C:/nxpdrv/LPC1700CMSIS/Drivers/include/lpc17xx_spi.h
Go to the documentation of this file.00001 /***********************************************************************/ 00021 /* Peripheral group ----------------------------------------------------------- */ 00027 #ifndef LPC17XX_SPI_H_ 00028 #define LPC17XX_SPI_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "LPC17xx.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 /* Private Macros ------------------------------------------------------------- */ 00045 /*********************************************************************/ 00054 #define SPI_SPCR_BIT_EN ((uint32_t)(1<<2)) 00055 00056 #define SPI_SPCR_CPHA_SECOND ((uint32_t)(1<<3)) 00057 00058 #define SPI_SPCR_CPOL_LOW ((uint32_t)(1<<4)) 00059 00060 #define SPI_SPCR_MSTR ((uint32_t)(1<<5)) 00061 00062 #define SPI_SPCR_LSBF ((uint32_t)(1<<6)) 00063 00064 #define SPI_SPCR_SPIE ((uint32_t)(1<<7)) 00065 00067 #define SPI_SPCR_BITS(n) ((n==0) ? ((uint32_t)0) : ((uint32_t)((n&0x0F)<<8))) 00068 00069 #define SPI_SPCR_BITMASK ((uint32_t)(0xFFC)) 00070 00071 00072 /*********************************************************************/ 00076 #define SPI_SPSR_ABRT ((uint32_t)(1<<3)) 00077 00078 #define SPI_SPSR_MODF ((uint32_t)(1<<4)) 00079 00080 #define SPI_SPSR_ROVR ((uint32_t)(1<<5)) 00081 00082 #define SPI_SPSR_WCOL ((uint32_t)(1<<6)) 00083 00084 #define SPI_SPSR_SPIF ((uint32_t)(1<<7)) 00085 00086 #define SPI_SPSR_BITMASK ((uint32_t)(0xF8)) 00087 00088 00089 /*********************************************************************/ 00093 #define SPI_SPDR_LO_MASK ((uint32_t)(0xFF)) 00094 00095 #define SPI_SPDR_HI_MASK ((uint32_t)(0xFF00)) 00096 00097 #define SPI_SPDR_BITMASK ((uint32_t)(0xFFFF)) 00098 00099 00100 /*********************************************************************/ 00104 #define SPI_SPCCR_COUNTER(n) ((uint32_t)(n&0xFF)) 00105 00106 #define SPI_SPCCR_BITMASK ((uint32_t)(0xFF)) 00107 00108 00109 /*********************************************************************** 00110 * Macro defines for SPI Test Control Register 00111 **********************************************************************/ 00113 #define SPI_SPTCR_TEST_MASK ((uint32_t)(0xFE)) 00114 00115 #define SPI_SPTCR_BITMASK ((uint32_t)(0xFE)) 00116 00117 00118 00119 /*********************************************************************/ 00123 #define SPI_SPTSR_ABRT ((uint32_t)(1<<3)) 00124 00125 #define SPI_SPTSR_MODF ((uint32_t)(1<<4)) 00126 00127 #define SPI_SPTSR_ROVR ((uint32_t)(1<<5)) 00128 00129 #define SPI_SPTSR_WCOL ((uint32_t)(1<<6)) 00130 00131 #define SPI_SPTSR_SPIF ((uint32_t)(1<<7)) 00132 00133 #define SPI_SPTSR_MASKBIT ((uint32_t)(0xF8)) 00134 00135 00136 00137 /*********************************************************************/ 00141 #define SPI_SPINT_INTFLAG ((uint32_t)(1<<0)) 00142 00143 #define SPI_SPINT_BITMASK ((uint32_t)(0x01)) 00144 00154 /* Public Types --------------------------------------------------------------- */ 00160 typedef struct { 00161 uint32_t Databit; 00163 uint32_t CPHA; 00166 uint32_t CPOL; 00169 uint32_t Mode; 00172 uint32_t DataOrder; 00175 uint32_t ClockRate; 00177 } SPI_CFG_Type; 00178 00179 00183 typedef enum { 00184 SPI_TRANSFER_POLLING = 0, 00185 SPI_TRANSFER_INTERRUPT 00186 } SPI_TRANSFER_Type; 00187 00191 typedef struct { 00192 void *tx_data; 00193 void *rx_data; 00194 uint32_t length; 00195 uint32_t counter; 00196 uint32_t status; 00197 void (*callback)(void); 00199 } SPI_DATA_SETUP_Type; 00200 00206 /* Public