tonc_math.h File Reference
#include "tonc_types.h"
Data Structures | |
struct | POINT32 |
2D Point struct More... | |
struct | RECT32 |
Rectangle struct. More... | |
struct | VECTOR |
Vector struct. More... | |
core math macros | |
#define | ABS(x) ( (x)>=0 ? (x) : -(x) ) |
Get the absolute value of x. | |
#define | SGN(x) ( (x)>=0 ? 1 : -1 ) |
Get the sign of x. | |
#define | SGN2 SGN |
Get the absolute value of x. | |
#define | SGN3(x) ( (x)>0 ? 1 : ( (x)<0 ? -1 : 0) ) |
Tri-state sign: -1 for negative, 0 for 0, +1 for positive. | |
#define | MAX(a, b) ( ((a) > (b)) ? (a) : (b) ) |
Get the maximum of a and b. | |
#define | MIN(a, b) ( ((a) < (b)) ? (a) : (b) ) |
Get the minimum of a and b. | |
#define | SWAP2(a, b) do { a=(a)-(b); b=(a)+(b); a=(b)-(a); } while(0) |
In-place swap. | |
#define | SWAP SWAP2 |
Get the absolute value of x. | |
#define | SWAP3(a, b, tmp) do { (tmp)=(a); (a)=(b); (b)=(tmp); } while(0) |
Swaps a and b, using tmp as a temporary. | |
INLINE int | sgn (int x) |
Get the sign of x. | |
INLINE int | sgn3 (int x) |
Tri-state sign of x: -1 for negative, 0 for 0, +1 for positive. | |
INLINE int | max (int a, int b) |
Get the maximum of a and b. | |
INLINE int | min (int a, int b) |
Get the minimum of a and b. | |
Boundary response macros | |
#define | IN_RANGE(x, min, max) ( ((x)>=(min)) && ((x)<(max)) ) |
Range check. | |
#define | CLAMP(x, min, max) ( (x)>=(max) ? ((max)-1) : ( ((x)<(min)) ? (min) : (x) ) ) |
Truncates x to stay in range [min, max>. | |
#define | REFLECT(x, min, max) ( (x)>=(max) ? 2*((max)-1)-(x) : ( ((x)<(min)) ? 2*(min)-(x) : (x) ) ) |
Reflects x at boundaries min and max. | |
#define | WRAP(x, min, max) ( (x)>=(max) ? (x)+(min)-(max) : ( ((x)<(min)) ? (x)+(max)-(min) : (x) ) ) |
Wraps x to stay in range [min, max>. | |
INLINE BOOL | in_range (int x, int min, int max) |
Range check. | |
INLINE int | clamp (int x, int min, int max) |
Truncates x to stay in range [min, max>. | |
INLINE int | reflect (int x, int min, int max) |
Reflects x at boundaries min and max. | |
INLINE int | wrap (int x, int min, int max) |
Wraps x to stay in range [min, max>. | |
Defines | |
#define | FIX_SHIFT 8 |
#define | FIX_SCALE ( 1<<FIX_SHIFT ) |
#define | FIX_MASK ( FIX_SCALE-1 ) |
#define | FIX_SCALEF ( (float)FIX_SCALE ) |
#define | FIX_SCALEF_INV ( 1.0/FIX_SCALEF ) |
#define | FIX_ONE FIX_SCALE |
#define | FX_RECIPROCAL(a, fp) ( ((1<<(fp))+(a)-1)/(a) ) |
Get the fixed point reciprocal of a, in fp fractional bits. | |
#define | FX_RECIMUL(x, a, fp) ( ((x)*((1<<(fp))+(a)-1)/(a))>>(fp) ) |
Perform the division x/ a by reciprocal multiplication. | |
#define | SIN_LUT_SIZE 514 |
#define | DIV_LUT_SIZE 257 |
Functions | |
INLINE FIXED | int2fx (int d) |
Convert an integer to fixed-point. | |
INLINE FIXED | float2fx (float f) |
Convert a float to fixed-point. | |
INLINE u32 | fx2uint (FIXED fx) |
Convert a FIXED point value to an unsigned integer (orly?). | |
INLINE u32 | fx2ufrac (FIXED fx) |
Get the unsigned fractional part of a fixed point value (orly?). | |
INLINE int | fx2int (FIXED fx) |
Convert a FIXED point value to an signed integer. | |
INLINE float | fx2float (FIXED fx) |
Convert a fixed point value to floating point. | |
INLINE FIXED | fxadd (FIXED fa, FIXED fb) |
Add two fixed point values. | |
INLINE FIXED | fxsub (FIXED fa, FIXED fb) |
Subtract two fixed point values. | |
INLINE FIXED | fxmul (FIXED fa, FIXED fb) |
Multiply two fixed point values. | |
INLINE FIXED | fxdiv (FIXED fa, FIXED fb) |
Divide two fixed point values. | |
INLINE FIXED | fxmul64 (FIXED fa, FIXED fb) |
Multiply two fixed point values using 64bit math. | |
