![]() |
XMC Peripheral Library for XMC4000 Family
2.1.16
|
Data Structures | |
struct | XMC_USBD_CAPABILITIES_t |
struct | XMC_USBD_DEVICE_t |
struct | XMC_USBD_DRIVER_t |
struct | XMC_USBD_EP_t |
struct | XMC_USBD_STATE_t |
struct | XMC_USBD_t |
Macros | |
#define | XMC_USBD_ENDPOINT_DIRECTION_MASK (0x80U) |
#define | XMC_USBD_ENDPOINT_MAX_PACKET_SIZE_MASK (0x07FFU) |
#define | XMC_USBD_ENDPOINT_NUMBER_MASK (0x0FU) |
#define | XMC_USBD_EP_DIR_MASK (0x80U) |
#define | XMC_USBD_EP_NUM_MASK (0x0FU) |
#define | XMC_USBD_MAX_FIFO_SIZE (2048U) |
#define | XMC_USBD_MAX_PACKET_SIZE (64U) |
#define | XMC_USBD_MAX_TRANSFER_SIZE_EP0 (64U) |
#define | XMC_USBD_NUM_EPS (7U) |
#define | XMC_USBD_NUM_TX_FIFOS (7U) |
#define | XMC_USBD_SETUP_COUNT (3U) |
#define | XMC_USBD_SETUP_SIZE (8U) |
#define | XMC_USBD_SPEED_FULL (1U) |
Typedefs | |
typedef void(* | XMC_USBD_SignalDeviceEvent_t) (XMC_USBD_EVENT_t event) |
typedef void(* | XMC_USBD_SignalEndpointEvent_t) (uint8_t ep_addr, XMC_USBD_EP_EVENT_t ep_event) |
Enumerations |
Variables | |
const XMC_USBD_DRIVER_t | Driver_USBD0 |
XMC_USBD_DEVICE_t | xmc_device |
Detailed Description
The USBD is the device driver for the USB0 hardware module on XMC4000 family of microcontrollers. The USB0 module can be used to establish a USB interface between outside world and XMC4000 family of controllers. The USB module includes the following features in device mode:
- Complies with the USB 2.0 Specification.
- Support for the Full-Speed (12-Mbps) mode.
- Supports up to 7 bidirectional endpoints, including control endpoint 0.
- Supports SOFs in Full-Speed modes.
- Supports clock gating for power saving.
- Supports USB suspend/resume.
- Supports USB soft disconnect.
- Supports DMA mode.
- Supports FIFO mode.
The below figure shows the overview of USB0 module in XMC4 microntroller.
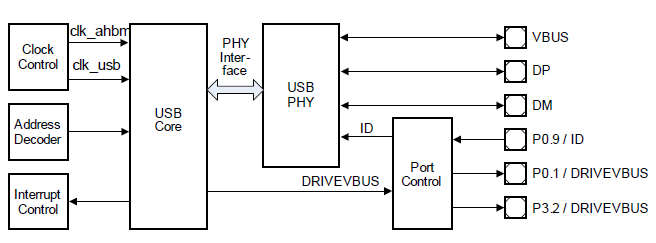
The below figure shows the USB device connection of USB0 module.
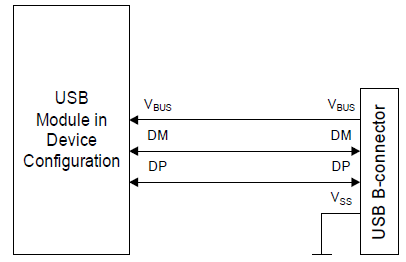
The USBD device driver supports the following features:
- Initialize/Uninitialize the USB0 module on XMC4000 device.
- Connect the USB device to host.
- Get USB device state.
- Set the USB device address.
- Configure/Unconfigure the USB endpoints.
- Stall/Abort the USB endpoints.
- USB IN transfers on EP0 and non EP0 endpoints.
- USB OUT transfers on EP0 and non EP0 endpoints.
The USBD device driver provides the configuration structure XMC_USBD_t which user need to configure before initializing the USB.
The following elements of configuration structure need to be initialized before calling the XMC_USBD_Init API:
- cb_xmc_device_event of type XMC_USBD_SignalDeviceEvent_t.
