![]() |
XMC Peripheral Library for XMC4000 Family
2.1.16
|
Data Structures | |
struct | GPDMA_CH_t |
struct | XMC_DMA_CH_CONFIG_t |
struct | XMC_DMA_LLI_t |
struct | XMC_DMA_t |
Macros | |
#define | XMC_DMA0 ((XMC_DMA_t *)GPDMA0_CH0_BASE) |
#define | XMC_DMA1 ((XMC_DMA_t *)GPDMA1_CH0_BASE) |
Typedefs | |
typedef void(* | XMC_DMA_CH_EVENT_HANDLER_t) (XMC_DMA_CH_EVENT_t event) |
typedef XMC_DMA_LLI_t ** | XMC_DMA_LIST_t |
Enumerations |
Functions | |
void | XMC_DMA_CH_ClearDestinationPeripheralRequest (XMC_DMA_t *const dma, uint8_t channel) |
void | XMC_DMA_CH_ClearEventStatus (XMC_DMA_t *const dma, const uint8_t channel, const uint32_t event) |
void | XMC_DMA_CH_ClearSourcePeripheralRequest (XMC_DMA_t *const dma, uint8_t channel) |
void | XMC_DMA_CH_Disable (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_DisableDestinationAddressReload (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_DisableDestinationScatter (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_DisableEvent (XMC_DMA_t *const dma, const uint8_t channel, const uint32_t event) |
void | XMC_DMA_CH_DisableSourceAddressReload (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_DisableSourceGather (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_Enable (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_EnableDestinationAddressReload (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_EnableDestinationScatter (XMC_DMA_t *const dma, const uint8_t channel, uint32_t interval, uint16_t count) |
void | XMC_DMA_CH_EnableEvent (XMC_DMA_t *const dma, const uint8_t channel, const uint32_t event) |
void | XMC_DMA_CH_EnableSourceAddressReload (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_EnableSourceGather (XMC_DMA_t *const dma, const uint8_t channel, uint32_t interval, uint16_t count) |
uint32_t | XMC_DMA_CH_GetEventStatus (XMC_DMA_t *const dma, const uint8_t channel) |
XMC_DMA_CH_STATUS_t | XMC_DMA_CH_Init (XMC_DMA_t *const dma, const uint8_t channel, const XMC_DMA_CH_CONFIG_t *const config) |
bool | XMC_DMA_CH_IsEnabled (XMC_DMA_t *const dma, const uint8_t channel) |
bool | XMC_DMA_CH_IsSuspended (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_RequestLastMultiblockTransfer (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_Resume (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_SetBlockSize (XMC_DMA_t *const dma, const uint8_t channel, uint32_t block_size) |
void | XMC_DMA_CH_SetDestinationAddress (XMC_DMA_t *const dma, const uint8_t channel, uint32_t addr) |
void | XMC_DMA_CH_SetEventHandler (XMC_DMA_t *const dma, const uint8_t channel, XMC_DMA_CH_EVENT_HANDLER_t event_handler) |
void | XMC_DMA_CH_SetLinkedListPointer (XMC_DMA_t *const dma, const uint8_t channel, XMC_DMA_LLI_t *ll_ptr) |
void | XMC_DMA_CH_SetSourceAddress (XMC_DMA_t *const dma, const uint8_t channel, uint32_t addr) |
void | XMC_DMA_CH_Suspend (XMC_DMA_t *const dma, const uint8_t channel) |
void | XMC_DMA_CH_TriggerDestinationRequest (XMC_DMA_t *const dma, const uint8_t channel, const XMC_DMA_CH_TRANSACTION_TYPE_t type, bool last) |
void | XMC_DMA_CH_TriggerSourceRequest (XMC_DMA_t *const dma, const uint8_t channel, const XMC_DMA_CH_TRANSACTION_TYPE_t type, bool last) |
void | XMC_DMA_ClearOverrunStatus (XMC_DMA_t *const dma, const uint8_t line) |
void | XMC_DMA_ClearRequestLine (XMC_DMA_t *const dma, uint8_t line) |
void | XMC_DMA_Disable (XMC_DMA_t *const dma) |
void | XMC_DMA_DisableRequestLine (XMC_DMA_t *const dma, uint8_t line) |
void | XMC_DMA_Enable (XMC_DMA_t *const dma) |
void | XMC_DMA_EnableRequestLine (XMC_DMA_t *const dma, uint8_t line, uint8_t peripheral) |
uint32_t | XMC_DMA_GetChannelsBlockCompleteStatus (XMC_DMA_t *const dma) |
uint32_t | XMC_DMA_GetChannelsDestinationTransactionCompleteStatus (XMC_DMA_t *const dma) |
uint32_t | XMC_DMA_GetChannelsErrorStatus (XMC_DMA_t *const dma) |
uint32_t | XMC_DMA_GetChannelsSourceTransactionCompleteStatus (XMC_DMA_t *const dma) |
uint32_t | XMC_DMA_GetChannelsTransferCompleteStatus (XMC_DMA_t *const dma) |