Macros -------------------------------------------------------------- */ 00212 #define PARAM_SPIx(n) (((uint32_t *)n)==((uint32_t *)LPC_SPI)) 00213 00214 /*********************************************************************/ 00218 #define SPI_CPHA_FIRST ((uint32_t)(0)) 00219 #define SPI_CPHA_SECOND SPI_SPCR_CPHA_SECOND 00220 #define PARAM_SPI_CPHA(n) ((n==SPI_CPHA_FIRST) || (n==SPI_CPHA_SECOND)) 00221 00223 #define SPI_CPOL_HI ((uint32_t)(0)) 00224 #define SPI_CPOL_LO SPI_SPCR_CPOL_LOW 00225 #define PARAM_SPI_CPOL(n) ((n==SPI_CPOL_HI) || (n==SPI_CPOL_LO)) 00226 00228 #define SPI_SLAVE_MODE ((uint32_t)(0)) 00229 #define SPI_MASTER_MODE SPI_SPCR_MSTR 00230 #define PARAM_SPI_MODE(n) ((n==SPI_SLAVE_MODE) || (n==SPI_MASTER_MODE)) 00231 00233 #define SPI_DATA_MSB_FIRST ((uint32_t)(0)) 00234 #define SPI_DATA_LSB_FIRST SPI_SPCR_LSBF 00235 #define PARAM_SPI_DATA_ORDER(n) ((n==SPI_DATA_MSB_FIRST) || (n==SPI_DATA_LSB_FIRST)) 00236 00238 #define SPI_DATABIT_16 SPI_SPCR_BITS(0) 00239 #define SPI_DATABIT_8 SPI_SPCR_BITS(0x08) 00240 #define SPI_DATABIT_9 SPI_SPCR_BITS(0x09) 00241 #define SPI_DATABIT_10 SPI_SPCR_BITS(0x0A) 00242 #define SPI_DATABIT_11 SPI_SPCR_BITS(0x0B) 00243 #define SPI_DATABIT_12 SPI_SPCR_BITS(0x0C) 00244 #define SPI_DATABIT_13 SPI_SPCR_BITS(0x0D) 00245 #define SPI_DATABIT_14 SPI_SPCR_BITS(0x0E) 00246 #define SPI_DATABIT_15 SPI_SPCR_BITS(0x0F) 00247 #define PARAM_SPI_DATABIT(n) ((n==SPI_DATABIT_16) || (n==SPI_DATABIT_8) \ 00248 || (n==SPI_DATABIT_9) || (n==SPI_DATABIT_10) \ 00249 || (n==SPI_DATABIT_11) || (n==SPI_DATABIT_12) \ 00250 || (n==SPI_DATABIT_13) || (n==SPI_DATABIT_14) \ 00251 || (n==SPI_DATABIT_15)) 00252 00253 00254 /*********************************************************************/ 00258 #define SPI_STAT_ABRT SPI_SPSR_ABRT 00259 00260 #define SPI_STAT_MODF SPI_SPSR_MODF 00261 00262 #define SPI_STAT_ROVR SPI_SPSR_ROVR 00263 00264 #define SPI_STAT_WCOL SPI_SPSR_WCOL 00265 00266 #define SPI_STAT_SPIF SPI_SPSR_SPIF 00267 #define PARAM_SPI_STAT(n) ((n==SPI_STAT_ABRT) || (n==SPI_STAT_MODF) \ 00268 || (n==SPI_STAT_ROVR) || (n==SPI_STAT_WCOL) \ 00269 || (n==SPI_STAT_SPIF)) 00270 00271 00272 /* SPI Status Implementation definitions */ 00273 #define SPI_STAT_DONE (1UL<<8) 00274 #define SPI_STAT_ERROR (1UL<<9) 00281 /* Public Functions ----------------------------------------------------------- */ 00282 00286 void SPI_SetClock (LPC_SPI_TypeDef *SPIx, uint32_t target_clock); 00287 void SPI_DeInit(LPC_SPI_TypeDef *SPIx); 00288 void SPI_Init(LPC_SPI_TypeDef *SPIx, SPI_CFG_Type *SPI_ConfigStruct); 00289 void SPI_ConfigStructInit(SPI_CFG_Type *SPI_InitStruct); 00290 void SPI_SendData(LPC_SPI_TypeDef *SPIx, uint16_t Data); 00291 uint16_t SPI_ReceiveData(LPC_SPI_TypeDef *SPIx); 00292 int32_t SPI_ReadWrite (LPC_SPI_TypeDef *SPIx, SPI_DATA_SETUP_Type *dataCfg, SPI_TRANSFER_Type xfType); 00293 void SPI_IntCmd(LPC_SPI_TypeDef *SPIx, FunctionalState NewState); 00294 IntStatus SPI_GetIntStatus (LPC_SPI_TypeDef *SPIx); 00295 void SPI_ClearIntPending(LPC_SPI_TypeDef *SPIx); 00296 uint32_t SPI_GetStatus(LPC_SPI_TypeDef *SPIx); 00297 FlagStatus SPI_CheckStatus (uint32_t inputSPIStatus, uint8_t SPIStatus); 00298 void SPI_StdIntHandler(void); 00299 00304 #ifdef __cplusplus 00305 } 00306 #endif 00307 00308 #endif /* LPC17XX_SPI_H_ */ 00309 00314 /* --------------------------------- End Of File ------------------------------ */
Generated on Mon Feb 8 10:01:37 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
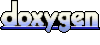