C:/nxpdrv/LPC1700CMSIS/Drivers/source/lpc17xx_mcpwm.c
Go to the documentation of this file.00001 00021 /* Peripheral group ----------------------------------------------------------- */ 00026 /* Includes ------------------------------------------------------------------- */ 00027 #include "lpc17xx_mcpwm.h" 00028 #include "lpc17xx_clkpwr.h" 00029 00030 /* If this source file built with example, the LPC17xx FW library configuration 00031 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00032 * otherwise the default FW library configuration file must be included instead 00033 */ 00034 #ifdef __BUILD_WITH_EXAMPLE__ 00035 #include "lpc17xx_libcfg.h" 00036 #else 00037 #include "lpc17xx_libcfg_default.h" 00038 #endif /* __BUILD_WITH_EXAMPLE__ */ 00039 00040 00041 #ifdef _MCPWM 00042 00043 /* Public Functions ----------------------------------------------------------- */ 00048 /*********************************************************************/ 00053 void MCPWM_Init(LPC_MCPWM_TypeDef *MCPWMx) 00054 { 00055 00056 /* Turn On MCPWM PCLK */ 00057 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCMC, ENABLE); 00058 /* As default, peripheral clock for MCPWM module 00059 * is set to FCCLK / 2 */ 00060 // CLKPWR_SetPCLKDiv(CLKPWR_PCLKSEL_MC, CLKPWR_PCLKSEL_CCLK_DIV_2); 00061 00062 MCPWMx->MCCAP_CLR = MCPWM_CAPCLR_CAP(0) | MCPWM_CAPCLR_CAP(1) | MCPWM_CAPCLR_CAP(2); 00063 MCPWMx->MCINTFLAG_CLR = MCPWM_INT_ILIM(0) | MCPWM_INT_ILIM(1) | MCPWM_INT_ILIM(2) \ 00064 | MCPWM_INT_IMAT(0) | MCPWM_INT_IMAT(1) | MCPWM_INT_IMAT(2) \ 00065 | MCPWM_INT_ICAP(0) | MCPWM_INT_ICAP(1) | MCPWM_INT_ICAP(2); 00066 MCPWMx->MCINTEN_CLR = MCPWM_INT_ILIM(0) | MCPWM_INT_ILIM(1) | MCPWM_INT_ILIM(2) \ 00067 | MCPWM_INT_IMAT(0) | MCPWM_INT_IMAT(1) | MCPWM_INT_IMAT(2) \ 00068 | MCPWM_INT_ICAP(0) | MCPWM_INT_ICAP(1) | MCPWM_INT_ICAP(2); 00069 } 00070 00071 00072 /*********************************************************************/ 00082 void MCPWM_ConfigChannel(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00083 MCPWM_CHANNEL_CFG_Type * channelSetup) 00084 { 00085 if ((channelNum >= 0) && (channelNum <= 2)) { 00086 if (channelNum == 0) { 00087 MCPWMx->MCTIM0 = channelSetup->channelTimercounterValue; 00088 MCPWMx->MCPER0 = channelSetup->channelPeriodValue; 00089 MCPWMx->MCPW0 = channelSetup->channelPulsewidthValue; 00090 } else if (channelNum == 1) { 00091 MCPWMx->MCTIM1 = channelSetup->channelTimercounterValue; 00092 MCPWMx->MCPER1 = channelSetup->channelPeriodValue; 00093 MCPWMx->MCPW1 = channelSetup->channelPulsewidthValue; 00094 } else if (channelNum == 2) { 00095 MCPWMx->MCTIM2 = channelSetup->channelTimercounterValue; 00096 MCPWMx->MCPER2 = channelSetup->channelPeriodValue; 00097 MCPWMx->MCPW2 = channelSetup->channelPulsewidthValue; 00098 } else { 00099 return; 00100 } 00101 00102 if (channelSetup->channelType /* == MCPWM_CHANNEL_CENTER_MODE */){ 00103 MCPWMx->MCCON_SET = MCPWM_CON_CENTER(channelNum); 00104 } else { 00105 MCPWMx->MCCON_CLR = MCPWM_CON_CENTER(channelNum); 00106 } 00107 00108 if (channelSetup->channelPolarity /* == MCPWM_CHANNEL_PASSIVE_HI */){ 00109 MCPWMx->MCCON_SET = MCPWM_CON_POLAR(channelNum); 00110 } else { 00111 MCPWMx->MCCON_CLR = MCPWM_CON_POLAR(channelNum); 00112 } 00113 00114 if (channelSetup->channelDeadtimeEnable /* == ENABLE */){ 00115 MCPWMx->MCCON_SET = MCPWM_CON_DTE(channelNum); 00116 MCPWMx->MCDEADTIME &= ~(MCPWM_DT(channelNum, 0x3FF)); 00117 MCPWMx->MCDEADTIME |= MCPWM_DT(channelNum, channelSetup->channelDeadtimeValue); 