- cb_endpoint_event of type XMC_USBD_SignalEndpointEvent_t.
- usbd_max_num_eps of type XMC_USBD_MAX_NUM_EPS_t.
- usbd_transfer_mode of type XMC_USBD_TRANSFER_MODE_t.
Macro Definition Documentation
#define XMC_USBD_ENDPOINT_DIRECTION_MASK (0x80U) |
USB Endpoint direction mask to get the EP direction from address.
#define XMC_USBD_ENDPOINT_MAX_PACKET_SIZE_MASK (0x07FFU) |
USB Endpoint Maximum Packet Size mask
#define XMC_USBD_ENDPOINT_NUMBER_MASK (0x0FU) |
USB Endpoint number mask to get the EP number from address.
#define XMC_USBD_EP_DIR_MASK (0x80U) |
USB Endpoint direction mask
#define XMC_USBD_EP_NUM_MASK (0x0FU) |
USB Endpoint number mask.
#define XMC_USBD_MAX_FIFO_SIZE (2048U) |
Maximum USBD endpoint fifo size
#define XMC_USBD_MAX_PACKET_SIZE (64U) |
Maximum packet size for all endpoints (including ep0) Maximum transfer size for endpoints.
It's based on the maximum payload, due to the fact, that we only can transfer 2^10 - 1 packets and this is less than the transfer size field can hold.
#define XMC_USBD_MAX_TRANSFER_SIZE_EP0 (64U) |
Maximum transfer size for endpoint 0
#define XMC_USBD_NUM_EPS (7U) |
Number of hardware endpoints
#define XMC_USBD_NUM_TX_FIFOS (7U) |
Number of hardware transmission endpoint fifos
#define XMC_USBD_SETUP_COUNT (3U) |
The number of USB setup packets
#define XMC_USBD_SETUP_SIZE (8U) |
The size of USB setup data
#define XMC_USBD_SPEED_FULL (1U) |
Speed Mode. Full Speed
Typedef Documentation
typedef void(* XMC_USBD_SignalDeviceEvent_t) (XMC_USBD_EVENT_t event) |
USB device/endpoint event function pointersPointer to USB device event call back. Uses type XMC_USBD_EVENT_t as the argument of callback.
typedef void(* XMC_USBD_SignalEndpointEvent_t) (uint8_t ep_addr, XMC_USBD_EP_EVENT_t ep_event) |
Pointer to USB endpoint event call back. Uses type XMC_USBD_EP_EVENT_t and EP address as the argument of callback.
Enumeration Type Documentation
Defines the options for the USB endpoint type. The values are from the USB 2.0 specification. Use type XMC_USBD_ENDPOINT_TYPE_t for this enum.
enum XMC_USBD_EP_EVENT_t |
Defines the generic USB endpoint events. Use type XMC_USBD_EP_EVENT_t for this enum.
Enumerator | |
---|---|
XMC_USBD_EP_EVENT_SETUP |
SETUP packet |
XMC_USBD_EP_EVENT_OUT |
OUT packet |
XMC_USBD_EP_EVENT_IN |
IN packet |
Defines the USB IN endpoint events. Use type XMC_USBD_EVENT_IN_EP_t for this enum.
Defines the USB OUT endpoint events. Use type XMC_USBD_EVENT_OUT_EP_t for this enum.
enum XMC_USBD_EVENT_t |
Defines the USB Device events. Use type XMC_USBD_EVENT_t for this enum.
Defines the options for the global receive fifo packet status. Use type XMC_USBD_GRXSTS_PKTSTS_t for this enum.
Defines the options for the maximum number of endpoints used. Use type XMC_USBD_MAX_NUM_EPS_t for this enum.
Defines the options for USB device state while setting the address. Use type XMC_USBD_SET_ADDRESS_STAGE_t for this enum.
Enumerator | |
---|---|
XMC_USBD_SET_ADDRESS_STAGE_SETUP |
Setup address |
XMC_USBD_SET_ADDRESS_STAGE_STATUS |
Status address |
enum XMC_USBD_STATUS_t |
Defines the USB Device Status of executed operation. Use type XMC_USBD_STATUS_t for this enum.
