C:/nxpdrv/LPC1700CMSIS/Drivers/source/lpc17xx_pwm.c
Go to the documentation of this file.00001 00020 /* Peripheral group ----------------------------------------------------------- */ 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_pwm.h" 00027 #include "lpc17xx_clkpwr.h" 00028 00029 /* If this source file built with example, the LPC17xx FW library configuration 00030 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00031 * otherwise the default FW library configuration file must be included instead 00032 */ 00033 #ifdef __BUILD_WITH_EXAMPLE__ 00034 #include "lpc17xx_libcfg.h" 00035 #else 00036 #include "lpc17xx_libcfg_default.h" 00037 #endif /* __BUILD_WITH_EXAMPLE__ */ 00038 00039 00040 #ifdef _PWM 00041 00042 00043 /* Public Functions ----------------------------------------------------------- */ 00049 /*********************************************************************/ 00064 IntStatus PWM_GetIntStatus(LPC_PWM_TypeDef *PWMx, uint32_t IntFlag) 00065 { 00066 CHECK_PARAM(PARAM_PWMx(PWMx)); 00067 CHECK_PARAM(PARAM_PWM_INTSTAT(IntFlag)); 00068 00069 return ((PWMx->IR & IntFlag) ? SET : RESET); 00070 } 00071 00072 00073 00074 /*********************************************************************/ 00089 void PWM_ClearIntPending(LPC_PWM_TypeDef *PWMx, uint32_t IntFlag) 00090 { 00091 CHECK_PARAM(PARAM_PWMx(PWMx)); 00092 CHECK_PARAM(PARAM_PWM_INTSTAT(IntFlag)); 00093 PWMx->IR = IntFlag; 00094 } 00095 00096 00097 00098 /*****************************************************************************/ 00115 void PWM_ConfigStructInit(uint8_t PWMTimerCounterMode, void *PWM_InitStruct) 00116 { 00117 CHECK_PARAM(PARAM_PWM_TC_MODE(PWMTimerCounterMode)); 00118 00119 PWM_TIMERCFG_Type *pTimeCfg = (PWM_TIMERCFG_Type *) PWM_InitStruct; 00120 PWM_COUNTERCFG_Type *pCounterCfg = (PWM_COUNTERCFG_Type *) PWM_InitStruct; 00121 00122 if (PWMTimerCounterMode == PWM_MODE_TIMER ) 00123 { 00124 pTimeCfg->PrescaleOption = PWM_TIMER_PRESCALE_USVAL; 00125 pTimeCfg->PrescaleValue = 1; 00126 } 00127 else if (PWMTimerCounterMode == PWM_MODE_COUNTER) 00128 { 00129 pCounterCfg->CountInputSelect = PWM_COUNTER_PCAP1_0; 00130 pCounterCfg->CounterOption = PWM_COUNTER_RISING; 00131 } 00132 } 00133 00134 00135 /*********************************************************************/ 00148 void PWM_Init(LPC_PWM_TypeDef *PWMx, uint32_t PWMTimerCounterMode, void *PWM_ConfigStruct) 00149 { 00150 CHECK_PARAM(PARAM_PWMx(PWMx)); 00151 CHECK_PARAM(PARAM_PWM_TC_MODE(PWMTimerCounterMode)); 00152 00153 PWM_TIMERCFG_Type *pTimeCfg = (PWM_TIMERCFG_Type *)PWM_ConfigStruct; 00154 PWM_COUNTERCFG_Type *pCounterCfg = (PWM_COUNTERCFG_Type *)PWM_ConfigStruct; 00155 uint64_t clkdlycnt; 00156 00157 if (PWMx == LPC_PWM1) 00158 { 00159 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCPWM1, ENABLE); 00160 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_PWM1, CLKPWR_PCLKSEL_CCLK_DIV_4); 00161 // Get peripheral clock of PWM1 00162 clkdlycnt = (uint64_t) CLKPWR_GetPCLK (CLKPWR_PCLKSEL_PWM1); 00163 } 00164 00165 // Clear all interrupts pending 00166 PWMx->IR = 0xFF & PWM_IR_BITMASK; 00167 PWMx->TCR = 0x00; 00168 PWMx->CTCR = 0x00; 00169 PWMx->MCR = 0x00; 00170 PWMx->CCR = 0x00; 00171 PWMx->PCR = 0x00; 00172 PWMx->LER = 0x00; 00173 00174 if (PWMTimerCounterMode == PWM_MODE_TIMER) 00175 { 00176 CHECK_PARAM(PARAM_PWM_TIMER_PRESCALE(pTimeCfg->PrescaleOption)); 00177 00178 /* Absolute prescale value */ 00179 if (pTimeCfg->PrescaleOption == PWM_TIMER_PRESCALE_TICKVAL) 00180 { 00181 PWMx->PR = pTimeCfg->PrescaleValue - 1; 00182 } 00183 /* uSecond prescale value */ 00184 else 00185 { 00186 clkdlycnt = (clkdlycnt * pTimeCfg->PrescaleValue) / 1000000; 00187 PWMx->PR = ((uint32_t) clkdlycnt) - 1; 00188 } 00189 00190 } 00191 else if (PWMTimerCounterMode == PWM_MODE_COUNTER) 00192 { 00193 CHECK_PARAM(PARAM_PWM_COUNTER_INPUTSEL(pCounterCfg->CountInputSelect)); 00194 CHECK_PARAM(PARAM_PWM_COUNTER_EDGE(pCounterCfg->CounterOption)); 00195 00196 PWMx->CTCR |= (PWM_CTCR_MODE((uint32_t)pCounterCfg->CounterOption)) \ 00197 | (PWM_CTCR_SELECT_INPUT((uint32_t)pCounterCfg->CountInputSelect)); 00198 } 00199 } 00200 00201 /*********************************************************************/ 00207 void PWM_DeInit (LPC_PWM_TypeDef *PWMx) 00208 { 00209 CHECK_PARAM(PARAM_PWMx(PWMx)); 00210 00211 // Disable PWM control (timer, counter and PWM) 00212 PWMx->TCR = 0x00; 00213 00214 if (PWMx == LPC_PWM1) 00215 { 00216 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCPWM1, DISABLE); 00217 } 00218 } 00219 00220 00221 /*********************************************************************/ 00229 void PWM_Cmd(LPC_PWM_TypeDef *PWMx, FunctionalState NewState) 00230 { 00231 CHECK_PARAM(PARAM_PWMx(PWMx)); 00232 CHECK_PARAM(PARAM_FUNCTIONALSTATE(NewState)); 00233 00234 if (NewState == ENABLE) 00235 { 00236 PWMx->TCR |= PWM_TCR_PWM_ENABLE; 00237 } 00238 else 00239 { 00240 PWMx->TCR &= (~PWM_TCR_PWM_ENABLE) & PWM_TCR_BITMASK; 00241 } 00242 } 00243 00244 00245 /*********************************************************************/ 00253 void PWM_CounterCmd(LPC_PWM_TypeDef *PWMx, FunctionalState NewState) 00254 { 00255 CHECK_PARAM(PARAM_PWMx(PWMx)); 00256 CHECK_PARAM(PARAM_FUNCTIONALSTATE(NewState)); 00257 if (NewState == ENABLE) 00258 { 00259 PWMx->TCR |= PWM_TCR_COUNTER_ENABLE; 00260 } 00261 else 00262 { 00263 PWMx->TCR &= (~PWM_TCR_COUNTER_ENABLE) & PWM_TCR_BITMASK; 00264 } 00265 } 00266 00267 00268 /*********************************************************************/ 00273 void PWM_ResetCounter(LPC_PWM_TypeDef *PWMx) 00274 { 00275 CHECK_PARAM(PARAM_PWMx(PWMx)); 00276 PWMx->TCR |= PWM_TCR_COUNTER_RESET; 00277 PWMx->TCR &= (~PWM_TCR_COUNTER_RESET) & PWM_TCR_BITMASK; 00278 } 00279 00280 00281 /*********************************************************************/ 00289 void PWM_ConfigMatch(LPC_PWM_TypeDef *PWMx, PWM_MATCHCFG_Type *PWM_MatchConfigStruct) 00290 { 00291 