![]() |
XMC Peripheral Library for XMC4000 Family
2.1.16
|
Data Structures | |
struct | XMC_UART_CH_CONFIG_t |
Macros | |
#define | XMC_UART0_CH0 XMC_USIC0_CH0 |
#define | XMC_UART0_CH1 XMC_USIC0_CH1 |
#define | XMC_UART1_CH0 XMC_USIC1_CH0 |
#define | XMC_UART1_CH1 XMC_USIC1_CH1 |
#define | XMC_UART2_CH0 XMC_USIC2_CH0 |
#define | XMC_UART2_CH1 XMC_USIC2_CH1 |
Enumerations |
Detailed Description
The UART driver uses Universal Serial Interface Channel(USIC) module to implement UART protocol. It provides APIs to configure USIC channel for UART communication. The driver enables the user in getting the status of UART protocol events, configuring interrupt service requests, protocol related parameter configuration etc.
UART driver features:
- Configuration structure XMC_UART_CH_CONFIG_t and initialization function XMC_UART_CH_Init()
- Enumeration of events with their bit masks XMC_UART_CH_EVENT_t, XMC_UART_CH_STATUS_FLAG_t
- Allows the selection of input source for the DX0 input stage using the API XMC_UART_CH_SetInputSource()
- Allows configuration of baudrate using XMC_UART_CH_SetBaudrate() and configuration of data length using XMC_UART_CH_SetWordLength() and XMC_UART_CH_SetFrameLength()
- Provides the status of UART protocol events, XMC_UART_CH_GetStatusFlag()
- Allows transmission of data using XMC_UART_CH_Transmit() and gets received data using XMC_UART_CH_GetReceivedData()
Macro Definition Documentation
#define XMC_UART0_CH0 XMC_USIC0_CH0 |
USIC0 channel 0 base address
#define XMC_UART0_CH1 XMC_USIC0_CH1 |
USIC0 channel 1 base address
#define XMC_UART1_CH0 XMC_USIC1_CH0 |
USIC1 channel 0 base address
#define XMC_UART1_CH1 XMC_USIC1_CH1 |
USIC1 channel 1 base address
#define XMC_UART2_CH0 XMC_USIC2_CH0 |
USIC2 channel 0 base address
#define XMC_UART2_CH1 XMC_USIC2_CH1 |
USIC2 channel 1 base address
Enumeration Type Documentation
enum XMC_UART_CH_EVENT_t |
UART configuration events. The enums can be used for configuring events using the CCR register.
enum XMC_UART_CH_INPUT_t |
UART channel interrupt node pointers
UART portocol status. The enum values can be used for getting the status of UART channel.
enum XMC_UART_CH_STATUS_t |
Function Documentation
void XMC_UART_CH_ClearStatusFlag | ( | XMC_USIC_CH_t *const | channel, |
const uint32_t | flag | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
flag UART events to be cleared.
Range: Use XMC_UART_CH_STATUS_FLAG_t enumerations for event bitmasks. XMC_UART_CH_STATUS_FLAG_TRANSMISSION_IDLE, XMC_UART_CH_STATUS_FLAG_RECEPTION_IDLE, XMC_UART_CH_STATUS_FLAG_SYNCHRONIZATION_BREAK_DETECTED etc.
- Returns
- None
- Description
- Clears the status of UART channel events.
Multiple events can be combined using the bitwise OR operation and configured in one function call. XMC_UART_CH_STATUS_FLAG_t enumerates multiple event bitmasks. These enumerations can be used as input to the API. Events are cleared by setting the bitmask to the PSCR register.
- Related APIs:
- XMC_UART_CH_DisableEvent(), XMC_UART_CH_GetStatusFlag()
void XMC_UART_CH_DisableDataTransmission | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Returns
- None
- Description
- Disable data transmission.
Use this function in combination with XMC_UART_CH_EnableDataTransmission() to fill the FIFO and send the FIFO content without gaps in the transmission. FIFO is filled using XMC_USIC_CH_TXFIFO_PutData().
- Related APIs:
- XMC_UART_CH_EnableDataTransmission()
void XMC_UART_CH_DisableEvent | ( | XMC_USIC_CH_t *const | channel, |
const uint32_t | event | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
event Bitmask of events to disable. Use the type XMC_UART_CH_EVENT_t for naming events.