Enumerator | |
---|---|
XMC_USBD_STATUS_OK |
USBD Status: Operation succeeded |
XMC_USBD_STATUS_BUSY |
Driver is busy and cannot handle request |
XMC_USBD_STATUS_ERROR |
USBD Status: Unspecified error |
Defines the options for the USB data transfer modes. Use type XMC_USBD_TRANSFER_MODE_t for this enum.
Enumerator | |
---|---|
XMC_USBD_USE_DMA |
Transfer by DMA |
XMC_USBD_USE_FIFO |
Transfer by FIFO |
Function Documentation
void XMC_USBD_ClearEvent | ( | XMC_USBD_EVENT_t | event | ) |
- Parameters
-
event The single event that needs to be cleared. Use XMC_USBD_EVENT_t as argument.
- Returns
- None.
- Description:
- Clears the selected USBD event.
It clears the event by writing to the GINTSTS register.
- Note:
- This API is called inside the USB interrupt handler to clear the event XMC_USBD_EVENT_t and maintain the device state machine.
- Related APIs:
- XMC_USBD_ClearEventOUTEP(),XMC_USBD_ClearEventINEP()
void XMC_USBD_ClearEventINEP | ( | uint32_t | event, |
uint8_t | ep_num | ||
) |
- Parameters
-
event The single event or multiple events that need to be cleared. ep_num The IN endpoint number on which the events to be cleared.
- Returns
- None.
- Description:
- Clears the single event or multiple events of the selected IN endpoint.
The multiple events can be selected by the bitwise OR operation of XMC_USBD_EVENT_IN_EP_t elements.
It clears the event by programming DIEPINT register.
- Note:
- This API is called inside the USB IN EP interrupt handler to clear the XMC_USBD_EVENT_IN_EP_t event and maintain the device state machine.
- Related APIs:
- XMC_USBD_ClearEventOUTEP()
void XMC_USBD_ClearEventOUTEP | ( | uint32_t | event, |
uint8_t | ep_num | ||
) |
- Parameters
-
event The single event or multiple events that need to be cleared. ep_num The OUT endpoint number on which the events to be cleared.
- Returns
- None.
- Description:
- Clears the single event or multiple events of the selected OUT endpoint.
The multiple events can be selected by the bitwise OR operation of XMC_USBD_EVENT_OUT_EP_t elements. It clears the event by writing to DOEPINT register.
- Note:
- This API is called inside the USB OUT EP interrupt handler to clear the XMC_USBD_EVENT_OUT_EP_t event and maintain the device state machine.
- Related APIs:
- XMC_USBD_ClearEventINEP()
XMC_USBD_STATUS_t XMC_USBD_DeviceConnect | ( | void | ) |
- Parameters
-
None.
- Returns
- XMC_USBD_STATUS_t.
- Description:
- Connects the USB device to host and triggers the USB enumeration.
Connects the USB device to host by programming DCTL register.
It resets the soft disconnect bit, which activates the speed pull up at d+ line of USB. XMC_USBD_Init() should be called before calling this API.
- Note:
- Once this API is called, USB host starts the enumeration process and the device should handle the descriptor requests.
- Related APIs:
- XMC_USBD_Init()
XMC_USBD_STATUS_t XMC_USBD_DeviceDisconnect | ( | void | ) |
- Parameters
-
None.
- Returns
- XMC_USBD_STATUS_t.
- Description:
- Disconnects the USB device from host.
By programming DCTL register, it sets the soft disconnect bit, which deactivates
the speed pull up at d+ line of USB.
- Note:
- Once this API is called, USB device will not be accessible from host.
- Related APIs:
- XMC_USBD_DeviceConnect()
XMC_USBD_STATE_t XMC_USBD_DeviceGetState | ( | const XMC_USBD_t *const | obj | ) |
- Parameters
-
obj The pointer to the USB device handle structure XMC_USBD_t.
- Returns
- XMC_USBD_STATE_t.
- Description:
- Retrieves the current USB device state.
Power,active,speed and connection status data are retrieved.
- Note:
- Before calling this API, USB should be initialized with XMC_USBD_Init.
