C:/nxpdrv/LPC1700CMSIS/Drivers/source/lpc17xx_qei.c
Go to the documentation of this file.00001 00020 /* Peripheral group ----------------------------------------------------------- */ 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_qei.h" 00027 #include "lpc17xx_clkpwr.h" 00028 00029 00030 /* If this source file built with example, the LPC17xx FW library configuration 00031 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00032 * otherwise the default FW library configuration file must be included instead 00033 */ 00034 #ifdef __BUILD_WITH_EXAMPLE__ 00035 #include "lpc17xx_libcfg.h" 00036 #else 00037 #include "lpc17xx_libcfg_default.h" 00038 #endif /* __BUILD_WITH_EXAMPLE__ */ 00039 00040 00041 #ifdef _QEI 00042 00043 /* Private Types -------------------------------------------------------------- */ 00051 typedef union { 00052 QEI_CFG_Type bmQEIConfig; 00053 uint32_t ulQEIConfig; 00054 } QEI_CFGOPT_Type; 00055 00061 /* Public Functions ----------------------------------------------------------- */ 00066 /*********************************************************************/ 00077 void QEI_Reset(LPC_QEI_TypeDef *QEIx, uint32_t ulResetType) 00078 { 00079 CHECK_PARAM(PARAM_QEIx(QEIx)); 00080 CHECK_PARAM(PARAM_QEI_RESET(ulResetType)); 00081 00082 QEIx->QEICON = ulResetType; 00083 } 00084 00085 /*********************************************************************/ 00094 void QEI_Init(LPC_QEI_TypeDef *QEIx, QEI_CFG_Type *QEI_ConfigStruct) 00095 { 00096 00097 CHECK_PARAM(PARAM_QEIx(QEIx)); 00098 CHECK_PARAM(PARAM_QEI_DIRINV(QEI_ConfigStruct->DirectionInvert)); 00099 CHECK_PARAM(PARAM_QEI_SIGNALMODE(QEI_ConfigStruct->SignalMode)); 00100 CHECK_PARAM(PARAM_QEI_CAPMODE(QEI_ConfigStruct->CaptureMode)); 00101 CHECK_PARAM(PARAM_QEI_INVINX(QEI_ConfigStruct->InvertIndex)); 00102 00103 /* Set up clock and power for QEI module */ 00104 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCQEI, ENABLE); 00105 00106 /* As default, peripheral clock for QEI module 00107 * is set to FCCLK / 2 */ 00108 CLKPWR_SetPCLKDiv(CLKPWR_PCLKSEL_QEI, CLKPWR_PCLKSEL_CCLK_DIV_1); 00109 00110 // Reset all remaining value in QEI peripheral 00111 QEIx->QEICON = QEI_CON_RESP | QEI_CON_RESV | QEI_CON_RESI; 00112 QEIx->QEIMAXPOS = 0x00; 00113 QEIx->CMPOS0 = 0x00; 00114 QEIx->CMPOS1 = 0x00; 00115 QEIx->CMPOS2 = 0x00; 00116 QEIx->INXCMP = 0x00; 00117 QEIx->QEILOAD = 0x00; 00118 QEIx->VELCOMP = 0x00; 00119 QEIx->FILTER = 0x00; 00120 // Disable all Interrupt 00121 QEIx->QEIIEC = QEI_IECLR_BITMASK; 00122 // Clear all Interrupt pending 00123 QEIx->QEICLR = QEI_INTCLR_BITMASK; 00124 // Set QEI configuration value corresponding to its setting up value 00125 QEIx->QEICONF = ((QEI_CFGOPT_Type *)QEI_ConfigStruct)->ulQEIConfig; 00126 } 00127 00128 00129 /*********************************************************************/ 00135 void QEI_DeInit(LPC_QEI_TypeDef *QEIx) 00136 { 00137 CHECK_PARAM(PARAM_QEIx(QEIx)); 00138 00139 /* Turn off clock and power for QEI module */ 00140 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCQEI, DISABLE); 00141 } 00142 