tonc_surface.h File Reference
#include "tonc_memmap.h"
#include "tonc_core.h"
Typedefs | |
Rendering procedure types | |
typedef u32(* | fnGetPixel )(const TSurface *src, int x, int y) |
typedef void(* | fnPlot )(const TSurface *dst, int x, int y, u32 clr) |
typedef void(* | fnHLine )(const TSurface *dst, int x1, int y, int x2, u32 clr) |
typedef void(* | fnVLine )(const TSurface *dst, int x, int y1, int y2, u32 clr) |
typedef void(* | fnLine )(const TSurface *dst, int x1, int y1, int x2, int y2, u32 clr) |
typedef void(* | fnRect )(const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
typedef void(* | fnFrame )(const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
typedef void(* | fnBlit )(const TSurface *dst, int dstX, int dstY, uint width, uint height, const TSurface *src, int srcX, int srcY) |
typedef void(* | fnFlood )(const TSurface *dst, int x, int y, u32 clr) |
Enumerations | |
enum | ESurfaceType { SRF_NONE = 0, SRF_BMP16 = 1, SRF_BMP8 = 2, SRF_CHR4R = 4, SRF_CHR4C = 5, SRF_CHR8 = 6, SRF_ALLOCATED = 0x80 } |
Surface types. More... | |
Functions | |
void | srf_init (TSurface *srf, enum ESurfaceType type, const void *data, uint width, uint height, uint bpp, u16 *pal) |
Initalize a surface for type formatted graphics. | |
void | srf_pal_copy (const TSurface *dst, const TSurface *src, uint count) |
Copy count colors from src's palette to dst's palette. | |
void * | srf_get_ptr (const TSurface *srf, uint x, uint y) |
Get the byte address of coordinates (x, y) on the surface. | |
INLINE uint | srf_align (uint width, uint bpp) |
Get the word-aligned number of bytes for a scanline. | |
INLINE void | srf_set_ptr (TSurface *srf, const void *ptr) |
Set Data-pointer surface for srf. | |
INLINE void | srf_set_pal (TSurface *srf, const u16 *pal, uint size) |
Set the palette pointer and its size. | |
INLINE void * | _srf_get_ptr (const TSurface *srf, uint x, uint y, uint stride) |
Inline and semi-safe version of srf_get_ptr(). Use with caution. | |
u32 | sbmp16_get_pixel (const TSurface *src, int x, int y) |
Get the pixel value of src at (x, y). | |
void | sbmp16_plot (const TSurface *dst, int x, int y, u32 clr) |
Plot a single pixel on a 16-bit buffer. | |
void | sbmp16_hline (const TSurface *dst, int x1, int y, int x2, u32 clr) |
Draw a horizontal line on an 16bit buffer. | |
void | sbmp16_vline (const TSurface *dst, int x, int y1, int y2, u32 clr) |
Draw a vertical line on an 16bit buffer. | |
void | sbmp16_line (const TSurface *dst, int x1, int y1, int x2, int y2, u32 clr) |
Draw a line on an 16bit buffer. | |
void | sbmp16_rect (const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
Draw a rectangle in 16bit mode. | |
void | sbmp16_frame (const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
Draw a rectangle in 16bit mode. | |
void | sbmp16_blit (const TSurface *dst, int dstX, int dstY, uint width, uint height, const TSurface *src, int srcX, int srcY) |
16bpp blitter. Copies a rectangle from one surface to another. | |
void | sbmp16_floodfill (const TSurface *dst, int x, int y, u32 clr) |
Floodfill an area of the same color with new color clr. | |
INLINE void | _sbmp16_plot (const TSurface *dst, int x, int y, u32 clr) |
Plot a single pixel on a 16-bit buffer; inline version. | |
INLINE u32 | _sbmp16_get_pixel (const TSurface *src, int x, int y) |
Get the pixel value of src at (x, y); inline version. | |
u32 | sbmp8_get_pixel (const TSurface *src, int x, int y) |
Get the pixel value of src at (x, y). | |
void | sbmp8_plot (const TSurface *dst, int x, int y, u32 clr) |
Plot a single pixel on a 8-bit buffer. | |
void | sbmp8_hline (const TSurface *dst, int x1, int y, int x2, u32 clr) |
Draw a horizontal line on an 8-bit buffer. | |
void | sbmp8_vline (const TSurface *dst, int x, int y1, int y2, u32 clr) |
Draw a vertical line on an 8-bit buffer. | |
void | sbmp8_line (const TSurface *dst, int x1, int y1, int x2, int y2, u32 clr) |
Draw a line on an 8-bit buffer. | |
void | sbmp8_rect (const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
Draw a rectangle in 8-bit mode. | |
void | sbmp8_frame (const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