- Related APIs:
- XMC_USBD_Init()
XMC_USBD_STATUS_t XMC_USBD_DeviceSetAddress | ( | uint8_t | address, |
XMC_USBD_SET_ADDRESS_STAGE_t | stage | ||
) |
- Parameters
-
address The address to be set for the USB device . stage The device request stage-setup or status XMC_USBD_SET_ADDRESS_STAGE_t.
- Returns
- XMC_USBD_STATUS_t.
- Description:
- Sets the USB device address.
The device address is programmed in the DCFG register.
The address should be more than 0; as 0 is the default USB device address at the starting of enumeration. As part of enumeration, host sends the control request to the device to set the USB address; and in turn,
in the USB device event call back handler, user has to set the address using this API for the set address request.
The stage parameter should be XMC_USBD_SET_ADDRESS_STAGE_SETUP from the enum XMC_USBD_SET_ADDRESS_STAGE_t.
- Note:
- Before calling this API, USB should be initialized with XMC_USBD_Init () and connected to USB host using XMC_USBD_DeviceConnect()
- Related APIs:
- XMC_USBD_Init(), XMC_USBD_DeviceConnect()
void XMC_USBD_Disable | ( | void | ) |
- Parameters
-
None.
- Returns
- None.
- Description:
- Disables the USB module in the XMC controller.
It asserts the peripheral reset on USB0 module and disables the USB power.
- Related APIs:
- XMC_USBD_Enable()
void XMC_USBD_Enable | ( | void | ) |
- Parameters
-
None.
- Returns
- None.
- Description:
- Enables the USB module in the XMC controller.
It de-asserts the peripheral reset on USB0 module and enables the USB power.
- Note:
- This API is called inside the XMC_USBD_Init().
- Related APIs:
- XMC_USBD_Disable()
void XMC_USBD_EnableEventINEP | ( | uint32_t | event | ) |
- Parameters
-
event The single event or multiple events that need to be enabled.
- Returns
- None.
- Description:
- Enables the event or multiple events of the USB IN endpoints.
The multiple events can be selected by the bitwise OR operation of XMC_USBD_EVENT_IN_EP_t elements. It enables the event by programming DIEPMSK register.
- Note:
- This API is called inside the XMC_USBD_Init() to enable the IN EP interrupts.
- Related APIs:
- XMC_USBD_EnableEventOUTEP()
void XMC_USBD_EnableEventOUTEP | ( | uint32_t | event | ) |
- Parameters
-
event The single event or multiple events that need to be enabled.
- Returns
- None.
- Description:
- Enables the event or multiple events of the OUT endpoints.
The multiple events can be selected by the bitwise OR operation of XMC_USBD_EVENT_OUT_EP_t elements. It enables the event by programming DOEPMSK register.
- Note:
- This API is called inside the XMC_USBD_Init() to enable the OUT EP interrupts.
- Related APIs:
- XMC_USBD_EnableEventINEP()
XMC_USBD_STATUS_t XMC_USBD_EndpointAbort | ( | uint8_t | ep_addr | ) |
- Parameters
-
ep_addr The address of the USB endpoint, on which the data need to be aborted.
- Returns
- XMC_USBD_STATUS_t
- Description:
- Abort the transfer on endpoint ep_addr.
On any failure with the USB transmission user can reset the endpoint into default state and clear all assigned buffers, to start from a clean point. The endpoint will not be unconfigured or disabled.
- Related APIs:
- XMC_USBD_EndpointUnconfigure()
XMC_USBD_STATUS_t XMC_USBD_EndpointConfigure | ( | uint8_t | ep_addr, |
XMC_USBD_ENDPOINT_TYPE_t | ep_type, | ||
uint16_t | ep_max_packet_size | ||
) |
- Parameters
-
ep_addr The address of the USB endpoint, which needs to be configured. ep_type The XMC_USBD_ENDPOINT_TYPE_t. ep_max_packet_size The maximum packet size of endpoint in USB full speed.
- Returns
- XMC_USBD_STATUS_t.
- Description:
- Configures the USB endpoint.
The endpoint is configured by programming the DAINT,DIEPCTL and DOEPCTL registers.
