C:/nxpdrv/LPC1700CMSIS/Drivers/include/lpc17xx_mcpwm.h
Go to the documentation of this file.00001 /***********************************************************************/ 00021 /* Peripheral group ----------------------------------------------------------- */ 00027 #ifndef LPC17XX_MCPWM_H_ 00028 #define LPC17XX_MCPWM_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "LPC17xx.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 00041 /* Private Macros ------------------------------------------------------------- */ 00050 /* MCPWM Control register, these macro definitions below can be applied for these 00051 * register type: 00052 * - MCPWM Control read address 00053 * - MCPWM Control set address 00054 * - MCPWM Control clear address 00055 */ 00056 #define MCPWM_CON_RUN(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<((n*8)+0))) : (0)) 00057 #define MCPWM_CON_CENTER(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<((n*8)+1))) : (0)) 00058 #define MCPWM_CON_POLAR(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<((n*8)+2))) : (0)) 00059 #define MCPWM_CON_DTE(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<((n*8)+3))) : (0)) 00060 #define MCPWM_CON_DISUP(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<((n*8)+4))) : (0)) 00061 #define MCPWM_CON_INVBDC ((uint32_t)(1<<29)) 00062 #define MCPWM_CON_ACMODE ((uint32_t)(1<<30)) 00063 #define MCPWM_CON_DCMODE ((uint32_t)(1<<31)) 00065 /* Capture Control register, these macro definitions below can be applied for these 00066 * register type: 00067 * - MCPWM Capture Control read address 00068 * - MCPWM Capture Control set address 00069 * - MCPWM Capture control clear address 00070 */ 00071 00072 #define MCPWM_CAPCON_CAPMCI_RE(cap,mci) (((cap>=0)&&(cap<=2)&&(mci>=0)&&(mci<=2)) ? ((uint32_t)(1<<((cap*6)+(mci*2)+0))) : (0)) 00073 00074 #define MCPWM_CAPCON_CAPMCI_FE(cap,mci) (((cap>=0)&&(cap<=2)&&(mci>=0)&&(mci<=2)) ? ((uint32_t)(1<<((cap*6)+(mci*2)+1))) : (0)) 00075 00076 #define MCPWM_CAPCON_RT(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<(18+(n)))) : (0)) 00077 00078 #define MCPWM_CAPCON_HNFCAP(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<(21+(n)))) : (0)) 00079 00080 /* Interrupt registers, these macro definitions below can be applied for these 00081 * register type: 00082 * - MCPWM Interrupt Enable read address 00083 * - MCPWM Interrupt Enable set address 00084 * - MCPWM Interrupt Enable clear address 00085 * - MCPWM Interrupt Flags read address 00086 * - MCPWM Interrupt Flags set address 00087 * - MCPWM Interrupt Flags clear address 00088 */ 00090 #define MCPWM_INT_ILIM(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<((n*4)+0))) : (0)) 00091 00092 #define MCPWM_INT_IMAT(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<((n*4)+1))) : (0)) 00093 00094 #define MCPWM_INT_ICAP(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<((n*4)+2))) : (0)) 00095 00096 #define MCPWM_INT_ABORT ((uint32_t)(1<<15)) 00097 00098 /* MCPWM Count Control register, these macro definitions below can be applied for these 00099 * register type: 00100 * - MCPWM Count Control read address 00101 * - MCPWM Count Control set address 00102 * - MCPWM Count Control clear address 00103 */ 00105 #define MCPWM_CNTCON_TCMCI_RE(tc,mci) (((tc>=0)&&(tc<=2)&&(mci>=0)&&(mci<=2)) ? ((uint32_t)(1<<((6*tc)+(2*mci)+0))) : (0)) 00106 00107 #define MCPWM_CNTCON_TCMCI_FE(tc,mci) (((tc>=0)&&(tc<=2)&&(mci>=0)&&(mci<=2)) ? ((uint32_t)(1<<((6*tc)+(2*mci)+1))) : (0)) 00108 00109 #define MCPWM_CNTCON_CNTR(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<(29+n))) : (0)) 00110 00111 /* MCPWM Timer/Counter 0-2 registers --------------------------------------------------- */ 00112 /* MCPWM Limit 0-2 registers ----------------------------------------------------------- */ 00113 /* MCPWM Match 0-2 registers ----------------------------------------------------------- */ 00114 00115 /* MCPWM Dead-time register ------------------------------------------------------------ */ 00117 #define MCPWM_DT(n,x) (((n>=0)&&(n<=2)) ? ((uint32_t)((x&0x3FF)<<(n*10))) : (0)) 00118 00119 /* MCPWM Communication Pattern register ------------------------------------------------ */ 00120 #define MCPWM_CP_A0 ((uint32_t)(1<<0)) 00121 #define MCPWM_CP_B0 ((uint32_t)(1<<1)) 00122 #define MCPWM_CP_A1 ((uint32_t)(1<<2)) 00123 #define MCPWM_CP_B1 ((uint32_t)(1<<3)) 00124 #define MCPWM_CP_A2 ((uint32_t)(1<<4)) 00125 #define MCPWM_CP_B2 ((uint32_t)(1<<5)) 00127 /* MCPWM Capture