tonc_video.h File Reference
#include "tonc_memmap.h"
#include "tonc_memdef.h"
#include "tonc_core.h"
mode 3 | |
#define | M3_CLEAR() memset32(vid_mem, 0, M3_SIZE/4) |
Fill the mode 3 background with color clr. | |
INLINE void | m3_fill (COLOR clr) |
Fill the mode 3 background with color clr. | |
INLINE void | m3_plot (int x, int y, COLOR clr) |
Plot a single clr colored pixel in mode 3 at (x, y). | |
INLINE void | m3_hline (int x1, int y, int x2, COLOR clr) |
Draw a clr colored horizontal line in mode 3. | |
INLINE void | m3_vline (int x, int y1, int y2, COLOR clr) |
Draw a clr colored vertical line in mode 3. | |
INLINE void | m3_line (int x1, int y1, int x2, int y2, COLOR clr) |
Draw a clr colored line in mode 3. | |
INLINE void | m3_rect (int left, int top, int right, int bottom, COLOR clr) |
Draw a clr colored rectangle in mode 3. | |
INLINE void | m3_frame (int left, int top, int right, int bottom, COLOR clr) |
Draw a clr colored frame in mode 3. | |
mode 4 | |
#define | M4_CLEAR() memset32(vid_page, 0, M4_SIZE/4) |
Fill the current mode 4 backbuffer with clrid. | |
INLINE void | m4_fill (u8 clrid) |
Fill the current mode 4 backbuffer with clrid. | |
INLINE void | m4_plot (int x, int y, u8 clrid) |
Plot a clrid pixel on the current mode 4 backbuffer. | |
INLINE void | m4_hline (int x1, int y, int x2, u8 clrid) |
Draw a clrid colored horizontal line in mode 4. | |
INLINE void | m4_vline (int x, int y1, int y2, u8 clrid) |
Draw a clrid colored vertical line in mode 4. | |
INLINE void | m4_line (int x1, int y1, int x2, int y2, u8 clrid) |
Draw a clrid colored line in mode 4. | |
INLINE void | m4_rect (int left, int top, int right, int bottom, u8 clrid) |
Draw a clrid colored rectangle in mode 4. | |
INLINE void | m4_frame (int left, int top, int right, int bottom, u8 clrid) |
Draw a clrid colored frame in mode 4. | |
mode 5 | |
#define | M5_CLEAR() memset32(vid_page, 0, M5_SIZE/4) |
Fill the current mode 5 backbuffer with clr. | |
INLINE void | m5_fill (COLOR clr) |
Fill the current mode 5 backbuffer with clr. | |
INLINE void | m5_plot (int x, int y, COLOR clr) |
Plot a clrid pixel on the current mode 5 backbuffer. | |
INLINE void | m5_hline (int x1, int y, int x2, COLOR clr) |
Draw a clr colored horizontal line in mode 5. | |
INLINE void | m5_vline (int x, int y1, int y2, COLOR clr) |
Draw a clr colored vertical line in mode 5. | |
INLINE void | m5_line (int x1, int y1, int x2, int y2, COLOR clr) |
Draw a clr colored line in mode 5. | |
INLINE void | m5_rect (int left, int top, int right, int bottom, COLOR clr) |
Draw a clr colored rectangle in mode 5. | |
INLINE void | m5_frame (int left, int top, int right, int bottom, COLOR clr) |
Draw a clr colored frame in mode 5. | |
Defines | |
#define | SCREEN_WIDTH 240 |
#define | SCREEN_HEIGHT 160 |
#define | M3_WIDTH SCREEN_WIDTH |
#define | M3_HEIGHT SCREEN_HEIGHT |
#define | M4_WIDTH SCREEN_WIDTH |
#define | M4_HEIGHT SCREEN_HEIGHT |
#define | M5_WIDTH 160 |
#define | M5_HEIGHT 128 |
#define | SCREEN_WIDTH_T (SCREEN_WIDTH/8) |
#define | SCREEN_HEIGHT_T (SCREEN_HEIGHT/8) |
#define | SCREEN_LINES 228 |
#define | SCR_W SCREEN_WIDTH |
#define | SCR_H SCREEN_HEIGHT |
#define | SCR_WT SCREEN_WIDTH_T |
#define | SCR_HT SCREEN_HEIGHT_T |
#define | LAYER_BG0 0x0001 |
#define | LAYER_BG1 0x0002 |
#define | LAYER_BG2 0x0004 |
#define | LAYER_BG3 0x0008 |
#define | LAYER_OBJ 0x0010 |
#define | LAYER_BD 0x0020 |
#define | CLR_MASK 0x001F |
#define | RED_MASK 0x001F |
#define | RED_SHIFT 0 |
#define | GREEN_MASK 0x03E0 |
#define | GREEN_SHIFT 5 |
