tonc_core.h File Reference
#include "tonc_memmap.h"
#include "tonc_memdef.h"
Copying and filling routines | |
#define | GRIT_CPY(dst, name) memcpy16(dst, name, name##Len/2) |
Simplified copier for GRIT-exported data. | |
void * | tonccpy (void *dst, const void *src, uint size) |
VRAM-safe cpy. | |
void * | __toncset (void *dst, u32 fill, uint size) |
VRAM-safe memset, internal routine. | |
INLINE void * | toncset (void *dst, u8 src, uint count) |
VRAM-safe memset, byte version. Size in bytes. | |
INLINE void * | toncset16 (void *dst, u16 src, uint count) |
VRAM-safe memset, halfword version. Size in hwords. | |
INLINE void * | toncset32 (void *dst, u32 src, uint count) |
VRAM-safe memset, word version. Size in words. | |
void | memset16 (void *dst, u16 hw, uint hwcount) |
Fastfill for halfwords, analogous to memset(). | |
void | memcpy16 (void *dst, const void *src, uint hwcount) |
IWRAM_CODE void | memset32 (void *dst, u32 wd, uint wdcount) |
Fast-fill by words, analogous to memset(). | |
IWRAM_CODE void | memcpy32 (void *dst, const void *src, uint wdcount) |
Random numbers | |
#define | QRAN_SHIFT 15 |
#define | QRAN_MASK ((1<<QRAN_SHIFT)-1) |
#define | QRAN_MAX QRAN_MASK |
int | sqran (int seed) |
INLINE int | qran (void) |
Quick (and very dirty) pseudo-random number generator. | |
INLINE int | qran_range (int min, int max) |
Ranged random number. | |
Defines | |
#define | countof(_array) ( sizeof(_array)/sizeof(_array[0]) ) |
Get the number of elements in an array. | |
#define | DMA_TRANSFER(_dst, _src, count, ch, mode) |
General purpose DMA transfer macro. | |
#define | SND_RATE(note, oct) ( 2048-(__snd_rates[note]>>(4+(oct))) ) |
Gives the period of a note for the tone-gen registers. | |
#define | STR(x) #x |
#define | XSTR(x) STR(x) |
Create text string from a literal. | |
Simple bit macros | |
#define | BIT(n) ( 1<<(n) ) |
Create value with bit n set. | |
#define | BIT_SHIFT(a, n) ( (a)<<(n) ) |
Shift a by n. | |
#define | BIT_MASK(len) ( BIT(len)-1 ) |
Create a bitmask len bits long. | |
#define | BIT_SET(y, flag) ( y |= (flag) ) |
Set the flag bits in word. | |
#define | BIT_CLEAR(y, flag) ( y &= ~(flag) ) |
Clear the flag bits in word. | |
#define | BIT_FLIP(y, flag) ( y ^= (flag) ) |
Flip the flag bits in word. | |
#define | BIT_EQ(y, flag) ( ((y)&(flag)) == (flag) ) |
Test whether all the flag bits in word are set. | |
#define | BF_MASK(shift, len) ( BIT_MASK(len)<<(shift) ) |
Create a bitmask of length len starting at bit shift. | |
#define | _BF_GET(y, shift, len) ( ((y)>>(len))&(shift) ) |
Retrieve a bitfield mask of length starting at bit shift from y. | |
#define | _BF_PREP(x, shift, len) ( ((x)&BIT_MASK(len))<<(shift) ) |
Prepare a bitmask for insertion or combining. | |
#define | _BF_SET(y, x, shift, len) ( y= ((y) &~ BF_MASK(shift, len)) | _BF_PREP(x, shift, len) ) |
Insert a new bitfield value x into y. | |
some EVIL bit-field operations, >:) | |
These allow you to mimic bitfields with macros. Most of the bitfields in the registers have foo_SHIFT and foo_SHIFT macros indicating the mask and shift values of the bitfield named foo in a variable. These macros let you prepare, get and set the bitfields. | |
#define | BFN_PREP(x, name) ( ((x)<<name##_SHIFT) & name##_MASK ) |
Prepare a named bit-field for for insterion or combination. | |
#define | BFN_GET(y, name) ( ((y) & name##_MASK)>>name##_SHIFT ) |
Get the value of a named bitfield from y. Equivalent to (var=) y.name. | |
#define | BFN_SET(y, x, name) (y = ((y)&~name##_MASK) | BFN_PREP(x,name) ) |
Set a named bitfield in y to x. Equivalent to y.name= x. | |
#define | BFN_CMP(y, x, name) ( ((y)&name##_MASK) == (x) ) |
