![]() |
XMC Peripheral Library for XMC4000 Family
2.1.16
|
Data Structures | |
struct | XMC_I2C_CH_CONFIG_t |
Macros | |
#define | XMC_I2C0_CH0 XMC_USIC0_CH0 |
#define | XMC_I2C0_CH1 XMC_USIC0_CH1 |
#define | XMC_I2C1_CH0 XMC_USIC1_CH0 |
#define | XMC_I2C1_CH1 XMC_USIC1_CH1 |
#define | XMC_I2C2_CH0 XMC_USIC2_CH0 |
#define | XMC_I2C2_CH1 XMC_USIC2_CH1 |
#define | XMC_I2C_10BIT_ADDR_GROUP (0x7800U) |
Enumerations |
Detailed Description
USIC IIC Features:
- Two-wire interface, with one line for shift clock transfer and synchronization (shift clock SCL), the other one for the data transfer (shift data SDA)
- Communication in standard mode (100 kBit/s) or in fast mode (up to 400 kBit/s)
- Support of 7-bit addressing, as well as 10-bit addressing
- Master mode operation, where the IIC controls the bus transactions and provides the clock signal.
- Slave mode operation, where an external master controls the bus transactions and provides the clock signal.
- Multi-master mode operation, where several masters can be connected to the bus and bus arbitration can take place, i.e. the IIC module can be master or slave.
The master/slave operation of an IIC bus participant can change from frame to frame.
- Efficient frame handling (low software effort), also allowing DMA transfers
- Powerful interrupt handling due to multitude of indication flags
Macro Definition Documentation
#define XMC_I2C0_CH0 XMC_USIC0_CH0 |
USIC0 channel 0 base address
#define XMC_I2C0_CH1 XMC_USIC0_CH1 |
USIC0 channel 1 base address
#define XMC_I2C1_CH0 XMC_USIC1_CH0 |
USIC1 channel 0 base address
#define XMC_I2C1_CH1 XMC_USIC1_CH1 |
USIC1 channel 1 base address
#define XMC_I2C2_CH0 XMC_USIC2_CH0 |
USIC2 channel 0 base address
#define XMC_I2C2_CH1 XMC_USIC2_CH1 |
USIC2 channel 1 base address
#define XMC_I2C_10BIT_ADDR_GROUP (0x7800U) |
Value to verify the address is 10-bit or not
Enumeration Type Documentation
enum XMC_I2C_CH_CMD_t |
enum XMC_I2C_CH_EVENT_t |
I2C events.
enum XMC_I2C_CH_INPUT_t |
I2C channel interrupt node pointers
I2C receiver status. The received data byte is available at the bit positions RBUF[7:0], whereas the additional information is monitored at the bit positions RBUF[12:8].
I2C status.
enum XMC_I2C_CH_STATUS_t |
Function Documentation
void XMC_I2C_CH_ClearStatusFlag | ( | XMC_USIC_CH_t *const | channel, |
uint32_t | flag | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t flag Status flag
- Returns
- None
- Description:
- Clears the status flag of I2C channel by setting the input parameter flag in PSCR register.
- Related APIs:
- XMC_I2C_CH_GetStatusFlag()
void XMC_I2C_CH_ConfigExternalInputSignalToBRG | ( | XMC_USIC_CH_t *const | channel, |
const uint16_t | pdiv, | ||
const uint32_t | oversampling, | ||
const XMC_USIC_CH_INPUT_COMBINATION_MODE_t | combination_mode | ||
) |
- Parameters
-
channel Pointer to USIC channel handler of type XMC_USIC_CH_t
Range: XMC_I2C0_CH0, XMC_I2C0_CH1,XMC_I2C1_CH0,XMC_I2C1_CH1,XMC_I2C2_CH0,XMC_I2C2_CH1
- Note
- Availability of I2C1 and I2C2 depends on device selection
- Parameters
-
pdiv Desired divider for the external frequency input. Range: minimum value = 1, maximum value = 1024
oversampling Required oversampling. The value indicates the number of time quanta for one symbol of data.
This can be related to the number of samples for each logic state of the data signal.
Range: 1 to 32. Value should be chosen based on the protocol used.combination_mode USIC channel input combination mode
- Returns
- None
- Description
- Enables the external frequency input for the Baudrate Generator and configures the divider, oversampling and the combination mode of the USIC channel.
void XMC_I2C_CH_DisableAcknowledgeAddress0 | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- None
- Description:
- This bit defines that slave device should not be sensitive to the slave address 00H.
