C:/nxpdrv/LPC1700CMSIS/Drivers/include/lpc17xx_emac.h
Go to the documentation of this file.00001 /***********************************************************************/ 00021 /* Peripheral group ----------------------------------------------------------- */ 00027 #ifndef LPC17XX_EMAC_H_ 00028 #define LPC17XX_EMAC_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "LPC17xx.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 00041 /* Private Macros ------------------------------------------------------------- */ 00052 /* EMAC Memory Buffer configuration for 16K Ethernet RAM */ 00053 #define EMAC_NUM_RX_FRAG 4 00054 #define EMAC_NUM_TX_FRAG 3 00055 #define EMAC_ETH_MAX_FLEN 1536 00056 #define EMAC_TX_FRAME_TOUT 0x00100000 00058 /* Ethernet MAC register definitions --------------------------------------------------------------------- */ 00059 /* MAC Configuration Register 1 */ 00060 #define EMAC_MAC1_REC_EN 0x00000001 00061 #define EMAC_MAC1_PASS_ALL 0x00000002 00062 #define EMAC_MAC1_RX_FLOWC 0x00000004 00063 #define EMAC_MAC1_TX_FLOWC 0x00000008 00064 #define EMAC_MAC1_LOOPB 0x00000010 00065 #define EMAC_MAC1_RES_TX 0x00000100 00066 #define EMAC_MAC1_RES_MCS_TX 0x00000200 00067 #define EMAC_MAC1_RES_RX 0x00000400 00068 #define EMAC_MAC1_RES_MCS_RX 0x00000800 00069 #define EMAC_MAC1_SIM_RES 0x00004000 00070 #define EMAC_MAC1_SOFT_RES 0x00008000 00072 /* MAC Configuration Register 2 */ 00073 #define EMAC_MAC2_FULL_DUP 0x00000001 00074 #define EMAC_MAC2_FRM_LEN_CHK 0x00000002 00075 #define EMAC_MAC2_HUGE_FRM_EN 0x00000004 00076 #define EMAC_MAC2_DLY_CRC 0x00000008 00077 #define EMAC_MAC2_CRC_EN 0x00000010 00078 #define EMAC_MAC2_PAD_EN 0x00000020 00079 #define EMAC_MAC2_VLAN_PAD_EN 0x00000040 00080 #define EMAC_MAC2_ADET_PAD_EN 0x00000080 00081 #define EMAC_MAC2_PPREAM_ENF 0x00000100 00082 #define EMAC_MAC2_LPREAM_ENF 0x00000200 00083 #define EMAC_MAC2_NO_BACKOFF 0x00001000 00084 #define EMAC_MAC2_BACK_PRESSURE 0x00002000 00085 #define EMAC_MAC2_EXCESS_DEF 0x00004000 00087 /* Back-to-Back Inter-Packet-Gap Register */ 00088 00090 #define EMAC_IPGT_BBIPG(n) (n&0x7F) 00091 00094 #define EMAC_IPGT_FULL_DUP (EMAC_IPGT_BBIPG(0x15)) 00095 00098 #define EMAC_IPGT_HALF_DUP (EMAC_IPGT_BBIPG(0x12)) 00099 00100 /* Non Back-to-Back Inter-Packet-Gap Register */ 00102 #define EMAC_IPGR_NBBIPG_P2(n) (n&0x7F) 00103 00104 #define EMAC_IPGR_P2_DEF (EMAC_IPGR_NBBIPG_P2(0x12)) 00105 00107 #define EMAC_IPGR_NBBIPG_P1(n) ((n&0x7F)<<8) 00108 00109 #define EMAC_IPGR_P1_DEF EMAC_IPGR_NBBIPG_P1(0x0C) 00110 00111 /* Collision Window/Retry Register */ 00114 #define EMAC_CLRT_MAX_RETX(n) (n&0x0F) 00115 00117 #define EMAC_CLRT_COLL(n) ((n&0x3F)<<8) 00118 00119 #define EMAC_CLRT_DEF ((EMAC_CLRT_MAX_RETX(0x0F))|(EMAC_CLRT_COLL(0x37))) 00120 00121 /* Maximum Frame Register */ 00123 #define EMAC_MAXF_MAXFRMLEN(n) (n&0xFFFF) 00124 00125 /* PHY Support Register */ 00126 #define EMAC_SUPP_SPEED 0x00000100 00127 #define EMAC_SUPP_RES_RMII 0x00000800 00129 /* Test Register */ 00130 #define EMAC_TEST_SHCUT_PQUANTA 0x00000001 00131 #define EMAC_TEST_TST_PAUSE 0x00000002 00132 #define EMAC_TEST_TST_BACKP 0x00000004 00134 /* MII Management Configuration Register */ 00135 #define EMAC_MCFG_SCAN_INC 0x00000001 00136 #define EMAC_MCFG_SUPP_PREAM 0x00000002 00137 #define EMAC_MCFG_CLK_SEL(n) ((n&0x0F)<<2) 00138 #define EMAC_MCFG_RES_MII 0x00008000 00139 #define EMAC_MCFG_MII_MAXCLK 2500000UL 00141 /* MII Management Command Register */ 00142 #define EMAC_MCMD_READ 0x00000001 00143 #define EMAC_MCMD_SCAN 0x00000002 00145 #define EMAC_MII_WR_TOUT 0x00050000 00146 #define EMAC_MII_RD_TOUT 0x00050000 00148 /* MII Management Address Register */ 00149 #define EMAC_MADR_REG_ADR(n) (n&0x1F) 00150 #define EMAC_MADR_PHY_ADR(n) ((n&0x1F)<<8) 00152 /* MII Management Write Data Register */ 00153 #define EMAC_MWTD_DATA(n) (n&0xFFFF) 00155 /* MII Management Read Data Register */ 00156 #define EMAC_MRDD_DATA(n) (n&0xFFFF) 00158 /* MII Management Indicators Register */ 00159 #define EMAC_MIND_BUSY 0x00000001 00160 #define EMAC_MIND_SCAN 0x00000002 00161 #define EMAC_MIND_NOT_VAL 0x00000004 00162 #define EMAC_MIND_MII_LINK_FAIL 0x00000008 00164 /* Station Address 0 Register */ 00165 /* Station Address 1 Register */ 00166 /* Station Address 2 Register */ 00167 00168 00169 /* Control register definitions --------------------------------------------------------------------------- */ 00170 /* Command Register */ 00171 #define EMAC_CR_RX_EN 0x00000001 00172 #define EMAC_CR_TX_EN 0x00000002 00173 #define EMAC_CR_REG_RES 0x00000008 00174 #define EMAC_CR_TX_RES 0x00000010 00175 #define EMAC_CR_RX_RES 0x00000020 00176 #define EMAC_CR_PASS_RUNT_FRM 0x00000040 00177 #define EMAC_CR_PASS_RX_FILT 0x00000080 00178 #define EMAC_CR_TX_FLOW_CTRL 0x00000100 00179 #define EMAC_CR_RMII 0x00000200 00180 #define EMAC_CR_FULL_DUP 0x00000400 00182 /* Status Register */ 00183 #define EMAC_SR_RX_EN 0x00000001 00184 #define EMAC_SR_TX_EN 0x00000002 00186 /* Receive Descriptor Base Address Register */ 00187 // 00188 00189 /* Receive Status Base Address Register */ 00190 // 00191 00192 /* Receive Number of Descriptors Register */ 00193 // 00194 00195 /* Receive Produce Index Register */ 00196 // 00197 00198 /* Receive Consume Index Register */ 00199 // 00200 00201 /* Transmit Descriptor Base Address Register */ 00202 // 00203 00204 /* Transmit Status Base Address Register */ 00205 // 00206 00207 /* Transmit Number of Descriptors Register */ 00208 // 00209 00210 /* Transmit Produce Index Register */ 00211 // 00212 