uint32_t | XMC_DMA_GetEventStatus (XMC_DMA_t *const dma) |
bool | XMC_DMA_GetOverrunStatus (XMC_DMA_t *const dma, const uint8_t line) |
void | XMC_DMA_Init (XMC_DMA_t *const dma) |
void | XMC_DMA_IRQHandler (XMC_DMA_t *const dma) |
bool | XMC_DMA_IsEnabled (const XMC_DMA_t *const dma) |
Detailed Description
The GPDMA is a highly configurable DMA controller that allows high-speed data transfers between peripherals and memories. Complex data transfers can be done with minimal intervention of the processor, making CPU available for other operations.
GPDMA provides extensive support for XMC microcontroller peripherals like A/D, D/A converters and timers. Data transfers through communication interfaces (USIC) using the GPDMA increase efficiency and parallelism for real-time applications.
The DMA low level driver provides functions to configure and initialize the GPDMA hardware peripheral.
Macro Definition Documentation
#define XMC_DMA0 ((XMC_DMA_t *)GPDMA0_CH0_BASE) |
DMA module 0
#define XMC_DMA1 ((XMC_DMA_t *)GPDMA1_CH0_BASE) |
DMA module 1, only available in XMC45xx series
Typedef Documentation
typedef void(* XMC_DMA_CH_EVENT_HANDLER_t) (XMC_DMA_CH_EVENT_t event) |
DMA channel event handler
typedef XMC_DMA_LLI_t** XMC_DMA_LIST_t |
Type definition for a linked list pointer
Enumeration Type Documentation
enum XMC_DMA_CH_EVENT_t |
DMA events
DMA hardware handshaking interface Hardware handshaking available only if DMA is flow controller
DMA channel priorities
enum XMC_DMA_CH_STATUS_t |
DMA transfer flow modes
DMA transfer types
Function Documentation
void XMC_DMA_CH_ClearDestinationPeripheralRequest | ( | XMC_DMA_t *const | dma, |
uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The destination peripheral request for which DMA channel is to be cleared?
- Returns
- None
- Description:
- Clear destination peripheral request
- The function is used to clear the destination peripheral request for a given DMA channel.
void XMC_DMA_CH_ClearEventStatus | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
const uint32_t | event | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The channel for which the event(s) need(s) to be disabled event A valid GPDMA event (XMC_DMA_CH_EVENT_t) or a valid combination of logically OR'd GPDMA events
- Returns
- None
- Description:
- Clear GPDMA event status
- The following events are supported by the GPDMA peripheral:
1) Transfer complete event
2) Block transfer complete event
3) Source transaction complete event
4) Destination transaction complete event
5) DMA error event
- The function is used to clear the status of one (or more) of the aforementioned events. Typically, its use is in the GPDMA interrupt handler function. Once an event is detected, an appropriate callback function must run and the event status should be cleared.
void XMC_DMA_CH_ClearSourcePeripheralRequest | ( | XMC_DMA_t *const | dma, |
uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The source peripheral request for which DMA channel is to be cleared?
- Returns
- None
- Description:
- Clear source peripheral request
- The function is used to clear the source peripheral request for a given DMA channel.
void XMC_DMA_CH_Disable | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel should be disabled?
- Returns
- None
- Description:
- Disable a GPDMA channel
- The function resets the GPDMA's CHENREG register to disable a DMA channel.
void XMC_DMA_CH_DisableDestinationAddressReload | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The channel for which the destination address reload must be disabled
- Returns
- None
- Description:
- Disable destination address reload
- The destination DMA address can be automatically reloaded from its initial value at the end of every block in a multi-block transfer. To disable this feature, use this function.
void XMC_DMA_CH_DisableDestinationScatter | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The source gather for which DMA channel is to be disabled?