- Related APIs:
- XMC_I2C_CH_EnableAcknowledgeAddress0()
void XMC_I2C_CH_DisableDataTransmission | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_I2C0_CH0, XMC_I2C0_CH1,XMC_I2C1_CH0,XMC_I2C1_CH1,XMC_I2C2_CH0,XMC_I2C2_CH1
- Note
- Availability of I2C1 and I2C2 depends on device selection
- Returns
- None
- Description
- Disable data transmission.
Use this function in combination with XMC_I2C_CH_EnableDataTransmission() to fill the FIFO and send the FIFO content without gaps in the transmission. FIFO is filled using XMC_USIC_CH_TXFIFO_PutData().
- Related APIs:
- XMC_I2C_CH_EnableDataTransmission()
void XMC_I2C_CH_DisableEvent | ( | XMC_USIC_CH_t *const | channel, |
uint32_t | event | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t event ORed values of XMC_I2C_CH_EVENT_t enum
- Returns
- None
- Description:
- Disables the input parameter XMC_I2C_CH_EVENT_t event using PCR_IICMode register.
- Related APIs:
- XMC_I2C_CH_EnableEvent()
void XMC_I2C_CH_EnableAcknowledgeAddress0 | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- None
- Description:
- Retrieves the status byte of I2C channel using PSR_IICMode register.
- Related APIs:
- XMC_I2C_CH_DisableAcknowledgeAddress0()
void XMC_I2C_CH_EnableDataTransmission | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel handle of type XMC_USIC_CH_t
Range: XMC_I2C0_CH0, XMC_I2C0_CH1,XMC_I2C1_CH0,XMC_I2C1_CH1,XMC_I2C2_CH0,XMC_I2C2_CH1
- Note
- Availability of I2C1 and I2C2 depends on device selection
- Returns
- None
- Description
- Enable data transmission.
Use this function in combination with XMC_I2C_CH_DisableDataTransmission() to fill the FIFO and send the FIFO content without gaps in the transmission. FIFO is filled using XMC_USIC_CH_TXFIFO_PutData().
- Note
- If you need more control over the start of transmission use XMC_USIC_CH_SetStartTransmisionMode()
- Related APIs:
- XMC_I2C_CH_DisableDataTransmission()
void XMC_I2C_CH_EnableEvent | ( | XMC_USIC_CH_t *const | channel, |
uint32_t | event | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t event ORed values of XMC_I2C_CH_EVENT_t enum
- Returns
- None
- Description:
- Enables the input parameter XMC_I2C_CH_EVENT_t event using PCR_IICMode register.
- Related APIs:
- XMC_I2C_CH_DisableEvent()
uint8_t XMC_I2C_CH_GetReceivedData | ( | const XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- uint8_t OUTR/RBUF register data
- Description:
- Reads the data from I2C channel.
- Data is read by using OUTR/RBUF register based on FIFO/non-FIFO modes.
- Related APIs:
- XMC_I2C_CH_MasterTransmit()
uint8_t XMC_I2C_CH_GetReceiverStatusFlag | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- uint8_t Receiver status flag
- Description:
- Gets the receiver status of I2C channel using RBUF register of bits 8-12 which gives information about receiver status.
- Related APIs:
- XMC_I2C_CH_MasterTransmit()
uint16_t XMC_I2C_CH_GetSlaveAddress | ( | const XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel handler of type XMC_USIC_CH_t
- Returns
- uint16_t Slave address
- Description:
- Gets the I2C channel slave address.
- Returns the address using PCR_IICMode register by checking if it is in 10-bit address group or 7-bit address group.
(If first five bits of address are assigned with 0xF0, then address mode is considered as 10-bit mode otherwise it is 7-bit mode)
- Note
- A 7-bit address will include an additional bit at the LSB. For example, address 0x05 will be returned as 0x0a. 10-bit address will not include the 10-bit address identifier 0b11110xx at the most signifcant bits.
- Related APIs:
- XMC_I2C_CH_SetSlaveAddress()
uint32_t XMC_I2C_CH_GetStatusFlag | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- uint32_t Status byte
- Description:
- Retrieves the status byte of I2C channel using PSR_IICMode register.
- Related APIs:
- XMC_I2C_CH_ClearStatusFlag()
void XMC_I2C_CH_Init | ( | XMC_USIC_CH_t *const | channel, |
const XMC_I2C_CH_CONFIG_t *const | config | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t config Constant pointer to I2C channel config structure of type XMC_I2C_CH_CONFIG_t
- Returns
- None
- Description:
- Initializes the I2C channel.
- Configures the data format in SCTR register. Sets the slave address, baud rate. Enables transmit data valid, clears status flags and disables parity generation.