00213 /* Transmit Consume Index Register */ 00214 // 00215 00216 /* Transmit Status Vector 0 Register */ 00217 #define EMAC_TSV0_CRC_ERR 0x00000001 00218 #define EMAC_TSV0_LEN_CHKERR 0x00000002 00219 #define EMAC_TSV0_LEN_OUTRNG 0x00000004 00220 #define EMAC_TSV0_DONE 0x00000008 00221 #define EMAC_TSV0_MCAST 0x00000010 00222 #define EMAC_TSV0_BCAST 0x00000020 00223 #define EMAC_TSV0_PKT_DEFER 0x00000040 00224 #define EMAC_TSV0_EXC_DEFER 0x00000080 00225 #define EMAC_TSV0_EXC_COLL 0x00000100 00226 #define EMAC_TSV0_LATE_COLL 0x00000200 00227 #define EMAC_TSV0_GIANT 0x00000400 00228 #define EMAC_TSV0_UNDERRUN 0x00000800 00229 #define EMAC_TSV0_BYTES 0x0FFFF000 00230 #define EMAC_TSV0_CTRL_FRAME 0x10000000 00231 #define EMAC_TSV0_PAUSE 0x20000000 00232 #define EMAC_TSV0_BACK_PRESS 0x40000000 00233 #define EMAC_TSV0_VLAN 0x80000000 00235 /* Transmit Status Vector 1 Register */ 00236 #define EMAC_TSV1_BYTE_CNT 0x0000FFFF 00237 #define EMAC_TSV1_COLL_CNT 0x000F0000 00239 /* Receive Status Vector Register */ 00240 #define EMAC_RSV_BYTE_CNT 0x0000FFFF 00241 #define EMAC_RSV_PKT_IGNORED 0x00010000 00242 #define EMAC_RSV_RXDV_SEEN 0x00020000 00243 #define EMAC_RSV_CARR_SEEN 0x00040000 00244 #define EMAC_RSV_REC_CODEV 0x00080000 00245 #define EMAC_RSV_CRC_ERR 0x00100000 00246 #define EMAC_RSV_LEN_CHKERR 0x00200000 00247 #define EMAC_RSV_LEN_OUTRNG 0x00400000 00248 #define EMAC_RSV_REC_OK 0x00800000 00249 #define EMAC_RSV_MCAST 0x01000000 00250 #define EMAC_RSV_BCAST 0x02000000 00251 #define EMAC_RSV_DRIB_NIBB 0x04000000 00252 #define EMAC_RSV_CTRL_FRAME 0x08000000 00253 #define EMAC_RSV_PAUSE 0x10000000 00254 #define EMAC_RSV_UNSUPP_OPC 0x20000000 00255 #define EMAC_RSV_VLAN 0x40000000 00257 /* Flow Control Counter Register */ 00258 #define EMAC_FCC_MIRR_CNT(n) (n&0xFFFF) 00259 #define EMAC_FCC_PAUSE_TIM(n) ((n&0xFFFF)<<16) 00261 /* Flow Control Status Register */ 00262 #define EMAC_FCS_MIRR_CNT(n) (n&0xFFFF) 00265 /* Receive filter register definitions -------------------------------------------------------- */ 00266 /* Receive Filter Control Register */ 00267 #define EMAC_RFC_UCAST_EN 0x00000001 00268 #define EMAC_RFC_BCAST_EN 0x00000002 00269 #define EMAC_RFC_MCAST_EN 0x00000004 00270 #define EMAC_RFC_UCAST_HASH_EN 0x00000008 00271 #define EMAC_RFC_MCAST_HASH_EN 0x00000010 00272 #define EMAC_RFC_PERFECT_EN 0x00000020 00273 #define EMAC_RFC_MAGP_WOL_EN 0x00001000 00274 #define EMAC_RFC_PFILT_WOL_EN 0x00002000 00276 /* Receive Filter WoL Status/Clear Registers */ 00277 #define EMAC_WOL_UCAST 0x00000001 00278 #define EMAC_WOL_BCAST 0x00000002 00279 #define EMAC_WOL_MCAST 0x00000004 00280 #define EMAC_WOL_UCAST_HASH 0x00000008 00281 #define EMAC_WOL_MCAST_HASH 0x00000010 00282 #define EMAC_WOL_PERFECT 0x00000020 00283 #define