Draw a rectangle in 8-bit mode. | |
void | sbmp8_blit (const TSurface *dst, int dstX, int dstY, uint width, uint height, const TSurface *src, int srcX, int srcY) |
16bpp blitter. Copies a rectangle from one surface to another. | |
void | sbmp8_floodfill (const TSurface *dst, int x, int y, u32 clr) |
Floodfill an area of the same color with new color clr. | |
INLINE void | _sbmp8_plot (const TSurface *dst, int x, int y, u32 clr) |
Plot a single pixel on a 8-bit surface; inline version. | |
INLINE u32 | _sbmp8_get_pixel (const TSurface *src, int x, int y) |
Get the pixel value of src at (x, y); inline version. | |
u32 | schr4c_get_pixel (const TSurface *src, int x, int y) |
Get the pixel value of src at (x, y). | |
void | schr4c_plot (const TSurface *dst, int x, int y, u32 clr) |
Plot a single pixel on a 4bpp tiled surface. | |
void | schr4c_hline (const TSurface *dst, int x1, int y, int x2, u32 clr) |
Draw a horizontal line on a 4bpp tiled surface. | |
void | schr4c_vline (const TSurface *dst, int x, int y1, int y2, u32 clr) |
Draw a vertical line on a 4bpp tiled surface. | |
void | schr4c_line (const TSurface *dst, int x1, int y1, int x2, int y2, u32 clr) |
Draw a line on a 4bpp tiled surface. | |
void | schr4c_rect (const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
Render a rectangle on a 4bpp tiled canvas. | |
void | schr4c_frame (const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
Draw a rectangle on a 4bpp tiled surface. | |
void | schr4c_blit (const TSurface *dst, int dstX, int dstY, uint width, uint height, const TSurface *src, int srcX, int srcY) |
Blitter for 4bpp tiled surfaces. Copies a rectangle from one surface to another. | |
void | schr4c_floodfill (const TSurface *dst, int x, int y, u32 clr) |
Floodfill an area of the same color with new color clr. | |
void | schr4c_prep_map (const TSurface *srf, u16 *map, u16 se0) |
Prepare a screen-entry map for use with chr4. | |
u32 * | schr4c_get_ptr (const TSurface *srf, int x, int y) |
Special pointer getter for chr4: start of in-tile line. | |
INLINE void | _schr4c_plot (const TSurface *dst, int x, int y, u32 clr) |
Plot a single pixel on a 4bpp tiled,col-jamor surface; inline version. | |
INLINE u32 | _schr4c_get_pixel (const TSurface *src, int x, int y) |
Get the pixel value of src at (x, y); inline version. | |
u32 | schr4r_get_pixel (const TSurface *src, int x, int y) |
Get the pixel value of src at (x, y). | |
void | schr4r_plot (const TSurface *dst, int x, int y, u32 clr) |
Plot a single pixel on a 4bpp tiled surface. | |
void | schr4r_hline (const TSurface *dst, int x1, int y, int x2, u32 clr) |
Draw a horizontal line on a 4bpp tiled surface. | |
void | schr4r_vline (const TSurface *dst, int x, int y1, int y2, u32 clr) |
Draw a vertical line on a 4bpp tiled surface. | |
void | schr4r_line (const TSurface *dst, int x1, int y1, int x2, int y2, u32 clr) |
Draw a line on a 4bpp tiled surface. | |
void | schr4r_rect (const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
Render a rectangle on a tiled canvas. | |
void | schr4r_frame (const TSurface *dst, int left, int top, int right, int bottom, u32 clr) |
Draw a rectangle on a 4bpp tiled surface. | |
void | schr4r_prep_map (const TSurface *srf, u16 *map, u16 se0) |
Prepare a screen-entry map for use with chr4. | |
u32 * | schr4r_get_ptr (const TSurface *srf, int x, int y) |
Special pointer getter for chr4: start of in-tile line. | |
INLINE void | _schr4r_plot (const TSurface *dst, int x, int y, u32 clr) |
Plot a single pixel on a 4bpp tiled,row-major surface; inline version. | |
INLINE u32 | _schr4r_get_pixel (const TSurface *src, int x, int y) |
Get the pixel value of src at (x, y); inline version. | |
Variables | |
const TSurface | m3_surface |
EWRAM_DATA TSurface | m4_surface |
EWRAM_DATA TSurface | m5_surface |
const TSurfaceProcTab | bmp16_tab |
const TSurfaceProcTab | bmp8_tab |
const TSurfaceProcTab | chr4c_tab |
Detailed Description
- Author:
- J Vijn
- Date:
- 20080119 - 20080514
Generated on Mon Aug 25 17:03:56 2008 for libtonc by
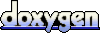