Configures the EP type, FIFO number,maximum packet size, enables endpoint and sets the DATA0 PID. This function also initializes the internal buffer handling for the specified endpoint, but does not start any transfers.
As part of enumeration, host sends the control request to the device to set the configuration; and in turn,
in the USB device event call back handler, user has to set the configuration and configure the endpoints
required for the device.
- Note:
- This API should only be used as part of enumeration.
int32_t XMC_USBD_EndpointRead | ( | const uint8_t | ep_addr, |
uint8_t * | buffer, | ||
uint32_t | length | ||
) |
- Parameters
-
ep_addr The address of the USB OUT endpoint, from which data need to be read. buffer The pointer to the user buffer,in which data need to be received. length The number of bytes to be read from OUT EP.
- Returns
The actual number of bytes received.
- Description:
- Read length number of bytes from an OUT endpoint ep_addr.
If data has been received for this endpoint, it gets copied into the user buffer until its full or no data is left in the driver buffer.
- Note:
- For preparing the next OUT token, use XMC_USBD_EndpointReadStart() after XMC_USBD_EndpointRead().
- Related APIs:
- XMC_USBD_EndpointReadStart()
XMC_USBD_STATUS_t XMC_USBD_EndpointReadStart | ( | const uint8_t | ep_addr, |
uint32_t | size | ||
) |
- Parameters
-
ep_addr The address of the USB endpoint, from which data need to be read. size The number of bytes to be read.
- Returns
- XMC_USBD_STATUS_t.
- Description:
- Prepares an endpoint to receive OUT tokens from the USB host.
The selected endpoint gets configured, so that it receives the specified amount of data from the host. As part of streaming of OUT data, after reading the current OUT buffer using XMC_USBD_EndpointRead(),
user can prepare endpoint for the next OUT packet by using XMC_USBD_EndpointReadStart().
The registers DOEPDMA,DOEPTSIZ and DOEPCTL are programmed to start a new read request.
- Note:
- For the data received on OUT EP buffer, use XMC_USBD_EndpointRead().
- Related APIs:
- XMC_USBD_EndpointRead()
XMC_USBD_STATUS_t XMC_USBD_EndpointStall | ( | uint8_t | ep_addr, |
bool | stall | ||
) |
- Parameters
-
ep_addr The address of the USB endpoint, on which stall needs to be set or cleared. stall The boolean variable to decide on set or clear of stall on EP.
- Returns
- XMC_USBD_STATUS_t.
- Description:
- Set or Clear stall on the USB endpoint ep_addr, based on stall parameter.
By programming stall bit in the doepctl and diepctl, it sets or clears the stall on the endpoint. The endpoint can be stalled when a non supported request comes from the USB host. The XMC_USBD_EndpointStall() should be called with stall set to 0, in the clear feature standard request in the USB device event call back handler. *
- Note:
- The host should clear the stall set on the endpoint by sending the clear feature standard request on the non EP0 endpoints. On EP0, the stall will automatically gets cleared on the next control request.
- Related APIs:
- XMC_USBD_EndpointAbort()
XMC_USBD_STATUS_t XMC_USBD_EndpointUnconfigure | ( | uint8_t | ep_addr | ) |
- Parameters
-
ep_addr The address of the USB endpoint, which needs to be unconfigured.
- Returns
- XMC_USBD_STATUS_t.
- Description:
- Unconfigures the USB endpoint.
The endpoint is unconfigured by programming the DAINT,DIEPCTL and DOEPCTL registers.
Disables the endpoint, unassign the fifo, deactivate it and only send nacks.
Waits until the endpoint has finished operation and disables it. All (eventuallly) allocated buffers gets freed. Forces the endpoint to stop immediately, any pending transfers are killed(Can cause device reset).
- Related APIs:
- XMC_USBD_EndpointConfigure()
int32_t XMC_USBD_EndpointWrite | ( | const uint8_t | ep_addr, |
const uint8_t * | buffer, | ||
uint32_t | length | ||
) |
- Parameters
-
ep_addr The address of the USB IN endpoint, on which data should be sent. buffer The pointer to the data buffer, to write to the endpoint. length The number of bytes to be written to IN EP.
- Returns
The actual amount of data written to the endpoint buffer.
