C:/nxpdrv/LPC1700CMSIS/Drivers/source/lpc17xx_rit.c
Go to the documentation of this file.00001 00020 /* Peripheral group ----------------------------------------------------------- */ 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_rit.h" 00027 #include "lpc17xx_clkpwr.h" 00028 00029 /* If this source file built with example, the LPC17xx FW library configuration 00030 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00031 * otherwise the default FW library configuration file must be included instead 00032 */ 00033 #ifdef __BUILD_WITH_EXAMPLE__ 00034 #include "lpc17xx_libcfg.h" 00035 #else 00036 #include "lpc17xx_libcfg_default.h" 00037 #endif /* __BUILD_WITH_EXAMPLE__ */ 00038 00039 #ifdef _RIT 00040 00041 /* Public Functions ----------------------------------------------------------- */ 00046 /******************************************************************************//* 00047 * @brief Initial for RIT 00048 * - Turn on power and clock 00049 * - Setup default register values 00050 * @param[in] RITx is RIT peripheral selected, should be: RIT 00051 * @return None 00052 *******************************************************************************/ 00053 void RIT_Init(LPC_RIT_TypeDef *RITx) 00054 { 00055 CHECK_PARAM(PARAM_RITx(RITx)); 00056 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCRIT, ENABLE); 00057 //Set up default register values 00058 RITx->RICOMPVAL = 0xFFFFFFFF; 00059 RITx->RIMASK = 0x00000000; 00060 RITx->RICTRL = 0x0C; 00061 RITx->RICOUNTER = 0x00000000; 00062 // Turn on power and clock 00063 00064 } 00065 /******************************************************************************//* 00066 * @brief DeInitial for RIT 00067 * - Turn off power and clock 00068 * - ReSetup default register values 00069 * @param[in] RITx is RIT peripheral selected, should be: RIT 00070 * @return None 00071 *******************************************************************************/ 00072 void RIT_DeInit(LPC_RIT_TypeDef *RITx) 00073 { 00074 CHECK_PARAM(PARAM_RITx(RITx)); 00075 00076 // Turn off power and clock 00077 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCRIT, DISABLE); 00078 //ReSetup default register values 00079 RITx->RICOMPVAL = 0xFFFFFFFF; 00080 RITx->RIMASK = 0x00000000; 00081 RITx->RICTRL = 0x0C; 00082 RITx->RICOUNTER = 0x00000000; 00083 } 00084 /******************************************************************************//* 00085 * @brief Set compare value, mask value and time counter value 00086 * @param[in] RITx is RIT peripheral selected, should be: RIT 00087 * @param[in] value: pointer to RIT_CMP_VAL Structure 00088 * @return None 00089 *******************************************************************************/ 00090 void RIT_TimerConfig(LPC_RIT_TypeDef *RITx, RIT_CMP_VAL *value) 00091 { 00092 CHECK_PARAM(PARAM_RITx(RITx)); 00093 00094 RITx->RICOMPVAL = value->CMPVAL; 00095 RITx->RIMASK = value->MASKVAL; 00096 RITx->RICOUNTER = value->COUNTVAL; 00097 } 00098 /******************************************************************************//* 00099 * @brief Enable/Disable Timer 00100 * @param[in] RITx is RIT peripheral selected, should be: RIT 00101 * @param[in] NewState New State of this function 00102 * -ENABLE: Enable Timer 00103 * -DISABLE: Disable Timer 00104 * @return None 00105 *******************************************************************************/ 00106 void RIT_Cmd(LPC_RIT_TypeDef *RITx, FunctionalState NewState) 00107 { 00108 CHECK_PARAM(PARAM_RITx(RITx)); 00109 CHECK_PARAM(PARAM_FUNCTIONALSTATE(NewState)); 00110 00111 //Enable or Disable Timer 00112 if(NewState==ENABLE) 00113 { 00114 RITx->RICTRL |= RIT_CTRL_TEN; 00115 } 00116 else 00117 { 00118 RITx->RICTRL &= ~RIT_CTRL_TEN; 00119 } 00120 } 00121 /******************************************************************************//* 00122 * @brief Timer Enable/Disable Clear 00123 * @param[in] RITx is RIT peripheral selected, should be: RIT 00124 * @param[in] NewState New State of this function 00125 * -ENABLE: The timer will be cleared to 0 whenever 00126 * the counter value equals the masked compare value specified 00127 * by the contents of RICOMPVAL and RIMASK register 00128 * -DISABLE: The timer will not be clear to 0 00129 * @return None 00130 *******************************************************************************/ 00131 void RIT_TimerClearCmd(LPC_RIT_TypeDef *RITx, FunctionalState NewState) 00132 { 00133 CHECK_PARAM(PARAM_RITx(RITx)); 00134 CHECK_PARAM(PARAM_FUNCTIONALSTATE(NewState)); 00135 00136 //Timer Enable/Disable Clear 00137 if(NewState==ENABLE) 00138 { 00139 RITx->RICTRL |= RIT_CTRL_ENCLR; 00140 } 00141 else 00142 { 00143 RITx->RICTRL &= ~RIT_CTRL_ENCLR; 00144 } 00145 } 00146 /******************************************************************************//* 00147 * @brief Timer Enable/Disable on break 00148 * @param[in] RITx is RIT peripheral selected, should be: RIT 00149 * @param[in] NewState New State of this function 00150 * -ENABLE: The timer is halted whenever a hardware break condition occurs 00151 * -DISABLE: Hardware break has no effect on the timer operation 00152 * @return None 00153 *******************************************************************************/ 00154 void RIT_TimerEnableOnBreakCmd(LPC_RIT_TypeDef *RITx, FunctionalState NewState) 00155 { 00156 CHECK_PARAM(PARAM_RITx(RITx)); 00157 CHECK_PARAM(PARAM_FUNCTIONALSTATE(NewState)); 00158 00159 //Timer Enable/Disable on break 00160 if(NewState==ENABLE) 00161 { 00162 RITx->RICTRL |= RIT_CTRL_ENBR; 00163 } 00164 else 00165 { 00166 RITx->RICTRL &= ~RIT_CTRL_ENBR; 00167 } 00168 } 00169 /******************************************************************************//* 00170 * @brief Check whether interrupt flag is set or not 00171 * @param[in] RITx is RIT peripheral selected, should be: RIT 00172 * @return Current interrupt status, could be: SET/RESET 00173 *******************************************************************************/ 00174 IntStatus RIT_GetIntStatus(LPC_RIT_TypeDef *RITx) 00175 { 00176 uint8_t result; 00177 CHECK_PARAM(PARAM_RITx(RITx)); 00178 if((RITx->RICTRL&RIT_CTRL_INTEN)==1) result= SET; 00179 else return RESET; 00180 //clear interrupt flag 00181 RITx->RICTRL |= RIT_CTRL_INTEN; 00182 return result; 00183 } 00184 00189 #endif /* _RIT */ 00190 00195 /* --------------------------------- End Of File ------------------------------ */
Generated on Mon Feb 8 10:01:38 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
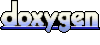