- Related APIs:
- XMC_USIC_CH_Enable()
void XMC_I2C_CH_MasterReceiveAck | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- None
- Description:
- Sends the Ack request from I2C master channel.
- Reads the transmit buffer status is busy or not and thereby updates IN/TBUF register based on FIFO/non-FIFO modes using Master Receive Ack command.
- Related APIs:
- XMC_I2C_CH_MasterTransmit()
void XMC_I2C_CH_MasterReceiveNack | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- None
- Description:
- Sends the Nack request from I2C master channel.
- Reads the transmit buffer status is busy or not and thereby updates IN/TBUF register based on FIFO/non-FIFO modes using Master Receive Nack command.
- Related APIs:
- XMC_I2C_CH_MasterTransmit()
void XMC_I2C_CH_MasterRepeatedStart | ( | XMC_USIC_CH_t *const | channel, |
const uint16_t | addr, | ||
const XMC_I2C_CH_CMD_t | command | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t addr I2C master address command read/write command
- Returns
- None
- Description:
- Sends the repeated start condition from I2C master channel.
- Sends the repeated start condition with read/write command by updating IN/TBUF register based on FIFO/non-FIFO modes.
- Note
- Address(addr) should reserve an additional bit at the LSB for read/write indication. For example, address 0x05 should be provided as 0x0a. If the address is 10-bit, only most significant bits with the 10-bit identifier should be sent using this function. For example, if the 10-bit address is 0x305, the address should be provided as 0xf6(prepend with 0b11110, upper two bits of address 0b11, followed by 1-bit field for read/write).
void XMC_I2C_CH_MasterStart | ( | XMC_USIC_CH_t *const | channel, |
const uint16_t | addr, | ||
const XMC_I2C_CH_CMD_t | command | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t addr I2C master address command read/write command
- Returns
- None
- Description:
- Starts the I2C master channel.
- Sends the Start condition with read/write command by updating IN/TBUF register based on FIFO/non-FIFO modes.
- Note
- Address(addr) should reserve an additional bit at the LSB for read/write indication. For example, address 0x05 should be provided as 0x0a. If the address is 10-bit, only most significant bits with the 10-bit identifier should be sent using this function. For example, if the 10-bit address is 0x305, the address should be provided as 0xf6(prepend with 0b11110, upper two bits of address 0b11, followed by 1-bit field for read/write).
void XMC_I2C_CH_MasterStop | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- None
- Description:
- Stops the I2C master channel.
- Reads the transmit buffer status is busy or not and thereby updates IN/TBUF register based on FIFO/non-FIFO modes using Stop command.
void XMC_I2C_CH_MasterTransmit | ( | XMC_USIC_CH_t *const | channel, |
const uint8_t | data | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t data data to transmit from I2C channel
- Returns
- None
- Description:
- Transmit the data from the I2C master channel.
- Reads the transmit buffer status is busy or not and thereby updates IN/TBUF register based on FIFO/non-FIFO modes using Master Send command.
- Related APIs:
- XMC_USIC_CH_GetTransmitBufferStatus()
void XMC_I2C_CH_SelectInterruptNodePointer | ( | XMC_USIC_CH_t *const | channel, |
const XMC_I2C_CH_INTERRUPT_NODE_POINTER_t | interrupt_node, | ||
const uint32_t | service_request | ||
) |
- Parameters
-
channel Pointer to USIC channel handler of type XMC_USIC_CH_t
Range: XMC_I2C0_CH0, XMC_I2C0_CH1,XMC_I2C1_CH0,XMC_I2C1_CH1,XMC_I2C2_CH0,XMC_I2C2_CH1
- Note
- Availability of I2C1 and I2C2 depends on device selection
- Parameters
-
interrupt_node Interrupt node pointer to be configured.
Range: XMC_I2C_CH_INTERRUPT_NODE_POINTER_TRANSMIT_SHIFT, XMC_I2C_CH_INTERRUPT_NODE_POINTER_TRANSMIT_BUFFER etc.service_request Service request number.
Range: 0 to 5.
- Returns
- None
- Description
- Sets the interrupt node for USIC channel events.
For an event to generate interrupt, node pointer should be configured with service request(SR0, SR1..SR5). The NVIC node gets linked to the interrupt event by doing so.
Note: NVIC node should be separately enabled to generate the interrupt.
- Related APIs:
- XMC_I2C_CH_EnableEvent()
XMC_I2C_CH_STATUS_t XMC_I2C_CH_SetBaudrate | ( | XMC_USIC_CH_t *const | channel, |
const uint32_t | rate | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t rate baud rate of I2C channel
- Returns
- None
- Description:
- Sets the rate of I2C channel.
