCbDat[9] != NULL)) { 00761 // Clear WoL interrupt 00762 LPC_EMAC->RxFilterWoLClear = EMAC_WOL_BITMASK; 00763 _pfnIntCbDat[9](); 00764 } 00765 } 00766 } 00767 00768 00769 /*********************************************************************/ 00787 void EMAC_SetupIntCBS(uint32_t ulIntType, EMAC_IntCBSType *pfnIntCb) 00788 { 00789 /* EMAC Ethernet Controller Interrupt function. */ 00790 uint32_t n; 00791 00792 if (ulIntType <= EMAC_INT_TX_DONE){ 00793 for (n = 0; n <= 7; n++) { 00794 // Found it, install cbs now 00795 if (ulIntType & (1 << n)) { 00796 _pfnIntCbDat[n] = pfnIntCb; 00797 // Don't install cbs any more 00798 break; 00799 } 00800 } 00801 } else if (ulIntType & EMAC_INT_SOFT_INT) { 00802 _pfnIntCbDat[8] = pfnIntCb; 00803 } else if (ulIntType & EMAC_INT_WAKEUP) { 00804 _pfnIntCbDat[9] = pfnIntCb; 00805 } 00806 } 00807 00808 /*********************************************************************/ 00826 void EMAC_IntCmd(uint32_t ulIntType, FunctionalState NewState) 00827 { 00828 if (NewState == ENABLE) { 00829 LPC_EMAC->IntEnable |= ulIntType; 00830 } else { 00831 LPC_EMAC->IntEnable &= ~(ulIntType); 00832 } 00833 } 00834 00835 /*********************************************************************/ 00852 IntStatus EMAC_IntGetStatus(uint32_t ulIntType) 00853 { 00854 if (LPC_EMAC->IntStatus & ulIntType) { 00855 LPC_EMAC->IntClear = ulIntType; 00856 return SET; 00857 } else { 00858 return RESET; 00859 } 00860 } 00861 00862 00863 /*********************************************************************/ 00873 Bool EMAC_CheckReceiveIndex(void) 00874 { 00875 if (LPC_EMAC->RxConsumeIndex != LPC_EMAC->RxProduceIndex) { 00876 return TRUE; 00877 } else { 00878 return FALSE; 00879 } 00880 } 00881 00882 00883 /*********************************************************************/ 00893 Bool EMAC_CheckTransmitIndex(void) 00894 { 00895 uint32_t tmp = LPC_EMAC->TxConsumeIndex -1; 00896 if (LPC_EMAC->TxProduceIndex == tmp) { 00897 return FALSE; 00898 } else { 00899 return TRUE; 00900 } 00901 } 00902 00903 00904 /*********************************************************************/ 00923 FlagStatus EMAC_CheckReceiveDataStatus(uint32_t ulRxStatType) 00924 { 00925 uint32_t idx; 00926 idx = LPC_EMAC->RxConsumeIndex; 00927 return (((Rx_Stat[idx].Info) & ulRxStatType) ? SET : RESET); 00928 } 00929 00930 00931 /*********************************************************************/ 00937 uint32_t EMAC_GetReceiveDataSize(void) 00938 { 00939 uint32_t idx; 00940 idx =LPC_EMAC->RxConsumeIndex; 00941 return ((Rx_Stat[idx].Info) & EMAC_RINFO_SIZE); 00942 } 00943 00944 /*********************************************************************/ 00951 void EMAC_UpdateRxConsumeIndex(void) 00952 { 00953 // Get current Rx consume index 00954 uint32_t idx = LPC_EMAC->RxConsumeIndex; 00955 00956 /* Release frame from EMAC buffer */ 00957 if (++idx == EMAC_NUM_RX_FRAG) idx = 0; 00958 LPC_EMAC->RxConsumeIndex = idx; 00959 } 00960 00961 /*********************************************************************/ 00968 void EMAC_UpdateTxProduceIndex(void) 00969 { 00970 // Get current Tx produce index 00971 uint32_t idx = LPC_EMAC->TxProduceIndex; 00972 00973 /* Start frame transmission */ 00974 if (++idx == EMAC_NUM_TX_FRAG) idx = 0; 00975 LPC_EMAC->TxProduceIndex = idx; 00976 } 00977 00978 00983 #endif /* _EMAC */ 00984 00989 /* --------------------------------- End Of File ------------------------------ */
Generated on Mon Feb 8 10:01:37 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
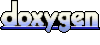