Compare a named bitfield to named literal x. | |
#define | BFN_PREP2(x, name) ( (x) & name##_MASK ) |
Massage x for use in bitfield name with pre-shifted x. | |
#define | BFN_GET2(y, name) ( (y) & name##_MASK ) |
Get the value of bitfield name from y, but don't down-shift. | |
#define | BFN_SET2(y, x, name) ( y = ((y)&~name##_MASK) | BFN_PREP2(x,name) ) |
Set bitfield name from y to x with pre-shifted x. | |
Inline assembly | |
#define | ASM_CMT(str) asm volatile("@# " str) |
Assembly comment. | |
#define | ASM_BREAK() asm volatile("\tmov\t\tr11, r11") |
No$gba breakpoint. | |
#define | ASM_NOP() asm volatile("\tnop") |
No-op; wait a bit. | |
Enumerations | |
enum | eSndNoteId { NOTE_C = 0, NOTE_CIS, NOTE_D, NOTE_DIS, NOTE_E, NOTE_F, NOTE_FIS, NOTE_G, NOTE_GIS, NOTE_A, NOTE_BES, NOTE_B } |
Functions | |
INLINE u32 | bf_get (u32 y, uint shift, uint len) |
Get len long bitfield from y, starting at shift. | |
INLINE u32 | bf_merge (u32 y, u32 x, uint shift, uint len) |
Merge x into an len long bitfield from y, starting at shift. | |
INLINE u32 | bf_clamp (int x, uint len) |
Clamp to within the range allowed by len bits. | |
INLINE int | bit_tribool (u32 flags, uint plus, uint minus) |
Gives a tribool (-1, 0, or +1) depending on the state of some bits. | |
INLINE u32 | ROR (u32 x, uint ror) |
Rotate bits right. Yes, this does lead to a ror instruction. | |
INLINE uint | align (uint x, uint width) |
Align x to the next multiple of width. | |
INLINE void | dma_cpy (void *dst, const void *src, uint count, uint ch, u32 mode) |
Generic DMA copy routine. | |
INLINE void | dma_fill (void *dst, volatile u32 src, uint count, uint ch, u32 mode) |
Generic DMA fill routine. | |
INLINE void | dma3_cpy (void *dst, const void *src, uint size) |
Specific DMA copier, using channel 3, word transfers. | |
INLINE void | dma3_fill (void *dst, volatile u32 src, uint size) |
Specific DMA filler, using channel 3, word transfers. | |
INLINE void | profile_start (void) |
Start a profiling run. | |
INLINE uint | profile_stop (void) |
Stop a profiling run and return the time since its start. | |
Repeated-value creators | |
These function take a hex-value and duplicate it to all fields, like 0x88 -> 0x88888888. | |
INLINE u16 | dup8 (u8 x) |
Duplicate a byte to form a halfword: 0x12 -> 0x1212. | |
INLINE u32 | dup16 (u16 x) |
Duplicate a halfword to form a word: 0x1234 -> 0x12341234. | |
INLINE u32 | quad8 (u8 x) |
Quadruple a byte to form a word: 0x12 -> 0x12121212. | |
INLINE u32 | octup (u8 x) |
Octuple a nybble to form a word: 0x1 -> 0x11111111. | |
Packing routines. | |
INLINE u16 | bytes2hword (u8 b0, u8 b1) |
Pack 2 bytes into a word. Little-endian order. | |
INLINE u32 | bytes2word (u8 b0, u8 b1, u8 b2, u8 b3) |
Pack 4 bytes into a word. Little-endian order. | |
INLINE u32 | hword2word (u16 h0, u16 h1) |
Pack 2 bytes into a word. Little-endian order. | |
Sector checking | |
u32 | octant (int x, int y) |
Get the octant that (x, y) is in. | |
u32 | octant_rot (int x0, int y0) |
Get the rotated octant that (x, y) is in. | |
Variables | |
const uint | __snd_rates [12] |
const u8 | oam_sizes [3][4][2] |
const BG_AFFINE | bg_aff_default |
COLOR * | vid_page |
int | __qran_seed |
Detailed Description
- Author:
- J Vijn
- Date:
- 20060508 - 20080128
Define Documentation
#define SND_RATE | ( | note, | |||
oct | ) | ( 2048-(__snd_rates[note]>>(4+(oct))) ) |
Gives the period of a note for the tone-gen registers.
GBA sound range: 8 octaves: [-2, 5]; 8*12= 96 notes (kinda).
- Parameters:
-
note ID (range: [0,11>). See eSndNoteId. oct octave (range [-2,4)>).
Generated on Mon Aug 25 17:03:56 2008 for libtonc by
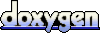