00143 00144 /*****************************************************************************/ 00154 void QEI_ConfigStructInit(QEI_CFG_Type *QIE_InitStruct) 00155 { 00156 QIE_InitStruct->CaptureMode = QEI_CAPMODE_4X; 00157 QIE_InitStruct->DirectionInvert = QEI_DIRINV_NONE; 00158 QIE_InitStruct->InvertIndex = QEI_INVINX_NONE; 00159 QIE_InitStruct->SignalMode = QEI_SIGNALMODE_QUAD; 00160 } 00161 00162 00163 /*********************************************************************/ 00170 FlagStatus QEI_GetStatus(LPC_QEI_TypeDef *QEIx, uint32_t ulFlagType) 00171 { 00172 CHECK_PARAM(PARAM_QEIx(QEIx)); 00173 CHECK_PARAM(PARAM_QEI_STATUS(ulFlagType)); 00174 return ((QEIx->QEISTAT & ulFlagType) ? SET : RESET); 00175 } 00176 00177 /*********************************************************************/ 00182 uint32_t QEI_GetPosition(LPC_QEI_TypeDef *QEIx) 00183 { 00184 CHECK_PARAM(PARAM_QEIx(QEIx)); 00185 return (QEIx->QEIPOS); 00186 } 00187 00188 /*********************************************************************/ 00194 void QEI_SetMaxPosition(LPC_QEI_TypeDef *QEIx, uint32_t ulMaxPos) 00195 { 00196 CHECK_PARAM(PARAM_QEIx(QEIx)); 00197 QEIx->QEIMAXPOS = ulMaxPos; 00198 } 00199 00200 /*********************************************************************/ 00210 void QEI_SetPositionComp(LPC_QEI_TypeDef *QEIx, uint8_t bPosCompCh, uint32_t ulPosComp) 00211 { 00212 uint32_t *tmp; 00213 00214 CHECK_PARAM(PARAM_QEIx(QEIx)); 00215 CHECK_PARAM(PARAM_QEI_COMPPOS_CH(bPosCompCh)); 00216 tmp = (uint32_t *) (&(QEIx->CMPOS0) + bPosCompCh * 4); 00217 *tmp = ulPosComp; 00218 00219 } 00220 00221 /*********************************************************************/ 00226 uint32_t QEI_GetIndex(LPC_QEI_TypeDef *QEIx) 00227 { 00228 CHECK_PARAM(PARAM_QEIx(QEIx)); 00229 return (QEIx->INXCNT); 00230 } 00231 00232 /*********************************************************************/ 00238 void QEI_SetIndexComp(LPC_QEI_TypeDef *QEIx, uint32_t ulIndexComp) 00239 { 00240 CHECK_PARAM(PARAM_QEIx(QEIx)); 00241 QEIx->INXCMP = ulIndexComp; 00242 } 00243 00244 /*********************************************************************/ 00253 void QEI_SetTimerReload(LPC_QEI_TypeDef *QEIx, QEI_RELOADCFG_Type *QEIReloadStruct) 00254 { 00255 uint64_t pclk; 00256 00257 CHECK_PARAM(PARAM_QEIx(QEIx)); 00258 CHECK_PARAM(PARAM_QEI_TIMERRELOAD(QEIReloadStruct->ReloadOption)); 00259 00260 if (QEIReloadStruct->ReloadOption == QEI_TIMERRELOAD_TICKVAL) { 00261 QEIx->QEILOAD = QEIReloadStruct->ReloadValue - 1; 00262 } else { 00263 pclk = (uint64_t)CLKPWR_GetPCLK(CLKPWR_PCLKSEL_QEI); 00264 pclk = (pclk /(1000000/QEIReloadStruct->ReloadValue)) - 1; 00265 QEIx->QEILOAD = (uint32_t)pclk; 00266 } 00267 } 00268 00269 /*********************************************************************/ 00274 uint32_t QEI_GetTimer(LPC_QEI_TypeDef *QEIx) 00275 { 00276 CHECK_PARAM(PARAM_QEIx(QEIx)); 00277 return (QEIx->QEITIME); 00278 } 00279 00280 /*********************************************************************/ 00285 uint32_t QEI_GetVelocity(LPC_QEI_TypeDef *QEIx) 00286 { 00287 CHECK_PARAM(PARAM_QEIx(QEIx)); 