Range: XMC_UART_CH_EVENT_RECEIVE_START, XMC_UART_CH_EVENT_DATA_LOST, XMC_UART_CH_EVENT_TRANSMIT_SHIFT, XMC_UART_CH_EVENT_TRANSMIT_BUFFER, etc.
- Returns
- None
- Description
- Disables the interrupt events by clearing the bits in CCR register.
Multiple events can be combined using the bitwise OR operation and configured in one function call. XMC_UART_CH_EVENT_FLAG_t enumerates multiple event bitmasks. These enumerations can be used as input to the API.
- Related APIs:
- XMC_UART_CH_ClearStatusFlag(), XMC_UART_CH_EnableEvent()
void XMC_UART_CH_DisableInputDigitalFilter | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_INPUT_t | input | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
input UART channel input stage of type XMC_UART_CH_INPUT_t.
Range: XMC_UART_CH_INPUT_RXD (for DX0), XMC_UART_CH_INPUT_RXD1 (for DX3), XMC_UART_CH_INPUT_RXD2 (for DX5).
- Returns
- None
- Description
- Disables the digital filter for UART input stage.
- Related APIs:
- XMC_UART_CH_EnableInputDigitalFilter()
void XMC_UART_CH_DisableInputInversion | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_INPUT_t | input | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
input UART channel input stage of type XMC_UART_CH_INPUT_t.
Range: XMC_UART_CH_INPUT_RXD (for DX0), XMC_UART_CH_INPUT_RXD1 (for DX3), XMC_UART_CH_INPUT_RXD2 (for DX5).
- Returns
- None
- Description
- Disables input inversion for UART input data signal.
Resets the input data polarity for the UART input data signal.
- Related APIs:
- XMC_UART_CH_EnableInputInversion()
void XMC_UART_CH_DisableInputSync | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_INPUT_t | input | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
input UART channel input stage of type XMC_UART_CH_INPUT_t.
Range: XMC_UART_CH_INPUT_RXD (for DX0), XMC_UART_CH_INPUT_RXD1 (for DX3), XMC_UART_CH_INPUT_RXD2 (for DX5).
- Returns
- None
- Description
- Disables synchronous input for the UART input stage.
void XMC_UART_CH_EnableDataTransmission | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Returns
- None
- Description
- Enable data transmission.
Use this function in combination with XMC_UART_CH_DisableDataTransmission() to fill the FIFO and send the FIFO content without gaps in the transmission. FIFO is filled using XMC_USIC_CH_TXFIFO_PutData().
- Note
- If you need more control over the start of transmission use XMC_USIC_CH_SetStartTransmisionMode()
- Related APIs:
- XMC_UART_CH_DisableDataTransmission()
void XMC_UART_CH_EnableEvent | ( | XMC_USIC_CH_t *const | channel, |
const uint32_t | event | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
event Event bitmasks to enable. Use the type XMC_UART_CH_EVENT_t for naming events.
Range: XMC_UART_CH_EVENT_RECEIVE_START, XMC_UART_CH_EVENT_DATA_LOST, XMC_UART_CH_EVENT_TRANSMIT_SHIFT, XMC_UART_CH_EVENT_TRANSMIT_BUFFER, etc.
- Returns
- None
- Description
- Enables interrupt events for UART communication.
Multiple events can be combined using the bitwise OR operation and configured in one function call. XMC_UART_CH_EVENT_t enumerates multiple event bitmasks. These enumerations can be used as input to the API. Events are configured by setting bits in the CCR register.
void XMC_UART_CH_EnableInputDigitalFilter | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_INPUT_t | input | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
input UART channel input stage of type XMC_UART_CH_INPUT_t.
Range: XMC_UART_CH_INPUT_RXD (for DX0), XMC_UART_CH_INPUT_RXD1 (for DX3), XMC_UART_CH_INPUT_RXD2 (for DX5).
- Returns
- None
- Description
- Enables the digital filter for UART input stage.
- Related APIs:
- XMC_UART_CH_DisableInputDigitalFilter()
void XMC_UART_CH_EnableInputInversion | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_INPUT_t | input | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
input UART channel input stage of type XMC_UART_CH_INPUT_t.
Range: XMC_UART_CH_INPUT_RXD (for DX0), XMC_UART_CH_INPUT_RXD1 (for DX3), XMC_UART_CH_INPUT_RXD2 (for DX5).
- Returns
- None
- Description
- Enables input inversion for UART input data signal.
Polarity of the input source can be changed to provide inverted data input.