INLINE FIXED | fxdiv64 (FIXED fa, FIXED fb) |
Divide two fixed point values using 64bit math. | |
INLINE s32 | lu_sin (uint theta) |
Look-up a sine value (2π = 0x10000). | |
INLINE s32 | lu_cos (uint theta) |
Look-up a cosine value (2π = 0x10000). | |
INLINE uint | lu_div (uint x) |
Look-up a division value between 0 and 255. | |
INLINE int | lu_lerp32 (const s32 lut[], uint x, const uint shift) |
Linear interpolator for 32bit LUTs. | |
INLINE int | lu_lerp16 (const s16 lut[], uint x, const uint shift) |
As lu_lerp32, but for 16bit LUTs. | |
INLINE POINT * | pt_set (POINT *pd, int x, int y) |
Initialize pd to (x, y). | |
INLINE POINT * | pt_add (POINT *pd, const POINT *pa, const POINT *pb) |
Point addition: pd = pa + pb. | |
INLINE POINT * | pt_sub (POINT *pd, const POINT *pa, const POINT *pb) |
Point subtraction: pd = pa - pb. | |
INLINE POINT * | pt_scale (POINT *pd, const POINT *pa, int c) |
Point scale: pd = c * pa. | |
INLINE POINT * | pt_add_eq (POINT *pd, const POINT *pb) |
Point increment: pd += pb. | |
INLINE POINT * | pt_sub_eq (POINT *pd, const POINT *pb) |
Point decrement: pd -= pb. | |
INLINE POINT * | pt_scale_eq (POINT *pd, int c) |
Point scale: pd *= c. | |
INLINE int | pt_cross (const POINT *pa, const POINT *pb) |
Point 'cross'-product: pa × pb. | |
INLINE int | pt_dot (const POINT *pa, const POINT *pb) |
Point 'dot'-product:pa · pb. | |
int | pt_in_rect (const POINT *pt, const struct RECT *rc) |
INLINE RECT * | rc_set (RECT *rc, int l, int t, int r, int b) |
Initialize a rectangle. | |
INLINE RECT * | rc_set2 (RECT *rc, int x, int y, int w, int h) |
Initialize a rectangle, with sizes inside of max boundaries. | |
INLINE int | rc_width (const RECT *rc) |
Get rectangle width. | |
INLINE int | rc_height (const RECT *rc) |
Get rectangle height. | |
INLINE RECT * | rc_set_pos (RECT *rc, int x, int y) |
Move rectangle to (x, y) position. | |
INLINE RECT * | rc_set_size (RECT *rc, int w, int h) |
Reside rectangle. | |
INLINE RECT * | rc_move (RECT *rc, int dx, int dy) |
Move rectangle by (dx, dy). | |
INLINE RECT * | rc_inflate (RECT *rc, int dw, int dh) |
Increase size by dw horizontally and dh vertically. | |
INLINE RECT * | rc_inflate2 (RECT *rc, const RECT *dr) |
Increase sizes on all sides by values of rectangle dr. | |
RECT * | rc_normalize (RECT *rc) |
INLINE VECTOR * | vec_set (VECTOR *vd, FIXED x, FIXED y, FIXED z) |
Initialize a vector. | |
INLINE VECTOR * | vec_add (VECTOR *vd, const VECTOR *va, const VECTOR *vb) |
Add vectors: d = a + b;. | |
INLINE VECTOR * | vec_sub (VECTOR *vd, const VECTOR *va, const VECTOR *vb) |
Subtract vectors: d = a - b;. | |
INLINE VECTOR * | vec_mul (VECTOR *vd, const VECTOR *va, const VECTOR *vb) |
Multiply vectors elements: d = S(ax, ay, az) �b. | |
INLINE VECTOR * | vec_scale (VECTOR *vd, const VECTOR *va, FIXED c) |
Scale vector: d = c*a. | |
INLINE FIXED | vec_dot (const VECTOR *va, const VECTOR *vb) |
Dot-product: d = a �b. | |
INLINE VECTOR * | vec_add_eq (VECTOR *vd, const VECTOR *vb) |
Increment vector: d += b;. | |
INLINE VECTOR * | vec_sub_eq (VECTOR *vd, const VECTOR *vb) |
Decrease vector: d -= b;. | |
INLINE VECTOR * | vec_mul_eq (VECTOR *vd, const VECTOR *vb) |
Multiply vectors elements: d = S(dx, dy, dz) �b. | |
INLINE VECTOR * | vec_scale_eq (VECTOR *vd, FIXED c) |
Scale vector: d = c*d. | |
VECTOR * | vec_cross (VECTOR *vd, const VECTOR *va, const VECTOR *vb) |
Variables | |
s32 | div_lut [257] |
s16 | sin_lut [514] |
Detailed Description
- Author:
- J Vijn
- Date:
- 20060508 - 20060908
Generated on Mon Aug 25 17:03:56 2008 for libtonc by
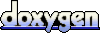