- Returns
- None
- Description:
- Disable source gather
- The function is used to disable the destination scatter feature in the GPDMA peripheral.
void XMC_DMA_CH_DisableEvent | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
const uint32_t | event | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The channel for which the event(s) need(s) to be disabled event A valid GPDMA event (XMC_DMA_CH_EVENT_t) or a valid combination of logically OR'd GPDMA events
- Returns
- None
- Description:
- Disable GPDMA event(s)
- The following events are supported by the GPDMA peripheral:
1) Transfer complete event
2) Block transfer complete event
3) Source transaction complete event
4) Destination transaction complete event
5) DMA error event
- The function can be used to disable one (or more) of the aforementioned events.
void XMC_DMA_CH_DisableSourceAddressReload | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The channel for which the source address reload must be disabled
- Returns
- None
- Description:
- Disable source address reload
- The source DMA address can be automatically reloaded from its initial value at the end of every block in a multi-block transfer. To disable this feature, use this function.
void XMC_DMA_CH_DisableSourceGather | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The source gather for which DMA channel is to be disabled?
- Returns
- None
- Description:
- Disable source gather
- The function is used to disable the source gather feature in the GPDMA peripheral.
void XMC_DMA_CH_Enable | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel should be enabled?
- Returns
- None
- Description:
- Enable a GPDMA channel
- The function sets the GPDMA's CHENREG register to enable a DMA channel. Please ensure that the GPDMA module itself is enabled before calling this function. See XMC_DMA_Enable() for details.
void XMC_DMA_CH_EnableDestinationAddressReload | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The channel for which the destination address must be reloaded
- Returns
- None
- Description:
- Enable source address reload
- The function is used to automatically reload the destination DMA address (from its initial value) at the end of every block in a multi-block transfer. The auto- reload will begin soon after the DMA channel initialization (configured for a multi-block transaction).
void XMC_DMA_CH_EnableDestinationScatter | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
uint32_t | interval, | ||
uint16_t | count | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel is used for destination scatter? interval Scatter interval count Scatter count
- Returns
- None
- Description:
- Enable destination scatter
- The function is used to enable the destination scatter feature in the GPDMA peripheral. The user must also specify the scatter count and interval. Once the configuration is successful, calling XMC_DMA_CH_EnableEvent() will initiate destination gather. This function is normally used in conjunction with source gather. Please see XMC_DMA_CH_EnableSourceGather() for additional information.
void XMC_DMA_CH_EnableEvent | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
const uint32_t | event | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The channel for which the event(s) need(s) to be enabled event A valid GPDMA event (XMC_DMA_CH_EVENT_t) or a valid combination of logically OR'd GPDMA events
- Returns
- None
- Description:
- Enable GPDMA event(s)
- The following events are supported by the GPDMA peripheral:
1) Transfer complete event
2) Block transfer complete event
3) Source transaction complete event
4) Destination transaction complete event
5) DMA error event
- The function can be used to enable one (or more) of the aforementioned events. Once the events have been enabled, XMC_DMA_CH_SetEventHandler() API can be used to set a callback function.
void XMC_DMA_CH_EnableSourceAddressReload | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The channel for which the source address must be reloaded
- Returns
- None
- Description:
- Enable source address reload
- The function is used to automatically reload the source DMA address (from its initial value) at the end of every block in a multi-block transfer. The auto- reload will begin soon after the DMA channel initialization (configured for a multi-block transaction).
void XMC_DMA_CH_EnableSourceGather | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
uint32_t | interval, | ||
uint16_t | count | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel is used for source gather? interval Gather interval count Gather count
- Returns
- None
- Description:
- Enable source gather
- The function is used to enable the source gather feature in the GPDMA peripheral. The user must also specify the gather count and interval. Once the configuration is successful, calling XMC_DMA_CH_EnableEvent() will initiate source gather. This function is normally used in conjunction with destination scatter. Please see XMC_DMA_CH_EnableDestinationScatter() for additional information.
uint32_t XMC_DMA_CH_GetEventStatus | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The channel for which the event(s) status must be obtained
- Returns
- Event status
- Description:
- Get GPDMA channel event status
- The function is used obtain the status of one (or more) of the aforementioned events. The return value may then be masked with any one of the following enumerations to obtain the status of individual DMA events.