EMAC_WOL_RX_FILTER 0x00000080 00284 #define EMAC_WOL_MAG_PACKET 0x00000100 00285 #define EMAC_WOL_BITMASK 0x01BF 00287 /* Hash Filter Table LSBs Register */ 00288 // 00289 00290 /* Hash Filter Table MSBs Register */ 00291 // 00292 00293 00294 /* Module control register definitions ---------------------------------------------------- */ 00295 /* Interrupt Status/Enable/Clear/Set Registers */ 00296 #define EMAC_INT_RX_OVERRUN 0x00000001 00297 #define EMAC_INT_RX_ERR 0x00000002 00298 #define EMAC_INT_RX_FIN 0x00000004 00299 #define EMAC_INT_RX_DONE 0x00000008 00300 #define EMAC_INT_TX_UNDERRUN 0x00000010 00301 #define EMAC_INT_TX_ERR 0x00000020 00302 #define EMAC_INT_TX_FIN 0x00000040 00303 #define EMAC_INT_TX_DONE 0x00000080 00304 #define EMAC_INT_SOFT_INT 0x00001000 00305 #define EMAC_INT_WAKEUP 0x00002000 00307 /* Power Down Register */ 00308 #define EMAC_PD_POWER_DOWN 0x80000000 00310 /* RX Descriptor Control Word */ 00311 #define EMAC_RCTRL_SIZE(n) (n&0x7FF) 00312 #define EMAC_RCTRL_INT 0x80000000 00314 /* RX Status Hash CRC Word */ 00315 #define EMAC_RHASH_SA 0x000001FF 00316 #define EMAC_RHASH_DA 0x001FF000 00318 /* RX Status Information Word */ 00319 #define EMAC_RINFO_SIZE 0x000007FF 00320 #define EMAC_RINFO_CTRL_FRAME 0x00040000 00321 #define EMAC_RINFO_VLAN 0x00080000 00322 #define EMAC_RINFO_FAIL_FILT 0x00100000 00323 #define EMAC_RINFO_MCAST 0x00200000 00324 #define EMAC_RINFO_BCAST 0x00400000 00325 #define EMAC_RINFO_CRC_ERR 0x00800000 00326 #define EMAC_RINFO_SYM_ERR 0x01000000 00327 #define EMAC_RINFO_LEN_ERR 0x02000000 00328 #define EMAC_RINFO_RANGE_ERR 0x04000000 00329 #define EMAC_RINFO_ALIGN_ERR 0x08000000 00330 #define EMAC_RINFO_OVERRUN 0x10000000 00331 #define EMAC_RINFO_NO_DESCR 0x20000000 00332 #define EMAC_RINFO_LAST_FLAG 0x40000000 00333 #define EMAC_RINFO_ERR 0x80000000 00336 #define EMAC_RINFO_ERR_MASK (EMAC_RINFO_FAIL_FILT | EMAC_RINFO_CRC_ERR | EMAC_RINFO_SYM_ERR | \ 00337 EMAC_RINFO_LEN_ERR | EMAC_RINFO_ALIGN_ERR | EMAC_RINFO_OVERRUN) 00338 00339 /* TX Descriptor Control Word */ 00340 #define EMAC_TCTRL_SIZE 0x000007FF 00341 #define EMAC_TCTRL_OVERRIDE 0x04000000 00342 #define EMAC_TCTRL_HUGE 0x08000000 00343 #define EMAC_TCTRL_PAD 0x10000000 00344 #define EMAC_TCTRL_CRC 0x20000000 00345 #define EMAC_TCTRL_LAST 0x40000000 00346 #define EMAC_TCTRL_INT 0x80000000 00348 /* TX Status Information Word */ 00349 #define EMAC_TINFO_COL_CNT 0x01E00000 00350 #define EMAC_TINFO_DEFER 0x02000000 00351 #define EMAC_TINFO_EXCESS_DEF 0x04000000 00352 #define EMAC_TINFO_EXCESS_COL 0x08000000 00353 #define EMAC_TINFO_LATE_COL 0x10000000 00354 #define EMAC_TINFO_UNDERRUN 0x20000000 00355 #define EMAC_TINFO_NO_DESCR 0x40000000 00356 #define EMAC_TINFO_ERR 0x80000000 00359 /* DP83848C PHY definition ------------------------------------------------------------ */ 00360 00362 #define EMAC_PHY_RESP_TOUT 0x100000UL 00363 00364 /* ENET Device Revision ID */ 00365 #define EMAC_OLD_EMAC_MODULE_ID 0x39022000 00367 /* DP83848C PHY Registers */ 00368 #define EMAC_PHY_REG_BMCR 0x00 00369 #define EMAC_PHY_REG_BMSR 0x01 00370 #define EMAC_PHY_REG_IDR1 0x02 00371 #define EMAC_PHY_REG_IDR2 0x03 00372 #define EMAC_PHY_REG_ANAR 0x04 00373 #define EMAC_PHY_REG_ANLPAR 0x05 00374 #define EMAC_PHY_REG_ANER 0x06 00375 #define EMAC_PHY_REG_ANNPTR 0x07 00376 #define EMAC_PHY_REG_LPNPA 0x08 00377 00378 00379 /* PHY Extended Registers */ 00380 #define EMAC_PHY_REG_STS 0x10 00381 #define EMAC_PHY_REG_MICR 0x11 00382 #define EMAC_PHY_REG_MISR 0x12 00383 #define EMAC_PHY_REG_FCSCR 0x14 00384 #define EMAC_PHY_REG_RECR 0x15 00385 #define EMAC_PHY_REG_PCSR 0x16 00386 #define EMAC_PHY_REG_RBR 0x17 00387 #define EMAC_PHY_REG_LEDCR 0x18 00388 #define EMAC_PHY_REG_PHYCR 0x19 00389 #define EMAC_PHY_REG_10BTSCR 0x1A 00390 #define EMAC_PHY_REG_CDCTRL1 0x1B 00391 #define EMAC_PHY_REG_EDCR 0x1D 00394 /* PHY Basic Mode Control Register (BMCR) bitmap definitions */ 00395 #define EMAC_PHY_BMCR_RESET (1<<15) 00396 #define EMAC_PHY_BMCR_LOOPBACK (1<<14) 00397 #define EMAC_PHY_BMCR_SPEED_SEL (1<<13) 00398 #define EMAC_PHY_BMCR_AN (1<<12) 00399 #define EMAC_PHY_BMCR_POWERDOWN (1<<11) 00400 #define EMAC_PHY_BMCR_ISOLATE (1<<10) 00401 #define EMAC_PHY_BMCR_RE_AN (1<<9) 00402 #define EMAC_PHY_BMCR_DUPLEX (1<<8) 00404 /* PHY Basic Mode Status Status Register (BMSR) bitmap definitions */ 00405 #define EMAC_PHY_BMSR_100BE_T4 (1<<15) 00406 #define EMAC_PHY_BMSR_100TX_FULL (1<<14) 00407 #define EMAC_PHY_BMSR_100TX_HALF (1<<13) 00408 #define EMAC_PHY_BMSR_10BE_FULL (1<<12) 00409 #define EMAC_PHY_BMSR_10BE_HALF (1<<11) 00410 #define EMAC_PHY_BMSR_NOPREAM (1<<6) 00411 #define EMAC_PHY_BMSR_AUTO_DONE (1<<5) 00412 #define EMAC_PHY_BMSR_REMOTE_FAULT (1<<4) 00413 #define EMAC_PHY_BMSR_NO_AUTO (1<<3) 00414 #define EMAC_PHY_BMSR_LINK_ESTABLISHED (1<<2) 00416 /* PHY Status Register bitmap definitions */ 00417 #define EMAC_PHY_SR_REMOTE_FAULT (1<<6) 00418 #define EMAC_PHY_SR_JABBER (1<<5) 00419 #define EMAC_PHY_SR_AUTO_DONE (1<<4) 00420 #define EMAC_PHY_SR_LOOPBACK (1<<3) 00421 #define EMAC_PHY_SR_DUP (1<<2) 00422 #define EMAC_PHY_SR_SPEED (1<<1) 00423 #define EMAC_PHY_SR_LINK (1<<0) 00426 #define EMAC_PHY_FULLD_100M 0x2100 00427 #define EMAC_PHY_HALFD_100M 0x2000 00428 #define EMAC_PHY_FULLD_10M 0x0100 00429 #define EMAC_PHY_HALFD_10M 0x0000 00430 #define EMAC_PHY_AUTO_NEG 0x3000 00432 #define EMAC_DP83848C_DEF_ADR 0x0100 00433 #define EMAC_DP83848C_ID 0x20005C90 00445 /* Public Types --------------------------------------------------------------- */ 00446 00450 /* Descriptor and