Registers ------------------------------------------------------------- */ 00128 /* MCPWM Capture read addresses */ 00129 00130 /* MCPWM Capture clear address --------------------------------------------------------- */ 00132 #define MCPWM_CAPCLR_CAP(n) (((n>=0)&&(n<=2)) ? ((uint32_t)(1<<n)) : (0)) 00133 00134 00144 /* Public Types --------------------------------------------------------------- */ 00152 typedef struct { 00153 uint32_t channelType; 00158 uint32_t channelPolarity; 00162 uint32_t channelDeadtimeEnable; 00166 uint32_t channelDeadtimeValue; 00167 uint32_t channelUpdateEnable; 00172 uint32_t channelTimercounterValue; 00173 uint32_t channelPeriodValue; 00174 uint32_t channelPulsewidthValue; 00175 } MCPWM_CHANNEL_CFG_Type; 00176 00180 typedef struct { 00181 uint32_t captureChannel; 00182 uint32_t captureRising; 00186 uint32_t captureFalling; 00190 uint32_t timerReset; 00194 uint32_t hnfEnable; 00198 } MCPWM_CAPTURE_CFG_Type; 00199 00200 00204 typedef struct { 00205 uint32_t counterChannel; 00206 uint32_t countRising; 00210 uint32_t countFalling; 00214 } MCPWM_COUNT_CFG_Type; 00215 00221 /* Public Macros -------------------------------------------------------------- */ 00228 #define MCPWM_CHANNEL_EDGE_MODE ((uint32_t)(0)) 00229 00230 #define MCPWM_CHANNEL_CENTER_MODE ((uint32_t)(1)) 00231 00233 #define MCPWM_CHANNEL_PASSIVE_LO ((uint32_t)(0)) 00234 00235 #define MCPWM_CHANNEL_PASSIVE_HI ((uint32_t)(1)) 00236 00237 /* Output Patent in 3-phase DC mode, the internal MCOA0 signal is routed to any or all of 00238 * the six output pins under the control of the bits in this register */ 00239 #define MCPWM_PATENT_A0 ((uint32_t)(1<<0)) 00240 #define MCPWM_PATENT_B0 ((uint32_t)(1<<1)) 00241 #define MCPWM_PATENT_A1 ((uint32_t)(1<<2)) 00242 #define MCPWM_PATENT_B1 ((uint32_t)(1<<3)) 00243 #define MCPWM_PATENT_A2 ((uint32_t)(1<<4)) 00244 #define MCPWM_PATENT_B2 ((uint32_t)(1<<5)) 00246 /* Interrupt type in MCPWM */ 00247 00248 #define MCPWM_INTFLAG_LIM0 MCPWM_INT_ILIM(0) 00249 00250 #define MCPWM_INTFLAG_MAT0 MCPWM_INT_IMAT(0) 00251 00252 #define MCPWM_INTFLAG_CAP0 MCPWM_INT_ICAP(0) 00253 00255 #define MCPWM_INTFLAG_LIM1 MCPWM_INT_ILIM(1) 00256 00257 #define MCPWM_INTFLAG_MAT1 MCPWM_INT_IMAT(1) 00258 00259 #define MCPWM_INTFLAG_CAP1 MCPWM_INT_ICAP(1) 00260 00262 #define MCPWM_INTFLAG_LIM2 MCPWM_INT_ILIM(2) 00263 00264 #define MCPWM_INTFLAG_MAT2 MCPWM_INT_IMAT(2) 00265 00266 #define MCPWM_INTFLAG_CAP2 MCPWM_INT_ICAP(2) 00267 00269 #define MCPWM_INTFLAG_ABORT MCPWM_INT_ABORT 00270 00271 00277 /* Public Functions ----------------------------------------------------------- */ 00282 void MCPWM_Init(LPC_MCPWM_TypeDef *MCPWMx); 00283 void MCPWM_ConfigChannel(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00284 MCPWM_CHANNEL_CFG_Type * channelSetup); 00285 void MCPWM_WriteToShadow(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00286 MCPWM_CHANNEL_CFG_Type *channelSetup); 00287 void MCPWM_ConfigCapture(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00288 MCPWM_CAPTURE_CFG_Type *captureConfig); 00289 void MCPWM_ClearCapture(LPC_MCPWM_TypeDef *MCPWMx, uint32_t captureChannel); 00290 uint32_t MCPWM_GetCapture(LPC_MCPWM_TypeDef *MCPWMx, uint32_t captureChannel); 00291 void MCPWM_CountConfig(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00292 uint32_t countMode, MCPWM_COUNT_CFG_Type *countConfig); 00293 void MCPWM_Start(LPC_MCPWM_TypeDef *MCPWMx,uint32_t channel0, uint32_t channel1, uint32_t channel2); 00294 void MCPWM_Stop(LPC_MCPWM_TypeDef *MCPWMx,uint32_t channel0, uint32_t channel1, uint32_t channel2); 00295 void MCPWM_ACMode(LPC_MCPWM_TypeDef *MCPWMx,uint32_t acMode); 00296 void MCPWM_DCMode(LPC_MCPWM_TypeDef *MCPWMx, uint32_t dcMode, 00297 uint32_t outputInvered, uint32_t outputPattern); 00298 void MCPWM_IntConfig(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType, FunctionalState NewState); 00299 void MCPWM_IntSet(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType); 00300 void MCPWM_IntClear(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType); 00301 FlagStatus MCPWM_GetIntStatus(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType); 00302 00307 #ifdef __cplusplus 00308 } 00309 #endif 00310 00311 #endif /* LPC17XX_MCPWM_H_ */ 00312 00317 /* --------------------------------- End Of File ------------------------------ */
Generated on Mon Feb 8 10:01:37 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
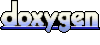