![]() |
X-CUBE-SPN11 for X-NUCLEO-IHM11M1
|
stm32f4xx_it.c
Go to the documentation of this file.
void TIM4_IRQHandler(void)
This function handles TIM4 global interrupt.
Definition: stm32f4xx_it.c:104
void USART2_IRQHandler(void)
This function handles USART2 global interrupt.
Definition: stm32f4xx_it.c:88
void TIM1_BRK_TIM9_IRQHandler(void)
This function handles TIM1 Break interrupt and TIM9 global interrupt.
Definition: stm32f4xx_it.c:132
void UART_Set_Value(void)
This file contains the headers of the interrupt handlers.
Definition: 6Step_Lib.h:78
void EXTI15_10_IRQHandler(void)
This function handles EXTI Line[15:10] interrupts.
Definition: stm32f4xx_it.c:118
This header file provides the set of functions for Motor Control library.
void HAL_IncTick(void)
This function is called to increment a global variable "uwTick" used as application time base...
Definition: 6Step_Lib.c:1607
Generated by
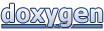