- Description:
- Write the length bytes of data to an IN endpoint ep_addr.
The User data gets copied into the driver buffer or will be send directly based on the buffer concept selected in the XMC_USBD_TRANSFER_MODE_t configuration.
Then the endpoint is set up to transfer the data to the host.
DIEPDMA,DIEPTSIZ and DIEPCTL registers are programmed to start the IN transfer.
- Related APIs:
- XMC_USBD_EndpointRead()
XMC_USBD_CAPABILITIES_t XMC_USBD_GetCapabilities | ( | void | ) |
- Parameters
-
None.
- Returns
- XMC_USBD_CAPABILITIES_t.
- Description:
- Retrieves the USB device capabilities of type XMC_USBD_CAPABILITIES_t
The USB device capabilities supported by the USBD driver, like power on/off, connect/disconnect, reset,suspend/resume,USB speed etc are retrieved.
It can be called after initializing the USB device to get the information on the USBD capabilities.
uint16_t XMC_USBD_GetFrameNumber | ( | void | ) |
- Parameters
-
None.
- Returns
- The 16 bit current USB frame number.
- Description:
- Read the current USB frame number.
* Reads the device status register (DSTS) and returns the SOFFN field.
XMC_USBD_STATUS_t XMC_USBD_Init | ( | XMC_USBD_t * | obj | ) |
- Parameters
-
obj The pointer to the USB device handle XMC_USBD_t.
- Returns
- XMC_USBD_STATUS_t The USB device status of type XMC_USBD_STATUS_t.
- Description:
- Initializes the USB device to get ready for connect to USB host.
Enables the USB module,sets the EP buffer sizes,registers the device and EP event call backs. Initializes the global,device and FIFO register base addresses. Configures the global AHB,enables the global interrupt and DMA by programming GAHBCFG register. Configures the USB in to device mode and enables the session request protocol by programming GUSBCFG register. Configures the USB device speed to full speed by programming DCFG register. Disconnects the USB device by programming DCTL register. Enables the USB common and device interrupts by programming GINTMSK register.
- Note:
- This API makes the USB device ready to connect to host.The user has to explicitly call the XMC_USBD_DeviceConnect() after the USB initialization to connect to USB host.
- Related APIs:
- XMC_USBD_DeviceConnect()
void XMC_USBD_IRQHandler | ( | const XMC_USBD_t *const | obj | ) |
- Parameters
-
obj The pointer to the USB device handle structure.
- Returns
- None.
- Description:
- USB device default IRQ handler.
USBD Peripheral LLD provides default implementation of ISR. The user needs to explicitly either use our default implementation or use its own one using the LLD APIs.
For example: XMC_USBD_t *obj; void USB0_0_IRQHandler(void) { XMC_USBD_IRQHandler(obj); }
- Note:
- The user should initialize the XMC USB device configuration structure before calling XMC_USBD_IRQHandler() in the actual USB0 IRQ handler.
uint32_t XMC_USBD_IsEnumDone | ( | void | ) |
- Parameters
-
None.
- Returns
- Returns 1, if the speed enumeration is done and 0 otherwise.
- Description:
- Gets the speed enumeration completion status of the USB device.
- Note:
- This should not be used for the actual USB enumeration completion status. For the actual USB enumeration status, the application layer should check for the completion of USB standard request 'Set configuration'.
XMC_USBD_STATUS_t XMC_USBD_Uninitialize | ( | void | ) |
- Parameters
-
None.
- Returns
- XMC_USBD_STATUS_t.
- Description:
- Uninitialises the USB device.
Disconnects the USB device by programming DCTL register and resets the XMC USB device data.
- Note:
- Once this API is called, USB device will not be accessible from host.
- Related APIs:
- XMC_USBD_Init()
Variable Documentation
const XMC_USBD_DRIVER_t Driver_USBD0 |
Defines the driver interface function table. To access the XMC device controller driver interface use this table of functions.
XMC_USBD_DEVICE_t xmc_device |
Defines the XMC USB device data The instance of XMC_USBD_DEVICE_t structure describing the XMC device.
Generated on Mon Aug 7 2017 11:33:57 for XMC Peripheral Library for XMC4000 Family by
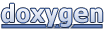