CHECK_PARAM(PARAM_PWMx(PWMx)); 00292 CHECK_PARAM(PARAM_PWM1_MATCH_CHANNEL(PWM_MatchConfigStruct->MatchChannel)); 00293 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_MatchConfigStruct->IntOnMatch)); 00294 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_MatchConfigStruct->ResetOnMatch)); 00295 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_MatchConfigStruct->StopOnMatch)); 00296 00297 //interrupt on MRn 00298 if (PWM_MatchConfigStruct->IntOnMatch == ENABLE) 00299 { 00300 PWMx->MCR |= PWM_MCR_INT_ON_MATCH(PWM_MatchConfigStruct->MatchChannel); 00301 } 00302 else 00303 { 00304 PWMx->MCR &= (~PWM_MCR_INT_ON_MATCH(PWM_MatchConfigStruct->MatchChannel)) \ 00305 & PWM_MCR_BITMASK; 00306 } 00307 00308 //reset on MRn 00309 if (PWM_MatchConfigStruct->ResetOnMatch == ENABLE) 00310 { 00311 PWMx->MCR |= PWM_MCR_RESET_ON_MATCH(PWM_MatchConfigStruct->MatchChannel); 00312 } 00313 else 00314 { 00315 PWMx->MCR &= (~PWM_MCR_RESET_ON_MATCH(PWM_MatchConfigStruct->MatchChannel)) \ 00316 & PWM_MCR_BITMASK; 00317 } 00318 00319 //stop on MRn 00320 if (PWM_MatchConfigStruct->StopOnMatch == ENABLE) 00321 { 00322 PWMx->MCR |= PWM_MCR_STOP_ON_MATCH(PWM_MatchConfigStruct->MatchChannel); 00323 } 00324 else 00325 { 00326 PWMx->MCR &= (~PWM_MCR_STOP_ON_MATCH(PWM_MatchConfigStruct->MatchChannel)) \ 00327 & PWM_MCR_BITMASK; 00328 } 00329 } 00330 00331 00332 /*********************************************************************/ 00340 void PWM_ConfigCapture(LPC_PWM_TypeDef *PWMx, PWM_CAPTURECFG_Type *PWM_CaptureConfigStruct) 00341 { 00342 CHECK_PARAM(PARAM_PWMx(PWMx)); 00343 CHECK_PARAM(PARAM_PWM1_CAPTURE_CHANNEL(PWM_CaptureConfigStruct->CaptureChannel)); 00344 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_CaptureConfigStruct->FallingEdge)); 00345 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_CaptureConfigStruct->IntOnCaption)); 00346 CHECK_PARAM(PARAM_FUNCTIONALSTATE(PWM_CaptureConfigStruct->RisingEdge)); 00347 00348 if (PWM_CaptureConfigStruct->RisingEdge == ENABLE) 00349 { 00350 PWMx->CCR |= PWM_CCR_CAP_RISING(PWM_CaptureConfigStruct->CaptureChannel); 00351 } 00352 else 00353 { 00354 PWMx->CCR &= (~PWM_CCR_CAP_RISING(PWM_CaptureConfigStruct->CaptureChannel)) \ 00355 & PWM_CCR_BITMASK; 00356 } 00357 00358 if (PWM_CaptureConfigStruct->FallingEdge == ENABLE) 00359 { 00360 PWMx->CCR |= PWM_CCR_CAP_FALLING(PWM_CaptureConfigStruct->CaptureChannel); 00361 } 00362 else 00363 { 00364 PWMx->CCR &= (~PWM_CCR_CAP_FALLING(PWM_CaptureConfigStruct->CaptureChannel)) \ 00365 & PWM_CCR_BITMASK; 00366 } 00367 00368 if (PWM_CaptureConfigStruct->IntOnCaption == ENABLE) 00369 { 00370 PWMx->CCR |= PWM_CCR_INT_ON_CAP(PWM_CaptureConfigStruct->CaptureChannel); 00371 } 00372 else 00373 { 00374 PWMx->CCR &= (~PWM_CCR_INT_ON_CAP(PWM_CaptureConfigStruct->CaptureChannel)) \ 00375 & PWM_CCR_BITMASK; 00376 } 00377 } 00378 00379 00380 /*********************************************************************/ 00387 uint32_t