- Related APIs:
- XMC_UART_CH_DisableInputInversion()
void XMC_UART_CH_EnableInputSync | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_INPUT_t | input | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
input UART channel input stage of type XMC_UART_CH_INPUT_t.
Range: XMC_UART_CH_INPUT_RXD (for DX0), XMC_UART_CH_INPUT_RXD1 (for DX3), XMC_UART_CH_INPUT_RXD2 (for DX5).
- Returns
- None
- Description
- Enables synchronous input for the UART input stage.
uint16_t XMC_UART_CH_GetReceivedData | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Returns
- uint16_t Received data over UART communication channel.
- Description
- Provides one word of data received over UART communication channel.
Based on the channel configuration, data is either read from the receive FIFO or RBUF register. Before returning the value, there is no check for data validity. User should check the appropriate data receive flags(standard receive/alternative receive/FIFO standard receive/FIFO alternative receive) before executing the API. Reading from an empty receive FIFO can generate a receive error event.
- Related APIs:
- XMC_UART_CH_GetStatusFlag(), XMC_UART_CH_Transmit()
uint32_t XMC_UART_CH_GetStatusFlag | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Returns
- Status of UART channel events.
Range: Use XMC_UART_CH_STATUS_FLAG_t enumerations for event bitmasks. XMC_UART_CH_STATUS_FLAG_TRANSMISSION_IDLE, XMC_UART_CH_STATUS_FLAG_RECEPTION_IDLE, XMC_UART_CH_STATUS_FLAG_SYNCHRONIZATION_BREAK_DETECTED etc.
- Description
- Provides the status of UART channel events.
Status provided by the API represents the status of multiple events at their bit positions. The bitmasks can be obtained using the enumeration XMC_UART_CH_STATUS_FLAG_t. Event status is obtained by reading the register PSR_ASCMode.
- Related APIs:
- XMC_UART_CH_EnableEvent(), XMC_UART_CH_ClearStatusFlag()
void XMC_UART_CH_Init | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_CONFIG_t *const | config | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0, XMC_UART1_CH1,XMC_UART2_CH0, XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
config Constant pointer to UART configuration structure of type XMC_UART_CH_CONFIG_t.
- Returns
- XMC_UART_CH_STATUS_t Status of initializing the USIC channel for UART protocol.
Range: XMC_UART_CH_STATUS_OK if initialization is successful.
XMC_UART_CH_STATUS_ERROR if configuration of baudrate failed.
- Description
- Initializes the USIC channel for UART protocol.
During the initialization, USIC channel is enabled, baudrate is configured with the defined oversampling value in the intialization structure. If the oversampling value is set to 0 in the structure, the default oversampling of 16 is considered. Sampling point for each symbol is configured at the half of sampling period. Symbol value is decided by the majority decision among 3 samples. Word length is configured with the number of data bits. If the value of frame_length is 0, then USIC channel frame length is set to the same value as word length. If frame_length is greater than 0, it is set as the USIC channel frame length. Parity mode is set to the value configured for parity_mode. The USIC channel should be set to UART mode by calling the XMC_UART_CH_Start() API after the initialization.
- Related APIs:
- XMC_UART_CH_Start(), XMC_UART_CH_Stop(), XMC_UART_CH_Transmit()
void XMC_UART_CH_SelectInterruptNodePointer | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_INTERRUPT_NODE_POINTER_t | interrupt_node, | ||
const uint32_t | service_request | ||
) |
- Parameters
-
channel Pointer to USIC channel handler of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
interrupt_node Interrupt node pointer to be configured.
Range: XMC_UART_CH_INTERRUPT_NODE_POINTER_TRANSMIT_SHIFT, XMC_UART_CH_INTERRUPT_NODE_POINTER_TRANSMIT_BUFFER etc.service_request Service request number.
Range: 0 to 5.
- Returns
- None
- Description
- Sets the interrupt node for USIC channel events.
For an event to generate interrupt, node pointer should be configured with service request(SR0, SR1..SR5). The NVIC node gets linked to the interrupt event by doing so.
Note: NVIC node should be separately enabled to generate the interrupt.
- Related APIs:
- XMC_UART_CH_EnableEvent()
XMC_UART_CH_STATUS_t XMC_UART_CH_SetBaudrate | ( | XMC_USIC_CH_t *const | channel, |
uint32_t | rate, | ||
uint32_t | oversampling | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1 ,XMC_UART1_CH0, XMC_UART1_CH1, XMC_UART2_CH0, XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
rate Desired baudrate.