00118 } else { 00119 MCPWMx->MCCON_CLR = MCPWM_CON_DTE(channelNum); 00120 } 00121 00122 if (channelSetup->channelUpdateEnable /* == ENABLE */){ 00123 MCPWMx->MCCON_CLR = MCPWM_CON_DISUP(channelNum); 00124 } else { 00125 MCPWMx->MCCON_SET = MCPWM_CON_DISUP(channelNum); 00126 } 00127 } 00128 } 00129 00130 00131 /*********************************************************************/ 00141 void MCPWM_WriteToShadow(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00142 MCPWM_CHANNEL_CFG_Type *channelSetup) 00143 { 00144 if (channelNum == 0){ 00145 MCPWMx->MCPER0 = channelSetup->channelPeriodValue; 00146 MCPWMx->MCPW0 = channelSetup->channelPulsewidthValue; 00147 } else if (channelNum == 1) { 00148 MCPWMx->MCPER1 = channelSetup->channelPeriodValue; 00149 MCPWMx->MCPW1 = channelSetup->channelPulsewidthValue; 00150 } else if (channelNum == 2) { 00151 MCPWMx->MCPER2 = channelSetup->channelPeriodValue; 00152 MCPWMx->MCPW2 = channelSetup->channelPulsewidthValue; 00153 } 00154 } 00155 00156 00157 00158 /*********************************************************************/ 00167 void MCPWM_ConfigCapture(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00168 MCPWM_CAPTURE_CFG_Type *captureConfig) 00169 { 00170 if ((channelNum >= 0) && (channelNum <= 2)) { 00171 00172 if (captureConfig->captureFalling /* == ENABLE */) { 00173 MCPWMx->MCCAPCON_SET = MCPWM_CAPCON_CAPMCI_FE(captureConfig->captureChannel, channelNum); 00174 } else { 00175 MCPWMx->MCCAPCON_CLR = MCPWM_CAPCON_CAPMCI_FE(captureConfig->captureChannel, channelNum); 00176 } 00177 00178 if (captureConfig->captureRising /* == ENABLE */) { 00179 MCPWMx->MCCAPCON_SET = MCPWM_CAPCON_CAPMCI_RE(captureConfig->captureChannel, channelNum); 00180 } else { 00181 MCPWMx->MCCAPCON_CLR = MCPWM_CAPCON_CAPMCI_RE(captureConfig->captureChannel, channelNum); 00182 } 00183 00184 if (captureConfig->timerReset /* == ENABLE */){ 00185 MCPWMx->MCCAPCON_SET = MCPWM_CAPCON_RT(captureConfig->captureChannel); 00186 } else { 00187 MCPWMx->MCCAPCON_CLR = MCPWM_CAPCON_RT(captureConfig->captureChannel); 00188 } 00189 00190 if (captureConfig->hnfEnable /* == ENABLE */){ 00191 MCPWMx->MCCAPCON_SET = MCPWM_CAPCON_HNFCAP(channelNum); 00192 } else { 00193 MCPWMx->MCCAPCON_CLR = MCPWM_CAPCON_HNFCAP(channelNum); 00194 } 00195 } 00196 } 00197 00198 00199 /*********************************************************************/ 00205 void MCPWM_ClearCapture(LPC_MCPWM_TypeDef *MCPWMx, uint32_t captureChannel) 00206 { 00207 MCPWMx->MCCAP_CLR = MCPWM_CAPCLR_CAP(captureChannel); 00208 } 00209 00210 /*********************************************************************/ 00216 uint32_t MCPWM_GetCapture(LPC_MCPWM_TypeDef *MCPWMx, uint32_t captureChannel) 00217 { 00218 if (captureChannel == 0){ 00219 return (MCPWMx->MCCR0); 00220 } else if (captureChannel == 1) { 00221 return (MCPWMx->MCCR1); 00222 } else if (captureChannel == 2) { 00223 return (MCPWMx->MCCR2); 00224 } 00225 return (0); 00226 } 00227 00228 00229 /*********************************************************************/ 00241 void MCPWM_CountConfig(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00242 uint32_t countMode, MCPWM_COUNT_CFG_Type *countConfig) 00243 { 00244 if ((channelNum >= 0) && (channelNum <= 2)) { 00245 if (countMode /* == ENABLE */){ 00246 MCPWMx->MCCNTCON_SET = MCPWM_CNTCON_CNTR(channelNum); 00247 if (countConfig->countFalling /* == ENABLE */) { 00248 MCPWMx->MCCNTCON_SET = MCPWM_CNTCON_TCMCI_FE(countConfig->counterChannel,channelNum); 00249 } else { 00250 MCPWMx->MCCNTCON_CLR = MCPWM_CNTCON_TCMCI_FE(countConfig->counterChannel,channelNum); 00251 } 00252 if (countConfig->countRising /* == ENABLE */) { 00253 MCPWMx->MCCNTCON_SET = MCPWM_CNTCON_TCMCI_RE(countConfig->counterChannel,channelNum); 00254 } else { 00255 MCPWMx->MCCNTCON_CLR = MCPWM_CNTCON_TCMCI_RE(countConfig->counterChannel,channelNum); 00256 } 00257 } else { 00258 MCPWMx->MCCNTCON_CLR = MCPWM_CNTCON_CNTR(channelNum); 00259 } 00260 } 00261 } 00262 00263 00264 /*********************************************************************/ 00278 void MCPWM_Start(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channel0, 00279 uint32_t channel1, uint32_t channel2) 00280 { 00281 uint32_t regVal = 0; 00282 regVal = (channel0 ? MCPWM_CON_RUN(0) : 0) | (channel1 ? MCPWM_CON_RUN(1) : 0) \ 00283 | (channel2 ? MCPWM_CON_RUN(2) : 0); 00284 MCPWMx->MCCON_SET = regVal; 00285 } 00286 00287 00288 /*********************************************************************/ 00302 void MCPWM_Stop(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channel0, 00303 uint32_t channel1, uint32_t channel2) 00304 { 00305 uint32_t regVal = 0; 00306 regVal = (channel0 ? MCPWM_CON_RUN(0) : 0) | (channel1 ? MCPWM_CON_RUN(1) : 0) \ 00307 | (channel2 ? MCPWM_CON_RUN(2) : 0); 00308 MCPWMx->MCCON_CLR = regVal; 00309 } 00310 00311 00312 /*********************************************************************/ 00320 void MCPWM_ACMode(LPC_MCPWM_TypeDef *MCPWMx, uint32_t acMode) 00321 { 00322 if (acMode){ 00323 MCPWMx->MCCON_SET = MCPWM_CON_ACMODE; 00324 } else { 00325 MCPWMx->MCCON_CLR = MCPWM_CON_ACMODE; 00326 } 00327 } 00328 00329 00330 /*********************************************************************/ 00354 void MCPWM_DCMode(LPC_MCPWM_TypeDef *MCPWMx, uint32_t dcMode, 00355 uint32_t outputInvered, uint32_t outputPattern) 00356 { 00357 if (dcMode){ 00358 MCPWMx->MCCON_SET = MCPWM_CON_DCMODE; 00359 } else { 00360 MCPWMx->MCCON_CLR = MCPWM_CON_DCMODE; 00361 } 00362 00363 if (outputInvered) { 00364 MCPWMx->MCCON_SET = MCPWM_CON_INVBDC; 00365 } else { 00366 MCPWMx->MCCON_CLR = MCPWM_CON_INVBDC; 00367 } 00368 00369 MCPWMx->MCCCP = outputPattern; 00370 } 00371 00372 00373 /*********************************************************************/ 00394 void MCPWM_IntConfig(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType, FunctionalState NewState) 00395 { 00396 if (NewState) { 00397 MCPWMx->MCINTEN_SET = ulIntType; 00398 } else { 00399 MCPWMx->MCINTEN_CLR = ulIntType; 00400 } 00401 } 00402 00403 00404 /*********************************************************************/ 00421 void MCPWM_IntSet(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType) 00422 { 00423 MCPWMx->MCINTFLAG_SET = ulIntType; 00424 } 00425 00426 00427 /*********************************************************************/ 00444 void MCPWM_IntClear(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType) 00445 { 00446 MCPWMx->MCINTFLAG_CLR = ulIntType; 00447 } 00448 00449 00450 /*********************************************************************/ 00466 FlagStatus MCPWM_GetIntStatus(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType) 00467 { 00468 return ((MCPWMx->MCINTFLAG & ulIntType) ? SET : RESET); 00469 } 00470 00475 #endif /* _MCPWM */ 00476 00481 /* --------------------------------- End Of File ------------------------------ */
Generated on Mon Feb 8 10:01:37 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
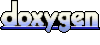