00288 return (QEIx->QEIVEL); 00289 } 00290 00291 /*********************************************************************/ 00298 uint32_t QEI_GetVelocityCap(LPC_QEI_TypeDef *QEIx) 00299 { 00300 CHECK_PARAM(PARAM_QEIx(QEIx)); 00301 return (QEIx->QEICAP); 00302 } 00303 00304 /*********************************************************************/ 00310 void QEI_SetVelocityComp(LPC_QEI_TypeDef *QEIx, uint32_t ulVelComp) 00311 { 00312 CHECK_PARAM(PARAM_QEIx(QEIx)); 00313 QEIx->VELCOMP = ulVelComp; 00314 } 00315 00316 /*********************************************************************/ 00323 void QEI_SetDigiFilter(LPC_QEI_TypeDef *QEIx, uint32_t ulSamplingPulse) 00324 { 00325 CHECK_PARAM(PARAM_QEIx(QEIx)); 00326 QEIx->FILTER = ulSamplingPulse; 00327 } 00328 00329 /*********************************************************************/ 00353 FlagStatus QEI_GetIntStatus(LPC_QEI_TypeDef *QEIx, uint32_t ulIntType) 00354 { 00355 CHECK_PARAM(PARAM_QEIx(QEIx)); 00356 CHECK_PARAM(PARAM_QEI_INTFLAG(ulIntType)); 00357 00358 return((QEIx->QEIINTSTAT & ulIntType) ? SET : RESET); 00359 } 00360 00361 /*********************************************************************/ 00387 void QEI_IntCmd(LPC_QEI_TypeDef *QEIx, uint32_t ulIntType, FunctionalState NewState) 00388 { 00389 CHECK_PARAM(PARAM_QEIx(QEIx)); 00390 CHECK_PARAM(PARAM_QEI_INTFLAG(ulIntType)); 00391 CHECK_PARAM(PARAM_FUNCTIONALSTATE(NewState)); 00392 00393 if (NewState == ENABLE) { 00394 QEIx->QEIIES = ulIntType; 00395 } else { 00396 QEIx->QEIIEC = ulIntType; 00397 } 00398 } 00399 00400 00401 /*********************************************************************/ 00424 void QEI_IntSet(LPC_QEI_TypeDef *QEIx, uint32_t ulIntType) 00425 { 00426 CHECK_PARAM(PARAM_QEIx(QEIx)); 00427 CHECK_PARAM(PARAM_QEI_INTFLAG(ulIntType)); 00428 00429 QEIx->QEISET = ulIntType; 00430 } 00431 00432 /*********************************************************************/ 00455 void QEI_IntClear(LPC_QEI_TypeDef *QEIx, uint32_t ulIntType) 00456 { 00457 CHECK_PARAM(PARAM_QEIx(QEIx)); 00458 CHECK_PARAM(PARAM_QEI_INTFLAG(ulIntType)); 00459 00460 QEIx->QEICLR = ulIntType; 00461 } 00462 00463 00464 /*********************************************************************/ 00474 uint32_t QEI_CalculateRPM(LPC_QEI_TypeDef *QEIx, uint32_t ulVelCapValue, uint32_t ulPPR) 00475 { 00476 uint64_t rpm, clock, Load, edges; 00477 00478 // Get current Clock rate for timer input 00479 clock = (uint64_t)CLKPWR_GetPCLK(CLKPWR_PCLKSEL_QEI); 00480 // Get Timer load value (velocity capture period) 00481 Load = (uint64_t)(QEIx->QEILOAD + 1); 00482 // Get Edge 00483 edges = (uint64_t)((QEIx->QEICONF & QEI_CONF_CAPMODE) ? 4 : 2); 00484 // Calculate RPM 00485 rpm = ((clock * ulVelCapValue * 60) / (Load * ulPPR * edges)); 00486 00487 return (uint32_t)(rpm); 00488 } 00489 00490 00495 #endif /* _QEI */ 00496 00501 /* --------------------------------- End Of File ------------------------------ */ 00502
Generated on Mon Feb 8 10:01:38 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
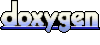