- Transfer complete -> XMC_DMA_CH_EVENT_TRANSFER_COMPLETE
Block transfer complete -> XMC_DMA_CH_EVENT_BLOCK_TRANSFER_COMPLETE
Source transaction complete -> XMC_DMA_CH_EVENT_SRC_TRANSACTION_COMPLETE
Destination transaction complete -> XMC_DMA_CH_EVENT_DST_TRANSACTION_COMPLETE
DMA error event -> XMC_DMA_CH_EVENT_ERROR
- Typically, its use is in the GPDMA interrupt handler function. Once an event is detected, an appropriate callback function must run and the event status should be cleared.
XMC_DMA_CH_STATUS_t XMC_DMA_CH_Init | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
const XMC_DMA_CH_CONFIG_t *const | config | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The GPDMA channel (number) which needs to be initialized config A constant pointer to XMC_DMA_CH_CONFIG_t, pointing to a const channel configuration
- Returns
- XMC_DMA_CH_STATUS_t Initialization status
- Description:
- Initialize a GPDMA channel with provided channel configuration
- The function sets up the following channel configuration parameters for a GPDMA channel (specified by the parameter channel):
1) Source and destination addresses (and linked list address if requested)
2) Source and destination handshaking interface (hardware or software?)
3) Scatter/gather configuration
4) Source and destination peripheral request (DMA is the flow controller)
5) Transfer flow and type
- The function returns one of the following values:
1) In case the DMA channel is not enabled: XMC_DMA_CH_STATUS_BUSY
2) If the GPDMA module itself is not enabled: XMC_DMA_CH_STATUS_ERROR
3) If the configuration was successful: XMC_DMA_CH_STATUS_OK
- Once the initialization is successful, calling XMC_DMA_CH_Enable() will trigger a GPDMA transfer.
bool XMC_DMA_CH_IsEnabled | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel should be disabled?
- Returns
- bool
- Description:
- Check if a GPDMA channel is enabled
- The function reads the GPDMA's CHENREG register to check if a DMA channel is enabled or not. The function returns "true" is the requested channel is enabled, "false" otherwise.
bool XMC_DMA_CH_IsSuspended | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel should be checked for a suspended transfer?
- Returns
- bool
- Description:
- Check if a GPDMA
- The function reads the CH_SUSP bit of the GPDMA's GFGL register to check if a DMA transfer for the requested channel has been suspended. The function returns "true" if it detects a transfer suspension or "false" if it doesn't.
- Related API:
- XMC_DMA_CH_Suspend(), XMC_DMA_CH_Resume()
void XMC_DMA_CH_RequestLastMultiblockTransfer | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel is participating in a multi-block transfer?
- Returns
- None
- Description:
- Trigger the end of a multi-block transfer
- The function is used signal the end of multi-block DMA transfer. It clears the RELOAD_SRC and RELOAD_DST bits of the CFGL register to keep the source and destination addresses from getting updated. The function is typically used in an event handler to signal that the next block getting transferred is the last block in the transfer sequence.
void XMC_DMA_CH_Resume | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel should resume transfer?
- Returns
- None
- Description:
- Resume a GPDMA channel
- The function clears the CH_SUSP bit of the GPDMA's GFGL register to resume a DMA transfer. The function may be called after enabling the DMA channel. Please see XMC_DMA_CH_Enable() for more information.
- Related API:
- XMC_DMA_CH_Suspend()
void XMC_DMA_CH_SetBlockSize | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
uint32_t | block_size | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel A DMA channel block_size Transfer size [1-2048]
- Returns
- None
- Description:
- This function sets the block size of a transfer
- The function may be called after enabling the DMA channel. Please see XMC_DMA_CH_Enable() for more information.
void XMC_DMA_CH_SetDestinationAddress | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
uint32_t | addr | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel A DMA channel addr destination address
- Returns
- None
- Description:
- This function sets the destination address of the specified channel
- The function may be called after enabling the DMA channel. Please see XMC_DMA_CH_Enable() for more information.
