![]() |
XMC Peripheral Library for XMC4000 Family
2.1.16
|
Data Structures | |
struct | XMC_GPIO_CONFIG_t |
struct | XMC_GPIO_PORT_t |
Enumerations |
Functions | |
void | XMC_GPIO_DisableDigitalInput (XMC_GPIO_PORT_t *const port, const uint8_t pin) |
void | XMC_GPIO_DisablePowerSaveMode (XMC_GPIO_PORT_t *const port, const uint8_t pin) |
void | XMC_GPIO_EnableDigitalInput (XMC_GPIO_PORT_t *const port, const uint8_t pin) |
void | XMC_GPIO_EnablePowerSaveMode (XMC_GPIO_PORT_t *const port, const uint8_t pin) |
uint32_t | XMC_GPIO_GetInput (XMC_GPIO_PORT_t *const port, const uint8_t pin) |
void | XMC_GPIO_Init (XMC_GPIO_PORT_t *const port, const uint8_t pin, const XMC_GPIO_CONFIG_t *const config) |
void | XMC_GPIO_SetHardwareControl (XMC_GPIO_PORT_t *const port, const uint8_t pin, const XMC_GPIO_HWCTRL_t hwctrl) |
void | XMC_GPIO_SetMode (XMC_GPIO_PORT_t *const port, const uint8_t pin, const XMC_GPIO_MODE_t mode) |
void | XMC_GPIO_SetOutputHigh (XMC_GPIO_PORT_t *const port, const uint8_t pin) |
void | XMC_GPIO_SetOutputLevel (XMC_GPIO_PORT_t *const port, const uint8_t pin, const XMC_GPIO_OUTPUT_LEVEL_t level) |
void | XMC_GPIO_SetOutputLow (XMC_GPIO_PORT_t *const port, const uint8_t pin) |
void | XMC_GPIO_SetOutputStrength (XMC_GPIO_PORT_t *const port, const uint8_t pin, XMC_GPIO_OUTPUT_STRENGTH_t strength) |
void | XMC_GPIO_ToggleOutput (XMC_GPIO_PORT_t *const port, const uint8_t pin) |
Detailed Description
GPIO driver provide a generic and very flexible software interface for all standard digital I/O port pins. Each port slice has individual interfaces for the operation as General Purpose I/O and it further provides the connectivity to the on-chip periphery and the control for the pad characteristics.
The driver is divided into Input and Output mode.
Input mode features:
- Configuration structure XMC_GPIO_CONFIG_t and initialization function XMC_GPIO_Init()
- Allows the selection of weak pull-up or pull-down device. Configuration structure XMC_GPIO_MODE_t and function XMC_GPIO_SetMode()
Output mode features:
- Allows the selection of push pull/open drain and Alternate output. Configuration structure XMC_GPIO_MODE_t and function XMC_GPIO_SetMode()
- Allows the selection of pad driver strength. Configuration structure XMC_GPIO_OUTPUT_STRENGTH_t and function XMC_GPIO_SetOutputStrength()
- Allows the selection of initial output level. Configuration structure XMC_GPIO_OUTPUT_LEVEL_t and function XMC_GPIO_SetOutputLevel()
Enumeration Type Documentation
enum XMC_GPIO_HWCTRL_t |
Defines direct hardware control characteristics of the pin . Use type XMC_GPIO_HWCTRL_t for this enum.
enum XMC_GPIO_MODE_t |
Defines the direction and characteristics of a pin. Use type XMC_GPIO_MODE_t for this enum. For the operation with alternate functions, the port pins are directly connected to input or output functions of the on-chip periphery.
Defines output strength and slew rate of a pin. Use type XMC_GPIO_OUTPUT_STRENGTH_t for this enum.
Function Documentation
void XMC_GPIO_DisableDigitalInput | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_PDISC. pin port pin number.
- Returns
- None
- Related APIs:
- None
- Description:
- Disable digital input path for analog pins and configures Pn_PDISC register.This configuration is applicable only for analog port pins.
void XMC_GPIO_DisablePowerSaveMode | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_PPS. pin port pin number.
- Returns
- None
- Description:
- Disables pin power save mode and configures Pn_PPS register.This configuration is useful when the controller enters Deep Sleep mode. This configuration enables input Schmitt-Trigger and output driver stage(if pin is enabled power save mode previously). By default port pin does not react to power save mode request.
- Related APIs:
- XMC_GPIO_EnablePowerSaveMode()
- Note:
- Do not enable the Pin Power Save function for pins configured for Hardware Control (Pn_HWSEL.HWx != 00B). Doing so may result in an undefined behavior of the pin when the device enters the Deep Sleep state.
void XMC_GPIO_EnableDigitalInput | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_PDISC. pin port pin number.
- Returns
- None
- Related APIs:
- None
- Description:
- Enable digital input path for analog pins and configures Pn_PDISC register.This configuration is applicable only for analog port pins.
void XMC_GPIO_EnablePowerSaveMode | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_PPS. pin port pin number.
- Returns
- None
- Description:
- Enables pin power save mode and configures Pn_PPS register.This configuration is useful when the controller enters Deep Sleep mode.Port pin enabled with power save mode option are set to a defined state and the input Schmitt-Trigger as well as the output driver stage are switched off. By default port pin does not react to power save mode request.
- Related APIs:
- XMC_GPIO_DisablePowerSaveMode()
Note: Do not enable the Pin Power Save function for pins configured for Hardware Control (Pn_HWSEL.HWx != 00B). Doing so may result in an undefined behavior of the pin when the device enters the Deep Sleep state.
uint32_t XMC_GPIO_GetInput | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_IN. pin Port pin number.