#define | BLUE_MASK 0x7C00 |
#define | BLUE_SHIFT 10 |
#define | CBB_CLEAR(cbb) memset32(&tile_mem[cbb], 0, CBB_SIZE/4) |
#define | SBB_CLEAR(sbb) memset32(&se_mem[sbb], 0, SBB_SIZE/4) |
#define | SBB_CLEAR_ROW(sbb, row) memset32(&se_mem[sbb][(row)*32], 0, 32/2) |
#define | __BG_TYPES ((0x0C7F<<16)|(0x0C40)) |
#define | BG_IS_AFFINE(n) ( (__BG_TYPES>>(4*(REG_DISPCNT&7)+(n) ))&1 ) |
#define | BG_IS_AVAIL(n) ( (__BG_TYPES>>(4*(REG_DISPCNT&7)+(n)+16))&1 ) |
Base Color constants | |
#define | CLR_BLACK 0x0000 |
#define | CLR_RED 0x001F |
#define | CLR_LIME 0x03E0 |
#define | CLR_YELLOW 0x03FF |
#define | CLR_BLUE 0x7C00 |
#define | CLR_MAG 0x7C1F |
#define | CLR_CYAN 0x7FE0 |
#define | CLR_WHITE 0x7FFF |
Additional colors | |
#define | CLR_DEAD 0xDEAD |
#define | CLR_MAROON 0x0010 |
#define | CLR_GREEN 0x0200 |
#define | CLR_OLIVE 0x0210 |
#define | CLR_ORANGE 0x021F |
#define | CLR_NAVY 0x4000 |
#define | CLR_PURPLE 0x4010 |
#define | CLR_TEAL 0x4200 |
#define | CLR_GRAY 0x4210 |
#define | CLR_MEDGRAY 0x5294 |
#define | CLR_SILVER 0x6318 |
#define | CLR_MONEYGREEN 0x6378 |
#define | CLR_FUCHSIA 0x7C1F |
#define | CLR_SKYBLUE 0x7B34 |
#define | CLR_CREAM 0x7BFF |
Functions | |
INLINE void | vid_vsync (void) |
void | vid_wait (uint frames) |
u16 * | vid_flip (void) |
Flip the display page. | |
void | clr_rotate (COLOR *clrs, uint nclrs, int ror) |
Rotate nclrs colors at clrs to the right by ror. | |
void | clr_blend (const COLOR *srca, const COLOR *srcb, COLOR *dst, u32 nclrs, u32 alpha) |
Blends color arrays srca and srcb into dst. | |
void | clr_fade (const COLOR *src, COLOR clr, COLOR *dst, u32 nclrs, u32 alpha) |
Fades color arrays srca to clr into dst. | |
void | clr_grayscale (COLOR *dst, const COLOR *src, uint nclrs) |
Transform colors to grayscale. | |
void | clr_rgbscale (COLOR *dst, const COLOR *src, uint nclrs, COLOR clr) |
Transform colors to an rgb-scale. | |
void | clr_adj_brightness (COLOR *dst, const COLOR *src, uint nclrs, FIXED bright) |
Adjust brightness by bright. | |
void | clr_adj_contrast (COLOR *dst, const COLOR *src, uint nclrs, FIXED contrast) |
Adjust contrast by contrast. | |
void | clr_adj_intensity (COLOR *dst, const COLOR *src, uint nclrs, FIXED intensity) |
Adjust intensity by intensity. | |
void | pal_gradient (COLOR *pal, int first, int last) |
Create a gradient between pal[first] and pal[last]. | |
void | pal_gradient_ex (COLOR *pal, int first, int last, COLOR clr_first, COLOR clr_last) |
Create a gradient between pal[first] and pal[last]. | |
IWRAM_CODE void | clr_blend_fast (COLOR *srca, COLOR *srcb, COLOR *dst, uint nclrs, u32 alpha) |
Blends color arrays srca and srcb into dst. | |
IWRAM_CODE void | clr_fade_fast (COLOR *src, COLOR clr, COLOR *dst, uint nclrs, u32 alpha) |
Fades color arrays srca to clr into dst. | |
INLINE COLOR | RGB15 (int red, int green, int blue) |
Create a 15bit BGR color. | |
INLINE COLOR | RGB15_SAFE (int red, int green, int blue) |
Create a 15bit BGR color, with proper masking of R,G,B components. | |
INLINE COLOR | RGB8 (u8 red, u8 green, u8 blue) |
Create a 15bit BGR color, using 8bit components. | |
INLINE void | se_fill (SCR_ENTRY *sbb, SCR_ENTRY se) |
Fill screenblock sbb with se. | |
INLINE void | se_plot (SCR_ENTRY *sbb, int x, int y, SCR_ENTRY se) |
Plot a screen entry at (x,y) of screenblock sbb. | |
INLINE void | se_rect (SCR_ENTRY *sbb, int left, int top, int right, int bottom, SCR_ENTRY se) |
Fill a rectangle on sbb with se. | |
INLINE void | se_frame (SCR_ENTRY *sbb, int left, int top, int right, int bottom, SCR_ENTRY se) |