Range: minimum value = 100, maximum value depends on the peripheral clock frequency
and oversampling. Maximum baudrate can be derived using the formula: (fperiph * 1023)/(1024 * oversampling)oversampling Required oversampling. The value indicates the number of time quanta for one symbol of data.
This can be related to the number of samples for each logic state of the data signal.
Range: 4 to 32. Value should be chosen based on the protocol used.
- Returns
- XMC_UART_CH_STATUS_t Status indicating the baudrate configuration.
Range: XMC_USIC_CH_STATUS_OK if baudrate is successfully configured, XMC_USIC_CH_STATUS_ERROR if desired baudrate or oversampling is invalid.
- Description:
- Sets the bus speed in bits per second.
Derives the values of STEP and PDIV to arrive at the optimum realistic speed possible. oversampling is the number of samples to be taken for each symbol of UART protocol. Default oversampling of 16 is considered if the input oversampling is less than 4. It is recommended to keep a minimum oversampling of 4 for UART.
- Related APIs:
- XMC_UART_CH_Init(), XMC_UART_CH_Stop()
void XMC_UART_CH_SetFrameLength | ( | XMC_USIC_CH_t *const | channel, |
const uint8_t | frame_length | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
frame_length Number of data bits in each UART frame.
Range: minimum= 1, maximum= 64.
- Returns
- None
- Description
- Sets the number of data bits for UART communication.
The frame length is configured by setting the input value to SCTR register. The value of frame_length will be decremented by 1, before setting it to the register. Frame length should not be set to 64 for UART communication.
- Related APIs:
- XMC_UART_CH_SetWordLength()
void XMC_UART_CH_SetInputSamplingFreq | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_INPUT_t | input, | ||
const XMC_UART_CH_INPUT_SAMPLING_FREQ_t | sampling_freq | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
input UART channel input stage of type XMC_UART_CH_INPUT_t.
Range: XMC_UART_CH_INPUT_RXD (for DX0), XMC_UART_CH_INPUT_RXD1 (for DX3), XMC_UART_CH_INPUT_RXD2 (for DX5).sampling_freq Input sampling frequency.
Range: XMC_UART_CH_INPUT_SAMPLING_FREQ_FPERIPH, XMC_UART_CH_INPUT_SAMPLING_FREQ_FRACTIONAL_DIVIDER.
- Returns
- None
- Description
- Sets the sampling frequency for the UART input stage.
void XMC_UART_CH_SetInputSource | ( | XMC_USIC_CH_t *const | channel, |
const XMC_UART_CH_INPUT_t | input, | ||
const uint8_t | source | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
input UART channel input stage of type XMC_UART_CH_INPUT_t.
Range: XMC_UART_CH_INPUT_RXD (for DX0), XMC_UART_CH_INPUT_RXD1 (for DX3), XMC_UART_CH_INPUT_RXD2 (for DX5).source Input source select for the input stage. The table provided below maps the decimal value with the input source. 0 DXnA 1 DXnB 2 DXnC 3 DXnD 4 DXnE 5 DXnF 6 DXnG 7 Always 1
- Returns
- None
- Description
- Sets input soource for the UART communication.
It is used for configuring the input stage for data reception. Selects the input data signal source among DXnA, DXnB.. DXnG for the input stage. The API can be used for the input stages DX0, DX3 and DX5.
- Related APIs:
- XMC_UART_CH_EnableInputInversion()
void XMC_UART_CH_SetInterruptNodePointer | ( | XMC_USIC_CH_t *const | channel, |
const uint8_t | service_request | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
service_request Service request number for generating protocol interrupts.
Range: 0 to 5.
- Returns
- None
- Description
- Sets the interrupt node for UART channel protocol events.
For all the protocol events enlisted in the enumeration XMC_UART_CH_EVENT_t, one common interrupt gets generated. The service request connects the interrupt node to the UART protocol events. Note: NVIC node should be separately enabled to generate the interrupt.
- Related APIs:
- XMC_UART_CH_EnableEvent()
void XMC_UART_CH_SetPulseLength | ( | XMC_USIC_CH_t *const | channel, |
const uint8_t | pulse_length | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
pulse_length Length of the zero pulse in number of time quanta.
Range: 0 to 7.