- Returns
- uint32_t pin logic level status.
- Description:
- Reads the Pn_IN register and returns the current logical value at the GPIO pin.
- Related APIs:
- None
- Note:
- Prior to this api, user has to configure port pin to input mode using XMC_GPIO_SetMode().
void XMC_GPIO_Init | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin, | ||
const XMC_GPIO_CONFIG_t *const | config | ||
) |
- Parameters
-
port Constant pointer pointing to GPIO port, to access port registers like Pn_OUT,Pn_OMR,Pn_IOCR etc. pin Port pin number. config GPIO configuration data structure. Refer data structure XMC_GPIO_CONFIG_t for details.
- Returns
- None
- Description:
- Initializes input / output mode settings like, pull up / pull down devices,push pull /open drain, and pad driver mode. Also configures alternate function outputs and clears hardware port control for selected port and pin . It configures hardware registers Pn_IOCR,Pn_OUT,Pn_OMR,Pn_PDISC and Pn_PDR.
- Related APIs:
- None
- Note:
- This API is called in definition of DAVE_init by code generation and therefore should not be explicitly called for the normal operation. Use other APIs only after DAVE_init is called successfully (returns DAVE_STATUS_SUCCESS).
void XMC_GPIO_SetHardwareControl | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin, | ||
const XMC_GPIO_HWCTRL_t | hwctrl | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_HWSEL. pin port pin number. hwctrl direct hardware control selection. Refer XMC_GPIO_HWCTRL_t for valid values.
- Returns
- None
- Description:
- Selects direct hard ware control and configures Pn_HWSEL register.This configuration is useful for the port pins overlaid with peripheral functions for which the connected peripheral needs hardware control.
- Related APIs:
- None
- Note:
- Do not enable the Pin Power Save function for pins configured for Hardware Control (Pn_HWSEL.HWx != 00B). Doing so may result in an undefined behavior of the pin when the device enters the Deep Sleep state.
void XMC_GPIO_SetMode | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin, | ||
const XMC_GPIO_MODE_t | mode | ||
) |
- Parameters
-
port Constant pointer pointing to GPIO port, to access hardware register Pn_IOCR. pin Port pin number. mode input / output functionality selection. Refer XMC_GPIO_MODE_t for valid values.
- Returns
- None
- Description:
- Sets digital input and output driver functionality and characteristics of a GPIO port pin. It configures hardware registers Pn_IOCR. mode is initially configured during initialization in XMC_GPIO_Init(). Call this API to alter the port direction functionality as needed later in the program.
- Related APIs:
- None
void XMC_GPIO_SetOutputHigh | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_OMR. pin Port pin number.
- Returns
- None
- Description:
- Sets port pin output to high. It configures hardware registers Pn_OMR.
- Related APIs:
- XMC_GPIO_SetOutputLow()
- Note:
- Prior to this api, user has to configure port pin to output mode using XMC_GPIO_SetMode().
Register Pn_OMR is virtual and does not contain any flip-flop. A read action delivers the value of 0.
void XMC_GPIO_SetOutputLevel | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin, | ||
const XMC_GPIO_OUTPUT_LEVEL_t | level | ||
) |
- Parameters
-
port Constant pointer pointing to GPIO port, to access hardware register Pn_OMR. pin Port pin number. level output level selection. Refer XMC_GPIO_OUTPUT_LEVEL_t for valid values.
- Returns
- None
- Description:
- Set port pin output level to high or low.It configures hardware registers Pn_OMR.level is initially configured during initialization in XMC_GPIO_Init(). Call this API to alter output level as needed later in the program.
- Related APIs:
- XMC_GPIO_SetOutputHigh(), XMC_GPIO_SetOutputLow().
- Note:
- Prior to this api, user has to configure port pin to output mode using XMC_GPIO_SetMode().
void XMC_GPIO_SetOutputLow | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_OMR. pin port pin number.
- Returns
- None
- Description:
- Sets port pin output to low. It configures hardware registers Pn_OMR.
- Related APIs:>
- XMC_GPIO_SetOutputHigh()
- Note:
- Prior to this api, user has to configure port pin to output mode using XMC_GPIO_SetMode(). Register Pn_OMR is virtual and does not contain any flip-flop. A read action delivers the value of 0.
void XMC_GPIO_SetOutputStrength | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin, | ||
XMC_GPIO_OUTPUT_STRENGTH_t | strength | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_PDR. pin Port pin number. strength Output driver mode selection. Refer data structure XMC_GPIO_OUTPUT_STRENGTH_t for details.
- Returns
- None
- Description:
- Sets port pin output strength and slew rate. It configures hardware registers Pn_PDR. strength is initially configured during initialization in XMC_GPIO_Init(). Call this API to alter output driver mode as needed later in the program.
- Related APIs:
- None
- Note:
- Prior to this api, user has to configure port pin to output mode using XMC_GPIO_SetMode().
void XMC_GPIO_ToggleOutput | ( | XMC_GPIO_PORT_t *const | port, |
const uint8_t | pin | ||
) |
- Parameters
-
port constant pointer pointing to GPIO port, to access hardware register Pn_OMR. pin port pin number.
- Returns
- None
- Description:
- Configures port pin output to Toggle. It configures hardware registers Pn_OMR.
- Related APIs:
- XMC_GPIO_SetOutputHigh(), XMC_GPIO_SetOutputLow().
- Note:
- Prior to this api, user has to configure port pin to output mode using XMC_GPIO_SetMode(). Register Pn_OMR is virtual and does not contain any flip-flop. A read action delivers the value of 0.
Generated on Mon Aug 7 2017 11:33:57 for XMC Peripheral Library for XMC4000 Family by
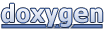