- Related API:
- XMC_DMA_CH_SetSourceAddress()
void XMC_DMA_CH_SetEventHandler | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
XMC_DMA_CH_EVENT_HANDLER_t | event_handler | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel The channel for which the event handler is being registered event_handler The event handler which will be invoked when the DMA event occurs
- Returns
- None
- Description:
- Set a GPDMA event handler to service GPDMA events
- The function is used to register user callback functions for servicing DMA events. Call this function after enabling the GPDMA events (See XMC_DMA_CH_EnableEvent())
void XMC_DMA_CH_SetLinkedListPointer | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
XMC_DMA_LLI_t * | ll_ptr | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel A DMA channel ll_ptr linked list pointer
- Returns
- None
- Description:
- This function sets the linked list pointer
- The function may be called after enabling the DMA channel. Please see XMC_DMA_CH_Enable() for more information.
void XMC_DMA_CH_SetSourceAddress | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
uint32_t | addr | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel A DMA channel addr source address
- Returns
- None
- Description:
- This function sets the source address of the specified channel
- The function may be called after enabling the DMA channel. Please see XMC_DMA_CH_Enable() for more information.
- Related API:
- XMC_DMA_CH_SetDestinationAddress()
void XMC_DMA_CH_Suspend | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel should suspend transfer?
- Returns
- None
- Description:
- Suspend a GPDMA channel transfer
- The function sets the CH_SUSP bit of the GPDMA's GFGL register to initiate a DMA transfer suspend. The function may be called after enabling the DMA channel. Please see XMC_DMA_CH_Enable() for more information.
- Related API:
- XMC_DMA_CH_Resume()
void XMC_DMA_CH_TriggerDestinationRequest | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
const XMC_DMA_CH_TRANSACTION_TYPE_t | type, | ||
bool | last | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel is being used? type Transaction type: Single/burst mode last Specify "true" if it is the last destination request trigger, "false" otherwise
- Returns
- None
- Description:
- Trigger destination request
- The function can be used for GPDMA transfers involving a peripheral in software handshaking mode viz. Peripheral -> memory and peripheral -> peripheral.
- One would typically use this function in a (source) peripheral's event callback function to trigger the destination request.
void XMC_DMA_CH_TriggerSourceRequest | ( | XMC_DMA_t *const | dma, |
const uint8_t | channel, | ||
const XMC_DMA_CH_TRANSACTION_TYPE_t | type, | ||
bool | last | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address channel Which DMA channel is being used? type Transaction type: Single/burst mode last Specify "true" if it is the last source request trigger, "false" otherwise
- Returns
- None
- Description:
- Trigger source request
- The function can be used for GPDMA transfers involving a peripheral in software handshaking mode viz. Memory -> peripheral and peripheral -> peripheral.
- One would typically use this function in a (destination) peripheral's event callback function to trigger the source request.
void XMC_DMA_ClearOverrunStatus | ( | XMC_DMA_t *const | dma, |
const uint8_t | line | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address line The line for which the overrun status must be cleared
- Returns
- None
- Description:
- Clear overrun status of a DLR line
- The function clears the overrun status of a line by setting the corresponding line bit in the DLR's OVERCLR register.
void XMC_DMA_ClearRequestLine | ( | XMC_DMA_t *const | dma, |
uint8_t | line | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address line Which DLR (DMA line router) line should the function use?
- Returns
- None
- Description:
- Clear request line
- The function clears a DLR (DMA line router) request line.
void XMC_DMA_Disable | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- None
- Description:
- Disable the GPDMA peripheral
- The function asserts the GPDMA peripheral reset. In addition, it gates the GPDMA0 peripheral clock for all XMC4000 series of microcontrollers with an exception of the XMC4500 microcontroller. The XMC4500 doesn't support gating.
void XMC_DMA_DisableRequestLine | ( | XMC_DMA_t *const | dma, |
uint8_t | line | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address line Which DLR (DMA line router) line should the function use?
- Returns
- None
- Description:
- Disable request line
- The function disables a DLR (DMA line router) line by clearing appropriate bits in the LNEN register.
void XMC_DMA_Enable | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- None
- Description:
- Enable the GPDMA peripheral
- The function de-asserts the GPDMA peripheral reset. In addition, it un-gates the GPDMA0 peripheral clock for all XMC4000 series of microcontrollers with an exception of the XMC4500 microcontroller. The XMC4500 doesn't support gating.
void XMC_DMA_EnableRequestLine | ( | XMC_DMA_t *const | dma, |
uint8_t | line, | ||
uint8_t | peripheral | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address line Which DLR (DMA line router) line should the function use? peripheral Which hardware peripheral is the GPDMA communicating with?