Create a border on sbb with se. | |
void | se_window (SCR_ENTRY *sbb, int left, int top, int right, int bottom, SCR_ENTRY se0) |
Create a framed rectangle. | |
void | se_hline (SCR_ENTRY *sbb, int x0, int x1, int y, SCR_ENTRY se) |
void | se_vline (SCR_ENTRY *sbb, int x, int y0, int y1, SCR_ENTRY se) |
INLINE void | bg_aff_set (BG_AFFINE *bgaff, FIXED pa, FIXED pb, FIXED pc, FIXED pd) |
Set the elements of an bg affine matrix. | |
INLINE void | bg_aff_identity (BG_AFFINE *bgaff) |
Set an bg affine matrix to the identity matrix. | |
INLINE void | bg_aff_scale (BG_AFFINE *bgaff, FIXED sx, FIXED sy) |
Set an bg affine matrix for scaling. | |
INLINE void | bg_aff_shearx (BG_AFFINE *bgaff, FIXED hx) |
INLINE void | bg_aff_sheary (BG_AFFINE *bgaff, FIXED hy) |
void | bg_aff_rotate (BG_AFFINE *bgaff, u16 alpha) |
Set bg matrix to counter-clockwise rotation. | |
void | bg_aff_rotscale (BG_AFFINE *bgaff, int sx, int sy, u16 alpha) |
Set bg matrix to 2d scaling, then counter-clockwise rotation. | |
void | bg_aff_premul (BG_AFFINE *dst, const BG_AFFINE *src) |
Pre-multiply dst by src: D = S*D. | |
void | bg_aff_postmul (BG_AFFINE *dst, const BG_AFFINE *src) |
Post-multiply dst by src: D= D*S. | |
void | bg_aff_rotscale2 (BG_AFFINE *bgaff, const AFF_SRC *as) |
Set bg matrix to 2d scaling, then counter-clockwise rotation. | |
void | bg_rotscale_ex (BG_AFFINE *bgaff, const AFF_SRC_EX *asx) |
Set bg affine matrix to a rot/scale around an arbitrary point. | |
INLINE void | bg_aff_copy (BG_AFFINE *dst, const BG_AFFINE *src) |
Copy bg affine aprameters. | |
Generic 8bpp bitmaps | |
void | bmp8_plot (int x, int y, u32 clr, void *dstBase, uint dstP) |
Plot a single pixel on a 8-bit buffer. | |
void | bmp8_hline (int x1, int y, int x2, u32 clr, void *dstBase, uint dstP) |
Draw a horizontal line on an 8bit buffer. | |
void | bmp8_vline (int x, int y1, int y2, u32 clr, void *dstBase, uint dstP) |
Draw a vertical line on an 8bit buffer. | |
void | bmp8_line (int x1, int y1, int x2, int y2, u32 clr, void *dstBase, uint dstP) |
Draw a line on an 8bit buffer. | |
void | bmp8_rect (int left, int top, int right, int bottom, u32 clr, void *dstBase, uint dstP) |
Draw a rectangle in 8bit mode; internal routine. | |
void | bmp8_frame (int left, int top, int right, int bottom, u32 clr, void *dstBase, uint dstP) |
Draw a rectangle in 8bit mode; internal routine. | |
Generic 16bpp bitmaps | |
void | bmp16_plot (int x, int y, u32 clr, void *dstBase, uint dstP) |
Plot a single pixel on a 16-bit buffer. | |
void | bmp16_hline (int x1, int y, int x2, u32 clr, void *dstBase, uint dstP) |
Draw a horizontal line on an 16bit buffer. | |
void | bmp16_vline (int x, int y1, int y2, u32 clr, void *dstBase, uint dstP) |
Draw a vertical line on an 16bit buffer. | |
void | bmp16_line (int x1, int y1, int x2, int y2, u32 clr, void *dstBase, uint dstP) |
Draw a line on an 16bit buffer. | |
void | bmp16_rect (int left, int top, int right, int bottom, u32 clr, void *dstBase, uint dstP) |
Draw a rectangle in 16bit mode; internal routine. | |
void | bmp16_frame (int left, int top, int right, int bottom, u32 clr, void *dstBase, uint dstP) |
Draw a rectangle in 16bit mode; internal routine. |
Detailed Description
- Author:
- J Vijn
- Date:
- 20060604 - 20080311
Function Documentation
u16* vid_flip | ( | void | ) |
Flip the display page.
Toggles the display page in REG_DISPCNT and sets vid_page to point to the back buffer.
- Returns:
- Current back buffer pointer.
Generated on Mon Aug 25 17:03:56 2008 for libtonc by
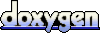