PWM_GetCaptureValue(LPC_PWM_TypeDef *PWMx, uint8_t CaptureChannel) 00388 { 00389 CHECK_PARAM(PARAM_PWMx(PWMx)); 00390 CHECK_PARAM(PARAM_PWM1_CAPTURE_CHANNEL(CaptureChannel)); 00391 00392 switch (CaptureChannel) 00393 { 00394 case 0: 00395 return PWMx->CR0; 00396 break; 00397 00398 case 1: 00399 return PWMx->CR1; 00400 break; 00401 00402 default: 00403 return (0); 00404 break; 00405 } 00406 } 00407 00408 00409 /********************************************************************/ 00421 void PWM_MatchUpdate(LPC_PWM_TypeDef *PWMx, uint8_t MatchChannel, \ 00422 uint32_t MatchValue, uint8_t UpdateType) 00423 { 00424 CHECK_PARAM(PARAM_PWMx(PWMx)); 00425 CHECK_PARAM(PARAM_PWM1_MATCH_CHANNEL(MatchChannel)); 00426 CHECK_PARAM(PARAM_PWM_MATCH_UPDATE(UpdateType)); 00427 00428 switch (MatchChannel) 00429 { 00430 case 0: 00431 PWMx->MR0 = MatchValue; 00432 break; 00433 00434 case 1: 00435 PWMx->MR1 = MatchValue; 00436 break; 00437 00438 case 2: 00439 PWMx->MR2 = MatchValue; 00440 break; 00441 00442 case 3: 00443 PWMx->MR3 = MatchValue; 00444 break; 00445 00446 case 4: 00447 PWMx->MR4 = MatchValue; 00448 break; 00449 00450 case 5: 00451 PWMx->MR5 = MatchValue; 00452 break; 00453 00454 case 6: 00455 PWMx->MR6 = MatchValue; 00456 break; 00457 } 00458 00459 // Write Latch register 00460 PWMx->LER |= PWM_LER_EN_MATCHn_LATCH(MatchChannel); 00461 00462 // In case of update now 00463 if (UpdateType == PWM_MATCH_UPDATE_NOW) 00464 { 00465 PWMx->TCR |= PWM_TCR_COUNTER_RESET; 00466 PWMx->TCR &= (~PWM_TCR_COUNTER_RESET) & PWM_TCR_BITMASK; 00467 } 00468 } 00469 00470 00471 /********************************************************************/ 00481 void PWM_ChannelConfig(LPC_PWM_TypeDef *PWMx, uint8_t PWMChannel, uint8_t ModeOption) 00482 { 00483 CHECK_PARAM(PARAM_PWMx(PWMx)); 00484 CHECK_PARAM(PARAM_PWM1_EDGE_MODE_CHANNEL(PWMChannel)); 00485 CHECK_PARAM(PARAM_PWM_CHANNEL_EDGE(ModeOption)); 00486 00487 // Single edge mode 00488 if (ModeOption == PWM_CHANNEL_SINGLE_EDGE) 00489 { 00490 PWMx->PCR &= (~PWM_PCR_PWMSELn(PWMChannel)) & PWM_PCR_BITMASK; 00491 } 00492 // Double edge mode 00493 else if (PWM_CHANNEL_DUAL_EDGE) 00494 { 00495 PWMx->PCR |= PWM_PCR_PWMSELn(PWMChannel); 00496 } 00497 } 00498 00499 00500 00501 /********************************************************************/ 00510 void PWM_ChannelCmd(LPC_PWM_TypeDef *PWMx, uint8_t PWMChannel, FunctionalState NewState) 00511 { 00512 CHECK_PARAM(PARAM_PWMx(PWMx)); 00513 CHECK_PARAM(PARAM_PWM1_CHANNEL(PWMChannel)); 00514 00515 if (NewState == ENABLE) 00516 { 00517 PWMx->PCR |= PWM_PCR_PWMENAn(PWMChannel); 00518 } 00519 else 00520 { 00521 PWMx->PCR &= (~PWM_PCR_PWMENAn(PWMChannel)) & PWM_PCR_BITMASK; 00522 } 00523 } 00524 00529 #endif /* _PWM */ 00530 00535 /* --------------------------------- End Of File ------------------------------ */
Generated on Mon Feb 8 10:01:38 2010 for LPC1700CMSIS Standard Peripheral Firmware Library by
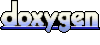