- Returns
- None
- Description:
- Enable request line
- The function enables a DLR (DMA line router) line and selects a service request source, resulting in the trigger of a DMA transfer.
- Note:
- The DLR is used for a DMA transfer typically involving a peripheral; For example, the ADC peripheral may use the DLR in hardware handshaking mode to transfer ADC conversion values to a destination memory block.
uint32_t XMC_DMA_GetChannelsBlockCompleteStatus | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- uint32_t DMA block complete status
- Description:
- Get block transfer complete status
- The function returns GPDMA block transfer complete interrupt status.
uint32_t XMC_DMA_GetChannelsDestinationTransactionCompleteStatus | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- uint32_t DMA event status
- Description:
- Get destination transaction complete status
- The function returns the destination transaction complete interrupt status
- Note:
- If the destination peripheral is memory, the destination transaction complete interrupt is ignored.
uint32_t XMC_DMA_GetChannelsErrorStatus | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- uint32_t DMA error event status
- Description:
- Get DMA error event status
- The function returns error interrupt status.
uint32_t XMC_DMA_GetChannelsSourceTransactionCompleteStatus | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- uint32_t DMA event status
- Description:
- Get source transaction complete status
- The function returns the source transaction complete interrupt status.
- Note:
- If the source peripheral is memory, the source transaction complete interrupt is ignored.
uint32_t XMC_DMA_GetChannelsTransferCompleteStatus | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- uint32_t DMA transfer complete status
- Description:
- Get transfer complete status
- The function returns GPDMA transfer complete interrupt status.
uint32_t XMC_DMA_GetEventStatus | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- uint32_t DMA event status
- Description:
- Get DMA event status
- The function returns the collective (global) status of GPDMA events. The following lists the various DMA events and their corresponding enumeration. The return value of this function may then be masked with any one of the following enumerations to obtain the status of individual DMA events.
- Transfer complete -> XMC_DMA_CH_EVENT_TRANSFER_COMPLETE
Block transfer complete -> XMC_DMA_CH_EVENT_BLOCK_TRANSFER_COMPLETE
Source transaction complete -> XMC_DMA_CH_EVENT_SRC_TRANSACTION_COMPLETE
Destination transaction complete -> XMC_DMA_CH_EVENT_DST_TRANSACTION_COMPLETE
DMA error event -> XMC_DMA_CH_EVENT_ERROR
bool XMC_DMA_GetOverrunStatus | ( | XMC_DMA_t *const | dma, |
const uint8_t | line | ||
) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address line The line for which the overrun status is requested
- Returns
- bool "true" if overrun occured, "false" otherwise
- Description:
- Get overrun status of a DLR line
- The DLR module's OVERSTAT register keeps track of DMA service request overruns. Should an overrun occur, the bit corresponding to the used DLR line is set. The function simply reads this status and returns "true" if an overrun is detected It returns "false" if an overrun isn't registered.
void XMC_DMA_Init | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- None
- Description:
- Initialize the GPDMA peripheral
- The function initializes a prioritized list of DMA channels and enables the GPDMA peripheral.
void XMC_DMA_IRQHandler | ( | XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- None
- Description:
- Default GPDMA IRQ handler
- The function implements a default GPDMA IRQ handler. It can be used within the following (device specific) routines:
1) GPDMA0_0_IRQHandler
2) GPDMA1_0_IRQHandler
The function handles the enabled GPDMA events and runs the user callback function registered by the user to service the event. To register a callback function, see XMC_DMA_CH_SetEventHandler()
bool XMC_DMA_IsEnabled | ( | const XMC_DMA_t *const | dma | ) |
- Parameters
-
dma A constant pointer to XMC_DMA_t, pointing to the GPDMA base address
- Returns
- bool
- Description:
- Check if the GPDMA peripheral is enabled
- For the XMC4500 microcontroller, the function checks if the GPDMA module is asserted and returns "false" if it is. In addition, it also checks if the clock is gated for the other XMC4000 series of microcontrollers. It returns "true" if the peripheral is enabled.
Generated on Mon Aug 7 2017 11:33:57 for XMC Peripheral Library for XMC4000 Family by
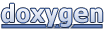