- Returns
- None
- Description
- Sets the length of zero pulse in number of time quanta. Value 0 indicates one time quanta.
Maximum possible is 8 time quanta with the value configured as 7.
The value is set to PCR_ASCMode register.
void XMC_UART_CH_SetSamplePoint | ( | XMC_USIC_CH_t *const | channel, |
const uint32_t | sample_point | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
sample_point Sample point among the number of samples.
Range: minimum= 0, maximum= oversampling (DCTQ).
- Returns
- None
- Description
- Sets the sample point among the multiple samples for each UART symbol.
The sample point is the one sample among number of samples set as oversampling. The value should be less than the oversampling value. XMC_UART_CH_Init() sets the sample point to the sample at the centre. For example if the oversampling is 16, then the sample point is set to 9.
void XMC_UART_CH_SetWordLength | ( | XMC_USIC_CH_t *const | channel, |
const uint8_t | word_length | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
word_length Data word length.
Range: minimum= 1, maximum= 16.
- Returns
- None
- Description
- Sets the data word length in number of bits.
Word length can range from 1 to 16. It indicates the number of data bits in a data word. The value of word_length will be decremented by 1 before setting the value to SCTR register. If the UART data bits is more than 16, then the frame length should be set to the actual number of bits and word length should be configured with the number of bits expected in each transaction. For example, if number of data bits for UART communication is 20 bits, then the frame length should be set as 20. Word length can be set based on the transmit and receive handling. If data is stored as 8bit array, then the word length can be set to 8. In this case, a full message of UART data should be transmitted/ received as 3 data words.
- Related APIs:
- XMC_UART_CH_SetFrameLength()
void XMC_UART_CH_Start | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Returns
- None
- Description
- Sets the USIC channel operation mode to UART mode.
CCR register bitfield Mode is set to 2(UART mode). This API should be called after configuring the USIC channel. Transmission and reception can happen only when the UART mode is set. This is an inline function.
- Related APIs:
- XMC_UART_CH_Stop(), XMC_UART_CH_Transmit()
XMC_UART_CH_STATUS_t XMC_UART_CH_Stop | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Returns
- XMC_UART_CH_STATUS_t Status to indicate if the communication channel is stopped successfully.
XMC_UART_CH_STATUS_OK if the communication channel is stopped. XMC_UART_CH_STATUS_BUSY if the communication channel is busy.
- Description
- Stops the UART communication.
CCR register bitfield Mode is reset. This disables the communication. Before starting the communication again, the channel has to be reconfigured.
- Related APIs:
- XMC_UART_CH_Init()
void XMC_UART_CH_Transmit | ( | XMC_USIC_CH_t *const | channel, |
const uint16_t | data | ||
) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
data Data to be transmitted.
Range: 16 bit unsigned data within the range 0 to 65535. Actual size of data transmitted depends on the configured number of bits for the UART protocol in the register SCTR.
- Returns
- None
- Description
- Transmits data over serial communication channel using UART protocol.
Based on the channel configuration, data is either put to the transmit FIFO or to TBUF register. Before putting data to TBUF, the API waits for TBUF to finish shifting its contents to shift register. So user can continuously execute the API without checking for TBUF busy status. Based on the number of data bits configured, the lower significant bits will be extracted for transmission.
Note: When FIFO is not configured, the API waits for the TBUF to be available. This makes the execution a blocking call.
- Related APIs:
- XMC_UART_CH_GetReceivedData()
void XMC_UART_CH_TriggerServiceRequest | ( | XMC_USIC_CH_t *const | channel, |
const uint32_t | service_request_line | ||
) |
- Parameters
-
channel Pointer to USIC channel handler of type XMC_USIC_CH_t
Range: XMC_UART0_CH0, XMC_UART0_CH1,XMC_UART1_CH0,XMC_UART1_CH1,XMC_UART2_CH0,XMC_UART2_CH1
- Note
- Availability of UART1 and UART2 depends on device selection
- Parameters
-
service_request_line service request number of the event to be triggered.
Range: 0 to 5.
- Returns
- None
- Description
- Trigger a UART interrupt service request.
When the UART service request is triggered, the NVIC interrupt associated with it will be generated if enabled.
- Related APIs:
- XMC_UART_CH_SelectInterruptNodePointer()
Generated on Mon Aug 7 2017 11:33:57 for XMC Peripheral Library for XMC4000 Family by
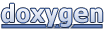