status formats ---------------------------------------------- */ 00451 00455 typedef struct { 00456 uint32_t Packet; 00457 uint32_t Ctrl; 00458 } RX_Desc; 00459 00463 typedef struct { 00464 uint32_t Info; 00465 uint32_t HashCRC; 00466 } RX_Stat; 00467 00471 typedef struct { 00472 uint32_t Packet; 00473 uint32_t Ctrl; 00474 } TX_Desc; 00475 00479 typedef struct { 00480 uint32_t Info; 00481 } TX_Stat; 00482 00483 00487 typedef struct { 00488 uint32_t ulDataLen; 00489 uint32_t *pbDataBuf; 00490 } EMAC_PACKETBUF_Type; 00491 00495 typedef struct { 00496 uint32_t Mode; 00503 uint8_t *pbEMAC_Addr; 00506 } EMAC_CFG_Type; 00507 00509 typedef void (EMAC_IntCBSType)(void); 00510 00511 00517 /* Public Macros -------------------------------------------------------------- */ 00523 /* EMAC PHY status type definitions */ 00524 #define EMAC_PHY_STAT_LINK (0) 00525 #define EMAC_PHY_STAT_SPEED (1) 00526 #define EMAC_PHY_STAT_DUP (2) 00528 /* EMAC PHY device Speed definitions */ 00529 #define EMAC_MODE_AUTO (0) 00530 #define EMAC_MODE_10M_FULL (1) 00531 #define EMAC_MODE_10M_HALF (2) 00532 #define EMAC_MODE_100M_FULL (3) 00533 #define EMAC_MODE_100M_HALF (4) 00540 /* Public Functions ----------------------------------------------------------- */ 00541 00545 Status EMAC_Init(EMAC_CFG_Type *EMAC_ConfigStruct); 00546 void EMAC_DeInit(void); 00547 int32_t EMAC_CheckPHYStatus(uint32_t ulPHYState); 00548 int32_t EMAC_SetPHYMode(uint32_t ulPHYMode); 00549 int32_t EMAC_UpdatePHYStatus(void); 00550 void EMAC_SetHashFilter(uint8_t dstMAC_addr[], FunctionalState NewState); 00551 int32_t EMAC_CRCCalc(uint8_t frame_no_fcs[], int32_t frame_len); 00552 void EMAC_SetFilterMode(uint32_t ulFilterMode, FunctionalState NewState); 00553 FlagStatus EMAC_GetWoLStatus(uint32_t ulWoLMode); 00554 void EMAC_WritePacketBuffer(EMAC_PACKETBUF_Type *pDataStruct); 00555 void EMAC_ReadPacketBuffer(EMAC_PACKETBUF_Type *pDataStruct); 00556 void EMAC_StandardIRQHandler(void); 00557 void EMAC_SetupIntCBS(uint32_t ulIntType, EMAC_IntCBSType *pfnIntCb); 00558 void EMAC_IntCmd(uint32_t ulIntType, FunctionalState NewState); 00559 IntStatus EMAC_IntGetStatus(uint32_t ulIntType); 00560 Bool EMAC_CheckReceiveIndex(void); 00561 Bool EMAC_CheckTransmitIndex(void); 00562 FlagStatus EMAC_CheckReceiveDataStatus(uint32_t ulRxStatType); 00563 uint32_t EMAC_GetReceiveDataSize(void); 00564 void EMAC_UpdateRxConsumeIndex(void); 00565 void EMAC_UpdateTxProduceIndex(void); 00566 00567 00572 #ifdef __cplusplus 00573 } 00574 #endif 00575 00576 #endif /* LPC17XX_EMAC_H_ */ 00577 00582 /* --------------------------------- End Of File ------------------------------ */
Generated on Mon Feb 8 10:01:36 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
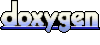