- Note:
- Standard over sampling is considered if rate <= 100KHz and fast over sampling is considered if rate > 100KHz.
- Related APIs:
- XMC_USIC_CH_SetBaudrate()
void XMC_I2C_CH_SetInputSource | ( | XMC_USIC_CH_t *const | channel, |
const XMC_I2C_CH_INPUT_t | input, | ||
const uint8_t | source | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t input I2C channel input stage of type XMC_I2C_CH_INPUT_t source Input source select for the input stage(0->DX0A, 1->DX1A, .. 7->DX7G)
- Returns
- None
- Description:
- Sets the input source for I2C channel.
Defines the input stage for the corresponding input line.
- Note
- After configuring the input source for corresponding channel, interrupt node pointer is set.
- Related APIs:
- XMC_USIC_CH_SetInptSource(), XMC_USIC_CH_SetInterruptNodePointer()
void XMC_I2C_CH_SetInterruptNodePointer | ( | XMC_USIC_CH_t *const | channel, |
const uint8_t | service_request | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t service_request Service request number in the range of 0-5
- Returns
- None
- Description:
- Sets the interrupt node for protocol interrupt.
- To generate interrupt for an event, node pointer should be configured with service request number(SR0, SR1..SR5). The NVIC node gets linked to the interrupt event by doing so.
- Note:
- NVIC node should be separately enabled to generate the interrupt. After setting the node pointer, desired event must be enabled.
- Related APIs:
- XMC_I2C_CH_EnableEvent(), NVIC_SetPriority(), NVIC_EnableIRQ(), XMC_I2C_CH_SetInputSource()
void XMC_I2C_CH_SetSlaveAddress | ( | XMC_USIC_CH_t *const | channel, |
const uint16_t | address | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t address I2C slave address
- Returns
- None
- Description:
- Sets the I2C channel slave address.
- Address is set in PCR_IICMode register by checking if it is in 10-bit address group or 7-bit address group. (If first five bits of address are assigned with 0xF0, then address mode is 10-bit mode otherwise it is 7-bit mode)
- Note
- A 7-bit address should include an additional bit at the LSB for read/write indication. For example, address 0x05 should be provided as 0x0a. A 10-bit address should be provided with the identifier 0b11110xx at the most significant bits. For example, address 0x305 should be provided as 0x7b05(bitwise OR with 0x7800).
- Related APIs:
- XMC_I2C_CH_GetSlaveAddress()
void XMC_I2C_CH_SlaveTransmit | ( | XMC_USIC_CH_t *const | channel, |
const uint8_t | data | ||
) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t data data to transmit from I2C channel
- Returns
- None
- Description:
- Transmit the data from the I2C slave channel.
- Reads the transmit buffer status is busy or not and thereby updates IN/TBUF register based on FIFO/non-FIFO modes using Slave Send command.
void XMC_I2C_CH_Start | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- None
- Description:
- Starts the I2C channel.
- Sets the USIC input operation mode to I2C mode using CCR register.
- Related APIs:
- XMC_USIC_CH_SetMode()
XMC_I2C_CH_STATUS_t XMC_I2C_CH_Stop | ( | XMC_USIC_CH_t *const | channel | ) |
- Parameters
-
channel Constant pointer to USIC channel structure of type XMC_USIC_CH_t
- Returns
- XMC_I2C_CH_STATUS_t
- Description:
- Stops the I2C channel.
- Sets the USIC input operation to IDLE mode using CCR register.
- Related APIs:
- XMC_USIC_CH_SetMode()
void XMC_I2C_CH_TriggerServiceRequest | ( | XMC_USIC_CH_t *const | channel, |
const uint32_t | service_request_line | ||
) |
- Parameters
-
channel Pointer to USIC channel handler of type XMC_USIC_CH_t
Range: XMC_I2C0_CH0, XMC_I2C0_CH1,XMC_I2C1_CH0,XMC_I2C1_CH1,XMC_I2C2_CH0,XMC_I2C2_CH1
- Note
- Availability of I2C1 and I2C2 depends on device selection
- Parameters
-
service_request_line service request number of the event to be triggered.
Range: 0 to 5.
- Returns
- None
- Description
- Trigger a I2C interrupt service request.
When the I2C service request is triggered, the NVIC interrupt associated with it will be generated if enabled.
- Related APIs:
- XMC_I2C_CH_SelectInterruptNodePointer()
Generated on Mon Aug 7 2017 11:33:57 for XMC Peripheral Library for XMC4000 Family by
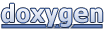